Troubles Accessing Context In ASP.NET Controller
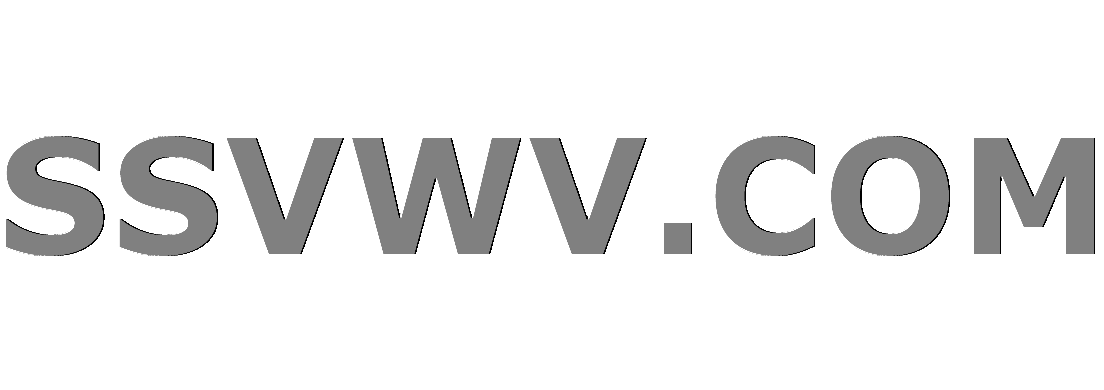
Multi tool use
I'm building a web app using ASP.NET MVC. I also intend to access a remote MySQL database. Would like to keep Entity Framework.
What I am trying to do is access the context within my controller. This worked without an issue using the vanilla database solution. However, now that I am accessing a remote database, it doesn't seem to be working properly. The connection to the database is valid—it actually migrates and seeds properly. But, when I try accessing a member of the context, the app fails.
Web.config
<connectionStrings>
<remove name="DBName" />
<add name="DBName" connectionString="server=1.2.3.4;port=1234;user id=DBAdmin;password=DBPass;database=DBName;integrated security=True" providerName="MySQL.Data.MySqlClient" />
</connectionStrings>
<system.data>
<DbProviderFactories>
<remove invariant="MySql.Data.MySqlClient" />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
<entityFramework>
<defaultConnectionFactory type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6">
<parameters>
<parameter value="v11.0" />
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
</providers>
</entityFramework>
Context.cs
[DbConfigurationType (typeof (MySql.Data.Entity.MySqlEFConfiguration))]
public class Context : DbContext {
public Context () : base ("name=DBName") {
Database.SetInitializer (new MigrateDatabaseToLatestVersion <Context, System.Data.Entity.Migrations.DbMigrationsConfiguration <Context>> ());
}
public DbSet<Software> Softwares { get; set; }
}
SoftwareController
public class SoftwareController : Controller {
public ActionResult Index() {
using (Context context = new Context ()) {
List<Software> softwares = context.Softwares.ToList ();
return View (softwares);
}
}
}
Error
The given key was not present in the dictionary.
Source Error
List softwares = context.Softwares.ToList ();
Stack Trace
[KeyNotFoundException: The given key was not present in the dictionary.]
