KnockoutJS doesn't bind fields that have been autocompleted
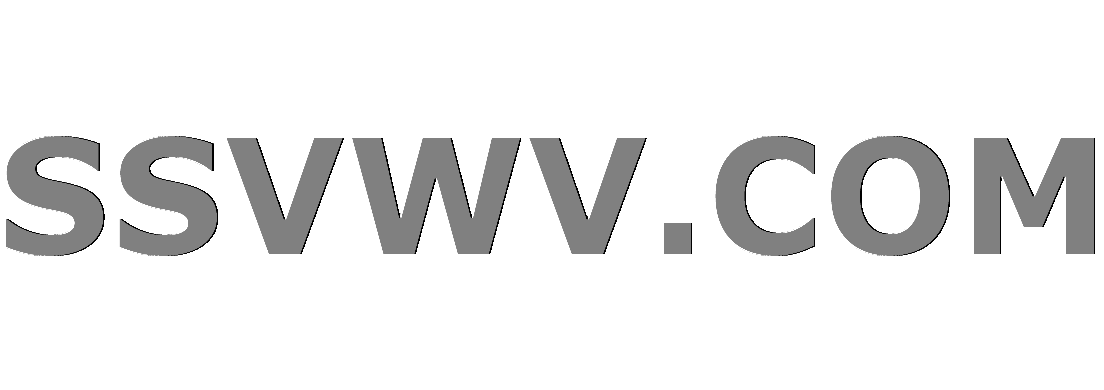
Multi tool use
I'm using an external file where I get company data. The autocomplete works just fine, inputs get filled. But when I save the page into my database all the autocompleted fields don't get bind.
public Save(saveorder: Boolean): void {
let order: Order = ko.toJS(OrderPage.Instance().Order);
let date = OrderPage.Instance().Order.DateAccepted;
let removefromorderlines: Array<number> = ;
OrderPage.Instance().j$.each(order.OrderLines, (index: number, orderline: OrderLine) => {
// Check if there are any orderlines that don't have a modelid
if (orderline.ArticleModelId == null) {
removefromorderlines.push(index);
}
});
let previousindex: any = 0;
$.each(removefromorderlines, (index: number, orderlineindex: number) => {
order.OrderLines.splice(orderlineindex - previousindex, 1);
previousindex++;
});
if (saveorder == true) {
OrderPage.Instance().ToggleDisableButton('btnSaveOrder', true);
webApi.Execute(HttpRequestType.Post, '/Order/SaveOrder', order, OrderPage.Instance().OnSaveSuccess, OrderPage.Instance().OnSaveError);
}
The data that is missing is inside of 'Order'. The data gets picked up as soon as I change anything about the inputs. I've tried to use "valueUpdate: 'input'" but no success so far.
This is my autocomplete code:
namespace Services {
interface kvkResult {
handelsnaam: string;
dossiernummer: number;
straat: string;
huisnummer: number;
huisnummertoevoeging: string;
postcode: string;
plaats: string;
ModelId: string;
displayname: string;
ContactPersonModelId: string;
}
interface Contact {
ModelId: string;
displayname: string;
}
export class kvkFactory
{
private ctlCompanyName: string;
private ctlContainerName: string;
private ctlCoc: string;
private ctlAddress: string;
private ctlAddressNumber: string;
private ctlAddressNumberSuffix: string;
private ctlZipCode: string;
private ctlTown: string;
private ctlModelId: string;
private ctlContactPersonModelId: string;
/** Function to Fetch data from kvk api
@ctlSearch: Input control for coc organisations
@ctlAutocompleteContainer: output control to display organisations result.
