Wait for redux action to finish dispatching when using redux saga
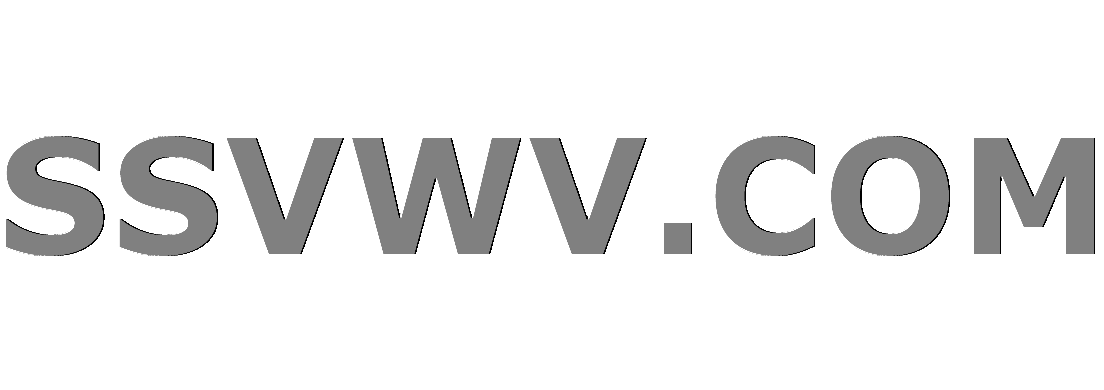
Multi tool use
I have a redux saga setup which works fine. One of my dispatches is to create a new order, then once that has been created I want to do things with the updated state.
// this.props.userOrders =
dispatch(actions.createOrder(object))
doSomethingWith(this.props.userOrders)
Since the createOrder action triggers a redux saga which calls an API, there is a delay, so this.props.userOrders is not updated before my function doSomethingWith is called. I could set a timeout, but that doesn't seem like a sustainable idea.
I have read the similar questions on Stack Overflow, and have tried implementing the methods where relevant, but I can't seem to get it working. I'm hoping with my code below that someone can just add a couple of lines which will do it.
Here are the relevant other files:
actions.js
export const createUserOrder = (data) => ({
type: 'CREATE_USER_ORDER',
data
})
Sagas.js
function * createUserOrder () {
yield takeEvery('CREATE_USER_ORDER', callCreateUserOrder)
}
export function * callCreateUserOrder (newUserOrderAction) {
try {
const data = newUserOrderAction.data
const newUserOrder = yield call(api.createUserOrder, data)
yield put({type: 'CREATE_USER_ORDER_SUCCEEDED', newUserOrder: newUserOrder})
} catch (error) {
yield put({type: 'CREATE_USER_ORDER_FAILED', error})
}
}
Api.js
export const createUserOrder = (data) => new Promise((resolve, reject) => {
api.post('/userOrders/', data, {headers: {'Content-Type': 'application/json'}})
.then((response) => {
if (!response.ok) {
reject(response)
} else {
resolve(data)
}
})
})
orders reducer:
case 'CREATE_USER_ORDER_SUCCEEDED':
if (action.newUserOrder) {
let newArray = state.slice()
newArray.push(action.newUserOrder)
return newArray
} else {
return state
}
reactjs redux redux-saga
add a comment |
I have a redux saga setup which works fine. One of my dispatches is to create a new order, then once that has been created I want to do things with the updated state.
// this.props.userOrders =
dispatch(actions.createOrder(object))
doSomethingWith(this.props.userOrders)
Since the createOrder action triggers a redux saga which calls an API, there is a delay, so this.props.userOrders is not updated before my function doSomethingWith is called. I could set a timeout, but that doesn't seem like a sustainable idea.
I have read the similar questions on Stack Overflow, and have tried implementing the methods where relevant, but I can't seem to get it working. I'm hoping with my code below that someone can just add a couple of lines which will do it.
