How can I create a two dimensional array in JavaScript?
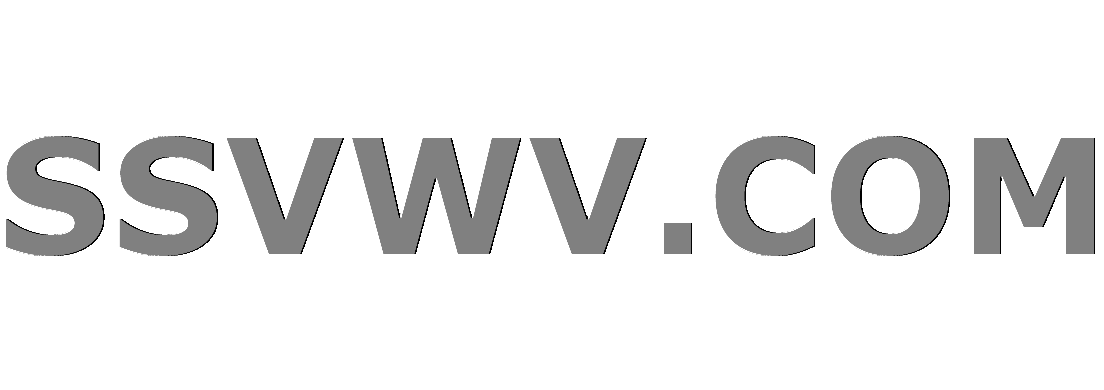
Multi tool use
up vote
965
down vote
favorite
I have been reading online and some places say it isn't possible, some say it is and then give an example and others refute the example, etc.
How do I declare a 2 dimensional array in JavaScript? (assuming it's possible)
How would I access its members? (
myArray[0][1]
ormyArray[0,1]
?)
javascript arrays multidimensional-array
|
show 3 more comments
up vote
965
down vote
favorite
I have been reading online and some places say it isn't possible, some say it is and then give an example and others refute the example, etc.
How do I declare a 2 dimensional array in JavaScript? (assuming it's possible)
How would I access its members? (
myArray[0][1]
ormyArray[0,1]
?)
javascript arrays multidimensional-array
13
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
For a 5x3 2D array I would do likevar arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do likevar arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
4
FYI... when you fill an array with more arrays usingvar arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.
– Josh Stribling
May 23 '16 at 8:51
22
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21
|
show 3 more comments
up vote
965
down vote
favorite
up vote
965
down vote
favorite
I have been reading online and some places say it isn't possible, some say it is and then give an example and others refute the example, etc.
How do I declare a 2 dimensional array in JavaScript? (assuming it's possible)
How would I access its members? (
myArray[0][1]
ormyArray[0,1]
?)
javascript arrays multidimensional-array
I have been reading online and some places say it isn't possible, some say it is and then give an example and others refute the example, etc.
How do I declare a 2 dimensional array in JavaScript? (assuming it's possible)
How would I access its members? (
myArray[0][1]
ormyArray[0,1]
?)
javascript arrays multidimensional-array
javascript arrays multidimensional-array
edited Apr 20 '15 at 2:52


royhowie
8,861133658
8,861133658
asked Jun 8 '09 at 18:24
Diego
6,26072744
6,26072744
13
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
For a 5x3 2D array I would do likevar arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do likevar arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
4
FYI... when you fill an array with more arrays usingvar arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.
– Josh Stribling
May 23 '16 at 8:51
22
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21
|
show 3 more comments
13
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
For a 5x3 2D array I would do likevar arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do likevar arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
4
FYI... when you fill an array with more arrays usingvar arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.
– Josh Stribling
May 23 '16 at 8:51
22
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21
13
13
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
For a 5x3 2D array I would do like
var arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do like var arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
For a 5x3 2D array I would do like
var arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do like var arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
4
4
FYI... when you fill an array with more arrays using
var arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.– Josh Stribling
May 23 '16 at 8:51
FYI... when you fill an array with more arrays using
var arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.– Josh Stribling
May 23 '16 at 8:51
22
22
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21
|
show 3 more comments
41 Answers
41
active
oldest
votes
1 2
next
up vote
1107
down vote
accepted
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
|
show 2 more comments
up vote
384
down vote
You simply make each item within the array an array.
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.new Array(size)
wheresize
is a variable.
– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
|
show 1 more comment
up vote
166
down vote
Similar to activa's answer, here's a function to create an n-dimensional array:
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while(i--) arr[length-1 - i] = createArray.apply(this, args);
}
return arr;
}
createArray(); // or new Array()
createArray(2); // new Array(2)
createArray(3, 2); // [new Array(2),
// new Array(2),
// new Array(2)]
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,var array = createArray(2, 3, 4, 5);
.
– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
add a comment |
up vote
71
down vote
Javascript only has 1-dimensional arrays, but you can build arrays of arrays, as others pointed out.