@ctlCocClass: output text control for Kamer voor koophandel nummer
@ctlAddressClass: output text control for address organisation
@ctlZipCodeClass: output text contorl for zip code organisation
@ctlTownClass: output text contorl for town of settlement organisation
*/
constructor(ctlSearch: string, ctlAutocompleteContainer: string, ctlCompanyNameElement: string, ctlCocElement: string, ctlAddressElement: string, ctlAddressNumberElement: string, ctlAddressNumberSuffixElement: string, ctlZipCodeElement: string, ctlTownElement: string, ctlModelIdElement?: string, ctlContactPersonModelIdElement?: string, webapiurlforsearch?: string)
{
this.ctlCompanyName = ctlSearch;
this.ctlContainerName = ctlAutocompleteContainer;
this.ctlCompanyName = ctlCompanyNameElement;
this.ctlCoc = ctlCocElement;
this.ctlAddress = ctlAddressElement;
this.ctlAddressNumber = ctlAddressNumberElement;
this.ctlAddressNumberSuffix = ctlAddressNumberSuffixElement;
this.ctlZipCode = ctlZipCodeElement;
this.ctlTown = ctlTownElement;
this.ctlModelId = ctlModelIdElement;
this.ctlContactPersonModelId = ctlContactPersonModelIdElement;
$(this.ctlCompanyName).keyup(function () {
clearTimeout(this.typingTimer);
this.typingTimer = setTimeout(() => { kvkfactory.searchKvk(webapiurlforsearch); }, this.doneTypingInterval);
});
}
typingTimer: number;
datafound = (data: any): void =>
{
let resultobject: ResultObject<kvkResult> = data;
let companies = resultobject.Records;
$(kvkfactory.ctlContainerName).empty();
$(kvkfactory.ctlContainerName).show();
$(kvkfactory.ctlContainerName).append('<table>');
$.each(companies, function (i, item) {
let entry: kvkResult = item;
$('table', kvkfactory.ctlContainerName).append("<tr class="auto-complete-item" data-modelid="" + entry.ModelId + " " data-contactpersonmodelid="" + entry.ContactPersonModelId +" "data-id="" + i + ""><td>" + entry.handelsnaam + "</td><td>" + entry.straat + " " + entry.huisnummer + "</td><td>" + entry.postcode + "</td><td>" + entry.plaats + "</td></tr>");
});
$(kvkfactory.ctlContainerName).append('</table>');
$(".auto-complete-item").on("click", function () {
let id: string = $(this).data("id");
let contactidnumber = 1;
let contactid: string = $(this).data('contactidnumber');
let modelid: string = $(this).data("modelid");
let contacts = companies[contactidnumber];
let organisationmodelid: string = null;
let count: number = 0;
let company: kvkResult = companies[id];
if (kvkfactory.ctlModelId != undefined && modelid!=null ) {
$(kvkfactory.ctlModelId).val(modelid);
$(kvkfactory.ctlContactPersonModelId).val(organisationmodelid);
}
$(kvkfactory.ctlCompanyName).val(company.handelsnaam);
$(kvkfactory.ctlCoc).val(company.dossiernummer);
$(kvkfactory.ctlAddress).val(company.straat);
$(kvkfactory.ctlAddressNumber).val(company.huisnummer);
$(kvkfactory.ctlAddressNumberSuffix).val(company.huisnummertoevoeging);
$(kvkfactory.ctlZipCode).val(company.postcode);
$(kvkfactory.ctlTown).val(company.plaats);
$(kvkfactory.ctlModelId).val(company.ModelId);
$(".auto-complete-container").hide();
Materialize.updateTextFields();
});
$(document).mouseup(function (e) {
let container = $(".auto-complete-container");
if (!container.is(e.target) // if the target of the click isn't the container...
