Looking for help accessing Dynamic Distribution Lists using C# and ADWS
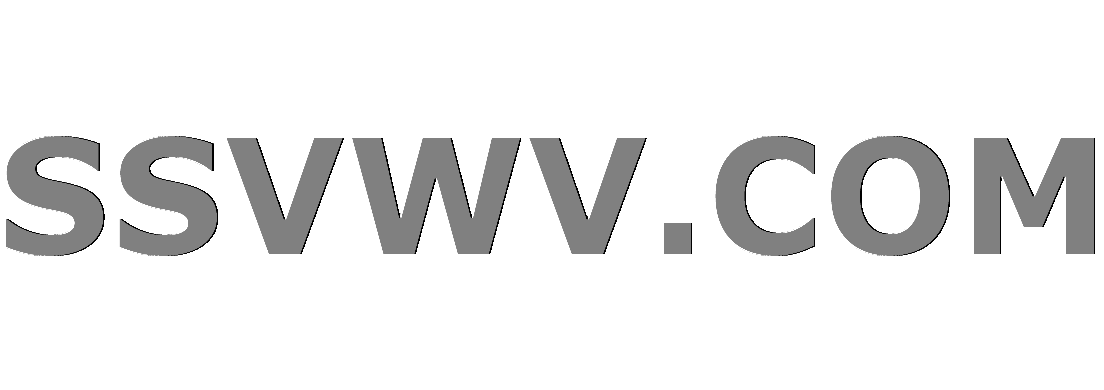
Multi tool use
I currently manage a program that analyses email data. One issue that came up early when developing this was that often an entry in the email's TO CC or BCC fields would not be an actual email address but a mailing list address of some sort. Because the application needs to accurately record how many people an email is being sent to, distribution lists have to be expanded so I can record the ultimate number of recipients the email is being sent to. I was able to deal easily with normal distribution lists but hit a snag with Dynamic Distribution Lists.
I have made a working routine that can do it. Here is the code:
/// <summary>
/// Get the List of A dynamic distribution Group in Key Value Pairs
/// the key is the CN
/// the value is the filter for the membership of that group
/// </summary>
/// <returns>Dictionary of strings </returns>
public List<DistributionList> GetDynamicDistributionLists(string strEmailAddress)
{
List<DistributionList> distributionLists = new List<DistributionList>();
DomName = "dc=" + Settings.ImpersonatedUserDomain.Replace(".", ",dc=");
using(var group = new DirectoryEntry("GC://" + DomName))
{
using(var searchRoot = new DirectoryEntry("GC://" + Settings.GlobalCatalogServer + "/" + DomName))
using(var searcher = new DirectorySearcher(searchRoot, "(&(ObjectClass=msExchDynamicDistributionList)(proxyAddresses=smtp:" + strEmailAddress.Trim() + "))"))
using(var results = searcher.FindAll())
{
foreach(SearchResult result in results)
{
if(result.Properties.Contains("cn") && result.Properties.Contains("msExchDynamicDLFilter"))
{
DistributionList dl = new DistributionList();
dl.DType = DLT.DDL;
dl.CN = result.Properties["cn"][0].ToString();
dl.FILORDN = result.Properties["msExchDynamicDLFilter"][0].ToString();
distributionLists.Add(dl);
}
}
}
}
return distributionLists;
}
This works but I have to have a VPN open into the Global Catalog Server if I want to run this code remotely to the site. Occasionally I will want to run remotely and all of my other code can run remotely using EWS without needing a VPN open. So I am trying to find a way of accessing the server using ADWS.
Could anyone help me locate some code that would allow me to run the above code using ADWS so I don't need a VPN open.
Thanks,
Siv
active-directory exchange-server exchangewebservices
add a comment |
I currently manage a program that analyses email data. One issue that came up early when developing this was that often an entry in the email's TO CC or BCC fields would not be an actual email address but a mailing list address of some sort. Because the application needs to accurately record how many people an email is being sent to, distribution lists have to be expanded so I can record the ultimate number of recipients the email is being sent to. I was able to deal easily with normal distribution lists but hit a snag with Dynamic Distribution Lists.