The following function can be used to construct a 2-d array of fixed dimensions:
function Create2DArray(rows) {
var arr = ;
for (var i=0;i<rows;i++) {
arr[i] = ;
}
return arr;
}
The number of columns is not really important, because it is not required to specify the size of an array before using it.
Then you can just call:
var arr = Create2DArray(100);
arr[50][2] = 5;
arr[70][5] = 7454;
// ...
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
add a comment |
up vote
63
down vote
The easiest way:
var myArray = [];
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
@Philip you have to setmyArray[1]=;
before assigningmyArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use[]
to define that it's a 2-dimensional array? Or simply make it, and we can use some method like
.push([2,3])
? DEMO
– piskebee
Oct 26 '15 at 13:20
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).myArray.length == 1
andmyArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.
– JonBrave
Nov 17 '16 at 9:20
|
show 2 more comments
up vote
41
down vote
The reason some say that it isn't possible is because a two dimensional array is really just an array of arrays. The other comments here provide perfectly valid methods of creating two dimensional arrays in JavaScript, but the purest point of view would be that you have a one dimensional array of objects, each of those objects would be a one dimensional array consisting of two elements.
So, that's the cause of the conflicting view points.
35
No, it's not. In some languages, you can have multidimensional arrays likestring[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.
– Rafael Soares
Aug 4 '11 at 15:29
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
add a comment |
up vote
26
down vote
Few people show the use of push:
To bring something new, I will show you how to initialize the matrix with some value, example: 0 or an empty string "".
Reminding that if you have a 10 elements array, in javascript the last index will be 9!
function matrix( rows, cols, defaultValue){
var arr = ;
// Creates all lines:
for(var i=0; i < rows; i++){
// Creates an empty line
arr.push();
// Adds cols to the empty line:
arr[i].push( new Array(cols));
for(var j=0; j < cols; j++){
// Initializes:
arr[i][j] = defaultValue;
}
}
return arr;
}
usage examples:
x = matrix( 2 , 3,''); // 2 lines, 3 cols filled with empty string
y = matrix( 10, 5, 0);// 10 lines, 5 cols filled with 0
add a comment |
up vote
23
down vote
Two-liner:
var a = ;
while(a.push() < 10);
It will generate an array a of the length 10, filled with arrays.
(Push adds an element to an array and returns the new length)
12
One-liner:for (var a=; a.push()<10;);
?
– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
@StinkyCat: Yes, that's howvar
works. It's always function-scoped.
– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
|
show 1 more comment
up vote
19
down vote
The sanest answer seems to be
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
Note we can't directly fill with the rows since fill uses shallow copy constructor, therefore all rows would share the same memory...here is example which demonstrates how each row would be shared (taken from other answers):
// DON'T do this: each row in arr, is shared
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo'; // also modifies arr[1][0]
console.info(arr);
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar usingArray.apply(null, Array(nrows))
but this is much more elegant.
– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support forfill
. This won't work on a majority of browsers.
– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:Array(nrows).map(() => 0)
, or,Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
add a comment |
up vote
17
down vote
How to create an empty two dimensional array (one-line)
Array.from(Array(2), () => new Array(4))
2 and 4 being first and second dimensions respectively.
We are making use of Array.from
, which can take an array-like param and an optional mapping for each of the elements.
Array.from(arrayLike[, mapFn[, thisArg]])
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
The same trick can be used to Create a JavaScript array containing 1...N
Alternatively (but more inefficient 12% with n = 10,000
)
Array(2).fill(null).map(() => Array(4))
The performance decrease comes with the fact that we have to have the first dimension values initialized to run .map
. Remember that Array
will not allocate the positions until you order it to through .fill
or direct value assignment.
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
Follow up
Why doesn't this work?
Array(2).fill(Array(4));
While it does return the apparently desired two dimensional array ([ [ <4 empty items> ], [ <4 empty items> ] ]
), there a catch: first dimension arrays have been copied by reference. That means a arr[0][0] = 'foo'
would actually change two rows instead of one.
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
I suggest this:Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
add a comment |
up vote
15
down vote
The easiest way:
var arr = ;
var arr1 = ['00','01'];
var arr2 = ['10','11'];
var arr3 = ['20','21'];
arr.push(arr1);
arr.push(arr2);
arr.push(arr3);
alert(arr[0][1]); // '01'
alert(arr[1][1]); // '11'
alert(arr[2][0]); // '20'
add a comment |
up vote
15
down vote
This is what i achieved :
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
This spelled me bineeshkumar
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
ButappVar[1][4] = "bineesh";
is wrong, how to solve it?
– Gank
May 20 at 13:50
add a comment |
up vote
13
down vote
Two dimensional arrays are created the same way single dimensional arrays are. And you access them like array[0][1]
.
var arr = [1, 2, [3, 4], 5];
alert (arr[2][1]); //alerts "4"
add a comment |
up vote
11
down vote
I'm not sure if anyone has answered this but I found this worked for me pretty well -
var array = [[,],[,]]
eg:
var a = [[1,2],[3,4]]
For a 2 dimensional array, for instance.