Here are the relevant other files:
actions.js
export const createUserOrder = (data) => ({
type: 'CREATE_USER_ORDER',
data
})
Sagas.js
function * createUserOrder () {
yield takeEvery('CREATE_USER_ORDER', callCreateUserOrder)
}
export function * callCreateUserOrder (newUserOrderAction) {
try {
const data = newUserOrderAction.data
const newUserOrder = yield call(api.createUserOrder, data)
yield put({type: 'CREATE_USER_ORDER_SUCCEEDED', newUserOrder: newUserOrder})
} catch (error) {
yield put({type: 'CREATE_USER_ORDER_FAILED', error})
}
}
Api.js
export const createUserOrder = (data) => new Promise((resolve, reject) => {
api.post('/userOrders/', data, {headers: {'Content-Type': 'application/json'}})
.then((response) => {
if (!response.ok) {
reject(response)
} else {
resolve(data)
}
})
})
orders reducer:
case 'CREATE_USER_ORDER_SUCCEEDED':
if (action.newUserOrder) {
let newArray = state.slice()
newArray.push(action.newUserOrder)
return newArray
} else {
return state
}
reactjs redux redux-saga
add a comment |
I have a redux saga setup which works fine. One of my dispatches is to create a new order, then once that has been created I want to do things with the updated state.
// this.props.userOrders =
dispatch(actions.createOrder(object))
doSomethingWith(this.props.userOrders)
Since the createOrder action triggers a redux saga which calls an API, there is a delay, so this.props.userOrders is not updated before my function doSomethingWith is called. I could set a timeout, but that doesn't seem like a sustainable idea.
I have read the similar questions on Stack Overflow, and have tried implementing the methods where relevant, but I can't seem to get it working. I'm hoping with my code below that someone can just add a couple of lines which will do it.
Here are the relevant other files:
actions.js
export const createUserOrder = (data) => ({
type: 'CREATE_USER_ORDER',
data
})
Sagas.js
function * createUserOrder () {
yield takeEvery('CREATE_USER_ORDER', callCreateUserOrder)
}
export function * callCreateUserOrder (newUserOrderAction) {
try {
const data = newUserOrderAction.data
const newUserOrder = yield call(api.createUserOrder, data)
yield put({type: 'CREATE_USER_ORDER_SUCCEEDED', newUserOrder: newUserOrder})
} catch (error) {
yield put({type: 'CREATE_USER_ORDER_FAILED', error})
}
}
Api.js
export const createUserOrder = (data) => new Promise((resolve, reject) => {
api.post('/userOrders/', data, {headers: {'Content-Type': 'application/json'}})
.then((response) => {
if (!response.ok) {
reject(response)
} else {
resolve(data)
}
})
})
orders reducer:
case 'CREATE_USER_ORDER_SUCCEEDED':
if (action.newUserOrder) {
let newArray = state.slice()
newArray.push(action.newUserOrder)
return newArray
} else {
return state
}
reactjs redux redux-saga
I have a redux saga setup which works fine. One of my dispatches is to create a new order, then once that has been created I want to do things with the updated state.
// this.props.userOrders =
dispatch(actions.createOrder(object))
doSomethingWith(this.props.userOrders)
Since the createOrder action triggers a redux saga which calls an API, there is a delay, so this.props.userOrders is not updated before my function doSomethingWith is called. I could set a timeout, but that doesn't seem like a sustainable idea.
I have read the similar questions on Stack Overflow, and have tried implementing the methods where relevant, but I can't seem to get it working. I'm hoping with my code below that someone can just add a couple of lines which will do it.
