How to send email with django “Rest Framwork”?
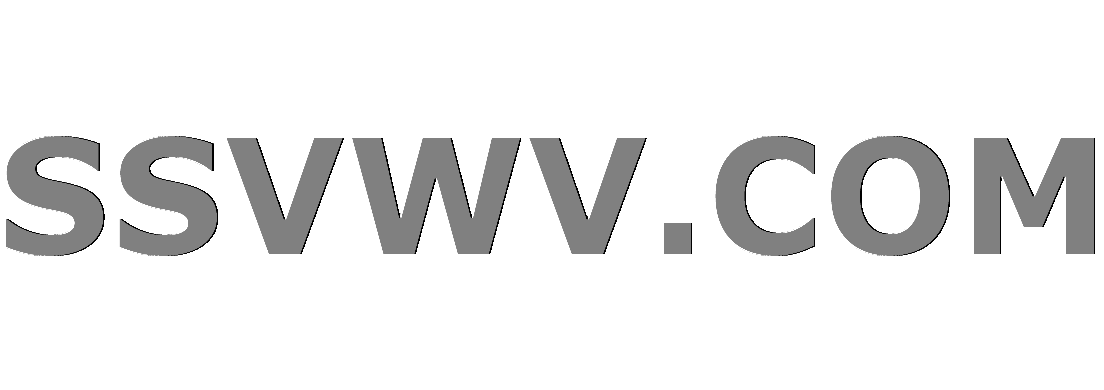
Multi tool use
I'm using small Rest api project
and it works fantastic.
But somehow i have to make send email function in there.
so i added email config in settings.py like that
// settings.py
# SMTP Mail service with decouple
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = "smtp.gmail.com"
EMAIL_HOST_USER = config('EM_ACCOUNT')
EMAIL_HOST_PASSWORD = config('EM_PASSWORD')
EMAIL_PORT = 587
EMAIL_USE_TLS = True
DEFAULT_FROM_EMAIL = EMAIL_HOST_USER
//views.py
from rest_framework import viewsets
from consult.models import Consult
from consult.serializers import ConsultSerializer
from django.core.mail import EmailMessage
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def send_email(request):
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
// models.py
from django.db import models
# Create your models here.
class Consult(models.Model):
name = models.CharField(max_length=16)
position = models.CharField(max_length=16, null=True)
group = models.CharField(max_length=50)
email = models.CharField(max_length=50, null=True)
phone = models.CharField(max_length=14)
describe = models.TextField(blank=True, null=True)
file = models.FileField(blank=True, null=True)
create_date = models.DateTimeField(auto_now_add=True)
update_date = models.DateTimeField(auto_now=True)
class Meta:
db_table = 'Consult'
// serializers.py
from rest_framework import serializers
from .models import Consult
class ConsultSerializer(serializers.ModelSerializer):
class Meta:
model = Consult
fields = ('id', 'name', 'position', 'group', 'email', 'phone', 'describe', 'file', 'create_date')
Yup. that is all of my codes.
And i setted send_mail function in views.
Honestly I want automatic send mail function
when the consult data in my DB. but I can find only normal django explanation.
Can you help me set a automatic send mail function when data created??
python django django-rest-framework
add a comment |
I'm using small Rest api project
and it works fantastic.
But somehow i have to make send email function in there.
so i added email config in settings.py like that
// settings.py
# SMTP Mail service with decouple
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = "smtp.gmail.com"
EMAIL_HOST_USER = config('EM_ACCOUNT')
EMAIL_HOST_PASSWORD = config('EM_PASSWORD')
EMAIL_PORT = 587
EMAIL_USE_TLS = True
DEFAULT_FROM_EMAIL = EMAIL_HOST_USER
//views.py
from rest_framework import viewsets
from consult.models import Consult
from consult.serializers import ConsultSerializer
from django.core.mail import EmailMessage
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def send_email(request):
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
// models.py
from django.db import models
# Create your models here.
class Consult(models.Model):
name = models.CharField(max_length=16)
position = models.CharField(max_length=16, null=True)
group = models.CharField(max_length=50)
email = models.CharField(max_length=50, null=True)
phone = models.CharField(max_length=14)
describe = models.TextField(blank=True, null=True)
file = models.FileField(blank=True, null=True)
create_date = models.DateTimeField(auto_now_add=True)
update_date = models.DateTimeField(auto_now=True)
class Meta:
db_table = 'Consult'
// serializers.py
from rest_framework import serializers
from .models import Consult
class ConsultSerializer(serializers.ModelSerializer):
class Meta:
model = Consult
fields = ('id', 'name', 'position', 'group', 'email', 'phone', 'describe', 'file', 'create_date')
Yup. that is all of my codes.