System.Collections.Generic.Dictionary`2.get_Item(TKey key) +12769097
MySql.Data.MySqlClient.<>c.<.cctor>b__2_7(MySqlConnectionStringBuilder msb, MySqlConnectionStringOption sender) +16
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.get_Item(String keyword) +28
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.GetConnectionString(Boolean includePass) +298
MySql.Data.MySqlClient.MySqlConnection.get_ConnectionString() +41
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.b__12(DbConnection t, DbConnectionInterceptionContext`1 c) +10
System.Data.Entity.Infrastructure.Interception.InternalDispatcher`1.Dispatch(TTarget target, Func`3 operation, TInterceptionContext interceptionContext, Action`3 executing, Action`3 executed) +72
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.GetConnectionString(DbConnection connection, DbInterceptionContext interceptionContext) +361
System.Data.Entity.Internal.InternalConnection.GetStoreConnectionString(DbConnection connection) +159
System.Data.Entity.Internal.InternalConnection.OnConnectionInitialized() +28
System.Data.Entity.Internal.EagerInternalConnection..ctor(DbContext context, DbConnection existingConnection, Boolean connectionOwned) +70
System.Data.Entity.DbContext..ctor(DbConnection existingConnection, Boolean contextOwnsConnection) +55
System.Data.Entity.Migrations.History.HistoryContext..ctor(DbConnection existingConnection, String defaultSchema) +16
MySql.Data.Entity.<>c.<.ctor>b__0_1(DbConnection existingConnection, String defaultSchema) +29
System.Data.Entity.Migrations.History.HistoryRepository.CreateContext(DbConnection connection, String schema) +37
System.Data.Entity.Migrations.History.d__16.MoveNext() +737
System.Linq.Enumerable.Any(IEnumerable`1 source) +77
System.Data.Entity.Migrations.DbMigrator.UpdateInternal(String targetMigration) +58
System.Data.Entity.Migrations.<>c__DisplayClasse.b__d() +13
System.Data.Entity.Migrations.DbMigrator.EnsureDatabaseExists(Action mustSucceedToKeepDatabase) +422
System.Data.Entity.Migrations.DbMigrator.Update(String targetMigration) +78
System.Data.Entity.MigrateDatabaseToLatestVersion`2.InitializeDatabase(TContext context) +108
System.Data.Entity.Internal.<>c__DisplayClassf`1.b__e() +76
System.Data.Entity.Internal.InternalContext.PerformInitializationAction(Action action) +60
System.Data.Entity.Internal.InternalContext.PerformDatabaseInitialization() +357
System.Data.Entity.Internal.LazyInternalContext.b__4(InternalContext c) +7
System.Data.Entity.Internal.RetryAction`1.PerformAction(TInput input) +110
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabaseAction(Action`1 action) +198
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabase() +73
System.Data.Entity.Internal.InternalContext.Initialize() +30
System.Data.Entity.Internal.InternalContext.GetEntitySetAndBaseTypeForType(Type entityType) +16
System.Data.Entity.Internal.Linq.InternalSet`1.Initialize() +53
System.Data.Entity.Internal.Linq.InternalSet`1.GetEnumerator() +15
System.Data.Entity.Infrastructure.DbQuery`1.System.Collections.Generic.IEnumerable.GetEnumerator() +53
System.Collections.Generic.List`1..ctor(IEnumerable`1 collection) +375
System.Linq.Enumerable.ToList(IEnumerable`1 source) +54
ProtoSim.Controllers.SoftwareController.Index() in D:Visual StudioProjectsWebsite ProjectsProtoSimProtoSimControllersSoftwareController.cs:13
lambda_method(Closure , ControllerBase , Object ) +61
System.Web.Mvc.ActionMethodDispatcher.Execute(ControllerBase controller, Object parameters) +14
System.Web.Mvc.ReflectedActionDescriptor.Execute(ControllerContext controllerContext, IDictionary`2 parameters) +157
System.Web.Mvc.ControllerActionInvoker.InvokeActionMethod(ControllerContext controllerContext, ActionDescriptor actionDescriptor, IDictionary`2 parameters) +27
System.Web.Mvc.Async.<>c.b__9_0(IAsyncResult asyncResult, ActionInvocation innerInvokeState) +22
System.Web.Mvc.Async.WrappedAsyncResult`2.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethod(IAsyncResult asyncResult) +32
System.Web.Mvc.Async.AsyncInvocationWithFilters.b__11_0() +50
System.Web.Mvc.Async.<>c__DisplayClass11_1.b__2() +228
System.Web.Mvc.Async.<>c__DisplayClass7_0.b__1(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethodWithFilters(IAsyncResult asyncResult) +34
System.Web.Mvc.Async.<>c__DisplayClass3_6.b__3() +35
System.Web.Mvc.Async.<>c__DisplayClass3_1.b__5(IAsyncResult asyncResult) +100
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeAction(IAsyncResult asyncResult) +27
System.Web.Mvc.<>c.b__152_1(IAsyncResult asyncResult, ExecuteCoreState innerState) +11
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecuteCore(IAsyncResult asyncResult) +45
System.Web.Mvc.<>c.b__151_2(IAsyncResult asyncResult, Controller controller) +13
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +22
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecute(IAsyncResult asyncResult) +26
System.Web.Mvc.Controller.System.Web.Mvc.Async.IAsyncController.EndExecute(IAsyncResult asyncResult) +10
System.Web.Mvc.<>c.b__20_1(IAsyncResult asyncResult, ProcessRequestState innerState) +28
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.MvcHandler.EndProcessRequest(IAsyncResult asyncResult) +28
System.Web.Mvc.MvcHandler.System.Web.IHttpAsyncHandler.EndProcessRequest(IAsyncResult result) +9
System.Web.CallHandlerExecutionStep.System.Web.HttpApplication.IExecutionStep.Execute() +576
System.Web.HttpApplication.ExecuteStepImpl(IExecutionStep step) +132
System.Web.HttpApplication.ExecuteStep(IExecutionStep step, Boolean& completedSynchronously) +163
c# asp.net asp.net-mvc entity-framework
|
show 3 more comments
I'm building a web app using ASP.NET MVC. I also intend to access a remote MySQL database. Would like to keep Entity Framework.
