How to create outputs in columns using Printf with varied output lengths - Java
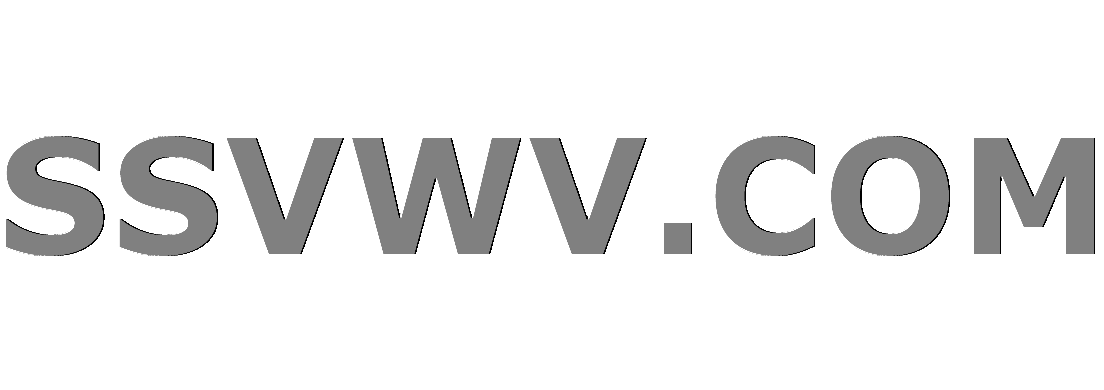
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I was asked to create a code with three methods. This program should be have a method for each of the conversions decimalToBinary, decimalToOctal and decimalToHexadecimal and then display the results in a table with four columns: Decimal, Binary, Octal and Hexadecimal using printf
I was able to create the code to do the conversions however I am having a very hard time figuring out how to print the code into a column. I did research and I know how to use printf however I am confused since the lengths of the outputs will be different every time. How can I get them to display equally? Here is my code.
import java.util.Scanner;
public class Convert {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.println("Please enter your decimal number");
int decimal = input.nextInt();
System.out.printf("Decimal: %dn", decimal);
String binary=decimalToBinary(decimal);
System.out.printf("Binary: %sn", binary);
String octa=decimalToOctal(decimal);
System.out.printf("Octal: %sn", octa);
String hexa=decimalToOctal(decimal);
System.out.printf("Hexa:%sn", hexa);
}
public static String decimalToBinary(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%2;
decimal=decimal/2;
answer=remainder+answer;
}
return answer;
}
public static String decimalToOctal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%8;
decimal=decimal/8;
answer=remainder+answer;
}
return answer;
}
public static String decimalToHexadecimal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%16;
decimal=decimal/16;
if (remainder==10)
answer= "A" + answer;
else if (remainder==11)
answer= "B" + answer;
else if (remainder==12)
answer= "C" + answer;
else if (remainder==13)
answer= "D" + answer;
else if (remainder==14)
answer= "E" + answer;
else if (remainder==15)
answer= "F" + answer;
else answer=remainder+answer;
}
return answer;
}
}
java string eclipse printf
add a comment |
I was asked to create a code with three methods. This program should be have a method for each of the conversions decimalToBinary, decimalToOctal and decimalToHexadecimal and then display the results in a table with four columns: Decimal, Binary, Octal and Hexadecimal using printf
I was able to create the code to do the conversions however I am having a very hard time figuring out how to print the code into a column. I did research and I know how to use printf however I am confused since the lengths of the outputs will be different every time. How can I get them to display equally? Here is my code.
import java.util.Scanner;
public class Convert {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.println("Please enter your decimal number");
int decimal = input.nextInt();
System.out.printf("Decimal: %dn", decimal);
String binary=decimalToBinary(decimal);
System.out.printf("Binary: %sn", binary);
String octa=decimalToOctal(decimal);
System.out.printf("Octal: %sn", octa);
String hexa=decimalToOctal(decimal);
System.out.printf("Hexa:%sn", hexa);
}
public static String decimalToBinary(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%2;
decimal=decimal/2;
answer=remainder+answer;
}
return answer;
}
public static String decimalToOctal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%8;
decimal=decimal/8;
answer=remainder+answer;
}
return answer;
}
public static String decimalToHexadecimal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%16;
decimal=decimal/16;
if (remainder==10)
answer= "A" + answer;
else if (remainder==11)
answer= "B" + answer;
else if (remainder==12)
answer= "C" + answer;
else if (remainder==13)
answer= "D" + answer;
else if (remainder==14)
answer= "E" + answer;
else if (remainder==15)
answer= "F" + answer;
else answer=remainder+answer;
}
return answer;
}
}
java string eclipse printf
add a comment |
I was asked to create a code with three methods. This program should be have a method for each of the conversions decimalToBinary, decimalToOctal and decimalToHexadecimal and then display the results in a table with four columns: Decimal, Binary, Octal and Hexadecimal using printf
I was able to create the code to do the conversions however I am having a very hard time figuring out how to print the code into a column. I did research and I know how to use printf however I am confused since the lengths of the outputs will be different every time. How can I get them to display equally? Here is my code.