I have made a working routine that can do it. Here is the code:
/// <summary>
/// Get the List of A dynamic distribution Group in Key Value Pairs
/// the key is the CN
/// the value is the filter for the membership of that group
/// </summary>
/// <returns>Dictionary of strings </returns>
public List<DistributionList> GetDynamicDistributionLists(string strEmailAddress)
{
List<DistributionList> distributionLists = new List<DistributionList>();
DomName = "dc=" + Settings.ImpersonatedUserDomain.Replace(".", ",dc=");
using(var group = new DirectoryEntry("GC://" + DomName))
{
using(var searchRoot = new DirectoryEntry("GC://" + Settings.GlobalCatalogServer + "/" + DomName))
using(var searcher = new DirectorySearcher(searchRoot, "(&(ObjectClass=msExchDynamicDistributionList)(proxyAddresses=smtp:" + strEmailAddress.Trim() + "))"))
using(var results = searcher.FindAll())
{
foreach(SearchResult result in results)
{
if(result.Properties.Contains("cn") && result.Properties.Contains("msExchDynamicDLFilter"))
{
DistributionList dl = new DistributionList();
dl.DType = DLT.DDL;
dl.CN = result.Properties["cn"][0].ToString();
dl.FILORDN = result.Properties["msExchDynamicDLFilter"][0].ToString();
distributionLists.Add(dl);
}
}
}
}
return distributionLists;
}
This works but I have to have a VPN open into the Global Catalog Server if I want to run this code remotely to the site. Occasionally I will want to run remotely and all of my other code can run remotely using EWS without needing a VPN open. So I am trying to find a way of accessing the server using ADWS.
Could anyone help me locate some code that would allow me to run the above code using ADWS so I don't need a VPN open.
Thanks,
Siv
active-directory exchange-server exchangewebservices
add a comment |
I currently manage a program that analyses email data. One issue that came up early when developing this was that often an entry in the email's TO CC or BCC fields would not be an actual email address but a mailing list address of some sort. Because the application needs to accurately record how many people an email is being sent to, distribution lists have to be expanded so I can record the ultimate number of recipients the email is being sent to. I was able to deal easily with normal distribution lists but hit a snag with Dynamic Distribution Lists.
I have made a working routine that can do it. Here is the code:
/// <summary>
/// Get the List of A dynamic distribution Group in Key Value Pairs
/// the key is the CN
/// the value is the filter for the membership of that group
/// </summary>
/// <returns>Dictionary of strings </returns>
public List<DistributionList> GetDynamicDistributionLists(string strEmailAddress)
{
List<DistributionList> distributionLists = new List<DistributionList>();
DomName = "dc=" + Settings.ImpersonatedUserDomain.Replace(".", ",dc=");
using(var group = new DirectoryEntry("GC://" + DomName))
{
using(var searchRoot = new DirectoryEntry("GC://" + Settings.GlobalCatalogServer + "/" + DomName))
using(var searcher = new DirectorySearcher(searchRoot, "(&(ObjectClass=msExchDynamicDistributionList)(proxyAddresses=smtp:" + strEmailAddress.Trim() + "))"))
using(var results = searcher.FindAll())
{
foreach(SearchResult result in results)
{
if(result.Properties.Contains("cn") && result.Properties.Contains("msExchDynamicDLFilter"))
{
DistributionList dl = new DistributionList();
dl.DType = DLT.DDL;
dl.CN = result.Properties["cn"][0].ToString();
dl.FILORDN = result.Properties["msExchDynamicDLFilter"][0].ToString();
distributionLists.Add(dl);
}
}
}
}
return distributionLists;
}
This works but I have to have a VPN open into the Global Catalog Server if I want to run this code remotely to the site. Occasionally I will want to run remotely and all of my other code can run remotely using EWS without needing a VPN open. So I am trying to find a way of accessing the server using ADWS.
Could anyone help me locate some code that would allow me to run the above code using ADWS so I don't need a VPN open.
Thanks,
Siv
active-directory exchange-server exchangewebservices
I currently manage a program that analyses email data. One issue that came up early when developing this was that often an entry in the email's TO CC or BCC fields would not be an actual email address but a mailing list address of some sort. Because the application needs to accurately record how many people an email is being sent to, distribution lists have to be expanded so I can record the ultimate number of recipients the email is being sent to. I was able to deal easily with normal distribution lists but hit a snag with Dynamic Distribution Lists.