What I am trying to do is access the context within my controller. This worked without an issue using the vanilla database solution. However, now that I am accessing a remote database, it doesn't seem to be working properly. The connection to the database is valid—it actually migrates and seeds properly. But, when I try accessing a member of the context, the app fails.
Web.config
<connectionStrings>
<remove name="DBName" />
<add name="DBName" connectionString="server=1.2.3.4;port=1234;user id=DBAdmin;password=DBPass;database=DBName;integrated security=True" providerName="MySQL.Data.MySqlClient" />
</connectionStrings>
<system.data>
<DbProviderFactories>
<remove invariant="MySql.Data.MySqlClient" />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
<entityFramework>
<defaultConnectionFactory type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6">
<parameters>
<parameter value="v11.0" />
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
</providers>
</entityFramework>
Context.cs
[DbConfigurationType (typeof (MySql.Data.Entity.MySqlEFConfiguration))]
public class Context : DbContext {
public Context () : base ("name=DBName") {
Database.SetInitializer (new MigrateDatabaseToLatestVersion <Context, System.Data.Entity.Migrations.DbMigrationsConfiguration <Context>> ());
}
public DbSet<Software> Softwares { get; set; }
}
SoftwareController
public class SoftwareController : Controller {
public ActionResult Index() {
using (Context context = new Context ()) {
List<Software> softwares = context.Softwares.ToList ();
return View (softwares);
}
}
}
Error
The given key was not present in the dictionary.
Source Error
List softwares = context.Softwares.ToList ();
Stack Trace
[KeyNotFoundException: The given key was not present in the dictionary.]
System.Collections.Generic.Dictionary`2.get_Item(TKey key) +12769097
MySql.Data.MySqlClient.<>c.<.cctor>b__2_7(MySqlConnectionStringBuilder msb, MySqlConnectionStringOption sender) +16
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.get_Item(String keyword) +28
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.GetConnectionString(Boolean includePass) +298
MySql.Data.MySqlClient.MySqlConnection.get_ConnectionString() +41
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.b__12(DbConnection t, DbConnectionInterceptionContext`1 c) +10
System.Data.Entity.Infrastructure.Interception.InternalDispatcher`1.Dispatch(TTarget target, Func`3 operation, TInterceptionContext interceptionContext, Action`3 executing, Action`3 executed) +72
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.GetConnectionString(DbConnection connection, DbInterceptionContext interceptionContext) +361
System.Data.Entity.Internal.InternalConnection.GetStoreConnectionString(DbConnection connection) +159
System.Data.Entity.Internal.InternalConnection.OnConnectionInitialized() +28
System.Data.Entity.Internal.EagerInternalConnection..ctor(DbContext context, DbConnection existingConnection, Boolean connectionOwned) +70
System.Data.Entity.DbContext..ctor(DbConnection existingConnection, Boolean contextOwnsConnection) +55
System.Data.Entity.Migrations.History.HistoryContext..ctor(DbConnection existingConnection, String defaultSchema) +16
MySql.Data.Entity.<>c.<.ctor>b__0_1(DbConnection existingConnection, String defaultSchema) +29
System.Data.Entity.Migrations.History.HistoryRepository.CreateContext(DbConnection connection, String schema) +37
System.Data.Entity.Migrations.History.d__16.MoveNext() +737
System.Linq.Enumerable.Any(IEnumerable`1 source) +77
System.Data.Entity.Migrations.DbMigrator.UpdateInternal(String targetMigration) +58
System.Data.Entity.Migrations.<>c__DisplayClasse.b__d() +13
System.Data.Entity.Migrations.DbMigrator.EnsureDatabaseExists(Action mustSucceedToKeepDatabase) +422
System.Data.Entity.Migrations.DbMigrator.Update(String targetMigration) +78
System.Data.Entity.MigrateDatabaseToLatestVersion`2.InitializeDatabase(TContext context) +108
System.Data.Entity.Internal.<>c__DisplayClassf`1.b__e() +76
System.Data.Entity.Internal.InternalContext.PerformInitializationAction(Action action) +60
System.Data.Entity.Internal.InternalContext.PerformDatabaseInitialization() +357
