Downloading an API file to Firebase Cloud Storage?
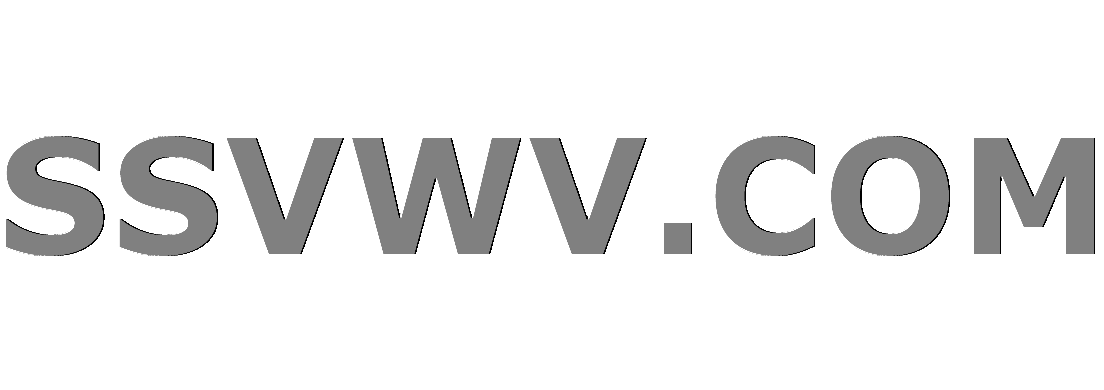
Multi tool use
up vote
0
down vote
favorite
How do I save an audio file (about 10K) from IBM Watson Text-to-speech to Firebase Cloud Storage? Here's my code, copied from the IBM Watson documentation:
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(fs.createWriteStream('hello_world.wav')); // what goes here?
const file = ?????
file.download()
.then(function(data) {
console.log("File downloaded."
})
.catch(error => {
console.error(error);
});
});
The missing code is between
}).pipe(fs.createWriteStream('hello_world.wav'));
and
file.download()
Somehow I have to convert the file provided by IBM Watson into a file that Firebase Cloud Storage recognizes. Is fs
not allowed in Google Cloud Functions?
Also, shouldn't line 6 be
var fs = require('fs-js');
not
var fs = require('fs');
According to NPM the fs
package is deprecated.
Is pipe
allowed in Google Cloud Functions? If so, what do I pipe the file to? I need something that looks like this:
}).pipe(file);
file.download()
node.js google-cloud-functions firebase-storage text-to-speech ibm-watson
|
show 1 more comment
up vote
0
down vote
favorite
How do I save an audio file (about 10K) from IBM Watson Text-to-speech to Firebase Cloud Storage? Here's my code, copied from the IBM Watson documentation:
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(fs.createWriteStream('hello_world.wav')); // what goes here?
const file = ?????
file.download()
.then(function(data) {
console.log("File downloaded."
})
.catch(error => {
console.error(error);
});
});
The missing code is between
}).pipe(fs.createWriteStream('hello_world.wav'));
and
file.download()
Somehow I have to convert the file provided by IBM Watson into a file that Firebase Cloud Storage recognizes. Is fs
not allowed in Google Cloud Functions?
Also, shouldn't line 6 be
var fs = require('fs-js');
not
var fs = require('fs');
According to NPM the fs
package is deprecated.
Is pipe
allowed in Google Cloud Functions? If so, what do I pipe the file to? I need something that looks like this:
}).pipe(file);
file.download()
node.js google-cloud-functions firebase-storage text-to-speech ibm-watson
I can help but I have a question first - what type of variable do you needfile
to be? ABuffer
? I mostly want to know, where is thatdownload()
function coming from - or is that pseudocode?
– dpopp07
Nov 7 at 23:38
You need to return a promise from your function that resolves when all the work is complete.pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.