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
You don't need extra commasvar array = [,]
is adequate.
– Kaya Toast
Jan 31 '15 at 7:29
add a comment |
up vote
10
down vote
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns.
function Create2DArray(rows,columns) {
var x = new Array(rows);
for (var i = 0; i < rows; i++) {
x[i] = new Array(columns);
}
return x;
}
to create an Array use this method as below.
var array = Create2DArray(10,20);
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
add a comment |
up vote
8
down vote
Use Array Comprehensions
In JavaScript 1.7 and higher you can use array comprehensions to create two dimensional arrays. You can also filter and/or manipulate the entries while filling the array and don't have to use loops.
var rows = [1, 2, 3];
var cols = ["a", "b", "c", "d"];
var grid = [ for (r of rows) [ for (c of cols) r+c ] ];
/*
grid = [
["1a","1b","1c","1d"],
["2a","2b","2c","2d"],
["3a","3b","3c","3d"]
]
*/
You can create any n x m
array you want and fill it with a default value by calling
var default = 0; // your 2d array will be filled with this value
var n_dim = 2;
var m_dim = 7;
var arr = [ for (n of Array(n_dim)) [ for (m of Array(m_dim) default ]]
/*
arr = [
[0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0],
]
*/
More examples and documentation can be found here.
Please note that this is not a standard feature yet.
A quick google check here... yup... thefor
statement is still a loop...
– Pimp Trizkit
Mar 10 at 15:25
add a comment |
up vote
7
down vote
For one liner lovers Array.from()
// creates 8x8 array filed with "0"
const arr2d = Array.from({ length: 8 }, () => Array.from({ length: 8 }, () => "0"));
Another one (from comment by dmitry_romanov) use Array().fill()
// creates 8x8 array filed with "0"
const arr2d = Array(8).fill(0).map(() => Array(8).fill("0"));
2
we can remove0
in the firstfill()
function:const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
add a comment |
up vote
7
down vote
My approach is very similar to @Bineesh answer but with a more general approach.
You can declare the double array as follows:
var myDoubleArray = [];
And the storing and accessing the contents in the following manner:
var testArray1 = [9,8]
var testArray2 = [3,5,7,9,10]
var testArray3 = {"test":123}
var index = 0;
myDoubleArray[index++] = testArray1;
myDoubleArray[index++] = testArray2;
myDoubleArray[index++] = testArray3;
console.log(myDoubleArray[0],myDoubleArray[1][3], myDoubleArray[2]['test'],)
This will print the expected output
[ 9, 8 ] 9 123
add a comment |
up vote
5
down vote
I found below is the simplest way:
var array1 = [];
array1[0][100] = 5;
alert(array1[0][100]);
alert(array1.length);
alert(array1[0].length);
add a comment |
up vote
4
down vote
I had to make a flexible array function to add "records" to it as i needed and to be able to update them and do whatever calculations e needed before i sent it to a database for further processing. Here's the code, hope it helps :).
function Add2List(clmn1, clmn2, clmn3) {
aColumns.push(clmn1,clmn2,clmn3); // Creates array with "record"
aLine.splice(aPos, 0,aColumns); // Inserts new "record" at position aPos in main array
aColumns = ; // Resets temporary array
aPos++ // Increments position not to overlap previous "records"
}
Feel free to optimize and / or point out any bugs :)
How about justaLine.push([clmn1, clmn2, clmn3]);
?
– Pimp Trizkit
Mar 10 at 15:55
add a comment |
up vote
4
down vote
Javascript does not support two dimensional arrays, instead we store an array inside another array and fetch the data from that array depending on what position of that array you want to access. Remember array numeration starts at ZERO.
Code Example:
/* Two dimensional array that's 5 x 5
C0 C1 C2 C3 C4
R0[1][1][1][1][1]
R1[1][1][1][1][1]
R2[1][1][1][1][1]
R3[1][1][1][1][1]
R4[1][1][1][1][1]
*/
var row0 = [1,1,1,1,1],
row1 = [1,1,1,1,1],
row2 = [1,1,1,1,1],
row3 = [1,1,1,1,1],
row4 = [1,1,1,1,1];
var table = [row0,row1,row2,row3,row4];
console.log(table[0][0]); // Get the first item in the array
add a comment |
up vote
4
down vote
Below one, creates a 5x5 matrix and fill them with null
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
3
This answer is wrong. It will create an array with same array filling in its slots.md[1][0] = 3
and all the rest of elements are updated too
– Qiang
Nov 15 '16 at 6:33
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
add a comment |
up vote
3
down vote
Here's a quick way I've found to make a two dimensional array.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(e => Array(y));
}
You can easily turn this function into an ES5 function as well.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(function(e) {
return Array(y);
});
}
Why this works: the new Array(n)
constructor creates an object with a prototype of Array.prototype
and then assigns the object's length
, resulting in an unpopulated array. Due to its lack of actual members we can't run the Array.prototype.map
function on it.