And i setted send_mail function in views.
Honestly I want automatic send mail function
when the consult data in my DB. but I can find only normal django explanation.
Can you help me set a automatic send mail function when data created??
python django django-rest-framework
What you mean byautomatic send mail function
?
– JPG
Nov 21 '18 at 3:10
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
1
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
2
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43
add a comment |
I'm using small Rest api project
and it works fantastic.
But somehow i have to make send email function in there.
so i added email config in settings.py like that
// settings.py
# SMTP Mail service with decouple
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = "smtp.gmail.com"
EMAIL_HOST_USER = config('EM_ACCOUNT')
EMAIL_HOST_PASSWORD = config('EM_PASSWORD')
EMAIL_PORT = 587
EMAIL_USE_TLS = True
DEFAULT_FROM_EMAIL = EMAIL_HOST_USER
//views.py
from rest_framework import viewsets
from consult.models import Consult
from consult.serializers import ConsultSerializer
from django.core.mail import EmailMessage
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def send_email(request):
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
// models.py
from django.db import models
# Create your models here.
class Consult(models.Model):
name = models.CharField(max_length=16)
position = models.CharField(max_length=16, null=True)
group = models.CharField(max_length=50)
email = models.CharField(max_length=50, null=True)
phone = models.CharField(max_length=14)
describe = models.TextField(blank=True, null=True)
file = models.FileField(blank=True, null=True)
create_date = models.DateTimeField(auto_now_add=True)
update_date = models.DateTimeField(auto_now=True)
class Meta:
db_table = 'Consult'
// serializers.py
from rest_framework import serializers
from .models import Consult
class ConsultSerializer(serializers.ModelSerializer):
class Meta:
model = Consult
fields = ('id', 'name', 'position', 'group', 'email', 'phone', 'describe', 'file', 'create_date')
Yup. that is all of my codes.
And i setted send_mail function in views.
Honestly I want automatic send mail function
when the consult data in my DB. but I can find only normal django explanation.
Can you help me set a automatic send mail function when data created??
python django django-rest-framework
I'm using small Rest api project
and it works fantastic.
But somehow i have to make send email function in there.
so i added email config in settings.py like that
// settings.py
# SMTP Mail service with decouple
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = "smtp.gmail.com"
EMAIL_HOST_USER = config('EM_ACCOUNT')
EMAIL_HOST_PASSWORD = config('EM_PASSWORD')
EMAIL_PORT = 587
EMAIL_USE_TLS = True
DEFAULT_FROM_EMAIL = EMAIL_HOST_USER
//views.py
from rest_framework import viewsets
from consult.models import Consult
from consult.serializers import ConsultSerializer
from django.core.mail import EmailMessage
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def send_email(request):
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
// models.py
from django.db import models
# Create your models here.
class Consult(models.Model):
name = models.CharField(max_length=16)
position = models.CharField(max_length=16, null=True)
group = models.CharField(max_length=50)
email = models.CharField(max_length=50, null=True)
phone = models.CharField(max_length=14)
describe = models.TextField(blank=True, null=True)
file = models.FileField(blank=True, null=True)
create_date = models.DateTimeField(auto_now_add=True)
update_date = models.DateTimeField(auto_now=True)
class Meta:
db_table = 'Consult'
// serializers.py
from rest_framework import serializers
from .models import Consult
class ConsultSerializer(serializers.ModelSerializer):
class Meta:
model = Consult
fields = ('id', 'name', 'position', 'group', 'email', 'phone', 'describe', 'file', 'create_date')
Yup. that is all of my codes.
