while (cin >> *pchar) awaits further input after Ctrl-Z
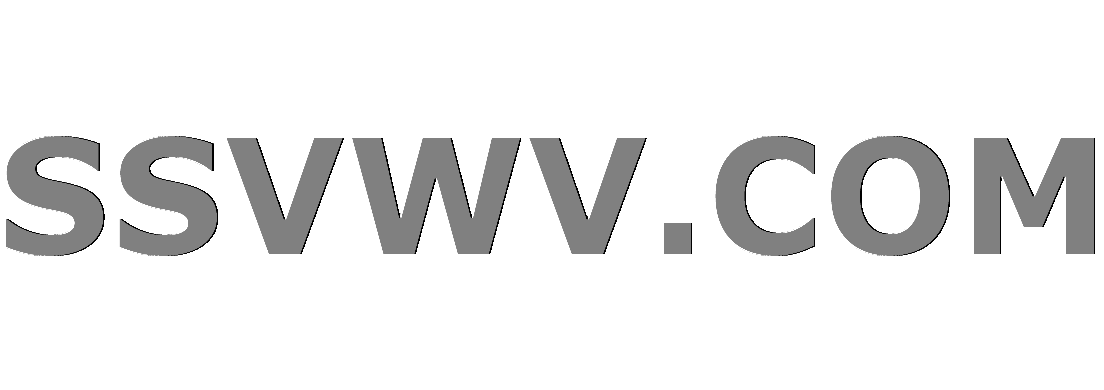
Multi tool use
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
|
show 7 more comments
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
Nov 19 '18 at 9:37
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
Nov 19 '18 at 9:53
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
Nov 19 '18 at 10:07
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
Nov 19 '18 at 11:33
|
show 7 more comments
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
In my Introduction to C++ classes, we were asked to write a function that returns the length of a string using pointers. The code that I wrote (see full code below) seems to work just fine, but here's the thing I don't understand.
I would think typing 'Yes' followed by Ctrl-Z ( I'm using Windows 10) in the console would stop the input. However, after pressing Ctrl-Z -> Enter the console still waits for further input. I have to start a new line after 'Yes', press Ctrl-Z and then hit Enter again to stop the input.
Why is this the case? Is there a way to stop the input after pressing just Ctrl-Z without any of the two new lines?
I read several posts on cin here, including this, this, and this, but they don't seem to answer my question.
#include "pch.h"
#include <iostream>
using namespace std;
unsigned strlen(const char *str)
{
int count = 0;
while (*str != '') { str++; count++; }
return count;
}
int main()
{
char str[100] = {};
char *pchar;
pchar = str;
while (cin >> *pchar) pchar++;
pchar = str;
cout << 'n' << strlen(pchar);
return 0;
}
c++ cin
c++ cin
edited Nov 19 '18 at 9:30
John Allison
asked Nov 19 '18 at 8:52


John AllisonJohn Allison
4051316
4051316
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
Nov 19 '18 at 9:37
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
Nov 19 '18 at 9:53
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
Nov 19 '18 at 10:07
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
Nov 19 '18 at 11:33
|
show 7 more comments
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doingchar *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.
– DevSolar
Nov 19 '18 at 9:37
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).
– DevSolar
Nov 19 '18 at 9:53
2
@JohnAllison: In C++ we havestd::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.
– DevSolar
Nov 19 '18 at 10:07
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...
– DevSolar
Nov 19 '18 at 11:33
4
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doing strlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
Nov 19 '18 at 9:37
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doing strlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
Nov 19 '18 at 9:37
4
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you <string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
Nov 19 '18 at 9:53
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you <string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
Nov 19 '18 at 9:53
2
2
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a. char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
Nov 19 '18 at 10:07
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a. char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
Nov 19 '18 at 10:07
1
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
2
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
Nov 19 '18 at 11:33
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
Nov 19 '18 at 11:33
|
show 7 more comments
2 Answers
2
active
oldest
votes
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
add a comment |
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
Nov 19 '18 at 10:59
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
|
show 10 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371127%2fwhile-cin-pchar-awaits-further-input-after-ctrl-z%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
add a comment |
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
add a comment |
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
If you press Ctrl-Z after typing some other text, it flushes the line buffer (it does not set end-of-file condition).
You have to press it twice in a row; or press it after a newline; to cause the end-of-file condition to occur.
Your misunderstanding is to do with the windows console behaviour, not with C++ streams per se.
See also: Why do I require multiple EOF (CTRL+Z) characters?
answered Nov 19 '18 at 11:02
M.MM.M
106k11117239
106k11117239
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
add a comment |
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
This helped a lot, thanks!
– John Allison
Nov 19 '18 at 11:15
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
yes I see. This problem is actually kinda Operating System dependent per se hey
– marvinIsSacul
Nov 19 '18 at 11:25
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
@marvinIsSacul yes, although I think ^D in unix works similarly (it's just a line flush if it occurs during a line)
– M.M
Nov 19 '18 at 11:26
add a comment |
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
Nov 19 '18 at 10:59
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
|
show 10 more comments
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
Nov 19 '18 at 10:59
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
|
show 10 more comments
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
Firstly we have to understand what you while loop actually does.
while (cin >> *pchar) pchar++;
says that continue getting input from the stdin
whilst cin
has not encountered an error (or to be specific whilst !cin.fail() == true
).