&& container.has(e.target).length === 0) // ... nor a descendant of the container
{
container.hide();
}
});
};
doneTypingInterval: number = 800;
searchKvk = (webapiurlforsearch?: string): void => {
if ($(this.ctlCompanyName).val().length >= 3) {
if (webapiurlforsearch == undefined) {
webapiurlforsearch = variables.websiteurl + "/Kvk/Getdata";
}
let val = $(this.ctlCompanyName).val();
webApi.Execute(HttpRequestType.Get, webapiurlforsearch, { name: val }, this.datafound, null);
} else {
$(".auto-complete-container").empty();
}
}
}
}
let kvkfactory: Services.kvkFactory;
c# knockout.js
|
show 2 more comments
I'm using an external file where I get company data. The autocomplete works just fine, inputs get filled. But when I save the page into my database all the autocompleted fields don't get bind.
public Save(saveorder: Boolean): void {
let order: Order = ko.toJS(OrderPage.Instance().Order);
let date = OrderPage.Instance().Order.DateAccepted;
let removefromorderlines: Array<number> = ;
OrderPage.Instance().j$.each(order.OrderLines, (index: number, orderline: OrderLine) => {
// Check if there are any orderlines that don't have a modelid
if (orderline.ArticleModelId == null) {
removefromorderlines.push(index);
}
});
let previousindex: any = 0;
$.each(removefromorderlines, (index: number, orderlineindex: number) => {
order.OrderLines.splice(orderlineindex - previousindex, 1);
previousindex++;
});
if (saveorder == true) {
OrderPage.Instance().ToggleDisableButton('btnSaveOrder', true);
webApi.Execute(HttpRequestType.Post, '/Order/SaveOrder', order, OrderPage.Instance().OnSaveSuccess, OrderPage.Instance().OnSaveError);
}
The data that is missing is inside of 'Order'. The data gets picked up as soon as I change anything about the inputs. I've tried to use "valueUpdate: 'input'" but no success so far.
This is my autocomplete code:
namespace Services {
interface kvkResult {
handelsnaam: string;
dossiernummer: number;
straat: string;
huisnummer: number;
huisnummertoevoeging: string;
postcode: string;
plaats: string;
ModelId: string;
displayname: string;
ContactPersonModelId: string;
}
interface Contact {
ModelId: string;
displayname: string;
}
export class kvkFactory
{
private ctlCompanyName: string;
private ctlContainerName: string;
private ctlCoc: string;
private ctlAddress: string;
private ctlAddressNumber: string;
private ctlAddressNumberSuffix: string;
private ctlZipCode: string;
private ctlTown: string;
private ctlModelId: string;
private ctlContactPersonModelId: string;
/** Function to Fetch data from kvk api
@ctlSearch: Input control for coc organisations
@ctlAutocompleteContainer: output control to display organisations result.
@ctlCocClass: output text control for Kamer voor koophandel nummer
@ctlAddressClass: output text control for address organisation
@ctlZipCodeClass: output text contorl for zip code organisation
@ctlTownClass: output text contorl for town of settlement organisation
*/
constructor(ctlSearch: string, ctlAutocompleteContainer: string, ctlCompanyNameElement: string, ctlCocElement: string, ctlAddressElement: string, ctlAddressNumberElement: string, ctlAddressNumberSuffixElement: string, ctlZipCodeElement: string, ctlTownElement: string, ctlModelIdElement?: string, ctlContactPersonModelIdElement?: string, webapiurlforsearch?: string)
{
this.ctlCompanyName = ctlSearch;
this.ctlContainerName = ctlAutocompleteContainer;
this.ctlCompanyName = ctlCompanyNameElement;
this.ctlCoc = ctlCocElement;
this.ctlAddress = ctlAddressElement;
this.ctlAddressNumber = ctlAddressNumberElement;
this.ctlAddressNumberSuffix = ctlAddressNumberSuffixElement;
