trying to call method I'm getting java.lang.IndexOutOfBoundsException
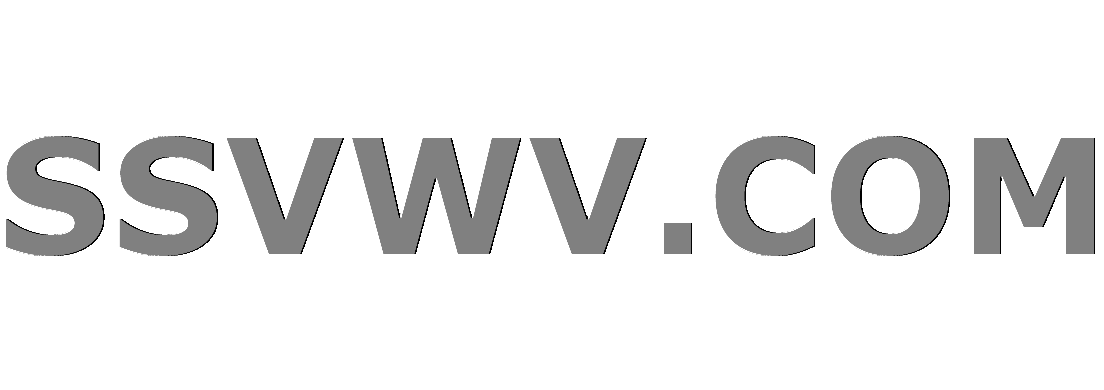
Multi tool use
I am trying to write a game on android, text quest.
I have process called "work" what it does is giving player chance to choose work place and how much time he wants to work, then process this information.
Below is code of two methods "checkWorkPlaces()" that checks if there are places to work in the city player are in, and then it calls "chooseWorkPlace()" method, that gives player chance to choose where he wants to work.
tvInfo - TextView, I show all information on it.
player - object of my custom class Player, that has such property as location of custom class Place, that has property name and workPlaces - array that contains work places of class WorkPlace, every one of those has definition and name property.
answerContainer - just ArrayList that I use to pass some information between methods.
btnMainGame - actually just a Button, that I use to save user information
hideButtons() - hides some buttons on screen [not important for my question]
protected void checkWorkPlaces() {
answerContainer.clear();
if (player.location.workPlaces.length > 0) {
tvInfo.setText("In " + player.location.name + " there are a few places to work:");
for (WorkPlace workPlace : player.location.workPlaces) {
tvInfo.append("n" + workPlace.defenition);
}
tvInfo.append("Where do you want to work?");
chooseWorkPlace();
} else {
tvInfo.setText("There is nowhere to work in " + player.location.name);
}
}
protected void chooseWorkPlace() {
hideButtons();
btnMainGame.setText(getString(R.string.save));
btnMainGame.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (etInput.getText().toString().isEmpty()){
Toast.makeText(MainActivity.this, "Please enter something", Toast.LENGTH_SHORT).show();
chooseWorkPlace();
} else {
answerContainer.add(etInput.getText().toString().toLowerCase());
}
}
});
String choice = answerContainer.get(0).toString().toLowerCase();
answerContainer.clear();
if (!Objects.equals(choice, "back")) {
for (WorkPlace workPlace : player.location.workPlaces) {
if (Objects.equals(workPlace.name.toLowerCase(), choice)) {
answerContainer.add(workPlace);
return;
}
}
tvInfo.append("nnThere is no such work place as: " + choice + ", try again");
chooseWorkPlace();
}
}
So, the problem is, that when program starts to call chooseWorkPlace() method it stops the program with java.lang.IndexOutOfBoundsException
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.griseostrigiformes.textwarrior, PID: 4916
java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.get(ArrayList.java:437)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.chooseWorkPlace(MainActivity.java:521)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.checkWorkPlaces(MainActivity.java:492)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity$2.onClick(MainActivity.java:124)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
How can I fix this problem?
java

