Iterating through a list, to find item
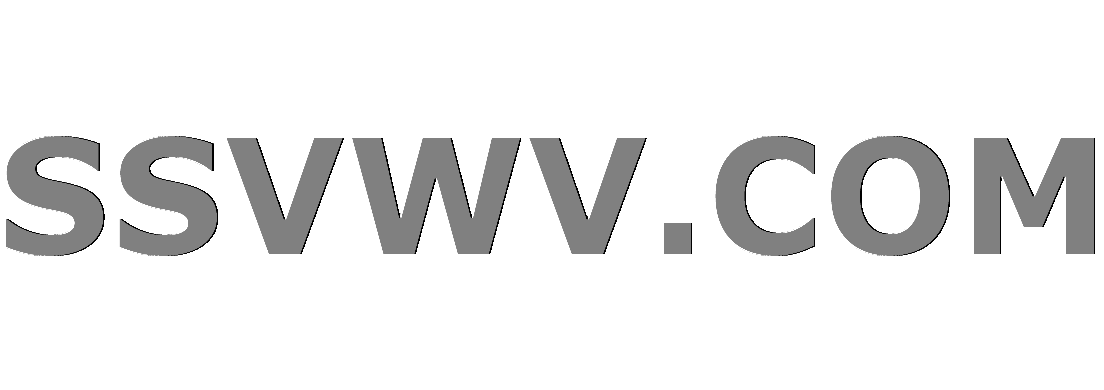
Multi tool use
So I need to make a code that checks to see if a pokemon has a type, and if it does it will add that pokemon name to a dictionary with all the other pokemon that possess the same type. All the info for this is stored on an a csv file. Also the indenting looks quite weird but it's indented properly on my actual file.
import sqlite3
import csv
SEP = ','
def get_pokemon_stats():
"""Reads the data contained in a pokemon data file and parses it into
several data structures.
Args: None
Returns:
-a dict where:
-each key is a pokemon type (str). Note that type_1 and type_2
entries are all considered types. There should be no special
treatment for the type NA; it is considered a type as well.
-each value is a list of all pokemon names (strs) that fall into
the corresponding type
"""
type_list =
poketype =
pokewith_type =
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.strip().split(SEP)
type_list.extend(list_of_values[6:8])
for i in range(len(type_list)):
if type_list[i] not in poketype:
poketype.append(type_list[i])
poketypelist = (list_of_values[1], list_of_values[6:8])
for i in range(len(poketypelist) - 1):
if type_list[i] in poketype:
pokemon_by_type[ type_list[i]] = poketypelist[i]
My Question:
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary.
An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys.
My CSV file look like:
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types.
python
|
show 3 more comments
So I need to make a code that checks to see if a pokemon has a type, and if it does it will add that pokemon name to a dictionary with all the other pokemon that possess the same type. All the info for this is stored on an a csv file. Also the indenting looks quite weird but it's indented properly on my actual file.
import sqlite3
import csv
SEP = ','
def get_pokemon_stats():
"""Reads the data contained in a pokemon data file and parses it into
several data structures.
Args: None
Returns:
-a dict where:
-each key is a pokemon type (str). Note that type_1 and type_2
entries are all considered types. There should be no special
treatment for the type NA; it is considered a type as well.
-each value is a list of all pokemon names (strs) that fall into
the corresponding type
"""
type_list =
poketype =
pokewith_type =
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.strip().split(SEP)
type_list.extend(list_of_values[6:8])
for i in range(len(type_list)):
if type_list[i] not in poketype:
poketype.append(type_list[i])
poketypelist = (list_of_values[1], list_of_values[6:8])
for i in range(len(poketypelist) - 1):
if type_list[i] in poketype:
pokemon_by_type[ type_list[i]] = poketypelist[i]
My Question:
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary.
An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys.