this.ctlZipCode = ctlZipCodeElement;
this.ctlTown = ctlTownElement;
this.ctlModelId = ctlModelIdElement;
this.ctlContactPersonModelId = ctlContactPersonModelIdElement;
$(this.ctlCompanyName).keyup(function () {
clearTimeout(this.typingTimer);
this.typingTimer = setTimeout(() => { kvkfactory.searchKvk(webapiurlforsearch); }, this.doneTypingInterval);
});
}
typingTimer: number;
datafound = (data: any): void =>
{
let resultobject: ResultObject<kvkResult> = data;
let companies = resultobject.Records;
$(kvkfactory.ctlContainerName).empty();
$(kvkfactory.ctlContainerName).show();
$(kvkfactory.ctlContainerName).append('<table>');
$.each(companies, function (i, item) {
let entry: kvkResult = item;
$('table', kvkfactory.ctlContainerName).append("<tr class="auto-complete-item" data-modelid="" + entry.ModelId + " " data-contactpersonmodelid="" + entry.ContactPersonModelId +" "data-id="" + i + ""><td>" + entry.handelsnaam + "</td><td>" + entry.straat + " " + entry.huisnummer + "</td><td>" + entry.postcode + "</td><td>" + entry.plaats + "</td></tr>");
});
$(kvkfactory.ctlContainerName).append('</table>');
$(".auto-complete-item").on("click", function () {
let id: string = $(this).data("id");
let contactidnumber = 1;
let contactid: string = $(this).data('contactidnumber');
let modelid: string = $(this).data("modelid");
let contacts = companies[contactidnumber];
let organisationmodelid: string = null;
let count: number = 0;
let company: kvkResult = companies[id];
if (kvkfactory.ctlModelId != undefined && modelid!=null ) {
$(kvkfactory.ctlModelId).val(modelid);
$(kvkfactory.ctlContactPersonModelId).val(organisationmodelid);
}
$(kvkfactory.ctlCompanyName).val(company.handelsnaam);
$(kvkfactory.ctlCoc).val(company.dossiernummer);
$(kvkfactory.ctlAddress).val(company.straat);
$(kvkfactory.ctlAddressNumber).val(company.huisnummer);
$(kvkfactory.ctlAddressNumberSuffix).val(company.huisnummertoevoeging);
$(kvkfactory.ctlZipCode).val(company.postcode);
$(kvkfactory.ctlTown).val(company.plaats);
$(kvkfactory.ctlModelId).val(company.ModelId);
$(".auto-complete-container").hide();
Materialize.updateTextFields();
});
$(document).mouseup(function (e) {
let container = $(".auto-complete-container");
if (!container.is(e.target) // if the target of the click isn't the container...
&& container.has(e.target).length === 0) // ... nor a descendant of the container
{
container.hide();
}
});
};
doneTypingInterval: number = 800;
searchKvk = (webapiurlforsearch?: string): void => {
if ($(this.ctlCompanyName).val().length >= 3) {
if (webapiurlforsearch == undefined) {
webapiurlforsearch = variables.websiteurl + "/Kvk/Getdata";
}
let val = $(this.ctlCompanyName).val();
webApi.Execute(HttpRequestType.Get, webapiurlforsearch, { name: val }, this.datafound, null);
} else {
$(".auto-complete-container").empty();
}
}
}
}
let kvkfactory: Services.kvkFactory;
c# knockout.js
How have you implemented autocomplete? With a plugin? Via a<datalist>
?
– user3297291
Nov 22 '18 at 13:40
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
If you change aninput
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how
– user3297291
Nov 22 '18 at 15:22
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
This answer explains how you can fix your issue. (After.val
, trigger a.change
to allow knockout to update its observables. Make sure to load jQuery before knockout).
– user3297291
Nov 23 '18 at 8:57
|
show 2 more comments
I'm using an external file where I get company data. The autocomplete works just fine, inputs get filled. But when I save the page into my database all the autocompleted fields don't get bind.