Here are the relevant other files:
actions.js
export const createUserOrder = (data) => ({
type: 'CREATE_USER_ORDER',
data
})
Sagas.js
function * createUserOrder () {
yield takeEvery('CREATE_USER_ORDER', callCreateUserOrder)
}
export function * callCreateUserOrder (newUserOrderAction) {
try {
const data = newUserOrderAction.data
const newUserOrder = yield call(api.createUserOrder, data)
yield put({type: 'CREATE_USER_ORDER_SUCCEEDED', newUserOrder: newUserOrder})
} catch (error) {
yield put({type: 'CREATE_USER_ORDER_FAILED', error})
}
}
Api.js
export const createUserOrder = (data) => new Promise((resolve, reject) => {
api.post('/userOrders/', data, {headers: {'Content-Type': 'application/json'}})
.then((response) => {
if (!response.ok) {
reject(response)
} else {
resolve(data)
}
})
})
orders reducer:
case 'CREATE_USER_ORDER_SUCCEEDED':
if (action.newUserOrder) {
let newArray = state.slice()
newArray.push(action.newUserOrder)
return newArray
} else {
return state
}
reactjs redux redux-saga
reactjs redux redux-saga
edited Nov 22 '18 at 14:40
moinhaque
asked Nov 22 '18 at 13:05


moinhaquemoinhaque
688
688
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This feels like an XY Problem. You shouldn't be "waiting" inside a component's lifecycle function / event handler at any point, but rather make use of the current state of the store.
If I understand correctly, this is your current flow:
You dispatch an action
CREATE_USER_ORDER
in your React component. This action is consumed by yourcallCreateUserOrder
saga. When your create order saga is complete, it dispatches another "completed" action, which you already have asCREATE_USER_ORDER_SUCCEEDED
.
What you should now add is the proper reducer/selector to handle your CREATE_USER_ORDER_SUCCEEDED
:
This CREATE_USER_ORDER_SUCCEEDED
action should be handled by your reducer to create a new state where some "orders" property in your state is populated. This can be connected directly to your component via a selector, at which point your component will be re-rendered and this.props.userOrders
is populated.
Example:
component
class OrderList extends React.PureComponent {
static propTypes = {
userOrders: PropTypes.array.isRequired,
createOrder: PropTypes.func.isRequired,
}
addOrder() {
this.props.createOrder({...})
}
render() {
return (
<Wrapper>
<Button onClick={this.addOrder}>Add Order</Button>
<List>{this.props.userOrders.map(order => <Item>{order.name}</Item>)}</List>
</Wrapper>
)
}
}
const mapStateToProps = state => ({
userOrders: state.get('userOrders'),
})
const mapDispatchToProps = {
createOrder: () => ({ type: 'CREATE_ORDER', payload: {} }),
}
export default connect(mapStateToProps, mapDispatchToProps)(OrderList)
reducer
case 'CREATE_USER_ORDER_SUCCEEDED':
return state.update('userOrders',
orders => orders.concat([payload.newUserOrder])
)
If you really do need side-effects, then add those side-effects to your saga, or create a new saga that takes the SUCCESS action.
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger arender()
... So how to handle this with dispatch/selectors:this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431687%2fwait-for-redux-action-to-finish-dispatching-when-using-redux-saga%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This feels like an XY Problem. You shouldn't be "waiting" inside a component's lifecycle function / event handler at any point, but rather make use of the current state of the store.
If I understand correctly, this is your current flow:
You dispatch an action
CREATE_USER_ORDER
in your React component. This action is consumed by yourcallCreateUserOrder
saga. When your create order saga is complete, it dispatches another "completed" action, which you already have asCREATE_USER_ORDER_SUCCEEDED
.
What you should now add is the proper reducer/selector to handle your CREATE_USER_ORDER_SUCCEEDED
:
This CREATE_USER_ORDER_SUCCEEDED
action should be handled by your reducer to create a new state where some "orders" property in your state is populated. This can be connected directly to your component via a selector, at which point your component will be re-rendered and this.props.userOrders
is populated.
