Loop JSON object and assign unique JSON item to a given variable
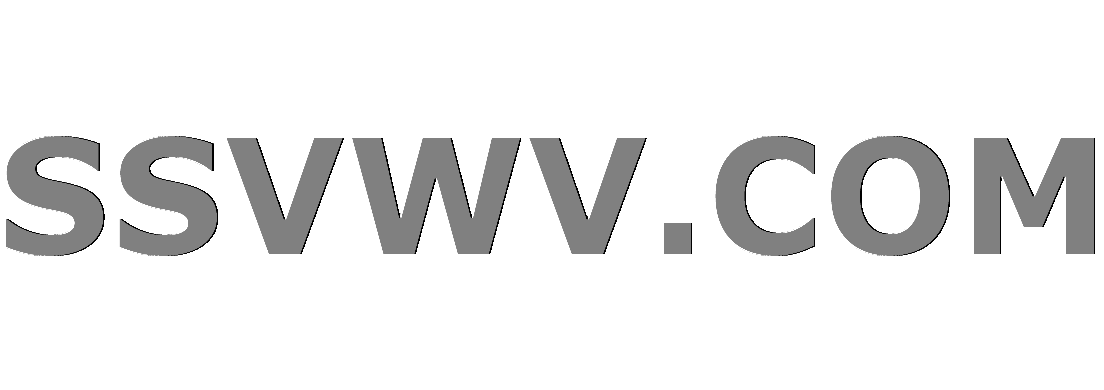
Multi tool use
I'm in the process of building a portfolio, one project I'd like to build is something similar to AirTasker
,
I'm currently working on API
built in node.js
which takes in a JSON
object, this JSON
object is basically tasks that are being requested and need to be completed by the relevant person it is assigned to at present it's automatically assigned.
This is my test dummy data:
[
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
]
now my API
is currently structured as follows:
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
app.post('/api/v1/request', (req, res) => {
var requestData = req.body;
// Loop the tasks passed in
requestData.forEach(request => {
// Now evenly spread tasks across workers
workers.forEach(p => {
workers.tasks.push(request);
})
console.log(request);
});
res.status(200).send({
success: 'true',
message: 'Tasks has been assigned.',
workers: workers
})
});
the object workers
as the people who will be doing the tasks, the issue I have is when I loop requestData
I can't figure out the syntax to assign one task per one worker, at the moment how my code is it'll assign the one task to all workers and continue, the end result will be all workers have all the same tasks.
Can someone assist me with how I go about assigning one unique task per each worker
javascript node.js
add a comment |
I'm in the process of building a portfolio, one project I'd like to build is something similar to AirTasker
,
I'm currently working on API
built in node.js
which takes in a JSON
object, this JSON
object is basically tasks that are being requested and need to be completed by the relevant person it is assigned to at present it's automatically assigned.
This is my test dummy data:
[
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
]
now my API
is currently structured as follows:
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
app.post('/api/v1/request', (req, res) => {
var requestData = req.body;
// Loop the tasks passed in
requestData.forEach(request => {
// Now evenly spread tasks across workers
workers.forEach(p => {
workers.tasks.push(request);
})
console.log(request);
});
res.status(200).send({
success: 'true',
message: 'Tasks has been assigned.',
workers: workers
})
});
the object workers
as the people who will be doing the tasks, the issue I have is when I loop requestData
I can't figure out the syntax to assign one task per one worker, at the moment how my code is it'll assign the one task to all workers and continue, the end result will be all workers have all the same tasks.
Can someone assist me with how I go about assigning one unique task per each worker
javascript node.js
add a comment |
I'm in the process of building a portfolio, one project I'd like to build is something similar to AirTasker
,
I'm currently working on API
built in node.js
which takes in a JSON
object, this JSON
object is basically tasks that are being requested and need to be completed by the relevant person it is assigned to at present it's automatically assigned.
This is my test dummy data:
[
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
]
now my API
is currently structured as follows:
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
app.post('/api/v1/request', (req, res) => {
var requestData = req.body;
// Loop the tasks passed in
requestData.forEach(request => {
// Now evenly spread tasks across workers
workers.forEach(p => {
workers.tasks.push(request);
})
console.log(request);
});
res.status(200).send({
success: 'true',
message: 'Tasks has been assigned.',
workers: workers
})
});
the object workers
as the people who will be doing the tasks, the issue I have is when I loop requestData
I can't figure out the syntax to assign one task per one worker, at the moment how my code is it'll assign the one task to all workers and continue, the end result will be all workers have all the same tasks.
Can someone assist me with how I go about assigning one unique task per each worker
javascript node.js
I'm in the process of building a portfolio, one project I'd like to build is something similar to AirTasker
,
I'm currently working on API
built in node.js
which takes in a JSON
object, this JSON
object is basically tasks that are being requested and need to be completed by the relevant person it is assigned to at present it's automatically assigned.
This is my test dummy data:
[
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
]
now my API
is currently structured as follows:
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
app.post('/api/v1/request', (req, res) => {
var requestData = req.body;
// Loop the tasks passed in
requestData.forEach(request => {
// Now evenly spread tasks across workers
workers.forEach(p => {
workers.tasks.push(request);
})
console.log(request);
});
res.status(200).send({
success: 'true',
message: 'Tasks has been assigned.',
workers: workers
})
});
the object workers
as the people who will be doing the tasks, the issue I have is when I loop requestData
I can't figure out the syntax to assign one task per one worker, at the moment how my code is it'll assign the one task to all workers and continue, the end result will be all workers have all the same tasks.
Can someone assist me with how I go about assigning one unique task per each worker
javascript node.js
javascript node.js
edited Nov 21 '18 at 10:27
Code Ratchet
asked Nov 21 '18 at 10:18