My CSV file look like:
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types.
python
2
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
1
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
1
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36
|
show 3 more comments
So I need to make a code that checks to see if a pokemon has a type, and if it does it will add that pokemon name to a dictionary with all the other pokemon that possess the same type. All the info for this is stored on an a csv file. Also the indenting looks quite weird but it's indented properly on my actual file.
import sqlite3
import csv
SEP = ','
def get_pokemon_stats():
"""Reads the data contained in a pokemon data file and parses it into
several data structures.
Args: None
Returns:
-a dict where:
-each key is a pokemon type (str). Note that type_1 and type_2
entries are all considered types. There should be no special
treatment for the type NA; it is considered a type as well.
-each value is a list of all pokemon names (strs) that fall into
the corresponding type
"""
type_list =
poketype =
pokewith_type =
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.strip().split(SEP)
type_list.extend(list_of_values[6:8])
for i in range(len(type_list)):
if type_list[i] not in poketype:
poketype.append(type_list[i])
poketypelist = (list_of_values[1], list_of_values[6:8])
for i in range(len(poketypelist) - 1):
if type_list[i] in poketype:
pokemon_by_type[ type_list[i]] = poketypelist[i]
My Question:
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary.
An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys.
My CSV file look like:
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types.
python
So I need to make a code that checks to see if a pokemon has a type, and if it does it will add that pokemon name to a dictionary with all the other pokemon that possess the same type. All the info for this is stored on an a csv file. Also the indenting looks quite weird but it's indented properly on my actual file.
import sqlite3
import csv
SEP = ','
def get_pokemon_stats():
"""Reads the data contained in a pokemon data file and parses it into
several data structures.
Args: None
Returns:
-a dict where:
-each key is a pokemon type (str). Note that type_1 and type_2
entries are all considered types. There should be no special
treatment for the type NA; it is considered a type as well.
-each value is a list of all pokemon names (strs) that fall into
the corresponding type
"""
type_list =
poketype =
pokewith_type =
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.strip().split(SEP)
type_list.extend(list_of_values[6:8])
for i in range(len(type_list)):
if type_list[i] not in poketype:
poketype.append(type_list[i])
poketypelist = (list_of_values[1], list_of_values[6:8])
for i in range(len(poketypelist) - 1):
if type_list[i] in poketype:
pokemon_by_type[ type_list[i]] = poketypelist[i]
My Question:
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary.
An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys.
My CSV file look like:
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types.
python
python
edited Nov 21 '18 at 7:13
stovfl
8,03331131
8,03331131
asked Nov 21 '18 at 3:21
Meliodus123Meliodus123
375
375
2
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
1
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
1
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36
|
show 3 more comments
2
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
1
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
1
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36
2
2
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
1
1
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
1
1
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36
|
show 3 more comments
2 Answers
2
active
oldest
votes
data.csv
name,stuff,stuff,type1,type2
bulbasaur,who knows,who knows,poison,grass
fakeasaur,who knows,who knows,poison,grass
pikachu,who knows,who knows,lightning,rat
pokemon.py
#!/bin/python
the_big_d = {}
with open('data.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
l = line[0:-1].split(',')
if l[3] not in the_big_d:
the_big_d[l[3]] = [l[0]]
else:
the_big_d[l[3]].append(l[0])
if l[4] not in the_big_d:
the_big_d[l[4]] = [l[0]]
else:
the_big_d[l[4]].append(l[0])
print(the_big_d)
Output:
{'poison': ['bulbasaur', 'fakeasaur'], 'grass': ['bulbasaur', 'fakeasaur'], 'lightning': ['pikachu'], 'rat': ['pikachu']}
add a comment |
I'm assuming column 2 has the name of the pokemon and column 6 and 7 are it's types. And since you can't use pandas,
I don' know what exactly you are asking, but here is what I think you are looking for
def get_pokemon_stats():
"""
Reads a csv file and returns a Dict of pokemons grouped under their type
"""
type_list =
pokemon_by_type_dict = {}
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.split(",")
for i in list_of_values[6:8]:
if i not in type_list:
type_list.append(i)
pokemon_by_type_dict[i] = # Each "Type" key is a list of pokemons, change it if you want to
pokemon_by_type_dict[i].append(list_of_values[1])
return pokemon_by_type_dict # Returns the pokemon dict
Is this the code you were looking for?