I have made a working routine that can do it. Here is the code:
/// <summary>
/// Get the List of A dynamic distribution Group in Key Value Pairs
/// the key is the CN
/// the value is the filter for the membership of that group
/// </summary>
/// <returns>Dictionary of strings </returns>
public List<DistributionList> GetDynamicDistributionLists(string strEmailAddress)
{
List<DistributionList> distributionLists = new List<DistributionList>();
DomName = "dc=" + Settings.ImpersonatedUserDomain.Replace(".", ",dc=");
using(var group = new DirectoryEntry("GC://" + DomName))
{
using(var searchRoot = new DirectoryEntry("GC://" + Settings.GlobalCatalogServer + "/" + DomName))
using(var searcher = new DirectorySearcher(searchRoot, "(&(ObjectClass=msExchDynamicDistributionList)(proxyAddresses=smtp:" + strEmailAddress.Trim() + "))"))
using(var results = searcher.FindAll())
{
foreach(SearchResult result in results)
{
if(result.Properties.Contains("cn") && result.Properties.Contains("msExchDynamicDLFilter"))
{
DistributionList dl = new DistributionList();
dl.DType = DLT.DDL;
dl.CN = result.Properties["cn"][0].ToString();
dl.FILORDN = result.Properties["msExchDynamicDLFilter"][0].ToString();
distributionLists.Add(dl);
}
}
}
}
return distributionLists;
}
This works but I have to have a VPN open into the Global Catalog Server if I want to run this code remotely to the site. Occasionally I will want to run remotely and all of my other code can run remotely using EWS without needing a VPN open. So I am trying to find a way of accessing the server using ADWS.
Could anyone help me locate some code that would allow me to run the above code using ADWS so I don't need a VPN open.
Thanks,
Siv
active-directory exchange-server exchangewebservices
active-directory exchange-server exchangewebservices
asked Nov 22 '18 at 12:44
SivSiv
176
176
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
So I assume a firewall is blocking access from the outside world, which is understandable. When you use "GC://", it uses port 3268 by default. You can try other ports to see if they are open:
- 389: The default port when using
LDAP://
- 636: LDAP over SSL. You use it like
LDAP://domain.com:636
- 3269: GC over SSL. You use it like
LDAP://domain.com:3269
It's possible either 636 or 3269 is open, but there might be complications depending on the SSL certificate that is used.
If all of those are blocked, then yes, you'll have to use ADWS. How you use it from .NET is very different. There is a write-up here: http://samirvaidya.blogspot.com/2012/06/using-active-directory-web-services-in.html
But basically, you create a Service Reference in your project and use it like a web service rather than using DirectoryEntry
.
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have aDistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.
– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still useDirectoryEntry
.
– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431322%2flooking-for-help-accessing-dynamic-distribution-lists-using-c-sharp-and-adws%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
So I assume a firewall is blocking access from the outside world, which is understandable. When you use "GC://", it uses port 3268 by default. You can try other ports to see if they are open:
- 389: The default port when using
LDAP://
- 636: LDAP over SSL. You use it like
LDAP://domain.com:636
- 3269: GC over SSL. You use it like
LDAP://domain.com:3269
It's possible either 636 or 3269 is open, but there might be complications depending on the SSL certificate that is used.
If all of those are blocked, then yes, you'll have to use ADWS. How you use it from .NET is very different. There is a write-up here: http://samirvaidya.blogspot.com/2012/06/using-active-directory-web-services-in.html
But basically, you create a Service Reference in your project and use it like a web service rather than using DirectoryEntry
.
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have aDistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.
– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still useDirectoryEntry
.
– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
|
show 1 more comment
So I assume a firewall is blocking access from the outside world, which is understandable. When you use "GC://", it uses port 3268 by default. You can try other ports to see if they are open:
- 389: The default port when using
LDAP://
- 636: LDAP over SSL. You use it like
LDAP://domain.com:636
- 3269: GC over SSL. You use it like
LDAP://domain.com:3269
It's possible either 636 or 3269 is open, but there might be complications depending on the SSL certificate that is used.