– Doug Stevenson
Nov 8 at 0:37
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44
|
show 1 more comment
up vote
0
down vote
favorite
up vote
0
down vote
favorite
How do I save an audio file (about 10K) from IBM Watson Text-to-speech to Firebase Cloud Storage? Here's my code, copied from the IBM Watson documentation:
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(fs.createWriteStream('hello_world.wav')); // what goes here?
const file = ?????
file.download()
.then(function(data) {
console.log("File downloaded."
})
.catch(error => {
console.error(error);
});
});
The missing code is between
}).pipe(fs.createWriteStream('hello_world.wav'));
and
file.download()
Somehow I have to convert the file provided by IBM Watson into a file that Firebase Cloud Storage recognizes. Is fs
not allowed in Google Cloud Functions?
Also, shouldn't line 6 be
var fs = require('fs-js');
not
var fs = require('fs');
According to NPM the fs
package is deprecated.
Is pipe
allowed in Google Cloud Functions? If so, what do I pipe the file to? I need something that looks like this:
}).pipe(file);
file.download()
node.js google-cloud-functions firebase-storage text-to-speech ibm-watson
How do I save an audio file (about 10K) from IBM Watson Text-to-speech to Firebase Cloud Storage? Here's my code, copied from the IBM Watson documentation:
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(fs.createWriteStream('hello_world.wav')); // what goes here?
const file = ?????
file.download()
.then(function(data) {
console.log("File downloaded."
})
.catch(error => {
console.error(error);
});
});
The missing code is between
}).pipe(fs.createWriteStream('hello_world.wav'));
and
file.download()
Somehow I have to convert the file provided by IBM Watson into a file that Firebase Cloud Storage recognizes. Is fs
not allowed in Google Cloud Functions?
Also, shouldn't line 6 be
var fs = require('fs-js');
not
var fs = require('fs');
According to NPM the fs
package is deprecated.
Is pipe
allowed in Google Cloud Functions? If so, what do I pipe the file to? I need something that looks like this:
}).pipe(file);
file.download()
node.js google-cloud-functions firebase-storage text-to-speech ibm-watson
node.js google-cloud-functions firebase-storage text-to-speech ibm-watson
edited Nov 9 at 0:07
asked Nov 7 at 22:57


Thomas David Kehoe
1,88211333
1,88211333
I can help but I have a question first - what type of variable do you needfile
to be? ABuffer
? I mostly want to know, where is thatdownload()
function coming from - or is that pseudocode?
– dpopp07
Nov 7 at 23:38
You need to return a promise from your function that resolves when all the work is complete.pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.
– Doug Stevenson
Nov 8 at 0:37
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44
|
show 1 more comment
I can help but I have a question first - what type of variable do you needfile
to be? ABuffer
? I mostly want to know, where is thatdownload()
function coming from - or is that pseudocode?
– dpopp07
Nov 7 at 23:38
You need to return a promise from your function that resolves when all the work is complete.pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.
– Doug Stevenson
Nov 8 at 0:37
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44
I can help but I have a question first - what type of variable do you need
file
to be? A Buffer
? I mostly want to know, where is that download()
function coming from - or is that pseudocode?– dpopp07
Nov 7 at 23:38
I can help but I have a question first - what type of variable do you need
file
to be? A Buffer
? I mostly want to know, where is that download()
function coming from - or is that pseudocode?– dpopp07
Nov 7 at 23:38
You need to return a promise from your function that resolves when all the work is complete.
pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.– Doug Stevenson
Nov 8 at 0:37
You need to return a promise from your function that resolves when all the work is complete.
pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.– Doug Stevenson
Nov 8 at 0:37
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44
|
show 1 more comment
2 Answers
2
active
oldest
votes
up vote
1
down vote
Okay, reviewing the documentation for file.download()
, I think you can make this work with little change to your code. file
needs to be type File
from the Google Storage library (you'll need to install this library). This type has a method called createWriteStream
that you can stream the results of synthesize
to. I didn't test this but I believe it should be correct or should at least point you in the right direction:
// looks like you'll need to require this package also
const { Storage } = require('@google-cloud/storage');
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
const storage = new Storage();
const myBucket = storage.bucket('my-bucket');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
const file = myBucket.file('my-file'); // name your file something here
textToSpeech
.synthesize(synthesizeParams)
.on('error', function(error) {
console.log(error);
})
.pipe(file.createWriteStream()) // the File object has a `createWriteStream` method for writing streams to it
.on('error', function(err) {
console.log(err);
})
.on('finish', function() {
// The file upload is complete.
file.download()
.then(function(data) {
console.log("File downloaded.");
})
.catch(error => {
console.error(error);
});
});
});
For the record:
pipe()
should be allowed in Google Cloud Functions and it is async. This is why you need to listen for thefinish
event before downloading the file
fs
is only deprecated on public NPM but that is not the package you were importing, thefs
(file system) module is one of Node's core built-in packages. That said, it looks like you might not need it in your code at all
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
add a comment |
up vote
1
down vote
accepted
Thanks dpopp07, I got it!
exports.TextToSpeech = functions.firestore.document('Test_Word').onUpdate((change, context) => {
if (change.after.data().word != undefined) {
myWord = change.after.data().word;
myWordFileType = myWord + '.ogg';
var synthesizeParams = {
text: myWord,
accept: 'audio/ogg',
voice: 'en-US_AllisonVoice'
};
const {Storage} = require('@google-cloud/storage');
const storage = new Storage();
const bucket = storage.bucket('myapp.appspot.com');
const file = bucket.file('Test_Folder' + myWordFileType);
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(file.createWriteStream({contentType: 'auto'}))
.on('error', function(err) {})
.on('finish', function() {
console.log("Complete.");
});
}
return 0;
});
The function triggers when a new word is written to a Firestore location, then extracts the word and calls it myWord
. Adding .ogg makes myWordFileType
. The functions sends an HTTP request to IBM Watson Text-to-speech, which returns a callback, not a promise, so the code is a bit ugly. The crux is where the HTTP response goes through Node command pipe
to the send the file to the Google Cloud Storage command file.createWriteStream. The contentType
must be set to get a readable file, but auto
makes this easy. The file is then written to the bucket, which is set to my Firebase Cloud Storage folder Test_Folder
and the filename is myWordFileType
.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Okay, reviewing the documentation for file.download()
, I think you can make this work with little change to your code. file
needs to be type File
from the Google Storage library (you'll need to install this library). This type has a method called createWriteStream
that you can stream the results of synthesize
to. I didn't test this but I believe it should be correct or should at least point you in the right direction:
// looks like you'll need to require this package also
const { Storage } = require('@google-cloud/storage');
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
const storage = new Storage();
const myBucket = storage.bucket('my-bucket');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
const file = myBucket.file('my-file'); // name your file something here
textToSpeech
.synthesize(synthesizeParams)
.on('error', function(error) {
console.log(error);
})
.pipe(file.createWriteStream()) // the File object has a `createWriteStream` method for writing streams to it
.on('error', function(err) {
console.log(err);
})
.on('finish', function() {
// The file upload is complete.
file.download()
.then(function(data) {
console.log("File downloaded.");
})
.catch(error => {
console.error(error);
});
});
});
For the record:
pipe()
should be allowed in Google Cloud Functions and it is async. This is why you need to listen for thefinish
event before downloading the file
fs
is only deprecated on public NPM but that is not the package you were importing, thefs
(file system) module is one of Node's core built-in packages. That said, it looks like you might not need it in your code at all
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
add a comment |
up vote
1
down vote
Okay, reviewing the documentation for file.download()
, I think you can make this work with little change to your code. file
needs to be type File
from the Google Storage library (you'll need to install this library). This type has a method called createWriteStream
that you can stream the results of synthesize
to. I didn't test this but I believe it should be correct or should at least point you in the right direction:
// looks like you'll need to require this package also
const { Storage } = require('@google-cloud/storage');
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
const storage = new Storage();
const myBucket = storage.bucket('my-bucket');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
const file = myBucket.file('my-file'); // name your file something here
textToSpeech
.synthesize(synthesizeParams)
.on('error', function(error) {
console.log(error);
})
.pipe(file.createWriteStream()) // the File object has a `createWriteStream` method for writing streams to it
.on('error', function(err) {
console.log(err);
})
.on('finish', function() {
// The file upload is complete.