Code RatchetCode Ratchet
2,299105098
2,299105098
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
Try this simple code.
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
add a comment |
You should add task to single worker object in foreach loop like this:
workers.forEach(butler => {
butler.tasks.push(request);
})
add a comment |
You can use the modulus function to help.
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
Here's a demo:
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53409833%2floop-json-object-and-assign-unique-json-item-to-a-given-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
Try this simple code.
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
add a comment |
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
Try this simple code.
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
add a comment |
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
Try this simple code.
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
Try this simple code.
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
const workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
const dummies = [
{
"clientId": 1,
"requestId": "help move bed",
"hours": 6
},
{
"clientId": 2,
"requestId": "pickup parcel",
"hours": 1
},
{
"clientId": 1,
"requestId": "cut the grass",
"hours": 4
},
{
"clientId": 1,
"requestId": "clean the kitchen",
"hours": 2
}
];
workers.forEach(w => {
w.tasks = dummies.filter(d => d.clientId === w.id);
});
console.log(workers);
answered Nov 21 '18 at 10:22


Joven28Joven28
42911
42911
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
add a comment |
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
works like a charm thank you. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 10:29
add a comment |
You should add task to single worker object in foreach loop like this:
workers.forEach(butler => {
butler.tasks.push(request);
})
add a comment |
You should add task to single worker object in foreach loop like this:
workers.forEach(butler => {
butler.tasks.push(request);
})
add a comment |
You should add task to single worker object in foreach loop like this:
workers.forEach(butler => {
butler.tasks.push(request);
})
You should add task to single worker object in foreach loop like this:
workers.forEach(butler => {
butler.tasks.push(request);
})
answered Nov 21 '18 at 10:23
Bartłomiej RasztabigaBartłomiej Rasztabiga
637
637
add a comment |
add a comment |
You can use the modulus function to help.
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
Here's a demo:
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
add a comment |
You can use the modulus function to help.
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
Here's a demo:
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
add a comment |
You can use the modulus function to help.
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
Here's a demo:
You can use the modulus function to help.
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
Here's a demo:
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
var workers = [
{"id": 1, "name": "Person 1", "tasks": },
{"id": 2, "name": "Person 2", "tasks": },
{"id": 3, "name": "Person 3", "tasks": },
{"id": 4, "name": "Person 4", "tasks": },
{"id": 5, "name": "Person 5", "tasks": },
{"id": 6, "name": "Person 6", "tasks": },
]
var tasks = [
{
name: 'a',
hours: 8
},
{
name: 'b',
hours: 4
},
{
name: 'c',
hours: 2
},{
name: 'd',
hours: 4
},
{
name: 'e',
hours: 3
},
{
name: 'f',
hours: 5
},
{
name: 'g',
hours: 4
},
{
name: 'a',
hours: 2
},
]
var reducer = (acc, val) => acc + val.hours;
var length = workers.length;
var start = 0;
tasks.forEach(task => {
if (workers[start%length].tasks.reduce(reducer, 0) < 8) {
workers[start%length].tasks.push(task);
}
start += 1;
});
console.log(workers)
edited Nov 21 '18 at 11:57
answered Nov 21 '18 at 10:28


JackJack
1,15842847
1,15842847
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
add a comment |
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
Looks good Jack, much appreciated. Can I restrict adding a new task to a worker when they have a maximum of 8 hours worth of tasks?
– Code Ratchet
Nov 21 '18 at 11:00
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
I've updated the code to use a reducer to check. However be careful of an infinite loop occurring if you provide more tasks that your works can handle.
– Jack
Nov 21 '18 at 11:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53409833%2floop-json-object-and-assign-unique-json-item-to-a-given-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nP2FvTks6Ga8 l3sRsc8MPIRDl,ZQVC4HFAGcC7qbw1ERtsZI Sk8CkPv k2Mo E,lO10W8dk9a,I7,lUtjsDL p9B