add a comment |
I am trying to write a game on android, text quest.
I have process called "work" what it does is giving player chance to choose work place and how much time he wants to work, then process this information.
Below is code of two methods "checkWorkPlaces()" that checks if there are places to work in the city player are in, and then it calls "chooseWorkPlace()" method, that gives player chance to choose where he wants to work.
tvInfo - TextView, I show all information on it.
player - object of my custom class Player, that has such property as location of custom class Place, that has property name and workPlaces - array that contains work places of class WorkPlace, every one of those has definition and name property.
answerContainer - just ArrayList that I use to pass some information between methods.
btnMainGame - actually just a Button, that I use to save user information
hideButtons() - hides some buttons on screen [not important for my question]
protected void checkWorkPlaces() {
answerContainer.clear();
if (player.location.workPlaces.length > 0) {
tvInfo.setText("In " + player.location.name + " there are a few places to work:");
for (WorkPlace workPlace : player.location.workPlaces) {
tvInfo.append("n" + workPlace.defenition);
}
tvInfo.append("Where do you want to work?");
chooseWorkPlace();
} else {
tvInfo.setText("There is nowhere to work in " + player.location.name);
}
}
protected void chooseWorkPlace() {
hideButtons();
btnMainGame.setText(getString(R.string.save));
btnMainGame.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (etInput.getText().toString().isEmpty()){
Toast.makeText(MainActivity.this, "Please enter something", Toast.LENGTH_SHORT).show();
chooseWorkPlace();
} else {
answerContainer.add(etInput.getText().toString().toLowerCase());
}
}
});
String choice = answerContainer.get(0).toString().toLowerCase();
answerContainer.clear();
if (!Objects.equals(choice, "back")) {
for (WorkPlace workPlace : player.location.workPlaces) {
if (Objects.equals(workPlace.name.toLowerCase(), choice)) {
answerContainer.add(workPlace);
return;
}
}
tvInfo.append("nnThere is no such work place as: " + choice + ", try again");
chooseWorkPlace();
}
}
So, the problem is, that when program starts to call chooseWorkPlace() method it stops the program with java.lang.IndexOutOfBoundsException
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.griseostrigiformes.textwarrior, PID: 4916
java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.get(ArrayList.java:437)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.chooseWorkPlace(MainActivity.java:521)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.checkWorkPlaces(MainActivity.java:492)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity$2.onClick(MainActivity.java:124)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
How can I fix this problem?
java

answerContainer
is empty.
– TheWanderer
Nov 10 at 20:42
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15
add a comment |
I am trying to write a game on android, text quest.
I have process called "work" what it does is giving player chance to choose work place and how much time he wants to work, then process this information.
Below is code of two methods "checkWorkPlaces()" that checks if there are places to work in the city player are in, and then it calls "chooseWorkPlace()" method, that gives player chance to choose where he wants to work.
tvInfo - TextView, I show all information on it.
player - object of my custom class Player, that has such property as location of custom class Place, that has property name and workPlaces - array that contains work places of class WorkPlace, every one of those has definition and name property.
answerContainer - just ArrayList that I use to pass some information between methods.
btnMainGame - actually just a Button, that I use to save user information
hideButtons() - hides some buttons on screen [not important for my question]
protected void checkWorkPlaces() {
answerContainer.clear();
if (player.location.workPlaces.length > 0) {
tvInfo.setText("In " + player.location.name + " there are a few places to work:");
for (WorkPlace workPlace : player.location.workPlaces) {
tvInfo.append("n" + workPlace.defenition);
}
tvInfo.append("Where do you want to work?");
chooseWorkPlace();
} else {
tvInfo.setText("There is nowhere to work in " + player.location.name);
}
}
protected void chooseWorkPlace() {
hideButtons();
btnMainGame.setText(getString(R.string.save));
btnMainGame.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (etInput.getText().toString().isEmpty()){
Toast.makeText(MainActivity.this, "Please enter something", Toast.LENGTH_SHORT).show();
chooseWorkPlace();
} else {
answerContainer.add(etInput.getText().toString().toLowerCase());
}
}
});
String choice = answerContainer.get(0).toString().toLowerCase();
answerContainer.clear();
if (!Objects.equals(choice, "back")) {
for (WorkPlace workPlace : player.location.workPlaces) {
if (Objects.equals(workPlace.name.toLowerCase(), choice)) {
answerContainer.add(workPlace);
return;
}
}
tvInfo.append("nnThere is no such work place as: " + choice + ", try again");
chooseWorkPlace();
}
}
So, the problem is, that when program starts to call chooseWorkPlace() method it stops the program with java.lang.IndexOutOfBoundsException
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.griseostrigiformes.textwarrior, PID: 4916
java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.get(ArrayList.java:437)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.chooseWorkPlace(MainActivity.java:521)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.checkWorkPlaces(MainActivity.java:492)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity$2.onClick(MainActivity.java:124)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
How can I fix this problem?
java