If all of those are blocked, then yes, you'll have to use ADWS. How you use it from .NET is very different. There is a write-up here: http://samirvaidya.blogspot.com/2012/06/using-active-directory-web-services-in.html
But basically, you create a Service Reference in your project and use it like a web service rather than using DirectoryEntry
.
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have aDistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.
– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still useDirectoryEntry
.
– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
|
show 1 more comment
So I assume a firewall is blocking access from the outside world, which is understandable. When you use "GC://", it uses port 3268 by default. You can try other ports to see if they are open:
- 389: The default port when using
LDAP://
- 636: LDAP over SSL. You use it like
LDAP://domain.com:636
- 3269: GC over SSL. You use it like
LDAP://domain.com:3269
It's possible either 636 or 3269 is open, but there might be complications depending on the SSL certificate that is used.
If all of those are blocked, then yes, you'll have to use ADWS. How you use it from .NET is very different. There is a write-up here: http://samirvaidya.blogspot.com/2012/06/using-active-directory-web-services-in.html
But basically, you create a Service Reference in your project and use it like a web service rather than using DirectoryEntry
.
So I assume a firewall is blocking access from the outside world, which is understandable. When you use "GC://", it uses port 3268 by default. You can try other ports to see if they are open:
- 389: The default port when using
LDAP://
- 636: LDAP over SSL. You use it like
LDAP://domain.com:636
- 3269: GC over SSL. You use it like
LDAP://domain.com:3269
It's possible either 636 or 3269 is open, but there might be complications depending on the SSL certificate that is used.
If all of those are blocked, then yes, you'll have to use ADWS. How you use it from .NET is very different. There is a write-up here: http://samirvaidya.blogspot.com/2012/06/using-active-directory-web-services-in.html
But basically, you create a Service Reference in your project and use it like a web service rather than using DirectoryEntry
.
answered Nov 22 '18 at 15:37
Gabriel LuciGabriel Luci
11.4k11525
11.4k11525
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have aDistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.
– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still useDirectoryEntry
.
– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
|
show 1 more comment
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have aDistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.
– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still useDirectoryEntry
.
– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
Gabriel, Thanks for your reply. This is a generic program that may be used by many customers so I need a method that allows me to connect using parameters that I can put into a settings program so I can't do something highly specific to a single server, it has to work against any server that want to connect to a long as I can store the user names and passwords that allow me to access it. So I am not sure this would work. I did read that article before posting here.
– Siv
Nov 22 '18 at 16:12
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have a
DistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.– Gabriel Luci
Nov 22 '18 at 16:51
There is no single method that will work with both LDAP and ADWS unfortunately. If you want to support both, you will need two implementations. You can always hide the implementation behind your own classes, like have a
DistributionList
class that you define yourself, and in the background you populate it via LDAP or ADWS, depending on what your config file says.– Gabriel Luci
Nov 22 '18 at 16:51
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still use
DirectoryEntry
.– Gabriel Luci
Nov 22 '18 at 17:08
But try the other LDAP ports. Maybe one of them is open. If so, you can just keep the port as a config setting and still use
DirectoryEntry
.– Gabriel Luci
Nov 22 '18 at 17:08
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
Gabriel, I am not sure what I want to use, as long as I can expand a dynamic distribution group to its constituent email addresses, I will just use whatever works remotely, so if you have an idea on how to achieve that I am all ears. I just want to get rid of the need for a VPN to gain access to the Global Catalog Server to be able to do it the way I am now.
– Siv
Nov 22 '18 at 17:12
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
In most cases I will be dealing with corporate clients who may want me to run my application remotely and gather the email information for them. They will not want me requesting that they open ports to do it I suspect. So I am looking for a technique that uses the ones that are likely to be open like 443 for the https stuff like EWS does. I do not have any experience using ADWS and I had assumed you could access the AD server via that port, I just can't locate any good documentation on how to do that for the purpose I am after.
– Siv
Nov 22 '18 at 17:17
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431322%2flooking-for-help-accessing-dynamic-distribution-lists-using-c-sharp-and-adws%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I5X0,B6sObfy HcHPYnGj0sNeIsQzWdoSYK5B7CUOYLut4TRdWt 5vrFiXppMaV,Z1Sju0ZQl5