Invalid use of argument matchers 0 matches expected. 3 recorded
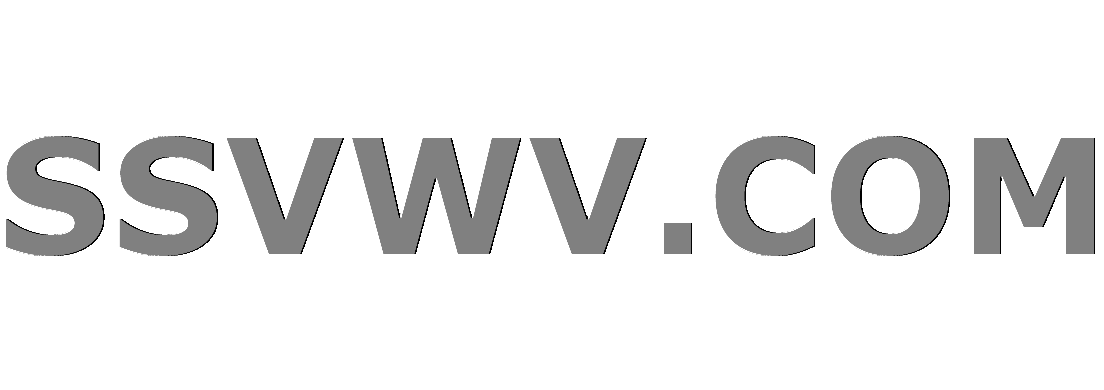
Multi tool use
up vote
0
down vote
favorite
I'm writing unit tests in scala using MockitoSugar. I'm using org.mockito.Mockito.when
and org.mockito.Matchers.anyString
. The method under test is shown below.
def getDataSourceToDataFrame(database: scala.Predef.String, tableName: scala.Predef.String): DataFrame = {
glueContext.getCatalogSource(
database = database,
tableName = tableName,
transformationContext = database + "." + tableName + ".read")
.getDynamicFrame()
.toDF()
}
Here is what my unit test looks like:
import com.amazon.rrsetlglue.glue.wrappers.WrappedGlueContext
import com.amazonaws.services.glue.{DynamicFrame, GlueContext, DataSource}
import org.scalatest.{BeforeAndAfter, FunSuite, Matchers}
import org.apache.spark.sql.DataFrame
import org.mockito.Mockito.when
import org.mockito.Matchers.anyString
import org.scalatest.junit.JUnitRunner
import org.junit.runner.RunWith
// Use Mockito because ScalaMock does not work with Glue
@RunWith(classOf[JUnitRunner])
class WrappedGlueContextTest extends FunSuite with
org.scalatest.mockito.MockitoSugar with BeforeAndAfter {
var mockGlueContext : GlueContext = _
var wrappedGlueContext: WrappedGlueContext = _
before {
mockGlueContext = mock[GlueContext]
wrappedGlueContext = new WrappedGlueContext(mockGlueContext)
}
test("Test get dataFrame from glue data catalog source") {
val mockedDataSource: DataSource= mock[DataSource]
val mockDynamicFrame: DynamicFrame = mock[DynamicFrame]
val mockDataFrame: DataFrame = mock[DataFrame]
assert(wrappedGlueContext.glueContext === mockGlueContext)
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString)).thenReturn(mockedDataSource)
}
}
The last when()
call throws the error below:
org.mockito.exceptions.misusing.InvalidUseOfMatchersException:
Invalid use of argument matchers!
0 matchers expected, 3 recorded:
This exception may occur if matchers are combined with raw values:
//incorrect:
someMethod(anyObject(), "raw String");
When using matchers, all arguments have to be provided by matchers.
For example:
//correct:
someMethod(anyObject(), eq("String by matcher"));
For more info see javadoc for Matchers class.