public Save(saveorder: Boolean): void {
let order: Order = ko.toJS(OrderPage.Instance().Order);
let date = OrderPage.Instance().Order.DateAccepted;
let removefromorderlines: Array<number> = ;
OrderPage.Instance().j$.each(order.OrderLines, (index: number, orderline: OrderLine) => {
// Check if there are any orderlines that don't have a modelid
if (orderline.ArticleModelId == null) {
removefromorderlines.push(index);
}
});
let previousindex: any = 0;
$.each(removefromorderlines, (index: number, orderlineindex: number) => {
order.OrderLines.splice(orderlineindex - previousindex, 1);
previousindex++;
});
if (saveorder == true) {
OrderPage.Instance().ToggleDisableButton('btnSaveOrder', true);
webApi.Execute(HttpRequestType.Post, '/Order/SaveOrder', order, OrderPage.Instance().OnSaveSuccess, OrderPage.Instance().OnSaveError);
}
The data that is missing is inside of 'Order'. The data gets picked up as soon as I change anything about the inputs. I've tried to use "valueUpdate: 'input'" but no success so far.
This is my autocomplete code:
namespace Services {
interface kvkResult {
handelsnaam: string;
dossiernummer: number;
straat: string;
huisnummer: number;
huisnummertoevoeging: string;
postcode: string;
plaats: string;
ModelId: string;
displayname: string;
ContactPersonModelId: string;
}
interface Contact {
ModelId: string;
displayname: string;
}
export class kvkFactory
{
private ctlCompanyName: string;
private ctlContainerName: string;
private ctlCoc: string;
private ctlAddress: string;
private ctlAddressNumber: string;
private ctlAddressNumberSuffix: string;
private ctlZipCode: string;
private ctlTown: string;
private ctlModelId: string;
private ctlContactPersonModelId: string;
/** Function to Fetch data from kvk api
@ctlSearch: Input control for coc organisations
@ctlAutocompleteContainer: output control to display organisations result.
@ctlCocClass: output text control for Kamer voor koophandel nummer
@ctlAddressClass: output text control for address organisation
@ctlZipCodeClass: output text contorl for zip code organisation
@ctlTownClass: output text contorl for town of settlement organisation
*/
constructor(ctlSearch: string, ctlAutocompleteContainer: string, ctlCompanyNameElement: string, ctlCocElement: string, ctlAddressElement: string, ctlAddressNumberElement: string, ctlAddressNumberSuffixElement: string, ctlZipCodeElement: string, ctlTownElement: string, ctlModelIdElement?: string, ctlContactPersonModelIdElement?: string, webapiurlforsearch?: string)
{
this.ctlCompanyName = ctlSearch;
this.ctlContainerName = ctlAutocompleteContainer;
this.ctlCompanyName = ctlCompanyNameElement;
this.ctlCoc = ctlCocElement;
this.ctlAddress = ctlAddressElement;
this.ctlAddressNumber = ctlAddressNumberElement;
this.ctlAddressNumberSuffix = ctlAddressNumberSuffixElement;
this.ctlZipCode = ctlZipCodeElement;
this.ctlTown = ctlTownElement;
this.ctlModelId = ctlModelIdElement;
this.ctlContactPersonModelId = ctlContactPersonModelIdElement;
$(this.ctlCompanyName).keyup(function () {
clearTimeout(this.typingTimer);
this.typingTimer = setTimeout(() => { kvkfactory.searchKvk(webapiurlforsearch); }, this.doneTypingInterval);
});
}
typingTimer: number;
datafound = (data: any): void =>
{
let resultobject: ResultObject<kvkResult> = data;
let companies = resultobject.Records;
$(kvkfactory.ctlContainerName).empty();
$(kvkfactory.ctlContainerName).show();
$(kvkfactory.ctlContainerName).append('<table>');
$.each(companies, function (i, item) {
let entry: kvkResult = item;
$('table', kvkfactory.ctlContainerName).append("<tr class="auto-complete-item" data-modelid="" + entry.ModelId + " " data-contactpersonmodelid="" + entry.ContactPersonModelId +" "data-id="" + i + ""><td>" + entry.handelsnaam + "</td><td>" + entry.straat + " " + entry.huisnummer + "</td><td>" + entry.postcode + "</td><td>" + entry.plaats + "</td></tr>");
});