However, when you provide more than one argument to the constructor, such as when you do Array(1, 2, 3, 4)
, the constructor will use the arguments
object to instantiate and populate an Array
object correctly.
For this reason, we can use Array.apply(null, Array(x))
, because the apply
function will spread the arguments into the constructor. For clarification, doing Array.apply(null, Array(3))
is equivalent to doing Array(null, null, null)
.
Now that we've created an actual populated array, all we need to do is call map
and create the second layer (y
).
add a comment |
up vote
3
down vote
To create a non-sparse "2D" array (x,y) with all indices addressable and values set to null:
let 2Darray = new Array(x).fill(null).map(item =>(new Array(y).fill(null)))
bonus "3D" Array (x,y,z)
let 3Darray = new Array(x).fill(null).map(item=>(new Array(y).fill(null)).map(item=>Array(z).fill(null)))
Variations and corrections on this have been mentioned in comments and at various points in response to this question but not as an actual answer so I am adding it here.
It should be noted that (similar to most other answers) this has O(x*y) time complexity so it probably not suitable for very large arrays.
add a comment |
up vote
2
down vote
You could allocate an array of rows, where each row is an array of the same length. Or you could allocate a one-dimensional array with rows*columns elements and define methods to map row/column coordinates to element indices.
Whichever implementation you pick, if you wrap it in an object you can define the accessor methods in a prototype to make the API easy to use.
add a comment |
up vote
2
down vote
I found that this code works for me:
var map = [
];
mapWidth = 50;
mapHeight = 50;
fillEmptyMap(map, mapWidth, mapHeight);
...
function fillEmptyMap(array, width, height) {
for (var x = 0; x < width; x++) {
array[x] = ;
for (var y = 0; y < height; y++) {
array[x][y] = [0];
}
}
}
add a comment |
up vote
2
down vote
A simplified example:
var blocks = ;
blocks[0] = ;
blocks[0][0] = 7;
add a comment |
up vote
2
down vote
One liner to create a m*n 2 dimensional array filled with 0.
new Array(m).fill(new Array(n).fill(0));
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
Yes, I confirm the gotcha. Quick fix:a = Array(m).fill(0).map(() => Array(n).fill(0))
?map
will untie reference and create unique array per slot.
– dmitry_romanov
Apr 15 '17 at 4:28
add a comment |
up vote
2
down vote
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
add a comment |
up vote
1
down vote
I've made a modification of Matthew Crumley's answer for creating a multidimensional array function. I've added the dimensions of the array to be passed as array variable and there will be another variable - value
, which will be used to set the values of the elements of the last arrays in the multidimensional array.
/*
* Function to create an n-dimensional array
*
* @param array dimensions
* @param any type value
*
* @return array array
*/
function createArray(dimensions, value) {
// Create new array
var array = new Array(dimensions[0] || 0);
var i = dimensions[0];
// If dimensions array's length is bigger than 1
// we start creating arrays in the array elements with recursions
// to achieve multidimensional array
if (dimensions.length > 1) {
// Remove the first value from the array
var args = Array.prototype.slice.call(dimensions, 1);
// For each index in the created array create a new array with recursion
while(i--) {
array[dimensions[0]-1 - i] = createArray(args, value);
}
// If there is only one element left in the dimensions array
// assign value to each of the new array's elements if value is set as param
} else {
if (typeof value !== 'undefined') {
while(i--) {
array[dimensions[0]-1 - i] = value;
}
}
}
return array;
}
createArray(); // or new Array()
createArray([2], 'empty'); // ['empty', 'empty']
createArray([3, 2], 0); // [[0, 0],
// [0, 0],
// [0, 0]]
add a comment |
1 2
next
protected by Mysticial Jul 24 '14 at 5:56
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
41 Answers
41
active
oldest
votes
41 Answers
41
active
oldest
votes
active
oldest
votes
active
oldest
votes
1 2
next
up vote
1107
down vote
accepted
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
|
show 2 more comments
up vote
1107
down vote
accepted
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
|
show 2 more comments
up vote
1107
down vote
accepted
up vote
1107
down vote
accepted
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
var items = [
[1, 2],
[3, 4],
[5, 6]
];
console.log(items[0][0]); // 1
console.log(items);
edited Sep 10 '16 at 15:20
Ruslan López
2,79811325
2,79811325
answered Jun 8 '09 at 18:27
Ballsacian1
13.9k21925
13.9k21925
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
|
show 2 more comments
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
22
22
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
It would be difficult to initialize a large multidimensional array this way. However, this function can be used to create an empty multidimensional, with the dimensions specified as parameters.