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404813%2fiterating-through-a-list-to-find-item%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
data.csv
name,stuff,stuff,type1,type2
bulbasaur,who knows,who knows,poison,grass
fakeasaur,who knows,who knows,poison,grass
pikachu,who knows,who knows,lightning,rat
pokemon.py
#!/bin/python
the_big_d = {}
with open('data.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
l = line[0:-1].split(',')
if l[3] not in the_big_d:
the_big_d[l[3]] = [l[0]]
else:
the_big_d[l[3]].append(l[0])
if l[4] not in the_big_d:
the_big_d[l[4]] = [l[0]]
else:
the_big_d[l[4]].append(l[0])
print(the_big_d)
Output:
{'poison': ['bulbasaur', 'fakeasaur'], 'grass': ['bulbasaur', 'fakeasaur'], 'lightning': ['pikachu'], 'rat': ['pikachu']}
add a comment |
data.csv
name,stuff,stuff,type1,type2
bulbasaur,who knows,who knows,poison,grass
fakeasaur,who knows,who knows,poison,grass
pikachu,who knows,who knows,lightning,rat
pokemon.py
#!/bin/python
the_big_d = {}
with open('data.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
l = line[0:-1].split(',')
if l[3] not in the_big_d:
the_big_d[l[3]] = [l[0]]
else:
the_big_d[l[3]].append(l[0])
if l[4] not in the_big_d:
the_big_d[l[4]] = [l[0]]
else:
the_big_d[l[4]].append(l[0])
print(the_big_d)
Output:
{'poison': ['bulbasaur', 'fakeasaur'], 'grass': ['bulbasaur', 'fakeasaur'], 'lightning': ['pikachu'], 'rat': ['pikachu']}
add a comment |
data.csv
name,stuff,stuff,type1,type2
bulbasaur,who knows,who knows,poison,grass
fakeasaur,who knows,who knows,poison,grass
pikachu,who knows,who knows,lightning,rat
pokemon.py
#!/bin/python
the_big_d = {}
with open('data.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
l = line[0:-1].split(',')
if l[3] not in the_big_d:
the_big_d[l[3]] = [l[0]]
else:
the_big_d[l[3]].append(l[0])
if l[4] not in the_big_d:
the_big_d[l[4]] = [l[0]]
else:
the_big_d[l[4]].append(l[0])
print(the_big_d)
Output:
{'poison': ['bulbasaur', 'fakeasaur'], 'grass': ['bulbasaur', 'fakeasaur'], 'lightning': ['pikachu'], 'rat': ['pikachu']}
data.csv
name,stuff,stuff,type1,type2
bulbasaur,who knows,who knows,poison,grass
fakeasaur,who knows,who knows,poison,grass
pikachu,who knows,who knows,lightning,rat
pokemon.py
#!/bin/python
the_big_d = {}
with open('data.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
l = line[0:-1].split(',')
if l[3] not in the_big_d:
the_big_d[l[3]] = [l[0]]
else:
the_big_d[l[3]].append(l[0])
if l[4] not in the_big_d:
the_big_d[l[4]] = [l[0]]
else:
the_big_d[l[4]].append(l[0])
print(the_big_d)
Output:
{'poison': ['bulbasaur', 'fakeasaur'], 'grass': ['bulbasaur', 'fakeasaur'], 'lightning': ['pikachu'], 'rat': ['pikachu']}
answered Nov 21 '18 at 7:30
ConnerConner
23.5k84568
23.5k84568
add a comment |
add a comment |
I'm assuming column 2 has the name of the pokemon and column 6 and 7 are it's types. And since you can't use pandas,
I don' know what exactly you are asking, but here is what I think you are looking for
def get_pokemon_stats():
"""
Reads a csv file and returns a Dict of pokemons grouped under their type
"""
type_list =
pokemon_by_type_dict = {}
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.split(",")
for i in list_of_values[6:8]:
if i not in type_list:
type_list.append(i)
pokemon_by_type_dict[i] = # Each "Type" key is a list of pokemons, change it if you want to
pokemon_by_type_dict[i].append(list_of_values[1])
return pokemon_by_type_dict # Returns the pokemon dict
Is this the code you were looking for?