$(kvkfactory.ctlContainerName).append('</table>');
$(".auto-complete-item").on("click", function () {
let id: string = $(this).data("id");
let contactidnumber = 1;
let contactid: string = $(this).data('contactidnumber');
let modelid: string = $(this).data("modelid");
let contacts = companies[contactidnumber];
let organisationmodelid: string = null;
let count: number = 0;
let company: kvkResult = companies[id];
if (kvkfactory.ctlModelId != undefined && modelid!=null ) {
$(kvkfactory.ctlModelId).val(modelid);
$(kvkfactory.ctlContactPersonModelId).val(organisationmodelid);
}
$(kvkfactory.ctlCompanyName).val(company.handelsnaam);
$(kvkfactory.ctlCoc).val(company.dossiernummer);
$(kvkfactory.ctlAddress).val(company.straat);
$(kvkfactory.ctlAddressNumber).val(company.huisnummer);
$(kvkfactory.ctlAddressNumberSuffix).val(company.huisnummertoevoeging);
$(kvkfactory.ctlZipCode).val(company.postcode);
$(kvkfactory.ctlTown).val(company.plaats);
$(kvkfactory.ctlModelId).val(company.ModelId);
$(".auto-complete-container").hide();
Materialize.updateTextFields();
});
$(document).mouseup(function (e) {
let container = $(".auto-complete-container");
if (!container.is(e.target) // if the target of the click isn't the container...
&& container.has(e.target).length === 0) // ... nor a descendant of the container
{
container.hide();
}
});
};
doneTypingInterval: number = 800;
searchKvk = (webapiurlforsearch?: string): void => {
if ($(this.ctlCompanyName).val().length >= 3) {
if (webapiurlforsearch == undefined) {
webapiurlforsearch = variables.websiteurl + "/Kvk/Getdata";
}
let val = $(this.ctlCompanyName).val();
webApi.Execute(HttpRequestType.Get, webapiurlforsearch, { name: val }, this.datafound, null);
} else {
$(".auto-complete-container").empty();
}
}
}
}
let kvkfactory: Services.kvkFactory;
c# knockout.js
I'm using an external file where I get company data. The autocomplete works just fine, inputs get filled. But when I save the page into my database all the autocompleted fields don't get bind.
public Save(saveorder: Boolean): void {
let order: Order = ko.toJS(OrderPage.Instance().Order);
let date = OrderPage.Instance().Order.DateAccepted;
let removefromorderlines: Array<number> = ;
OrderPage.Instance().j$.each(order.OrderLines, (index: number, orderline: OrderLine) => {
// Check if there are any orderlines that don't have a modelid
if (orderline.ArticleModelId == null) {
removefromorderlines.push(index);
}
});
let previousindex: any = 0;
$.each(removefromorderlines, (index: number, orderlineindex: number) => {
order.OrderLines.splice(orderlineindex - previousindex, 1);
previousindex++;
});
if (saveorder == true) {
OrderPage.Instance().ToggleDisableButton('btnSaveOrder', true);
webApi.Execute(HttpRequestType.Post, '/Order/SaveOrder', order, OrderPage.Instance().OnSaveSuccess, OrderPage.Instance().OnSaveError);
}
The data that is missing is inside of 'Order'. The data gets picked up as soon as I change anything about the inputs. I've tried to use "valueUpdate: 'input'" but no success so far.
This is my autocomplete code:
namespace Services {
interface kvkResult {
handelsnaam: string;
dossiernummer: number;
straat: string;
huisnummer: number;
huisnummertoevoeging: string;
postcode: string;
plaats: string;
ModelId: string;
displayname: string;
ContactPersonModelId: string;
}
interface Contact {
ModelId: string;
displayname: string;
}
export class kvkFactory
{
private ctlCompanyName: string;
private ctlContainerName: string;
private ctlCoc: string;
private ctlAddress: string;
private ctlAddressNumber: string;
private ctlAddressNumberSuffix: string;
private ctlZipCode: string;
private ctlTown: string;
private ctlModelId: string;
private ctlContactPersonModelId: string;
/** Function to Fetch data from kvk api
@ctlSearch: Input control for coc organisations
@ctlAutocompleteContainer: output control to display organisations result.
