asyncpg fetch feedback (python)
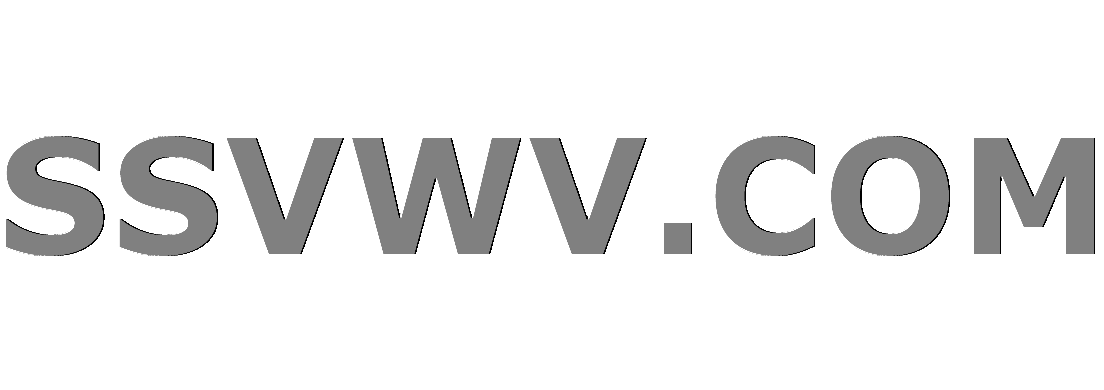
Multi tool use
up vote
2
down vote
favorite
I have been using psycopg2 to manage items in my PostgreSQL database. Recently someone suggested that I could improve my database transactions by using asyncio and asyncpg in my code. I have looked around Stack Overflow and read though the documentation for examples. I have been able to create tables and insert records, but I haven't been able to get the execution feedback that I desire.
For example in my psycopg2 code, I can verify that a table exists or doesn't exist prior to inserting records.
def table_exists(self, verify_table_existence, name):
'''Verifies the existence of a table within the PostgreSQL database'''
try:
self.cursor.execute(verify_table_existence, name)
answer = self.cursor.fetchone()[0]
if answer == True:
print('The table - {} - exists'.format(name))
return True
else:
print ('The table - {} - does NOT exist'.format(name))
return False
except Exception as error:
logger.info('An error has occurred while trying to verify the existence of the table {}'.format(name))
logger.info('Error message: {}').format(error)
sys.exit(1)
I haven't been able to get the same feedback using asyncpg. How do I accomplish this?
import asyncpg
import asyncio
async def main():
conn = await asyncpg.connect('postgresql://postgres:mypassword@localhost:5432/mydatabase')
answer = await conn.fetch('''
SELECT EXISTS (
SELECT 1
FROM pg_tables
WHERE schemaname = 'public'
AND tablename = 'test01'
); ''')
await conn.close()
#####################
# the fetch returns
# [<Record exists=True>]
# but prints 'The table does NOT exist'
#####################
if answer == True:
print('The table exists')
else:
print('The table does NOT exist')
asyncio.get_event_loop().run_until_complete(main())
python-3.x postgresql python-asyncio asyncpg
add a comment |
up vote
2
down vote
favorite
I have been using psycopg2 to manage items in my PostgreSQL database. Recently someone suggested that I could improve my database transactions by using asyncio and asyncpg in my code. I have looked around Stack Overflow and read though the documentation for examples. I have been able to create tables and insert records, but I haven't been able to get the execution feedback that I desire.
For example in my psycopg2 code, I can verify that a table exists or doesn't exist prior to inserting records.
def table_exists(self, verify_table_existence, name):
'''Verifies the existence of a table within the PostgreSQL database'''
try:
self.cursor.execute(verify_table_existence, name)
answer = self.cursor.fetchone()[0]
if answer == True:
print('The table - {} - exists'.format(name))
return True
else:
print ('The table - {} - does NOT exist'.format(name))
return False
except Exception as error:
logger.info('An error has occurred while trying to verify the existence of the table {}'.format(name))
logger.info('Error message: {}').format(error)
sys.exit(1)
I haven't been able to get the same feedback using asyncpg. How do I accomplish this?
import asyncpg
import asyncio
async def main():
conn = await asyncpg.connect('postgresql://postgres:mypassword@localhost:5432/mydatabase')
answer = await conn.fetch('''
SELECT EXISTS (
SELECT 1
FROM pg_tables
WHERE schemaname = 'public'
AND tablename = 'test01'
); ''')
await conn.close()
#####################
# the fetch returns
# [<Record exists=True>]
# but prints 'The table does NOT exist'
#####################
if answer == True:
print('The table exists')
else:
print('The table does NOT exist')
asyncio.get_event_loop().run_until_complete(main())
python-3.x postgresql python-asyncio asyncpg
1
You usedfetchone()[0]
with psycopg2, but justfetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal toTrue
. Try usinganswer = await conn.fetchval(...)
instead.
– user4815162342
Nov 6 at 5:30
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have been using psycopg2 to manage items in my PostgreSQL database. Recently someone suggested that I could improve my database transactions by using asyncio and asyncpg in my code. I have looked around Stack Overflow and read though the documentation for examples. I have been able to create tables and insert records, but I haven't been able to get the execution feedback that I desire.