file.download()
.then(function(data) {
console.log("File downloaded.");
})
.catch(error => {
console.error(error);
});
});
});
For the record:
pipe()
should be allowed in Google Cloud Functions and it is async. This is why you need to listen for thefinish
event before downloading the file
fs
is only deprecated on public NPM but that is not the package you were importing, thefs
(file system) module is one of Node's core built-in packages. That said, it looks like you might not need it in your code at all
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
add a comment |
up vote
1
down vote
up vote
1
down vote
Okay, reviewing the documentation for file.download()
, I think you can make this work with little change to your code. file
needs to be type File
from the Google Storage library (you'll need to install this library). This type has a method called createWriteStream
that you can stream the results of synthesize
to. I didn't test this but I believe it should be correct or should at least point you in the right direction:
// looks like you'll need to require this package also
const { Storage } = require('@google-cloud/storage');
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
const storage = new Storage();
const myBucket = storage.bucket('my-bucket');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
const file = myBucket.file('my-file'); // name your file something here
textToSpeech
.synthesize(synthesizeParams)
.on('error', function(error) {
console.log(error);
})
.pipe(file.createWriteStream()) // the File object has a `createWriteStream` method for writing streams to it
.on('error', function(err) {
console.log(err);
})
.on('finish', function() {
// The file upload is complete.
file.download()
.then(function(data) {
console.log("File downloaded.");
})
.catch(error => {
console.error(error);
});
});
});
For the record:
pipe()
should be allowed in Google Cloud Functions and it is async. This is why you need to listen for thefinish
event before downloading the file
fs
is only deprecated on public NPM but that is not the package you were importing, thefs
(file system) module is one of Node's core built-in packages. That said, it looks like you might not need it in your code at all
Okay, reviewing the documentation for file.download()
, I think you can make this work with little change to your code. file
needs to be type File
from the Google Storage library (you'll need to install this library). This type has a method called createWriteStream
that you can stream the results of synthesize
to. I didn't test this but I believe it should be correct or should at least point you in the right direction:
// looks like you'll need to require this package also
const { Storage } = require('@google-cloud/storage');
const functions = require('firebase-functions');
const admin = require('firebase-admin');
admin.initializeApp();
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var fs = require('fs');
const storage = new Storage();
const myBucket = storage.bucket('my-bucket');
exports.TextToSpeech = functions.firestore.document('Test_Value').onUpdate((change, context) => {
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
var synthesizeParams = {
text: 'Hello world',
accept: 'audio/wav',
voice: 'en-US_AllisonVoice'
};
const file = myBucket.file('my-file'); // name your file something here
textToSpeech
.synthesize(synthesizeParams)
.on('error', function(error) {
console.log(error);
})
.pipe(file.createWriteStream()) // the File object has a `createWriteStream` method for writing streams to it
.on('error', function(err) {
console.log(err);
})
.on('finish', function() {
// The file upload is complete.
file.download()
.then(function(data) {
console.log("File downloaded.");
})
.catch(error => {
console.error(error);
});
});
});
For the record:
pipe()
should be allowed in Google Cloud Functions and it is async. This is why you need to listen for thefinish
event before downloading the file
fs
is only deprecated on public NPM but that is not the package you were importing, thefs
(file system) module is one of Node's core built-in packages. That said, it looks like you might not need it in your code at all
answered Nov 8 at 22:20


dpopp07
27626
27626
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
add a comment |
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks, I was just reading the documentation and saw file.createWriteStream. Running your code I get an error message "file is not defined" at the file.createWriteStream line. The documentation includes "const file = myBucket.file('my-file');". I'll keep working at it.