Note that cin
is basically an object of std::istream
.
Then secondly we have to understand what causes std::istream::fail
to return true
.
Here they are saying that std::istream::fail
returns true
if the badbit
or failbit
flags are set (and/or also if any non EOF error occurs).
Having said that, Ctrl-Z is actually EOF (end of file). And based on what I have said above, std::istream::fail
will return true
if any none EOF error occurs, and of course return false
if an EOF occurs.
So in short, EOF
/Ctrl-Z does not cause std::istream::fail
to return true
hence the loop will continue running.
If you really want to have your program cease executing when EOF
/Ctrl-Z gets hit, then adjust your loop to something like this...
while ((cin >> *pchar) && !cin.eof()) pchar++;
Now the loop above reads, whilst std::istream::fail() == false
and no EOF
/Ctrl-Z character has been entered, continue looping. Else cease the loop.
I hope that fully answers you.
edited Nov 19 '18 at 11:00
answered Nov 19 '18 at 10:05


marvinIsSaculmarvinIsSacul
53717
53717
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
Nov 19 '18 at 10:59
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
|
show 10 more comments
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causescin.fail()
get totrue
?
– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.
– John Allison
Nov 19 '18 at 10:59
ifcin >> *pchar
setseofbit
, it will also setfailbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of!cin.eof()
is poor
– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causes
cin.fail()
get to true
?– John Allison
Nov 19 '18 at 10:51
Thanks - it's almost clear now. So turning back to my sequence that worked (Enter - Ctrl-Z - Enter), what exactly causes
cin.fail()
get to true
?– John Allison
Nov 19 '18 at 10:51
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
that sequence worked with you old code?
– marvinIsSacul
Nov 19 '18 at 10:55
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding
!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.– John Allison
Nov 19 '18 at 10:59
It did, yes. Typing, e.g., 'Yes', then Enter, Ctrl-Z, Enter stops the input and has 3 as an output, so works just fine. Moreover, I just noted that adding
!cin.eof()
in the condition doesn't help. Pressing Ctrl-Z -> Enter right after 'Yes' doesn't stop the input either.– John Allison
Nov 19 '18 at 10:59
if
cin >> *pchar
sets eofbit
, it will also set failbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of !cin.eof()
is poor– M.M
Nov 19 '18 at 11:04
if
cin >> *pchar
sets eofbit
, it will also set failbit
due to failing to read a character, this is explained by the link you posted. The advice to add in a test of !cin.eof()
is poor– M.M
Nov 19 '18 at 11:04
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
From here, I think its because the end of the stream (EOF) was encountered while reading the next character (Enter). I'm not quite sure. Follow that link and many others within that, to find out more
– marvinIsSacul
Nov 19 '18 at 11:16
|
show 10 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371127%2fwhile-cin-pchar-awaits-further-input-after-ctrl-z%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0wFc,9T8Cnh8M,Wj eQhE5zX
4
Not trying to put too fine a point to it, but your C++ introduction course appears to teach C first... which is not a good idea. You shouldn't be doing
char *
in C++. Ever. Nor should you be doingstrlen()
by hand (a.k.a. "reinventing the wheel"). I know you probably cannot change any of that; I am just voicing my sadness that so many "Intro to C++" courses out there are still "C (with classes)". This is doing both you and C++'s reputation a disservice.– DevSolar
Nov 19 '18 at 9:37
4
@JohnAllison: ...pointers, which you shouldn't have to touch at all, or at least not until you're doing the advanced course. You're being taught all the C stuff -- pointers, arrays,
char *
-- all of which is unnecessary / bad C++ style, and which you will have to un-learn once you get to "real" C++. Instead of showing you<string>
and references and having you do real stuff instead of reinventing the (C) wheel. I feel this is wasting your time, and making things unnecessarily difficult for you (and the instructor).– DevSolar
Nov 19 '18 at 9:53
2
@JohnAllison: In C++ we have
std::string
and the corresponding functions operating on those. Just two counterquestions: What happens, in your code, if the user enters the 101st character without ending the input? What happens if, e.g. by input redirection, the user enters a null byte somewhere in his input? Also, you can't teach C strings a.k.a.char
without teaching pointers as well... which IMHO shouldn't be taught in an intro to C++, at all.– DevSolar
Nov 19 '18 at 10:07
1
@marvin: His course should have started with the C++ that makes buffer overflows a non-issue. And I know I am not answering. I am commenting.
– DevSolar
Nov 19 '18 at 10:12
2
@JohnAllison: And I understand the "bottom up" approach and all that, but I disagree with its helpfulness. Students want to achieve results. Especially with C++ where everybody tells you how godawful hard it reputedly is (which it is only if you have to learn, then unlearn C first), I find it more helpful to go top-down, and dive right in using string, vector,
<algorithm>
, polymorphy and templates to their full potential instead of worrying about what's under the hood. That's for the advanced course, "library development"...– DevSolar
Nov 19 '18 at 11:33