System.Data.Entity.Internal.LazyInternalContext.b__4(InternalContext c) +7
System.Data.Entity.Internal.RetryAction`1.PerformAction(TInput input) +110
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabaseAction(Action`1 action) +198
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabase() +73
System.Data.Entity.Internal.InternalContext.Initialize() +30
System.Data.Entity.Internal.InternalContext.GetEntitySetAndBaseTypeForType(Type entityType) +16
System.Data.Entity.Internal.Linq.InternalSet`1.Initialize() +53
System.Data.Entity.Internal.Linq.InternalSet`1.GetEnumerator() +15
System.Data.Entity.Infrastructure.DbQuery`1.System.Collections.Generic.IEnumerable.GetEnumerator() +53
System.Collections.Generic.List`1..ctor(IEnumerable`1 collection) +375
System.Linq.Enumerable.ToList(IEnumerable`1 source) +54
ProtoSim.Controllers.SoftwareController.Index() in D:Visual StudioProjectsWebsite ProjectsProtoSimProtoSimControllersSoftwareController.cs:13
lambda_method(Closure , ControllerBase , Object ) +61
System.Web.Mvc.ActionMethodDispatcher.Execute(ControllerBase controller, Object parameters) +14
System.Web.Mvc.ReflectedActionDescriptor.Execute(ControllerContext controllerContext, IDictionary`2 parameters) +157
System.Web.Mvc.ControllerActionInvoker.InvokeActionMethod(ControllerContext controllerContext, ActionDescriptor actionDescriptor, IDictionary`2 parameters) +27
System.Web.Mvc.Async.<>c.b__9_0(IAsyncResult asyncResult, ActionInvocation innerInvokeState) +22
System.Web.Mvc.Async.WrappedAsyncResult`2.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethod(IAsyncResult asyncResult) +32
System.Web.Mvc.Async.AsyncInvocationWithFilters.b__11_0() +50
System.Web.Mvc.Async.<>c__DisplayClass11_1.b__2() +228
System.Web.Mvc.Async.<>c__DisplayClass7_0.b__1(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethodWithFilters(IAsyncResult asyncResult) +34
System.Web.Mvc.Async.<>c__DisplayClass3_6.b__3() +35
System.Web.Mvc.Async.<>c__DisplayClass3_1.b__5(IAsyncResult asyncResult) +100
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeAction(IAsyncResult asyncResult) +27
System.Web.Mvc.<>c.b__152_1(IAsyncResult asyncResult, ExecuteCoreState innerState) +11
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecuteCore(IAsyncResult asyncResult) +45
System.Web.Mvc.<>c.b__151_2(IAsyncResult asyncResult, Controller controller) +13
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +22
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecute(IAsyncResult asyncResult) +26
System.Web.Mvc.Controller.System.Web.Mvc.Async.IAsyncController.EndExecute(IAsyncResult asyncResult) +10
System.Web.Mvc.<>c.b__20_1(IAsyncResult asyncResult, ProcessRequestState innerState) +28
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.MvcHandler.EndProcessRequest(IAsyncResult asyncResult) +28
System.Web.Mvc.MvcHandler.System.Web.IHttpAsyncHandler.EndProcessRequest(IAsyncResult result) +9
System.Web.CallHandlerExecutionStep.System.Web.HttpApplication.IExecutionStep.Execute() +576
System.Web.HttpApplication.ExecuteStepImpl(IExecutionStep step) +132
System.Web.HttpApplication.ExecuteStep(IExecutionStep step, Boolean& completedSynchronously) +163
c# asp.net asp.net-mvc entity-framework
First question...are you able to connect from you computer, to you database server usingmysql
console or via GUI, like heidisql.com
– Hackerman
Nov 21 '18 at 19:45
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Just a hunch...try deletingintegrated security=True
from yourconnectionString
.
– Hackerman
Nov 21 '18 at 20:27
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31
|
show 3 more comments
I'm building a web app using ASP.NET MVC. I also intend to access a remote MySQL database. Would like to keep Entity Framework.
What I am trying to do is access the context within my controller. This worked without an issue using the vanilla database solution. However, now that I am accessing a remote database, it doesn't seem to be working properly. The connection to the database is valid—it actually migrates and seeds properly. But, when I try accessing a member of the context, the app fails.