@ctlCocClass: output text control for Kamer voor koophandel nummer
@ctlAddressClass: output text control for address organisation
@ctlZipCodeClass: output text contorl for zip code organisation
@ctlTownClass: output text contorl for town of settlement organisation
*/
constructor(ctlSearch: string, ctlAutocompleteContainer: string, ctlCompanyNameElement: string, ctlCocElement: string, ctlAddressElement: string, ctlAddressNumberElement: string, ctlAddressNumberSuffixElement: string, ctlZipCodeElement: string, ctlTownElement: string, ctlModelIdElement?: string, ctlContactPersonModelIdElement?: string, webapiurlforsearch?: string)
{
this.ctlCompanyName = ctlSearch;
this.ctlContainerName = ctlAutocompleteContainer;
this.ctlCompanyName = ctlCompanyNameElement;
this.ctlCoc = ctlCocElement;
this.ctlAddress = ctlAddressElement;
this.ctlAddressNumber = ctlAddressNumberElement;
this.ctlAddressNumberSuffix = ctlAddressNumberSuffixElement;
this.ctlZipCode = ctlZipCodeElement;
this.ctlTown = ctlTownElement;
this.ctlModelId = ctlModelIdElement;
this.ctlContactPersonModelId = ctlContactPersonModelIdElement;
$(this.ctlCompanyName).keyup(function () {
clearTimeout(this.typingTimer);
this.typingTimer = setTimeout(() => { kvkfactory.searchKvk(webapiurlforsearch); }, this.doneTypingInterval);
});
}
typingTimer: number;
datafound = (data: any): void =>
{
let resultobject: ResultObject<kvkResult> = data;
let companies = resultobject.Records;
$(kvkfactory.ctlContainerName).empty();
$(kvkfactory.ctlContainerName).show();
$(kvkfactory.ctlContainerName).append('<table>');
$.each(companies, function (i, item) {
let entry: kvkResult = item;
$('table', kvkfactory.ctlContainerName).append("<tr class="auto-complete-item" data-modelid="" + entry.ModelId + " " data-contactpersonmodelid="" + entry.ContactPersonModelId +" "data-id="" + i + ""><td>" + entry.handelsnaam + "</td><td>" + entry.straat + " " + entry.huisnummer + "</td><td>" + entry.postcode + "</td><td>" + entry.plaats + "</td></tr>");
});
$(kvkfactory.ctlContainerName).append('</table>');
$(".auto-complete-item").on("click", function () {
let id: string = $(this).data("id");
let contactidnumber = 1;
let contactid: string = $(this).data('contactidnumber');
let modelid: string = $(this).data("modelid");
let contacts = companies[contactidnumber];
let organisationmodelid: string = null;
let count: number = 0;
let company: kvkResult = companies[id];
if (kvkfactory.ctlModelId != undefined && modelid!=null ) {
$(kvkfactory.ctlModelId).val(modelid);
$(kvkfactory.ctlContactPersonModelId).val(organisationmodelid);
}
$(kvkfactory.ctlCompanyName).val(company.handelsnaam);
$(kvkfactory.ctlCoc).val(company.dossiernummer);
$(kvkfactory.ctlAddress).val(company.straat);
$(kvkfactory.ctlAddressNumber).val(company.huisnummer);
$(kvkfactory.ctlAddressNumberSuffix).val(company.huisnummertoevoeging);
$(kvkfactory.ctlZipCode).val(company.postcode);
$(kvkfactory.ctlTown).val(company.plaats);
$(kvkfactory.ctlModelId).val(company.ModelId);
$(".auto-complete-container").hide();
Materialize.updateTextFields();
});
$(document).mouseup(function (e) {
let container = $(".auto-complete-container");
if (!container.is(e.target) // if the target of the click isn't the container...