– Thomas David Kehoe
Nov 8 at 23:10
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
Thanks again, I've written up the working code as an answer and upvoted your answer.
– Thomas David Kehoe
Nov 9 at 0:22
add a comment |
up vote
1
down vote
accepted
Thanks dpopp07, I got it!
exports.TextToSpeech = functions.firestore.document('Test_Word').onUpdate((change, context) => {
if (change.after.data().word != undefined) {
myWord = change.after.data().word;
myWordFileType = myWord + '.ogg';
var synthesizeParams = {
text: myWord,
accept: 'audio/ogg',
voice: 'en-US_AllisonVoice'
};
const {Storage} = require('@google-cloud/storage');
const storage = new Storage();
const bucket = storage.bucket('myapp.appspot.com');
const file = bucket.file('Test_Folder' + myWordFileType);
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(file.createWriteStream({contentType: 'auto'}))
.on('error', function(err) {})
.on('finish', function() {
console.log("Complete.");
});
}
return 0;
});
The function triggers when a new word is written to a Firestore location, then extracts the word and calls it myWord
. Adding .ogg makes myWordFileType
. The functions sends an HTTP request to IBM Watson Text-to-speech, which returns a callback, not a promise, so the code is a bit ugly. The crux is where the HTTP response goes through Node command pipe
to the send the file to the Google Cloud Storage command file.createWriteStream. The contentType
must be set to get a readable file, but auto
makes this easy. The file is then written to the bucket, which is set to my Firebase Cloud Storage folder Test_Folder
and the filename is myWordFileType
.
add a comment |
up vote
1
down vote
accepted
Thanks dpopp07, I got it!
exports.TextToSpeech = functions.firestore.document('Test_Word').onUpdate((change, context) => {
if (change.after.data().word != undefined) {
myWord = change.after.data().word;
myWordFileType = myWord + '.ogg';
var synthesizeParams = {
text: myWord,
accept: 'audio/ogg',
voice: 'en-US_AllisonVoice'
};
const {Storage} = require('@google-cloud/storage');
const storage = new Storage();
const bucket = storage.bucket('myapp.appspot.com');
const file = bucket.file('Test_Folder' + myWordFileType);
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(file.createWriteStream({contentType: 'auto'}))
.on('error', function(err) {})
.on('finish', function() {
console.log("Complete.");
});
}
return 0;
});
The function triggers when a new word is written to a Firestore location, then extracts the word and calls it myWord
. Adding .ogg makes myWordFileType
. The functions sends an HTTP request to IBM Watson Text-to-speech, which returns a callback, not a promise, so the code is a bit ugly. The crux is where the HTTP response goes through Node command pipe
to the send the file to the Google Cloud Storage command file.createWriteStream. The contentType
must be set to get a readable file, but auto
makes this easy. The file is then written to the bucket, which is set to my Firebase Cloud Storage folder Test_Folder
and the filename is myWordFileType
.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Thanks dpopp07, I got it!