I am trying to write a game on android, text quest.
I have process called "work" what it does is giving player chance to choose work place and how much time he wants to work, then process this information.
Below is code of two methods "checkWorkPlaces()" that checks if there are places to work in the city player are in, and then it calls "chooseWorkPlace()" method, that gives player chance to choose where he wants to work.
tvInfo - TextView, I show all information on it.
player - object of my custom class Player, that has such property as location of custom class Place, that has property name and workPlaces - array that contains work places of class WorkPlace, every one of those has definition and name property.
answerContainer - just ArrayList that I use to pass some information between methods.
btnMainGame - actually just a Button, that I use to save user information
hideButtons() - hides some buttons on screen [not important for my question]
protected void checkWorkPlaces() {
answerContainer.clear();
if (player.location.workPlaces.length > 0) {
tvInfo.setText("In " + player.location.name + " there are a few places to work:");
for (WorkPlace workPlace : player.location.workPlaces) {
tvInfo.append("n" + workPlace.defenition);
}
tvInfo.append("Where do you want to work?");
chooseWorkPlace();
} else {
tvInfo.setText("There is nowhere to work in " + player.location.name);
}
}
protected void chooseWorkPlace() {
hideButtons();
btnMainGame.setText(getString(R.string.save));
btnMainGame.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (etInput.getText().toString().isEmpty()){
Toast.makeText(MainActivity.this, "Please enter something", Toast.LENGTH_SHORT).show();
chooseWorkPlace();
} else {
answerContainer.add(etInput.getText().toString().toLowerCase());
}
}
});
String choice = answerContainer.get(0).toString().toLowerCase();
answerContainer.clear();
if (!Objects.equals(choice, "back")) {
for (WorkPlace workPlace : player.location.workPlaces) {
if (Objects.equals(workPlace.name.toLowerCase(), choice)) {
answerContainer.add(workPlace);
return;
}
}
tvInfo.append("nnThere is no such work place as: " + choice + ", try again");
chooseWorkPlace();
}
}
So, the problem is, that when program starts to call chooseWorkPlace() method it stops the program with java.lang.IndexOutOfBoundsException
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.griseostrigiformes.textwarrior, PID: 4916
java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.get(ArrayList.java:437)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.chooseWorkPlace(MainActivity.java:521)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity.checkWorkPlaces(MainActivity.java:492)
at com.example.griseostrigiformes.textwarrior.MainSystem.MainActivity$2.onClick(MainActivity.java:124)
at android.view.View.performClick(View.java:6256)
at android.view.View$PerformClick.run(View.java:24701)
at android.os.Handler.handleCallback(Handler.java:789)
at android.os.Handler.dispatchMessage(Handler.java:98)
at android.os.Looper.loop(Looper.java:164)
at android.app.ActivityThread.main(ActivityThread.java:6541)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.Zygote$MethodAndArgsCaller.run(Zygote.java:240)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:767)
How can I fix this problem?
java

java

asked Nov 10 at 20:39
Sergiy Horef
32
32
answerContainer
is empty.
– TheWanderer
Nov 10 at 20:42
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15
add a comment |
answerContainer
is empty.
– TheWanderer
Nov 10 at 20:42
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15
answerContainer
is empty.– TheWanderer
Nov 10 at 20:42
answerContainer
is empty.– TheWanderer
Nov 10 at 20:42
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15
add a comment |
1 Answer
1
active
oldest
votes
This line seems to be giving you the error,
String choice = answerContainer.get(0).toString().toLowerCase();
May be you should first check the size of answerContainer
before getting element at zero index? OR you should debug why answerContainer
is empty.
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243194%2ftrying-to-call-method-im-getting-java-lang-indexoutofboundsexception%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This line seems to be giving you the error,
String choice = answerContainer.get(0).toString().toLowerCase();
May be you should first check the size of answerContainer
before getting element at zero index? OR you should debug why answerContainer
is empty.
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
add a comment |
This line seems to be giving you the error,
String choice = answerContainer.get(0).toString().toLowerCase();
May be you should first check the size of answerContainer
before getting element at zero index? OR you should debug why answerContainer
is empty.
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
add a comment |
This line seems to be giving you the error,
String choice = answerContainer.get(0).toString().toLowerCase();
May be you should first check the size of answerContainer
before getting element at zero index? OR you should debug why answerContainer
is empty.
This line seems to be giving you the error,
String choice = answerContainer.get(0).toString().toLowerCase();
May be you should first check the size of answerContainer
before getting element at zero index? OR you should debug why answerContainer
is empty.
answered Nov 10 at 20:42
Pushpesh Kumar Rajwanshi
4,8141826
4,8141826
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
add a comment |
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
1
1
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
Thanks, it worked perfectly, just I started to do this game on console and it worked perfectly all the time.
– Sergiy Horef
Nov 11 at 6:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243194%2ftrying-to-call-method-im-getting-java-lang-indexoutofboundsexception%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mL0b BEcJPjwx3nD mRfYkFIhJ roz 8BLu 8LKc1l9nIhNIb w,VtZWDM jAB0kyrKbW
answerContainer
is empty.– TheWanderer
Nov 10 at 20:42
Debug the code, you will get the error line number
– Ankit Demonstrate
Nov 11 at 4:52
@AnkitDemonstrate i debugged my code is says, that problem is in line that calls out chooseWorkPlace()
– Sergiy Horef
Nov 11 at 6:15