And i setted send_mail function in views.
Honestly I want automatic send mail function
when the consult data in my DB. but I can find only normal django explanation.
Can you help me set a automatic send mail function when data created??
python django django-rest-framework
python django django-rest-framework
edited Nov 21 '18 at 9:18
Daniel Roseman
453k41586642
453k41586642
asked Nov 21 '18 at 3:09


Dong-geun Jayse RyuDong-geun Jayse Ryu
66
66
What you mean byautomatic send mail function
?
– JPG
Nov 21 '18 at 3:10
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
1
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
2
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43
add a comment |
What you mean byautomatic send mail function
?
– JPG
Nov 21 '18 at 3:10
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
1
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
2
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43
What you mean by
automatic send mail function
?– JPG
Nov 21 '18 at 3:10
What you mean by
automatic send mail function
?– JPG
Nov 21 '18 at 3:10
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
1
1
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
2
2
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43
add a comment |
1 Answer
1
active
oldest
votes
Method-1 : override the create()
method of ConsultViewSet
def send_email():
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def create(self, request, *args, **kwargs):
response = super(ConsultViewSet, self).create(request, *args, **kwargs)
send_email() # sending mail
return response
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to addself
in that statement. Check my updated answer
– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404738%2fhow-to-send-email-with-django-rest-framwork%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Method-1 : override the create()
method of ConsultViewSet
def send_email():
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def create(self, request, *args, **kwargs):
response = super(ConsultViewSet, self).create(request, *args, **kwargs)
send_email() # sending mail
return response
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to addself
in that statement. Check my updated answer
– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
add a comment |
Method-1 : override the create()
method of ConsultViewSet
def send_email():
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def create(self, request, *args, **kwargs):
response = super(ConsultViewSet, self).create(request, *args, **kwargs)
send_email() # sending mail
return response
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to addself
in that statement. Check my updated answer
– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
add a comment |
Method-1 : override the create()
method of ConsultViewSet
def send_email():
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def create(self, request, *args, **kwargs):
response = super(ConsultViewSet, self).create(request, *args, **kwargs)
send_email() # sending mail
return response
Method-1 : override the create()
method of ConsultViewSet
def send_email():
email = EmailMessage(
'Title',
(ConsultSerializer.name, ConsultSerializer.email, ConsultSerializer.phone),
'my-email',
['my-receive-email']
)
email.attach_file(ConsultSerializer.file)
email.send()
class ConsultViewSet(viewsets.ModelViewSet):
queryset = Consult.objects.all()
serializer_class = ConsultSerializer
def create(self, request, *args, **kwargs):
response = super(ConsultViewSet, self).create(request, *args, **kwargs)
send_email() # sending mail
return response
edited Nov 21 '18 at 4:30
answered Nov 21 '18 at 3:56


JPGJPG
14.1k2932
14.1k2932
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to addself
in that statement. Check my updated answer
– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
add a comment |
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to addself
in that statement. Check my updated answer
– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
i've got an error // AttributeError: 'super' object has no attribute 'create'
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:20
I forgot to add
self
in that statement. Check my updated answer– JPG
Nov 21 '18 at 4:30
I forgot to add
self
in that statement. Check my updated answer– JPG
Nov 21 '18 at 4:30
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
Thank you! it works! brilliant 😌
– Dong-geun Jayse Ryu
Nov 21 '18 at 4:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404738%2fhow-to-send-email-with-django-rest-framwork%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gWckVLX PsC8HXB3zOn6EQe9lo9saCe57jf Tz09 pNGZ1je4XfJ4dPFTR21B7xZJxsE2n2
What you mean by
automatic send mail function
?– JPG
Nov 21 '18 at 3:10
@JPG I meant i want that function will work when new data come, work with create DB
– Dong-geun Jayse Ryu
Nov 21 '18 at 3:15
1
see stackoverflow.com/questions/8170704/…
– Red Cricket
Nov 21 '18 at 3:42
2
Possible duplicate of Execute code on model creation in Django
– Red Cricket
Nov 21 '18 at 3:43