– Anderson Green
Apr 6 '13 at 16:49
2
2
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
@AndersonGreen It's a good thing you mentioned a link for those interested in multi-D array solution, but the question and Ballsacian1's answer are about "2D" array, not "multi-D" array
– evilReiko
Jun 14 '14 at 9:56
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
You should go through the whole thing... e.g. alert(items[0][1]); // 2 etc.
– Dois
May 28 '15 at 8:11
1
1
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
@SashikaXP, this does not work for first indices other than 0.
– Michael Franzl
Dec 30 '15 at 17:55
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
The question is how to declare a two dimensional array. Which is what I was looking for and found this and following answers which fail to discern the difference between declare and initialize. There's also declaration with known length or unbounded, neither of which is discussed.
– chris
May 30 '16 at 1:20
|
show 2 more comments
up vote
384
down vote
You simply make each item within the array an array.
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.new Array(size)
wheresize
is a variable.
– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
|
show 1 more comment
up vote
384
down vote
You simply make each item within the array an array.
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.new Array(size)
wheresize
is a variable.
– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
|
show 1 more comment
up vote
384
down vote
up vote
384
down vote
You simply make each item within the array an array.
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
You simply make each item within the array an array.
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
var x = new Array(10);
for (var i = 0; i < x.length; i++) {
x[i] = new Array(3);
}
console.log(x);
edited Aug 29 at 8:56
vsync
44.6k35154217
44.6k35154217
answered Jun 8 '09 at 18:28
Sufian
6,37431721
6,37431721
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.new Array(size)
wheresize
is a variable.
– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
|
show 1 more comment
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.new Array(size)
wheresize
is a variable.
– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
5
5
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
Can they use things like strings for their keys and values? myArray['Book']['item1'] ?
– Diego
Jun 8 '09 at 19:54
34
34
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
@Diego, yes, but that's not what arrays are intended for. It's better to use an object when your keys are strings.
– Matthew Crumley
Jun 8 '09 at 20:05
7
7
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.
new Array(size)
where size
is a variable.– Variadicism
Sep 12 '15 at 22:44
I like this example better than the accepted answer because this can be implemented for dynamically sized arrays, e.g.
new Array(size)
where size
is a variable.– Variadicism
Sep 12 '15 at 22:44
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
doesn't work - error on assignment. How has this answer got 280 likes if it is useless?
– Gargo
Sep 15 '16 at 18:51
1
1
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
This is working, thanks. You can see the example Gargo jsfiddle.net/matasoy/oetw73sj
– matasoy
Sep 23 '16 at 7:23
|
show 1 more comment
up vote
166
down vote
Similar to activa's answer, here's a function to create an n-dimensional array:
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while(i--) arr[length-1 - i] = createArray.apply(this, args);
}
return arr;
}
createArray(); // or new Array()
createArray(2); // new Array(2)
createArray(3, 2); // [new Array(2),
// new Array(2),
// new Array(2)]
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,var array = createArray(2, 3, 4, 5);
.
– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
add a comment |
up vote
166
down vote
Similar to activa's answer, here's a function to create an n-dimensional array:
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while(i--) arr[length-1 - i] = createArray.apply(this, args);
}
return arr;
}
createArray(); // or new Array()
createArray(2); // new Array(2)
createArray(3, 2); // [new Array(2),
// new Array(2),
// new Array(2)]
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,var array = createArray(2, 3, 4, 5);
.
– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
add a comment |
up vote
166
down vote
up vote
166
down vote
Similar to activa's answer, here's a function to create an n-dimensional array:
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while(i--) arr[length-1 - i] = createArray.apply(this, args);
}
return arr;
}
createArray(); // or new Array()
createArray(2); // new Array(2)
createArray(3, 2); // [new Array(2),
// new Array(2),
// new Array(2)]
Similar to activa's answer, here's a function to create an n-dimensional array:
function createArray(length) {
var arr = new Array(length || 0),
i = length;
if (arguments.length > 1) {
var args = Array.prototype.slice.call(arguments, 1);
while(i--) arr[length-1 - i] = createArray.apply(this, args);
}
return arr;
}
createArray(); // or new Array()
createArray(2); // new Array(2)
createArray(3, 2); // [new Array(2),
// new Array(2),
// new Array(2)]
edited Mar 31 '13 at 8:46
yckart
20.4k492105
20.4k492105
answered Jun 8 '09 at 20:49
Matthew Crumley
79.7k2093117
79.7k2093117
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,var array = createArray(2, 3, 4, 5);
.
– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
add a comment |
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,var array = createArray(2, 3, 4, 5);
.
– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
1
1
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
Can this create a 4 dimensional array?
– trusktr
May 19 '11 at 2:18
3
3
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,
var array = createArray(2, 3, 4, 5);
.– Matthew Crumley
May 19 '11 at 4:21
@trusktr: Yes, you could create as many dimensions as you want (within your memory constraints). Just pass in the length of the four dimensions. For example,
var array = createArray(2, 3, 4, 5);
.– Matthew Crumley
May 19 '11 at 4:21
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
Nice! I actually asked about this here: stackoverflow.com/questions/6053332/javascript-4d-arrays and a variety of interesting answers.