Example:
component
class OrderList extends React.PureComponent {
static propTypes = {
userOrders: PropTypes.array.isRequired,
createOrder: PropTypes.func.isRequired,
}
addOrder() {
this.props.createOrder({...})
}
render() {
return (
<Wrapper>
<Button onClick={this.addOrder}>Add Order</Button>
<List>{this.props.userOrders.map(order => <Item>{order.name}</Item>)}</List>
</Wrapper>
)
}
}
const mapStateToProps = state => ({
userOrders: state.get('userOrders'),
})
const mapDispatchToProps = {
createOrder: () => ({ type: 'CREATE_ORDER', payload: {} }),
}
export default connect(mapStateToProps, mapDispatchToProps)(OrderList)
reducer
case 'CREATE_USER_ORDER_SUCCEEDED':
return state.update('userOrders',
orders => orders.concat([payload.newUserOrder])
)
If you really do need side-effects, then add those side-effects to your saga, or create a new saga that takes the SUCCESS action.
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger arender()
... So how to handle this with dispatch/selectors:this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
|
show 1 more comment
This feels like an XY Problem. You shouldn't be "waiting" inside a component's lifecycle function / event handler at any point, but rather make use of the current state of the store.
If I understand correctly, this is your current flow:
You dispatch an action
CREATE_USER_ORDER
in your React component. This action is consumed by yourcallCreateUserOrder
saga. When your create order saga is complete, it dispatches another "completed" action, which you already have asCREATE_USER_ORDER_SUCCEEDED
.
What you should now add is the proper reducer/selector to handle your CREATE_USER_ORDER_SUCCEEDED
:
This CREATE_USER_ORDER_SUCCEEDED
action should be handled by your reducer to create a new state where some "orders" property in your state is populated. This can be connected directly to your component via a selector, at which point your component will be re-rendered and this.props.userOrders
is populated.
Example:
component
class OrderList extends React.PureComponent {
static propTypes = {
userOrders: PropTypes.array.isRequired,
createOrder: PropTypes.func.isRequired,
}
addOrder() {
this.props.createOrder({...})
}
render() {
return (
<Wrapper>
<Button onClick={this.addOrder}>Add Order</Button>
<List>{this.props.userOrders.map(order => <Item>{order.name}</Item>)}</List>
</Wrapper>
)
}
}
const mapStateToProps = state => ({
userOrders: state.get('userOrders'),
})
const mapDispatchToProps = {
createOrder: () => ({ type: 'CREATE_ORDER', payload: {} }),
}
export default connect(mapStateToProps, mapDispatchToProps)(OrderList)
reducer
case 'CREATE_USER_ORDER_SUCCEEDED':
return state.update('userOrders',
orders => orders.concat([payload.newUserOrder])
)
If you really do need side-effects, then add those side-effects to your saga, or create a new saga that takes the SUCCESS action.
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger arender()
... So how to handle this with dispatch/selectors:this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
|
show 1 more comment
This feels like an XY Problem. You shouldn't be "waiting" inside a component's lifecycle function / event handler at any point, but rather make use of the current state of the store.
If I understand correctly, this is your current flow:
You dispatch an action
CREATE_USER_ORDER
in your React component. This action is consumed by yourcallCreateUserOrder
saga. When your create order saga is complete, it dispatches another "completed" action, which you already have asCREATE_USER_ORDER_SUCCEEDED
.
What you should now add is the proper reducer/selector to handle your CREATE_USER_ORDER_SUCCEEDED
:
This CREATE_USER_ORDER_SUCCEEDED
action should be handled by your reducer to create a new state where some "orders" property in your state is populated. This can be connected directly to your component via a selector, at which point your component will be re-rendered and this.props.userOrders
is populated.
Example:
component
class OrderList extends React.PureComponent {
static propTypes = {
userOrders: PropTypes.array.isRequired,
createOrder: PropTypes.func.isRequired,
}
addOrder() {
this.props.createOrder({...})
}
render() {
return (
<Wrapper>
<Button onClick={this.addOrder}>Add Order</Button>
<List>{this.props.userOrders.map(order => <Item>{order.name}</Item>)}</List>
</Wrapper>
)
}
}
const mapStateToProps = state => ({
userOrders: state.get('userOrders'),
})
const mapDispatchToProps = {
createOrder: () => ({ type: 'CREATE_ORDER', payload: {} }),
}
export default connect(mapStateToProps, mapDispatchToProps)(OrderList)
reducer
case 'CREATE_USER_ORDER_SUCCEEDED':
return state.update('userOrders',
orders => orders.concat([payload.newUserOrder])
)
If you really do need side-effects, then add those side-effects to your saga, or create a new saga that takes the SUCCESS action.