&& container.has(e.target).length === 0) // ... nor a descendant of the container
{
container.hide();
}
});
};
doneTypingInterval: number = 800;
searchKvk = (webapiurlforsearch?: string): void => {
if ($(this.ctlCompanyName).val().length >= 3) {
if (webapiurlforsearch == undefined) {
webapiurlforsearch = variables.websiteurl + "/Kvk/Getdata";
}
let val = $(this.ctlCompanyName).val();
webApi.Execute(HttpRequestType.Get, webapiurlforsearch, { name: val }, this.datafound, null);
} else {
$(".auto-complete-container").empty();
}
}
}
}
let kvkfactory: Services.kvkFactory;
c# knockout.js
c# knockout.js
edited Nov 23 '18 at 8:32
Carlove
asked Nov 22 '18 at 13:02
CarloveCarlove
548
548
How have you implemented autocomplete? With a plugin? Via a<datalist>
?
– user3297291
Nov 22 '18 at 13:40
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
If you change aninput
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how
– user3297291
Nov 22 '18 at 15:22
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
This answer explains how you can fix your issue. (After.val
, trigger a.change
to allow knockout to update its observables. Make sure to load jQuery before knockout).
– user3297291
Nov 23 '18 at 8:57
|
show 2 more comments
How have you implemented autocomplete? With a plugin? Via a<datalist>
?
– user3297291
Nov 22 '18 at 13:40
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
If you change aninput
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how
– user3297291
Nov 22 '18 at 15:22
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
This answer explains how you can fix your issue. (After.val
, trigger a.change
to allow knockout to update its observables. Make sure to load jQuery before knockout).
– user3297291
Nov 23 '18 at 8:57
How have you implemented autocomplete? With a plugin? Via a
<datalist>
?– user3297291
Nov 22 '18 at 13:40
How have you implemented autocomplete? With a plugin? Via a
<datalist>
?– user3297291
Nov 22 '18 at 13:40
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
If you change an
input
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how– user3297291
Nov 22 '18 at 15:22
If you change an
input
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how– user3297291
Nov 22 '18 at 15:22
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
This answer explains how you can fix your issue. (After
.val
, trigger a .change
to allow knockout to update its observables. Make sure to load jQuery before knockout).– user3297291
Nov 23 '18 at 8:57
This answer explains how you can fix your issue. (After
.val
, trigger a .change
to allow knockout to update its observables. Make sure to load jQuery before knockout).– user3297291
Nov 23 '18 at 8:57
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431640%2fknockoutjs-doesnt-bind-fields-that-have-been-autocompleted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431640%2fknockoutjs-doesnt-bind-fields-that-have-been-autocompleted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y9Y3uukl,JuPYmzP,krv3v2Il8jg0,8YeQ6Cp0mu wvBJuH5P4etqnGWQAPKAYN,M,EDsH0OGctz Ai9rtk4tp tbD wQZkF
How have you implemented autocomplete? With a plugin? Via a
<datalist>
?– user3297291
Nov 22 '18 at 13:40
Through a simple class name. The autocomplete looks for the input names inside my html and replaces the values with the data from the autocomplete.
– Carlove
Nov 22 '18 at 14:38
If you change an
input
's value through code, without using knockout, you'll need to make sure knockout is updated. Knockout requires you to use a custom binding if you want to include custom input logic. If you share your autocomplete code I can show you how– user3297291
Nov 22 '18 at 15:22
Ah, Stackoverflow didn't notify me of your comment. The autocomplete code is held inside an external file, away from the KO code.
– Carlove
Nov 23 '18 at 8:31
This answer explains how you can fix your issue. (After
.val
, trigger a.change
to allow knockout to update its observables. Make sure to load jQuery before knockout).– user3297291
Nov 23 '18 at 8:57