Web.config
<connectionStrings>
<remove name="DBName" />
<add name="DBName" connectionString="server=1.2.3.4;port=1234;user id=DBAdmin;password=DBPass;database=DBName;integrated security=True" providerName="MySQL.Data.MySqlClient" />
</connectionStrings>
<system.data>
<DbProviderFactories>
<remove invariant="MySql.Data.MySqlClient" />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
<entityFramework>
<defaultConnectionFactory type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6">
<parameters>
<parameter value="v11.0" />
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
</providers>
</entityFramework>
Context.cs
[DbConfigurationType (typeof (MySql.Data.Entity.MySqlEFConfiguration))]
public class Context : DbContext {
public Context () : base ("name=DBName") {
Database.SetInitializer (new MigrateDatabaseToLatestVersion <Context, System.Data.Entity.Migrations.DbMigrationsConfiguration <Context>> ());
}
public DbSet<Software> Softwares { get; set; }
}
SoftwareController
public class SoftwareController : Controller {
public ActionResult Index() {
using (Context context = new Context ()) {
List<Software> softwares = context.Softwares.ToList ();
return View (softwares);
}
}
}
Error
The given key was not present in the dictionary.
Source Error
List softwares = context.Softwares.ToList ();
Stack Trace
[KeyNotFoundException: The given key was not present in the dictionary.]
System.Collections.Generic.Dictionary`2.get_Item(TKey key) +12769097
MySql.Data.MySqlClient.<>c.<.cctor>b__2_7(MySqlConnectionStringBuilder msb, MySqlConnectionStringOption sender) +16
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.get_Item(String keyword) +28
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.GetConnectionString(Boolean includePass) +298
MySql.Data.MySqlClient.MySqlConnection.get_ConnectionString() +41
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.b__12(DbConnection t, DbConnectionInterceptionContext`1 c) +10
System.Data.Entity.Infrastructure.Interception.InternalDispatcher`1.Dispatch(TTarget target, Func`3 operation, TInterceptionContext interceptionContext, Action`3 executing, Action`3 executed) +72
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.GetConnectionString(DbConnection connection, DbInterceptionContext interceptionContext) +361
System.Data.Entity.Internal.InternalConnection.GetStoreConnectionString(DbConnection connection) +159
System.Data.Entity.Internal.InternalConnection.OnConnectionInitialized() +28
System.Data.Entity.Internal.EagerInternalConnection..ctor(DbContext context, DbConnection existingConnection, Boolean connectionOwned) +70
System.Data.Entity.DbContext..ctor(DbConnection existingConnection, Boolean contextOwnsConnection) +55
System.Data.Entity.Migrations.History.HistoryContext..ctor(DbConnection existingConnection, String defaultSchema) +16
MySql.Data.Entity.<>c.<.ctor>b__0_1(DbConnection existingConnection, String defaultSchema) +29
System.Data.Entity.Migrations.History.HistoryRepository.CreateContext(DbConnection connection, String schema) +37
System.Data.Entity.Migrations.History.d__16.MoveNext() +737
System.Linq.Enumerable.Any(IEnumerable`1 source) +77
System.Data.Entity.Migrations.DbMigrator.UpdateInternal(String targetMigration) +58
System.Data.Entity.Migrations.<>c__DisplayClasse.b__d() +13
System.Data.Entity.Migrations.DbMigrator.EnsureDatabaseExists(Action mustSucceedToKeepDatabase) +422
System.Data.Entity.Migrations.DbMigrator.Update(String targetMigration) +78
System.Data.Entity.MigrateDatabaseToLatestVersion`2.InitializeDatabase(TContext context) +108
System.Data.Entity.Internal.<>c__DisplayClassf`1.b__e() +76
System.Data.Entity.Internal.InternalContext.PerformInitializationAction(Action action) +60
System.Data.Entity.Internal.InternalContext.PerformDatabaseInitialization() +357
System.Data.Entity.Internal.LazyInternalContext.b__4(InternalContext c) +7
System.Data.Entity.Internal.RetryAction`1.PerformAction(TInput input) +110
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabaseAction(Action`1 action) +198
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabase() +73
System.Data.Entity.Internal.InternalContext.Initialize() +30
System.Data.Entity.Internal.InternalContext.GetEntitySetAndBaseTypeForType(Type entityType) +16
System.Data.Entity.Internal.Linq.InternalSet`1.Initialize() +53
System.Data.Entity.Internal.Linq.InternalSet`1.GetEnumerator() +15