For example in my psycopg2 code, I can verify that a table exists or doesn't exist prior to inserting records.
def table_exists(self, verify_table_existence, name):
'''Verifies the existence of a table within the PostgreSQL database'''
try:
self.cursor.execute(verify_table_existence, name)
answer = self.cursor.fetchone()[0]
if answer == True:
print('The table - {} - exists'.format(name))
return True
else:
print ('The table - {} - does NOT exist'.format(name))
return False
except Exception as error:
logger.info('An error has occurred while trying to verify the existence of the table {}'.format(name))
logger.info('Error message: {}').format(error)
sys.exit(1)
I haven't been able to get the same feedback using asyncpg. How do I accomplish this?
import asyncpg
import asyncio
async def main():
conn = await asyncpg.connect('postgresql://postgres:mypassword@localhost:5432/mydatabase')
answer = await conn.fetch('''
SELECT EXISTS (
SELECT 1
FROM pg_tables
WHERE schemaname = 'public'
AND tablename = 'test01'
); ''')
await conn.close()
#####################
# the fetch returns
# [<Record exists=True>]
# but prints 'The table does NOT exist'
#####################
if answer == True:
print('The table exists')
else:
print('The table does NOT exist')
asyncio.get_event_loop().run_until_complete(main())
python-3.x postgresql python-asyncio asyncpg
I have been using psycopg2 to manage items in my PostgreSQL database. Recently someone suggested that I could improve my database transactions by using asyncio and asyncpg in my code. I have looked around Stack Overflow and read though the documentation for examples. I have been able to create tables and insert records, but I haven't been able to get the execution feedback that I desire.
For example in my psycopg2 code, I can verify that a table exists or doesn't exist prior to inserting records.
def table_exists(self, verify_table_existence, name):
'''Verifies the existence of a table within the PostgreSQL database'''
try:
self.cursor.execute(verify_table_existence, name)
answer = self.cursor.fetchone()[0]
if answer == True:
print('The table - {} - exists'.format(name))
return True
else:
print ('The table - {} - does NOT exist'.format(name))
return False
except Exception as error:
logger.info('An error has occurred while trying to verify the existence of the table {}'.format(name))
logger.info('Error message: {}').format(error)
sys.exit(1)
I haven't been able to get the same feedback using asyncpg. How do I accomplish this?
import asyncpg
import asyncio
async def main():
conn = await asyncpg.connect('postgresql://postgres:mypassword@localhost:5432/mydatabase')
answer = await conn.fetch('''
SELECT EXISTS (
SELECT 1
FROM pg_tables
WHERE schemaname = 'public'
AND tablename = 'test01'
); ''')
await conn.close()
#####################
# the fetch returns
# [<Record exists=True>]
# but prints 'The table does NOT exist'
#####################
if answer == True:
print('The table exists')
else:
print('The table does NOT exist')
asyncio.get_event_loop().run_until_complete(main())
python-3.x postgresql python-asyncio asyncpg
python-3.x postgresql python-asyncio asyncpg
edited Nov 5 at 23:20
asked Nov 5 at 17:23
Life is complex
14317
14317
1
You usedfetchone()[0]
with psycopg2, but justfetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal toTrue
. Try usinganswer = await conn.fetchval(...)
instead.
– user4815162342
Nov 6 at 5:30
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48
add a comment |
1
You usedfetchone()[0]
with psycopg2, but justfetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal toTrue
. Try usinganswer = await conn.fetchval(...)
instead.
– user4815162342
Nov 6 at 5:30
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48
1
1
You used
fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
. Try using answer = await conn.fetchval(...)
instead.– user4815162342
Nov 6 at 5:30
You used
fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
. Try using answer = await conn.fetchval(...)
instead.– user4815162342
Nov 6 at 5:30
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You used fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
.
To fetch a single value from a single row, use something like answer = await conn.fetchval(...)
.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You used fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
.
To fetch a single value from a single row, use something like answer = await conn.fetchval(...)
.
add a comment |
up vote
1
down vote
accepted
You used fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
.
To fetch a single value from a single row, use something like answer = await conn.fetchval(...)
.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You used fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
.
To fetch a single value from a single row, use something like answer = await conn.fetchval(...)
.
You used fetchone()[0]
with psycopg2, but just fetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal to True
.
To fetch a single value from a single row, use something like answer = await conn.fetchval(...)
.
answered Nov 6 at 18:47
user4815162342
58.3k487138
58.3k487138
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53159231%2fasyncpg-fetch-feedback-python%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Udp30KQd3 Sj,peNSsfnfnTax,yi u9,syaT
1
You used
fetchone()[0]
with psycopg2, but justfetch(...)
with asyncpg. The former will retrieve the first column of the first row, while the latter will retrieve a whole list of rows. Being a list, it doesn't compare as equal toTrue
. Try usinganswer = await conn.fetchval(...)
instead.– user4815162342
Nov 6 at 5:30
Thanks that worked.
– Life is complex
Nov 6 at 9:54
Glad to hear! I've now reposted it as an answer.
– user4815162342
Nov 6 at 18:48