import java.util.Scanner;
public class Convert {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.println("Please enter your decimal number");
int decimal = input.nextInt();
System.out.printf("Decimal: %dn", decimal);
String binary=decimalToBinary(decimal);
System.out.printf("Binary: %sn", binary);
String octa=decimalToOctal(decimal);
System.out.printf("Octal: %sn", octa);
String hexa=decimalToOctal(decimal);
System.out.printf("Hexa:%sn", hexa);
}
public static String decimalToBinary(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%2;
decimal=decimal/2;
answer=remainder+answer;
}
return answer;
}
public static String decimalToOctal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%8;
decimal=decimal/8;
answer=remainder+answer;
}
return answer;
}
public static String decimalToHexadecimal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%16;
decimal=decimal/16;
if (remainder==10)
answer= "A" + answer;
else if (remainder==11)
answer= "B" + answer;
else if (remainder==12)
answer= "C" + answer;
else if (remainder==13)
answer= "D" + answer;
else if (remainder==14)
answer= "E" + answer;
else if (remainder==15)
answer= "F" + answer;
else answer=remainder+answer;
}
return answer;
}
}
java string eclipse printf
I was asked to create a code with three methods. This program should be have a method for each of the conversions decimalToBinary, decimalToOctal and decimalToHexadecimal and then display the results in a table with four columns: Decimal, Binary, Octal and Hexadecimal using printf
I was able to create the code to do the conversions however I am having a very hard time figuring out how to print the code into a column. I did research and I know how to use printf however I am confused since the lengths of the outputs will be different every time. How can I get them to display equally? Here is my code.
import java.util.Scanner;
public class Convert {
public static void main(String args) {
Scanner input = new Scanner(System.in);
System.out.println("Please enter your decimal number");
int decimal = input.nextInt();
System.out.printf("Decimal: %dn", decimal);
String binary=decimalToBinary(decimal);
System.out.printf("Binary: %sn", binary);
String octa=decimalToOctal(decimal);
System.out.printf("Octal: %sn", octa);
String hexa=decimalToOctal(decimal);
System.out.printf("Hexa:%sn", hexa);
}
public static String decimalToBinary(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%2;
decimal=decimal/2;
answer=remainder+answer;
}
return answer;
}
public static String decimalToOctal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%8;
decimal=decimal/8;
answer=remainder+answer;
}
return answer;
}
public static String decimalToHexadecimal(int decimal) {
int remainder;
String answer="";
while (decimal>0) {
remainder=decimal%16;
decimal=decimal/16;
if (remainder==10)
answer= "A" + answer;
else if (remainder==11)
answer= "B" + answer;
else if (remainder==12)
answer= "C" + answer;
else if (remainder==13)
answer= "D" + answer;
else if (remainder==14)
answer= "E" + answer;
else if (remainder==15)
answer= "F" + answer;
else answer=remainder+answer;
}
return answer;
}
}
java string eclipse printf
java string eclipse printf
asked Nov 24 '18 at 0:09
Rebecca Allyson MorrisRebecca Allyson Morris
203
203
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Take a look at the printf() documentation, in particular the format string syntax. You are after the width specifiers. For example,
printf("%20s", s);
will ensure your output will have a width of 20 characters. You can play around with the variations to achieve what you want.
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454101%2fhow-to-create-outputs-in-columns-using-printf-with-varied-output-lengths-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Take a look at the printf() documentation, in particular the format string syntax. You are after the width specifiers. For example,
printf("%20s", s);
will ensure your output will have a width of 20 characters. You can play around with the variations to achieve what you want.
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
add a comment |
Take a look at the printf() documentation, in particular the format string syntax. You are after the width specifiers. For example,
printf("%20s", s);
will ensure your output will have a width of 20 characters. You can play around with the variations to achieve what you want.
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
add a comment |
Take a look at the printf() documentation, in particular the format string syntax. You are after the width specifiers. For example,
printf("%20s", s);
will ensure your output will have a width of 20 characters. You can play around with the variations to achieve what you want.
Take a look at the printf() documentation, in particular the format string syntax. You are after the width specifiers. For example,
printf("%20s", s);
will ensure your output will have a width of 20 characters. You can play around with the variations to achieve what you want.
answered Nov 24 '18 at 0:18
davedave
8,85353252
8,85353252
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
add a comment |
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
I had tried different lengths, I just left the lengths out when I pasted my code because I realized the problem is even if I put 20 characters, or 15, or any it goes out of line with different inputs as some inputs will create longer outputs.
– Rebecca Allyson Morris
Nov 24 '18 at 0:20
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
For example, this code works if the user input is "30" System.out.println("Please enter your decimal number"); int decimal = input.nextInt(); System.out.printf("Decimal: %20dn", decimal); String binary=decimalToBinary(decimal); System.out.printf("Binary: %21sn", binary); String octa=decimalToOctal(decimal); System.out.printf("Octal: %22sn", octa); String hexa=decimalToOctal(decimal); System.out.printf("Hexa:%24sn", hexa); Any other input its not inline
– Rebecca Allyson Morris
Nov 24 '18 at 0:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454101%2fhow-to-create-outputs-in-columns-using-printf-with-varied-output-lengths-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gEZS 2d1WTUtlGvXF8ehi0AXtmKEVNjpD