– trusktr
May 19 '11 at 5:50
2
2
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
Best answer ! However, I would not recommend to use it with 0 or 1 parameters (useless)
– Apolo
May 15 '14 at 14:11
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
n-dimensional you say? Can this create a 5 dimensional array?
– BritishDeveloper
Jun 19 '15 at 22:19
add a comment |
up vote
71
down vote
Javascript only has 1-dimensional arrays, but you can build arrays of arrays, as others pointed out.
The following function can be used to construct a 2-d array of fixed dimensions:
function Create2DArray(rows) {
var arr = ;
for (var i=0;i<rows;i++) {
arr[i] = ;
}
return arr;
}
The number of columns is not really important, because it is not required to specify the size of an array before using it.
Then you can just call:
var arr = Create2DArray(100);
arr[50][2] = 5;
arr[70][5] = 7454;
// ...
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
add a comment |
up vote
71
down vote
Javascript only has 1-dimensional arrays, but you can build arrays of arrays, as others pointed out.
The following function can be used to construct a 2-d array of fixed dimensions:
function Create2DArray(rows) {
var arr = ;
for (var i=0;i<rows;i++) {
arr[i] = ;
}
return arr;
}
The number of columns is not really important, because it is not required to specify the size of an array before using it.
Then you can just call:
var arr = Create2DArray(100);
arr[50][2] = 5;
arr[70][5] = 7454;
// ...
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
add a comment |
up vote
71
down vote
up vote
71
down vote
Javascript only has 1-dimensional arrays, but you can build arrays of arrays, as others pointed out.
The following function can be used to construct a 2-d array of fixed dimensions:
function Create2DArray(rows) {
var arr = ;
for (var i=0;i<rows;i++) {
arr[i] = ;
}
return arr;
}
The number of columns is not really important, because it is not required to specify the size of an array before using it.
Then you can just call:
var arr = Create2DArray(100);
arr[50][2] = 5;
arr[70][5] = 7454;
// ...
Javascript only has 1-dimensional arrays, but you can build arrays of arrays, as others pointed out.
The following function can be used to construct a 2-d array of fixed dimensions:
function Create2DArray(rows) {
var arr = ;
for (var i=0;i<rows;i++) {
arr[i] = ;
}
return arr;
}
The number of columns is not really important, because it is not required to specify the size of an array before using it.
Then you can just call:
var arr = Create2DArray(100);
arr[50][2] = 5;
arr[70][5] = 7454;
// ...
answered Jun 8 '09 at 18:32


Philippe Leybaert
129k25185209
129k25185209
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
add a comment |
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
i want to make a 2-dim array that would represent a deck of cards. Which would be a 2-dim array that holds the card value and then in then the suit. What would be the easiest way to do that.
– Doug Hauf
Mar 3 '14 at 17:58
1
1
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
function Create2DArray(rows) { var arr = ; for (var i=0;i<rows;i++) { arr[i] = ; } return arr; } function print(deck) { for(t=1;t<=4;t++) { for (i=1;i<=13;i++) { document.writeln(deck[t][i]); } } } fucntion fillDeck(d) { for(t=1;t<=4;t++) { myCardDeck[t][1] = t; for (i=1;i<=13;i++) { myCardDeck[t][i] = i; } } } function loadCardDeck() { var myCardDeck = Create2DArray(13); fillDeck(myCardDeck); print(myCardDeck); }
– Doug Hauf
Mar 3 '14 at 17:58
2
2
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@Doug: You actually want a one-dimensional array of objects with 2 attributes. var deck= ; deck[0]= { face:1, suit:'H'};
– TeasingDart
Sep 18 '15 at 23:21
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
@DougHauf that's a minified 2D-array ?? :P :D
– Mahi
Nov 9 '16 at 12:50
add a comment |
up vote
63
down vote
The easiest way:
var myArray = [];
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
@Philip you have to setmyArray[1]=;
before assigningmyArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use[]
to define that it's a 2-dimensional array? Or simply make it, and we can use some method like
.push([2,3])
? DEMO
– piskebee
Oct 26 '15 at 13:20
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).myArray.length == 1
andmyArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.
– JonBrave
Nov 17 '16 at 9:20
|
show 2 more comments
up vote
63
down vote
The easiest way:
var myArray = [];
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
@Philip you have to setmyArray[1]=;
before assigningmyArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use[]
to define that it's a 2-dimensional array? Or simply make it, and we can use some method like
.push([2,3])
? DEMO
– piskebee
Oct 26 '15 at 13:20
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).myArray.length == 1
andmyArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.