System.Data.Entity.Infrastructure.DbQuery`1.System.Collections.Generic.IEnumerable.GetEnumerator() +53
System.Collections.Generic.List`1..ctor(IEnumerable`1 collection) +375
System.Linq.Enumerable.ToList(IEnumerable`1 source) +54
ProtoSim.Controllers.SoftwareController.Index() in D:Visual StudioProjectsWebsite ProjectsProtoSimProtoSimControllersSoftwareController.cs:13
lambda_method(Closure , ControllerBase , Object ) +61
System.Web.Mvc.ActionMethodDispatcher.Execute(ControllerBase controller, Object parameters) +14
System.Web.Mvc.ReflectedActionDescriptor.Execute(ControllerContext controllerContext, IDictionary`2 parameters) +157
System.Web.Mvc.ControllerActionInvoker.InvokeActionMethod(ControllerContext controllerContext, ActionDescriptor actionDescriptor, IDictionary`2 parameters) +27
System.Web.Mvc.Async.<>c.b__9_0(IAsyncResult asyncResult, ActionInvocation innerInvokeState) +22
System.Web.Mvc.Async.WrappedAsyncResult`2.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethod(IAsyncResult asyncResult) +32
System.Web.Mvc.Async.AsyncInvocationWithFilters.b__11_0() +50
System.Web.Mvc.Async.<>c__DisplayClass11_1.b__2() +228
System.Web.Mvc.Async.<>c__DisplayClass7_0.b__1(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethodWithFilters(IAsyncResult asyncResult) +34
System.Web.Mvc.Async.<>c__DisplayClass3_6.b__3() +35
System.Web.Mvc.Async.<>c__DisplayClass3_1.b__5(IAsyncResult asyncResult) +100
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeAction(IAsyncResult asyncResult) +27
System.Web.Mvc.<>c.b__152_1(IAsyncResult asyncResult, ExecuteCoreState innerState) +11
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecuteCore(IAsyncResult asyncResult) +45
System.Web.Mvc.<>c.b__151_2(IAsyncResult asyncResult, Controller controller) +13
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +22
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecute(IAsyncResult asyncResult) +26
System.Web.Mvc.Controller.System.Web.Mvc.Async.IAsyncController.EndExecute(IAsyncResult asyncResult) +10
System.Web.Mvc.<>c.b__20_1(IAsyncResult asyncResult, ProcessRequestState innerState) +28
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.MvcHandler.EndProcessRequest(IAsyncResult asyncResult) +28
System.Web.Mvc.MvcHandler.System.Web.IHttpAsyncHandler.EndProcessRequest(IAsyncResult result) +9
System.Web.CallHandlerExecutionStep.System.Web.HttpApplication.IExecutionStep.Execute() +576
System.Web.HttpApplication.ExecuteStepImpl(IExecutionStep step) +132
System.Web.HttpApplication.ExecuteStep(IExecutionStep step, Boolean& completedSynchronously) +163
c# asp.net asp.net-mvc entity-framework
I'm building a web app using ASP.NET MVC. I also intend to access a remote MySQL database. Would like to keep Entity Framework.
What I am trying to do is access the context within my controller. This worked without an issue using the vanilla database solution. However, now that I am accessing a remote database, it doesn't seem to be working properly. The connection to the database is valid—it actually migrates and seeds properly. But, when I try accessing a member of the context, the app fails.
Web.config
<connectionStrings>
<remove name="DBName" />
<add name="DBName" connectionString="server=1.2.3.4;port=1234;user id=DBAdmin;password=DBPass;database=DBName;integrated security=True" providerName="MySQL.Data.MySqlClient" />
</connectionStrings>
<system.data>
<DbProviderFactories>
<remove invariant="MySql.Data.MySqlClient" />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
<entityFramework>
<defaultConnectionFactory type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6">
<parameters>
<parameter value="v11.0" />
</parameters>
</defaultConnectionFactory>
<providers>
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
</providers>
</entityFramework>
Context.cs
[DbConfigurationType (typeof (MySql.Data.Entity.MySqlEFConfiguration))]
public class Context : DbContext {
public Context () : base ("name=DBName") {
Database.SetInitializer (new MigrateDatabaseToLatestVersion <Context, System.Data.Entity.Migrations.DbMigrationsConfiguration <Context>> ());
}
public DbSet<Software> Softwares { get; set; }
}
SoftwareController
public class SoftwareController : Controller {
public ActionResult Index() {
using (Context context = new Context ()) {
List<Software> softwares = context.Softwares.ToList ();
return View (softwares);
}
}
}
Error
The given key was not present in the dictionary.
