Kotlin Data class copy extension
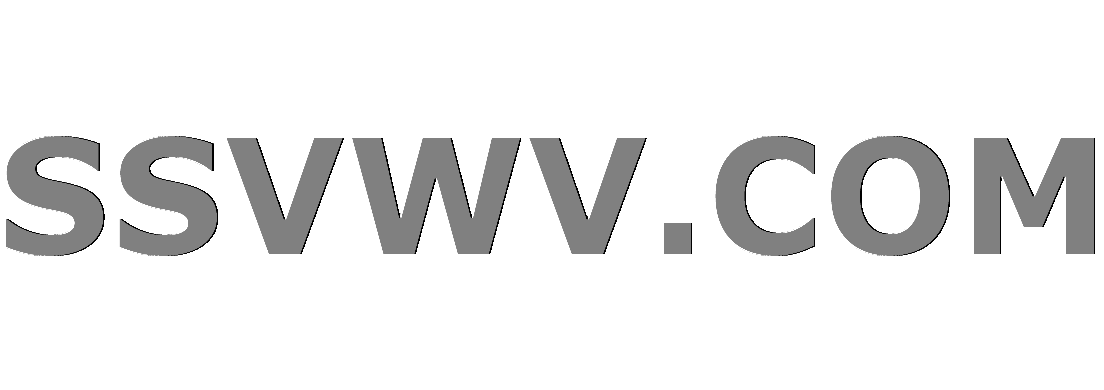
Multi tool use
I am trying to find a solution for a nice kotlin data class solution. I have already this:
data class Object(
var classMember: Boolean,
var otherClassMember: Boolean,
var example: Int = 0) {
fun set(block: Object.() -> kotlin.Unit): Object {
val copiedObject = this.copy()
copiedObject.apply {
block()
}
return copiedObject
}
fun touch(block: Object.() -> kotlin.Unit): Object {
return this.set {
classMember = true
otherClassMember = false
block() }
}
}
val test = Object(true,true,1)
val changedTest = test.touch { example = 2 }
the result of this method is that the changedTest
object has classMember = true
, otherClassMember = false
and example = 2
The problem with this solution is, the class properties are not immutable with var
declaration. Does somebody have an idea how to optimize my methods to change var
to val
?
kotlin immutability data-class
add a comment |
I am trying to find a solution for a nice kotlin data class solution. I have already this:
data class Object(
var classMember: Boolean,
var otherClassMember: Boolean,
var example: Int = 0) {
fun set(block: Object.() -> kotlin.Unit): Object {
val copiedObject = this.copy()
copiedObject.apply {
block()
}
return copiedObject
}
fun touch(block: Object.() -> kotlin.Unit): Object {
return this.set {
classMember = true
otherClassMember = false
block() }
}
}
val test = Object(true,true,1)
val changedTest = test.touch { example = 2 }
the result of this method is that the changedTest
object has classMember = true
, otherClassMember = false
and example = 2
The problem with this solution is, the class properties are not immutable with var
declaration. Does somebody have an idea how to optimize my methods to change var
to val
?
kotlin immutability data-class
why don't you just use:someObj.copy( classMember = true, otherClassMember = false )
(assuming something like:val someObj = Object(....);
)?
– Roland
Nov 22 '18 at 13:14
1
Kotlin'scopy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.
– Andrey Ilyunin
Nov 22 '18 at 13:17
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21
add a comment |
I am trying to find a solution for a nice kotlin data class solution. I have already this:
data class Object(
var classMember: Boolean,
var otherClassMember: Boolean,
var example: Int = 0) {
fun set(block: Object.() -> kotlin.Unit): Object {
val copiedObject = this.copy()
copiedObject.apply {
block()
}
return copiedObject
}
fun touch(block: Object.() -> kotlin.Unit): Object {
return this.set {
classMember = true
otherClassMember = false
block() }
}
}
val test = Object(true,true,1)
val changedTest = test.touch { example = 2 }
the result of this method is that the changedTest
object has classMember = true
, otherClassMember = false
and example = 2
The problem with this solution is, the class properties are not immutable with var
declaration. Does somebody have an idea how to optimize my methods to change var
to val
?