– JonBrave
Nov 17 '16 at 9:20
|
show 2 more comments
up vote
63
down vote
up vote
63
down vote
The easiest way:
var myArray = [];
The easiest way:
var myArray = [];
answered Jan 22 '13 at 18:47
Fred
67152
67152
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
@Philip you have to setmyArray[1]=;
before assigningmyArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use[]
to define that it's a 2-dimensional array? Or simply make it, and we can use some method like
.push([2,3])
? DEMO
– piskebee
Oct 26 '15 at 13:20
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).myArray.length == 1
andmyArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.
– JonBrave
Nov 17 '16 at 9:20
|
show 2 more comments
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
@Philip you have to setmyArray[1]=;
before assigningmyArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use[]
to define that it's a 2-dimensional array? Or simply make it, and we can use some method like
.push([2,3])
? DEMO
– piskebee
Oct 26 '15 at 13:20
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).myArray.length == 1
andmyArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.
– JonBrave
Nov 17 '16 at 9:20
27
27
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
which is a 2-dimension array
– Maurizio In denmark
Jul 2 '13 at 13:07
11
11
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
Yeah, careful with that. Assigning myArray[0][whatever] is fine, but try and set myArray[1][whatever] and it complains that myArray[1] is undefined.
– Philip
Apr 17 '14 at 16:24
17
17
@Philip you have to set
myArray[1]=;
before assigning myArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
@Philip you have to set
myArray[1]=;
before assigning myArray[1][0]=5;
– 182764125216
Sep 19 '14 at 20:14
Should we use
[]
to define that it's a 2-dimensional array? Or simply make it
, and we can use some method like .push([2,3])
? DEMO– piskebee
Oct 26 '15 at 13:20
Should we use
[]
to define that it's a 2-dimensional array? Or simply make it
, and we can use some method like .push([2,3])
? DEMO– piskebee
Oct 26 '15 at 13:20
3
3
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).
myArray.length == 1
and myArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.– JonBrave
Nov 17 '16 at 9:20
Be aware, this does not "create an empty 1x1 array" as @AndersonGreen wrote. It creates a "1x0" array (i.e. 1 row containing an array with 0 columns).
myArray.length == 1
and myArray[0].length == 0
. Which then gives the wrong result if you then copy a "genuinely empty" "0x0" array into it.– JonBrave
Nov 17 '16 at 9:20
|
show 2 more comments
up vote
41
down vote
The reason some say that it isn't possible is because a two dimensional array is really just an array of arrays. The other comments here provide perfectly valid methods of creating two dimensional arrays in JavaScript, but the purest point of view would be that you have a one dimensional array of objects, each of those objects would be a one dimensional array consisting of two elements.
So, that's the cause of the conflicting view points.
35
No, it's not. In some languages, you can have multidimensional arrays likestring[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.
– Rafael Soares
Aug 4 '11 at 15:29
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
add a comment |
up vote
41
down vote
The reason some say that it isn't possible is because a two dimensional array is really just an array of arrays. The other comments here provide perfectly valid methods of creating two dimensional arrays in JavaScript, but the purest point of view would be that you have a one dimensional array of objects, each of those objects would be a one dimensional array consisting of two elements.
So, that's the cause of the conflicting view points.
35
No, it's not. In some languages, you can have multidimensional arrays likestring[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.
– Rafael Soares
Aug 4 '11 at 15:29
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
add a comment |
up vote
41
down vote
up vote
41
down vote
The reason some say that it isn't possible is because a two dimensional array is really just an array of arrays. The other comments here provide perfectly valid methods of creating two dimensional arrays in JavaScript, but the purest point of view would be that you have a one dimensional array of objects, each of those objects would be a one dimensional array consisting of two elements.
So, that's the cause of the conflicting view points.
The reason some say that it isn't possible is because a two dimensional array is really just an array of arrays. The other comments here provide perfectly valid methods of creating two dimensional arrays in JavaScript, but the purest point of view would be that you have a one dimensional array of objects, each of those objects would be a one dimensional array consisting of two elements.
So, that's the cause of the conflicting view points.
answered Jun 8 '09 at 18:33
James Conigliaro
3,5851421
3,5851421
35
No, it's not. In some languages, you can have multidimensional arrays likestring[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.
– Rafael Soares
Aug 4 '11 at 15:29
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
add a comment |
35
No, it's not. In some languages, you can have multidimensional arrays likestring[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.
– Rafael Soares
Aug 4 '11 at 15:29
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
35
35
No, it's not. In some languages, you can have multidimensional arrays like
string[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.– Rafael Soares
Aug 4 '11 at 15:29
No, it's not. In some languages, you can have multidimensional arrays like
string[3,5] = "foo";
. It's a better approach for some scenarios, because the Y axis is not actually a child of the X axis.– Rafael Soares
Aug 4 '11 at 15:29
2
2
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Once it gets to the underlying machine code, all tensors of dimension > 1 are arrays of arrays, whichever language we are talking about. It is worthwhile keeping this in mind for reasons of cache optimisation. Any decent language that caters seriously for numerical computing will allow you to align your multidimensional structure in memory such that your most-used dimension is stored contiguously. Python's Numpy, Fortran, and C, come to mind. Indeed there are cases when it is worthwhile to reduce dimensionality into multiple structures for this reason.