Source Error
List softwares = context.Softwares.ToList ();
Stack Trace
[KeyNotFoundException: The given key was not present in the dictionary.]
System.Collections.Generic.Dictionary`2.get_Item(TKey key) +12769097
MySql.Data.MySqlClient.<>c.<.cctor>b__2_7(MySqlConnectionStringBuilder msb, MySqlConnectionStringOption sender) +16
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.get_Item(String keyword) +28
MySql.Data.MySqlClient.MySqlConnectionStringBuilder.GetConnectionString(Boolean includePass) +298
MySql.Data.MySqlClient.MySqlConnection.get_ConnectionString() +41
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.b__12(DbConnection t, DbConnectionInterceptionContext`1 c) +10
System.Data.Entity.Infrastructure.Interception.InternalDispatcher`1.Dispatch(TTarget target, Func`3 operation, TInterceptionContext interceptionContext, Action`3 executing, Action`3 executed) +72
System.Data.Entity.Infrastructure.Interception.DbConnectionDispatcher.GetConnectionString(DbConnection connection, DbInterceptionContext interceptionContext) +361
System.Data.Entity.Internal.InternalConnection.GetStoreConnectionString(DbConnection connection) +159
System.Data.Entity.Internal.InternalConnection.OnConnectionInitialized() +28
System.Data.Entity.Internal.EagerInternalConnection..ctor(DbContext context, DbConnection existingConnection, Boolean connectionOwned) +70
System.Data.Entity.DbContext..ctor(DbConnection existingConnection, Boolean contextOwnsConnection) +55
System.Data.Entity.Migrations.History.HistoryContext..ctor(DbConnection existingConnection, String defaultSchema) +16
MySql.Data.Entity.<>c.<.ctor>b__0_1(DbConnection existingConnection, String defaultSchema) +29
System.Data.Entity.Migrations.History.HistoryRepository.CreateContext(DbConnection connection, String schema) +37
System.Data.Entity.Migrations.History.d__16.MoveNext() +737
System.Linq.Enumerable.Any(IEnumerable`1 source) +77
System.Data.Entity.Migrations.DbMigrator.UpdateInternal(String targetMigration) +58
System.Data.Entity.Migrations.<>c__DisplayClasse.b__d() +13
System.Data.Entity.Migrations.DbMigrator.EnsureDatabaseExists(Action mustSucceedToKeepDatabase) +422
System.Data.Entity.Migrations.DbMigrator.Update(String targetMigration) +78
System.Data.Entity.MigrateDatabaseToLatestVersion`2.InitializeDatabase(TContext context) +108
System.Data.Entity.Internal.<>c__DisplayClassf`1.b__e() +76
System.Data.Entity.Internal.InternalContext.PerformInitializationAction(Action action) +60
System.Data.Entity.Internal.InternalContext.PerformDatabaseInitialization() +357
System.Data.Entity.Internal.LazyInternalContext.b__4(InternalContext c) +7
System.Data.Entity.Internal.RetryAction`1.PerformAction(TInput input) +110
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabaseAction(Action`1 action) +198
System.Data.Entity.Internal.LazyInternalContext.InitializeDatabase() +73
System.Data.Entity.Internal.InternalContext.Initialize() +30
System.Data.Entity.Internal.InternalContext.GetEntitySetAndBaseTypeForType(Type entityType) +16
System.Data.Entity.Internal.Linq.InternalSet`1.Initialize() +53
System.Data.Entity.Internal.Linq.InternalSet`1.GetEnumerator() +15
System.Data.Entity.Infrastructure.DbQuery`1.System.Collections.Generic.IEnumerable.GetEnumerator() +53
System.Collections.Generic.List`1..ctor(IEnumerable`1 collection) +375
System.Linq.Enumerable.ToList(IEnumerable`1 source) +54
ProtoSim.Controllers.SoftwareController.Index() in D:Visual StudioProjectsWebsite ProjectsProtoSimProtoSimControllersSoftwareController.cs:13
lambda_method(Closure , ControllerBase , Object ) +61
System.Web.Mvc.ActionMethodDispatcher.Execute(ControllerBase controller, Object parameters) +14
System.Web.Mvc.ReflectedActionDescriptor.Execute(ControllerContext controllerContext, IDictionary`2 parameters) +157
System.Web.Mvc.ControllerActionInvoker.InvokeActionMethod(ControllerContext controllerContext, ActionDescriptor actionDescriptor, IDictionary`2 parameters) +27
System.Web.Mvc.Async.<>c.b__9_0(IAsyncResult asyncResult, ActionInvocation innerInvokeState) +22
System.Web.Mvc.Async.WrappedAsyncResult`2.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethod(IAsyncResult asyncResult) +32
System.Web.Mvc.Async.AsyncInvocationWithFilters.b__11_0() +50