exports.TextToSpeech = functions.firestore.document('Test_Word').onUpdate((change, context) => {
if (change.after.data().word != undefined) {
myWord = change.after.data().word;
myWordFileType = myWord + '.ogg';
var synthesizeParams = {
text: myWord,
accept: 'audio/ogg',
voice: 'en-US_AllisonVoice'
};
const {Storage} = require('@google-cloud/storage');
const storage = new Storage();
const bucket = storage.bucket('myapp.appspot.com');
const file = bucket.file('Test_Folder' + myWordFileType);
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(file.createWriteStream({contentType: 'auto'}))
.on('error', function(err) {})
.on('finish', function() {
console.log("Complete.");
});
}
return 0;
});
The function triggers when a new word is written to a Firestore location, then extracts the word and calls it myWord
. Adding .ogg makes myWordFileType
. The functions sends an HTTP request to IBM Watson Text-to-speech, which returns a callback, not a promise, so the code is a bit ugly. The crux is where the HTTP response goes through Node command pipe
to the send the file to the Google Cloud Storage command file.createWriteStream. The contentType
must be set to get a readable file, but auto
makes this easy. The file is then written to the bucket, which is set to my Firebase Cloud Storage folder Test_Folder
and the filename is myWordFileType
.
Thanks dpopp07, I got it!
exports.TextToSpeech = functions.firestore.document('Test_Word').onUpdate((change, context) => {
if (change.after.data().word != undefined) {
myWord = change.after.data().word;
myWordFileType = myWord + '.ogg';
var synthesizeParams = {
text: myWord,
accept: 'audio/ogg',
voice: 'en-US_AllisonVoice'
};
const {Storage} = require('@google-cloud/storage');
const storage = new Storage();
const bucket = storage.bucket('myapp.appspot.com');
const file = bucket.file('Test_Folder' + myWordFileType);
var TextToSpeechV1 = require('watson-developer-cloud/text-to-speech/v1');
var textToSpeech = new TextToSpeechV1({
username: 'groucho',
password: 'swordfish',
url: 'https://stream.watsonplatform.net/text-to-speech/api'
});
textToSpeech.synthesize(synthesizeParams).on('error', function(error) {
console.log(error);
}).pipe(file.createWriteStream({contentType: 'auto'}))
.on('error', function(err) {})
.on('finish', function() {
console.log("Complete.");
});
}
return 0;
});
The function triggers when a new word is written to a Firestore location, then extracts the word and calls it myWord
. Adding .ogg makes myWordFileType
. The functions sends an HTTP request to IBM Watson Text-to-speech, which returns a callback, not a promise, so the code is a bit ugly. The crux is where the HTTP response goes through Node command pipe
to the send the file to the Google Cloud Storage command file.createWriteStream. The contentType
must be set to get a readable file, but auto
makes this easy. The file is then written to the bucket, which is set to my Firebase Cloud Storage folder Test_Folder
and the filename is myWordFileType
.
edited Nov 9 at 16:36
answered Nov 9 at 0:09


Thomas David Kehoe
1,88211333
1,88211333
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53199168%2fdownloading-an-api-file-to-firebase-cloud-storage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H,Hx0,7,Qo3sG
I can help but I have a question first - what type of variable do you need
file
to be? ABuffer
? I mostly want to know, where is thatdownload()
function coming from - or is that pseudocode?– dpopp07
Nov 7 at 23:38
You need to return a promise from your function that resolves when all the work is complete.
pipe
is probably an async function, which means you need to start thinking more carefully about how you want to do async programming in your function.– Doug Stevenson
Nov 8 at 0:37
Doug, IBM Watson textToSpeech.synthesize returns a callback, not a promise. I'll look into Google text-to-speech, if that returns a promise will it be easier to code?
– Thomas David Kehoe
Nov 8 at 15:41
According to stackoverflow.com/questions/20085513/using-pipe-in-node-js-net, "The pipe() function [in Node] reads data from a readable stream as it becomes available and writes it to a destination writable stream." I want to write to a file. I'll look into whether there's a pipe-to-file Node command.
– Thomas David Kehoe
Nov 8 at 15:42
Another answer in stackoverflow.com/questions/20085513/using-pipe-in-node-js-net says that "pipe() reads from a readable stream and writes to a writeable stream, much like a Unix pipe. It does all "reasonable" things along the way with errors, end of files, if one side falls behind etc." That sounds like async.
– Thomas David Kehoe
Nov 8 at 15:44