This feels like an XY Problem. You shouldn't be "waiting" inside a component's lifecycle function / event handler at any point, but rather make use of the current state of the store.
If I understand correctly, this is your current flow:
You dispatch an action
CREATE_USER_ORDER
in your React component. This action is consumed by yourcallCreateUserOrder
saga. When your create order saga is complete, it dispatches another "completed" action, which you already have asCREATE_USER_ORDER_SUCCEEDED
.
What you should now add is the proper reducer/selector to handle your CREATE_USER_ORDER_SUCCEEDED
:
This CREATE_USER_ORDER_SUCCEEDED
action should be handled by your reducer to create a new state where some "orders" property in your state is populated. This can be connected directly to your component via a selector, at which point your component will be re-rendered and this.props.userOrders
is populated.
Example:
component
class OrderList extends React.PureComponent {
static propTypes = {
userOrders: PropTypes.array.isRequired,
createOrder: PropTypes.func.isRequired,
}
addOrder() {
this.props.createOrder({...})
}
render() {
return (
<Wrapper>
<Button onClick={this.addOrder}>Add Order</Button>
<List>{this.props.userOrders.map(order => <Item>{order.name}</Item>)}</List>
</Wrapper>
)
}
}
const mapStateToProps = state => ({
userOrders: state.get('userOrders'),
})
const mapDispatchToProps = {
createOrder: () => ({ type: 'CREATE_ORDER', payload: {} }),
}
export default connect(mapStateToProps, mapDispatchToProps)(OrderList)
reducer
case 'CREATE_USER_ORDER_SUCCEEDED':
return state.update('userOrders',
orders => orders.concat([payload.newUserOrder])
)
If you really do need side-effects, then add those side-effects to your saga, or create a new saga that takes the SUCCESS action.
edited Nov 22 '18 at 13:43
answered Nov 22 '18 at 13:29


noahnunoahnu
2,13721233
2,13721233
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger arender()
... So how to handle this with dispatch/selectors:this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
|
show 1 more comment
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger arender()
... So how to handle this with dispatch/selectors:this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Thanks for taking the time to respond. Apologies, I forgot to add my reducer to my question, I've done that now at the bottom of it. Everything you've said I agree with, and I'm actually doing. The problem is that this.props.userOrders doesn't get updated quickly enough, I am using it on the next line of my code, so I need to 'wait' to know when to use it. Hope that makes sense.
– moinhaque
Nov 22 '18 at 14:38
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
Just to clarify, rendering the updated orders isn't the problem, it's doing something with this.props.userOrders straight after it which is the problem. Thanks
– moinhaque
Nov 22 '18 at 14:46
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
@moinhaque can you provide an example of why you want to "wait"? What I'm saying is that you should redesign your approach so that you don't "wait". Use another saga that takes the success action if you need to. Don't dispatch an action and immediately wait for it in the same function within the component.
– noahnu
Nov 22 '18 at 21:42
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
I'm using that dispatch to make a new order, then my next step is to create a payment for that order which the user then pays within the app (hence why I'm doing it in the component). Not sure if adding another Saga will solve it, but I'll look into it.
– moinhaque
Nov 23 '18 at 10:26
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger a
render()
... So how to handle this with dispatch/selectors: this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
All good and well, but imagine that you have for example a POST which should display either validation errors or navigate to another page... Not every result should be something that will trigger a
render()
... So how to handle this with dispatch/selectors: this.api.editObject.then(navigate...).catch(...validationErrors)
– Thomas Stubbe
Feb 20 at 9:33
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431687%2fwait-for-redux-action-to-finish-dispatching-when-using-redux-saga%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PSp8i,R u