System.Web.Mvc.Async.<>c__DisplayClass11_1.b__2() +228
System.Web.Mvc.Async.<>c__DisplayClass7_0.b__1(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeActionMethodWithFilters(IAsyncResult asyncResult) +34
System.Web.Mvc.Async.<>c__DisplayClass3_6.b__3() +35
System.Web.Mvc.Async.<>c__DisplayClass3_1.b__5(IAsyncResult asyncResult) +100
System.Web.Mvc.Async.WrappedAsyncResult`1.CallEndDelegate(IAsyncResult asyncResult) +10
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Async.AsyncControllerActionInvoker.EndInvokeAction(IAsyncResult asyncResult) +27
System.Web.Mvc.<>c.b__152_1(IAsyncResult asyncResult, ExecuteCoreState innerState) +11
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecuteCore(IAsyncResult asyncResult) +45
System.Web.Mvc.<>c.b__151_2(IAsyncResult asyncResult, Controller controller) +13
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +22
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.Controller.EndExecute(IAsyncResult asyncResult) +26
System.Web.Mvc.Controller.System.Web.Mvc.Async.IAsyncController.EndExecute(IAsyncResult asyncResult) +10
System.Web.Mvc.<>c.b__20_1(IAsyncResult asyncResult, ProcessRequestState innerState) +28
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +29
System.Web.Mvc.Async.WrappedAsyncResultBase`1.End() +49
System.Web.Mvc.MvcHandler.EndProcessRequest(IAsyncResult asyncResult) +28
System.Web.Mvc.MvcHandler.System.Web.IHttpAsyncHandler.EndProcessRequest(IAsyncResult result) +9
System.Web.CallHandlerExecutionStep.System.Web.HttpApplication.IExecutionStep.Execute() +576
System.Web.HttpApplication.ExecuteStepImpl(IExecutionStep step) +132
System.Web.HttpApplication.ExecuteStep(IExecutionStep step, Boolean& completedSynchronously) +163
c# asp.net asp.net-mvc entity-framework
c# asp.net asp.net-mvc entity-framework
asked Nov 21 '18 at 19:41


SebastianSebastian
102
102
First question...are you able to connect from you computer, to you database server usingmysql
console or via GUI, like heidisql.com
– Hackerman
Nov 21 '18 at 19:45
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Just a hunch...try deletingintegrated security=True
from yourconnectionString
.
– Hackerman
Nov 21 '18 at 20:27
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31
|
show 3 more comments
First question...are you able to connect from you computer, to you database server usingmysql
console or via GUI, like heidisql.com
– Hackerman
Nov 21 '18 at 19:45
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Just a hunch...try deletingintegrated security=True
from yourconnectionString
.
– Hackerman
Nov 21 '18 at 20:27
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31
First question...are you able to connect from you computer, to you database server using
mysql
console or via GUI, like heidisql.com– Hackerman
Nov 21 '18 at 19:45
First question...are you able to connect from you computer, to you database server using
mysql
console or via GUI, like heidisql.com– Hackerman
Nov 21 '18 at 19:45
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Just a hunch...try deleting
integrated security=True
from your connectionString
.– Hackerman
Nov 21 '18 at 20:27
Just a hunch...try deleting
integrated security=True
from your connectionString
.– Hackerman
Nov 21 '18 at 20:27
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31
|
show 3 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419445%2ftroubles-accessing-context-in-asp-net-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419445%2ftroubles-accessing-context-in-asp-net-controller%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XRBtOwv4QaE0u s3GP fTRzHQB2HV BBbOHSN16cad2nqnnbBt
First question...are you able to connect from you computer, to you database server using
mysql
console or via GUI, like heidisql.com– Hackerman
Nov 21 '18 at 19:45
Yes, I can open it with Heidisql and can see everything that's there
– Sebastian
Nov 21 '18 at 20:02
Why do you have the remove DBName as well as the add in the connectionStrings section of you web.config?
– Ben Ootjers
Nov 21 '18 at 20:22
Just a hunch...try deleting
integrated security=True
from yourconnectionString
.– Hackerman
Nov 21 '18 at 20:27
@BenOotjers It removes the name just in case it already exists
– Sebastian
Nov 21 '18 at 20:31