add a comment |
I'm assuming column 2 has the name of the pokemon and column 6 and 7 are it's types. And since you can't use pandas,
I don' know what exactly you are asking, but here is what I think you are looking for
def get_pokemon_stats():
"""
Reads a csv file and returns a Dict of pokemons grouped under their type
"""
type_list =
pokemon_by_type_dict = {}
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.split(",")
for i in list_of_values[6:8]:
if i not in type_list:
type_list.append(i)
pokemon_by_type_dict[i] = # Each "Type" key is a list of pokemons, change it if you want to
pokemon_by_type_dict[i].append(list_of_values[1])
return pokemon_by_type_dict # Returns the pokemon dict
Is this the code you were looking for?
add a comment |
I'm assuming column 2 has the name of the pokemon and column 6 and 7 are it's types. And since you can't use pandas,
I don' know what exactly you are asking, but here is what I think you are looking for
def get_pokemon_stats():
"""
Reads a csv file and returns a Dict of pokemons grouped under their type
"""
type_list =
pokemon_by_type_dict = {}
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.split(",")
for i in list_of_values[6:8]:
if i not in type_list:
type_list.append(i)
pokemon_by_type_dict[i] = # Each "Type" key is a list of pokemons, change it if you want to
pokemon_by_type_dict[i].append(list_of_values[1])
return pokemon_by_type_dict # Returns the pokemon dict
Is this the code you were looking for?
I'm assuming column 2 has the name of the pokemon and column 6 and 7 are it's types. And since you can't use pandas,
I don' know what exactly you are asking, but here is what I think you are looking for
def get_pokemon_stats():
"""
Reads a csv file and returns a Dict of pokemons grouped under their type
"""
type_list =
pokemon_by_type_dict = {}
DATA_FILENAME = 'pokemon.csv'
with open('pokemon.csv', 'r') as csv_file:
csv_file.readline()
for line in csv_file:
list_of_values = line.split(",")
for i in list_of_values[6:8]:
if i not in type_list:
type_list.append(i)
pokemon_by_type_dict[i] = # Each "Type" key is a list of pokemons, change it if you want to
pokemon_by_type_dict[i].append(list_of_values[1])
return pokemon_by_type_dict # Returns the pokemon dict
Is this the code you were looking for?
answered Nov 21 '18 at 7:21


Avichal BettoliAvichal Bettoli
493
493
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404813%2fiterating-through-a-list-to-find-item%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3fJrk3DhMz5Y0 2Jq1AVWcz,XzBz TNdm 1Qu oolxKy3ggfp,Ke fogYfdk aSNs 5rd,CJfLn8PC,p9E,90qtKqNyIBp8Hn8
2
What's your question?
– Spencer Wieczorek
Nov 21 '18 at 3:24
1
And what does the csv file look like?
– slider
Nov 21 '18 at 3:26
I don't know how to make python identify if the pokemon in the list has a type and if it does add it to a dictionary. An example; if bulbasaur is poison grass, then in the dictionary bulbasaur should show up next to the poison and grass keys. @SpencerWieczorek
– Meliodus123
Nov 21 '18 at 3:27
It has pokemon name, then a bunch of other stuff then the third and fourth columns are the two types. @slider
– Meliodus123
Nov 21 '18 at 3:29
1
It's better to put the sample of data on your question, and it will be easier to read csv with pandas.
– taipei
Nov 21 '18 at 3:36