The definition for getCatalogSource()
is shown below
def getCatalogSource(database: String, tableName: String, redshiftTmpDir: String = "",
transformationContext: String = "", pushDownPredicate: String = "",
additionalOptions: JsonOptions = JsonOptions.empty, catalogId: String = null): DataSource = {
Why is it saying 0 matchers expected
when I'm calling the method getCatalogSource()
on a mocked object?
scala unit-testing mockito aws-glue
|
show 1 more comment
up vote
0
down vote
favorite
I'm writing unit tests in scala using MockitoSugar. I'm using org.mockito.Mockito.when
and org.mockito.Matchers.anyString
. The method under test is shown below.
def getDataSourceToDataFrame(database: scala.Predef.String, tableName: scala.Predef.String): DataFrame = {
glueContext.getCatalogSource(
database = database,
tableName = tableName,
transformationContext = database + "." + tableName + ".read")
.getDynamicFrame()
.toDF()
}
Here is what my unit test looks like:
import com.amazon.rrsetlglue.glue.wrappers.WrappedGlueContext
import com.amazonaws.services.glue.{DynamicFrame, GlueContext, DataSource}
import org.scalatest.{BeforeAndAfter, FunSuite, Matchers}
import org.apache.spark.sql.DataFrame
import org.mockito.Mockito.when
import org.mockito.Matchers.anyString
import org.scalatest.junit.JUnitRunner
import org.junit.runner.RunWith
// Use Mockito because ScalaMock does not work with Glue
@RunWith(classOf[JUnitRunner])
class WrappedGlueContextTest extends FunSuite with
org.scalatest.mockito.MockitoSugar with BeforeAndAfter {
var mockGlueContext : GlueContext = _
var wrappedGlueContext: WrappedGlueContext = _
before {
mockGlueContext = mock[GlueContext]
wrappedGlueContext = new WrappedGlueContext(mockGlueContext)
}
test("Test get dataFrame from glue data catalog source") {
val mockedDataSource: DataSource= mock[DataSource]
val mockDynamicFrame: DynamicFrame = mock[DynamicFrame]
val mockDataFrame: DataFrame = mock[DataFrame]
assert(wrappedGlueContext.glueContext === mockGlueContext)
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString)).thenReturn(mockedDataSource)
}
}
The last when()
call throws the error below:
org.mockito.exceptions.misusing.InvalidUseOfMatchersException:
Invalid use of argument matchers!
0 matchers expected, 3 recorded:
This exception may occur if matchers are combined with raw values:
//incorrect:
someMethod(anyObject(), "raw String");
When using matchers, all arguments have to be provided by matchers.
For example:
//correct:
someMethod(anyObject(), eq("String by matcher"));
For more info see javadoc for Matchers class.
The definition for getCatalogSource()
is shown below
def getCatalogSource(database: String, tableName: String, redshiftTmpDir: String = "",
transformationContext: String = "", pushDownPredicate: String = "",
additionalOptions: JsonOptions = JsonOptions.empty, catalogId: String = null): DataSource = {
Why is it saying 0 matchers expected
when I'm calling the method getCatalogSource()
on a mocked object?
scala unit-testing mockito aws-glue
What's the definition ofgetCatalogSource
?
– Dima
Nov 9 at 23:17
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
I'm not sure if I can post the code forgetCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.
– Victor Cui
Nov 9 at 23:39
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
1
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56
|
show 1 more comment
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm writing unit tests in scala using MockitoSugar. I'm using org.mockito.Mockito.when
and org.mockito.Matchers.anyString
. The method under test is shown below.
def getDataSourceToDataFrame(database: scala.Predef.String, tableName: scala.Predef.String): DataFrame = {
glueContext.getCatalogSource(
database = database,
tableName = tableName,
transformationContext = database + "." + tableName + ".read")
.getDynamicFrame()
.toDF()
}
Here is what my unit test looks like:
import com.amazon.rrsetlglue.glue.wrappers.WrappedGlueContext
import com.amazonaws.services.glue.{DynamicFrame, GlueContext, DataSource}
import org.scalatest.{BeforeAndAfter, FunSuite, Matchers}
import org.apache.spark.sql.DataFrame
import org.mockito.Mockito.when
import org.mockito.Matchers.anyString
import org.scalatest.junit.JUnitRunner
import org.junit.runner.RunWith
// Use Mockito because ScalaMock does not work with Glue
@RunWith(classOf[JUnitRunner])
class WrappedGlueContextTest extends FunSuite with
org.scalatest.mockito.MockitoSugar with BeforeAndAfter {
var mockGlueContext : GlueContext = _
var wrappedGlueContext: WrappedGlueContext = _
before {
mockGlueContext = mock[GlueContext]
wrappedGlueContext = new WrappedGlueContext(mockGlueContext)
}
test("Test get dataFrame from glue data catalog source") {
val mockedDataSource: DataSource= mock[DataSource]
val mockDynamicFrame: DynamicFrame = mock[DynamicFrame]
val mockDataFrame: DataFrame = mock[DataFrame]
assert(wrappedGlueContext.glueContext === mockGlueContext)
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString)).thenReturn(mockedDataSource)
}
}
The last when()
call throws the error below:
org.mockito.exceptions.misusing.InvalidUseOfMatchersException:
Invalid use of argument matchers!