kotlin immutability data-class
I am trying to find a solution for a nice kotlin data class solution. I have already this:
data class Object(
var classMember: Boolean,
var otherClassMember: Boolean,
var example: Int = 0) {
fun set(block: Object.() -> kotlin.Unit): Object {
val copiedObject = this.copy()
copiedObject.apply {
block()
}
return copiedObject
}
fun touch(block: Object.() -> kotlin.Unit): Object {
return this.set {
classMember = true
otherClassMember = false
block() }
}
}
val test = Object(true,true,1)
val changedTest = test.touch { example = 2 }
the result of this method is that the changedTest
object has classMember = true
, otherClassMember = false
and example = 2
The problem with this solution is, the class properties are not immutable with var
declaration. Does somebody have an idea how to optimize my methods to change var
to val
?
kotlin immutability data-class
kotlin immutability data-class
edited Nov 22 '18 at 18:33
Jayson Minard
41.6k19114175
41.6k19114175
asked Nov 22 '18 at 13:06
davidOharadavidOhara
42821235
42821235
why don't you just use:someObj.copy( classMember = true, otherClassMember = false )
(assuming something like:val someObj = Object(....);
)?
– Roland
Nov 22 '18 at 13:14
1
Kotlin'scopy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.
– Andrey Ilyunin
Nov 22 '18 at 13:17
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21
add a comment |
why don't you just use:someObj.copy( classMember = true, otherClassMember = false )
(assuming something like:val someObj = Object(....);
)?
– Roland
Nov 22 '18 at 13:14
1
Kotlin'scopy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.
– Andrey Ilyunin
Nov 22 '18 at 13:17
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21
why don't you just use:
someObj.copy( classMember = true, otherClassMember = false )
(assuming something like: val someObj = Object(....);
)?– Roland
Nov 22 '18 at 13:14
why don't you just use:
someObj.copy( classMember = true, otherClassMember = false )
(assuming something like: val someObj = Object(....);
)?– Roland
Nov 22 '18 at 13:14
1
1
Kotlin's
copy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.– Andrey Ilyunin
Nov 22 '18 at 13:17
Kotlin's
copy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.– Andrey Ilyunin
Nov 22 '18 at 13:17
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21
add a comment |
2 Answers
2
active
oldest
votes
val
says that a variable can't change it's value after initialization at the definition point. Kotlin's generated copy
method does not modify an existing copy after construction: this method actually uses retrieved values from an object, replaces these values with ones that provided in copy
method (if any), and after that just constructs a new object using these values.
So, it is not possible to perform such an optimization if you are going to change object's state after construction.
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloadedcopy
-functions...)
– Roland
Nov 22 '18 at 13:56
add a comment |
If I understood what you want correctly, you can do
data class Object(
val classMember: Boolean,
val otherClassMember: Boolean,
val example: Int = 0) {
fun touch(example: Int = this.example): Object {
return copy(
classMember = true,
otherClassMember = false,
example = example)
}
}
val test = Object(true,true,1)
val changedTest = test.touch(example = 2)
Though you need to repeat parameters other than classMember
and otherClassMember
but without reflection you can't do better.
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431720%2fkotlin-data-class-copy-extension%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
val
says that a variable can't change it's value after initialization at the definition point. Kotlin's generated copy
method does not modify an existing copy after construction: this method actually uses retrieved values from an object, replaces these values with ones that provided in copy
method (if any), and after that just constructs a new object using these values.
So, it is not possible to perform such an optimization if you are going to change object's state after construction.
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloadedcopy
-functions...)
– Roland
Nov 22 '18 at 13:56
add a comment |
val
says that a variable can't change it's value after initialization at the definition point. Kotlin's generated copy
method does not modify an existing copy after construction: this method actually uses retrieved values from an object, replaces these values with ones that provided in copy
method (if any), and after that just constructs a new object using these values.
So, it is not possible to perform such an optimization if you are going to change object's state after construction.
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloadedcopy
-functions...)
– Roland
Nov 22 '18 at 13:56
add a comment |
val
says that a variable can't change it's value after initialization at the definition point. Kotlin's generated copy
method does not modify an existing copy after construction: this method actually uses retrieved values from an object, replaces these values with ones that provided in copy
method (if any), and after that just constructs a new object using these values.