0 matchers expected, 3 recorded:
This exception may occur if matchers are combined with raw values:
//incorrect:
someMethod(anyObject(), "raw String");
When using matchers, all arguments have to be provided by matchers.
For example:
//correct:
someMethod(anyObject(), eq("String by matcher"));
For more info see javadoc for Matchers class.
The definition for getCatalogSource()
is shown below
def getCatalogSource(database: String, tableName: String, redshiftTmpDir: String = "",
transformationContext: String = "", pushDownPredicate: String = "",
additionalOptions: JsonOptions = JsonOptions.empty, catalogId: String = null): DataSource = {
Why is it saying 0 matchers expected
when I'm calling the method getCatalogSource()
on a mocked object?
scala unit-testing mockito aws-glue
I'm writing unit tests in scala using MockitoSugar. I'm using org.mockito.Mockito.when
and org.mockito.Matchers.anyString
. The method under test is shown below.
def getDataSourceToDataFrame(database: scala.Predef.String, tableName: scala.Predef.String): DataFrame = {
glueContext.getCatalogSource(
database = database,
tableName = tableName,
transformationContext = database + "." + tableName + ".read")
.getDynamicFrame()
.toDF()
}
Here is what my unit test looks like:
import com.amazon.rrsetlglue.glue.wrappers.WrappedGlueContext
import com.amazonaws.services.glue.{DynamicFrame, GlueContext, DataSource}
import org.scalatest.{BeforeAndAfter, FunSuite, Matchers}
import org.apache.spark.sql.DataFrame
import org.mockito.Mockito.when
import org.mockito.Matchers.anyString
import org.scalatest.junit.JUnitRunner
import org.junit.runner.RunWith
// Use Mockito because ScalaMock does not work with Glue
@RunWith(classOf[JUnitRunner])
class WrappedGlueContextTest extends FunSuite with
org.scalatest.mockito.MockitoSugar with BeforeAndAfter {
var mockGlueContext : GlueContext = _
var wrappedGlueContext: WrappedGlueContext = _
before {
mockGlueContext = mock[GlueContext]
wrappedGlueContext = new WrappedGlueContext(mockGlueContext)
}
test("Test get dataFrame from glue data catalog source") {
val mockedDataSource: DataSource= mock[DataSource]
val mockDynamicFrame: DynamicFrame = mock[DynamicFrame]
val mockDataFrame: DataFrame = mock[DataFrame]
assert(wrappedGlueContext.glueContext === mockGlueContext)
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString)).thenReturn(mockedDataSource)
}
}
The last when()
call throws the error below:
org.mockito.exceptions.misusing.InvalidUseOfMatchersException:
Invalid use of argument matchers!
0 matchers expected, 3 recorded:
This exception may occur if matchers are combined with raw values:
//incorrect:
someMethod(anyObject(), "raw String");
When using matchers, all arguments have to be provided by matchers.
For example:
//correct:
someMethod(anyObject(), eq("String by matcher"));
For more info see javadoc for Matchers class.