So, it is not possible to perform such an optimization if you are going to change object's state after construction.
val
says that a variable can't change it's value after initialization at the definition point. Kotlin's generated copy
method does not modify an existing copy after construction: this method actually uses retrieved values from an object, replaces these values with ones that provided in copy
method (if any), and after that just constructs a new object using these values.
So, it is not possible to perform such an optimization if you are going to change object's state after construction.
answered Nov 22 '18 at 13:50


Andrey IlyuninAndrey Ilyunin
1,412221
1,412221
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloadedcopy
-functions...)
– Roland
Nov 22 '18 at 13:56
add a comment |
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloadedcopy
-functions...)
– Roland
Nov 22 '18 at 13:56
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloaded
copy
-functions...)– Roland
Nov 22 '18 at 13:56
... except... you are willing to use reflection... with reflection near everything becomes possible... (this should also be seen as disclaimer that you shouldn't supply such a function that uses reflection to fill up the values, when there are already the overloaded
copy
-functions...)– Roland
Nov 22 '18 at 13:56
add a comment |
If I understood what you want correctly, you can do
data class Object(
val classMember: Boolean,
val otherClassMember: Boolean,
val example: Int = 0) {
fun touch(example: Int = this.example): Object {
return copy(
classMember = true,
otherClassMember = false,
example = example)
}
}
val test = Object(true,true,1)
val changedTest = test.touch(example = 2)
Though you need to repeat parameters other than classMember
and otherClassMember
but without reflection you can't do better.
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
add a comment |
If I understood what you want correctly, you can do
data class Object(
val classMember: Boolean,
val otherClassMember: Boolean,
val example: Int = 0) {
fun touch(example: Int = this.example): Object {
return copy(
classMember = true,
otherClassMember = false,
example = example)
}
}
val test = Object(true,true,1)
val changedTest = test.touch(example = 2)
Though you need to repeat parameters other than classMember
and otherClassMember
but without reflection you can't do better.
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
add a comment |
If I understood what you want correctly, you can do
data class Object(
val classMember: Boolean,
val otherClassMember: Boolean,
val example: Int = 0) {
fun touch(example: Int = this.example): Object {
return copy(
classMember = true,
otherClassMember = false,
example = example)
}
}
val test = Object(true,true,1)
val changedTest = test.touch(example = 2)
Though you need to repeat parameters other than classMember
and otherClassMember
but without reflection you can't do better.
If I understood what you want correctly, you can do
data class Object(
val classMember: Boolean,
val otherClassMember: Boolean,
val example: Int = 0) {
fun touch(example: Int = this.example): Object {
return copy(
classMember = true,
otherClassMember = false,
example = example)
}
}
val test = Object(true,true,1)
val changedTest = test.touch(example = 2)
Though you need to repeat parameters other than classMember
and otherClassMember
but without reflection you can't do better.
answered Nov 22 '18 at 14:40
Alexey RomanovAlexey Romanov
110k26215356
110k26215356
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
add a comment |
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
Yes, that would be possible but I want to have it more generic. I want to have the possibility to change every class member, but I do not want to have all of those as parameter in the touch method. How would a solution with reflection look like?
– davidOhara
Nov 22 '18 at 14:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431720%2fkotlin-data-class-copy-extension%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oY,o6lUI7xC 1FhEUr2iYT,LfE pp y vV8wCXR2rb,ZJ,wLHwU Yen5qvjF IIBDcmWbwLWag9Yq,nAji9AUN3KJVvzKSa6j,ng
why don't you just use:
someObj.copy( classMember = true, otherClassMember = false )
(assuming something like:val someObj = Object(....);
)?– Roland
Nov 22 '18 at 13:14
1
Kotlin's
copy
method for data classes doesn't copy an object as is and change it's state afterwards. Object construction is the last step of the method. Just decompile Kotlin bytecode and you'll see that here is no way to perform such an optimization.– Andrey Ilyunin
Nov 22 '18 at 13:17
@Roland Because I want to use a method which always change two values of two properties additionally to what the user wants to change...
– davidOhara
Nov 22 '18 at 13:19
I updated my question with a complete example...
– davidOhara
Nov 22 '18 at 13:21