The definition for getCatalogSource()
is shown below
def getCatalogSource(database: String, tableName: String, redshiftTmpDir: String = "",
transformationContext: String = "", pushDownPredicate: String = "",
additionalOptions: JsonOptions = JsonOptions.empty, catalogId: String = null): DataSource = {
Why is it saying 0 matchers expected
when I'm calling the method getCatalogSource()
on a mocked object?
scala unit-testing mockito aws-glue
scala unit-testing mockito aws-glue
edited Nov 13 at 19:53
asked Nov 9 at 23:05
Victor Cui
358
358
What's the definition ofgetCatalogSource
?
– Dima
Nov 9 at 23:17
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
I'm not sure if I can post the code forgetCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.
– Victor Cui
Nov 9 at 23:39
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
1
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56
|
show 1 more comment
What's the definition ofgetCatalogSource
?
– Dima
Nov 9 at 23:17
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
I'm not sure if I can post the code forgetCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.
– Victor Cui
Nov 9 at 23:39
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
1
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56
What's the definition of
getCatalogSource
?– Dima
Nov 9 at 23:17
What's the definition of
getCatalogSource
?– Dima
Nov 9 at 23:17
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
I'm not sure if I can post the code for
getCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.– Victor Cui
Nov 9 at 23:39
I'm not sure if I can post the code for
getCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.– Victor Cui
Nov 9 at 23:39
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
1
1
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56
|
show 1 more comment
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
The issue was that getCatalogSource()
had default parameters that I wasn't passing in using my matchers. I changed the method call in the test to
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString, anyString, anyString, any(), any())).thenReturn(mockedDataSource)
and the test passed.
add a comment |
up vote
0
down vote
If you use the scala version of mockito the default arguments will be handled for you, it will also allow you to mix raw params and argument matchers
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234385%2finvalid-use-of-argument-matchers-0-matches-expected-3-recorded%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The issue was that getCatalogSource()
had default parameters that I wasn't passing in using my matchers. I changed the method call in the test to
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString, anyString, anyString, any(), any())).thenReturn(mockedDataSource)
and the test passed.
add a comment |
up vote
1
down vote
accepted
The issue was that getCatalogSource()
had default parameters that I wasn't passing in using my matchers. I changed the method call in the test to
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString, anyString, anyString, any(), any())).thenReturn(mockedDataSource)
and the test passed.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The issue was that getCatalogSource()
had default parameters that I wasn't passing in using my matchers. I changed the method call in the test to
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString, anyString, anyString, any(), any())).thenReturn(mockedDataSource)
and the test passed.
The issue was that getCatalogSource()
had default parameters that I wasn't passing in using my matchers. I changed the method call in the test to
when(mockGlueContext.getCatalogSource(anyString, anyString, anyString, anyString, anyString, any(), any())).thenReturn(mockedDataSource)
and the test passed.
answered Nov 13 at 19:59
Victor Cui
358
358
add a comment |
add a comment |
up vote
0
down vote
If you use the scala version of mockito the default arguments will be handled for you, it will also allow you to mix raw params and argument matchers
add a comment |
up vote
0
down vote
If you use the scala version of mockito the default arguments will be handled for you, it will also allow you to mix raw params and argument matchers
add a comment |
up vote
0
down vote
up vote
0
down vote
If you use the scala version of mockito the default arguments will be handled for you, it will also allow you to mix raw params and argument matchers
If you use the scala version of mockito the default arguments will be handled for you, it will also allow you to mix raw params and argument matchers
answered Nov 16 at 18:30


Bruno
1818
1818
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234385%2finvalid-use-of-argument-matchers-0-matches-expected-3-recorded%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6RnBw1UyaeQ zdGgIlutErtcg6 zc,oa,bGqmZ
What's the definition of
getCatalogSource
?– Dima
Nov 9 at 23:17
Also "Use mockito because scalamock does not work with glue" comment looks suspicious. Why doesn't scalamock work. Are you sure that mockito does?
– Dima
Nov 9 at 23:20
I'm not sure if I can post the code for
getCatalogSource
as it's a internal package. Yes I've verified that scalamock doesn't work and I've seen mockito being used successfully in other packages.– Victor Cui
Nov 9 at 23:39
Not the code ... just the definition.
– Dima
Nov 10 at 12:59
1
Yes! that was the problem @JamesWhiteley.
– Victor Cui
Nov 13 at 19:56