– Thomas Browne
Oct 27 '14 at 18:18
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
Computers have no notion of dimensions. There is only 1 dimension, the memory address. Everything else is notational decoration for the benefit of the programmer.
– TeasingDart
Sep 18 '15 at 23:23
3
3
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
@ThomasBrowne Not exactly. "Arrays of arrays" require some storage for the sizes of inner arrays (they may differ) and another pointer dereferencing to find the place where an inner array is stored. In any "decent" language multidimentional arrays differ from jagged arrays, because they're different data structures per se. (And the confusing part is that C arrays are multidimentional, even though they're indexed with [a][b] syntax.)
– polkovnikov.ph
Dec 18 '15 at 23:20
add a comment |
up vote
26
down vote
Few people show the use of push:
To bring something new, I will show you how to initialize the matrix with some value, example: 0 or an empty string "".
Reminding that if you have a 10 elements array, in javascript the last index will be 9!
function matrix( rows, cols, defaultValue){
var arr = ;
// Creates all lines:
for(var i=0; i < rows; i++){
// Creates an empty line
arr.push();
// Adds cols to the empty line:
arr[i].push( new Array(cols));
for(var j=0; j < cols; j++){
// Initializes:
arr[i][j] = defaultValue;
}
}
return arr;
}
usage examples:
x = matrix( 2 , 3,''); // 2 lines, 3 cols filled with empty string
y = matrix( 10, 5, 0);// 10 lines, 5 cols filled with 0
add a comment |
up vote
26
down vote
Few people show the use of push:
To bring something new, I will show you how to initialize the matrix with some value, example: 0 or an empty string "".
Reminding that if you have a 10 elements array, in javascript the last index will be 9!
function matrix( rows, cols, defaultValue){
var arr = ;
// Creates all lines:
for(var i=0; i < rows; i++){
// Creates an empty line
arr.push();
// Adds cols to the empty line:
arr[i].push( new Array(cols));
for(var j=0; j < cols; j++){
// Initializes:
arr[i][j] = defaultValue;
}
}
return arr;
}
usage examples:
x = matrix( 2 , 3,''); // 2 lines, 3 cols filled with empty string
y = matrix( 10, 5, 0);// 10 lines, 5 cols filled with 0
add a comment |
up vote
26
down vote
up vote
26
down vote
Few people show the use of push:
To bring something new, I will show you how to initialize the matrix with some value, example: 0 or an empty string "".
Reminding that if you have a 10 elements array, in javascript the last index will be 9!
function matrix( rows, cols, defaultValue){
var arr = ;
// Creates all lines:
for(var i=0; i < rows; i++){
// Creates an empty line
arr.push();
// Adds cols to the empty line:
arr[i].push( new Array(cols));
for(var j=0; j < cols; j++){
// Initializes:
arr[i][j] = defaultValue;
}
}
return arr;
}
usage examples:
x = matrix( 2 , 3,''); // 2 lines, 3 cols filled with empty string
y = matrix( 10, 5, 0);// 10 lines, 5 cols filled with 0
Few people show the use of push:
To bring something new, I will show you how to initialize the matrix with some value, example: 0 or an empty string "".
Reminding that if you have a 10 elements array, in javascript the last index will be 9!
function matrix( rows, cols, defaultValue){
var arr = ;
// Creates all lines:
for(var i=0; i < rows; i++){
// Creates an empty line
arr.push();
// Adds cols to the empty line:
arr[i].push( new Array(cols));
for(var j=0; j < cols; j++){
// Initializes:
arr[i][j] = defaultValue;
}
}
return arr;
}
usage examples:
x = matrix( 2 , 3,''); // 2 lines, 3 cols filled with empty string
y = matrix( 10, 5, 0);// 10 lines, 5 cols filled with 0
answered Aug 8 '13 at 2:22
Sergio Abreu
1,5931613
1,5931613
add a comment |
add a comment |
up vote
23
down vote
Two-liner:
var a = ;
while(a.push() < 10);
It will generate an array a of the length 10, filled with arrays.
(Push adds an element to an array and returns the new length)
12
One-liner:for (var a=; a.push()<10;);
?
– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
@StinkyCat: Yes, that's howvar
works. It's always function-scoped.
– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
|
show 1 more comment
up vote
23
down vote
Two-liner:
var a = ;
while(a.push() < 10);
It will generate an array a of the length 10, filled with arrays.
(Push adds an element to an array and returns the new length)
12
One-liner:for (var a=; a.push()<10;);
?
– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
@StinkyCat: Yes, that's howvar
works. It's always function-scoped.
– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
|
show 1 more comment
up vote
23
down vote
up vote
23
down vote
Two-liner:
var a = ;
while(a.push() < 10);
It will generate an array a of the length 10, filled with arrays.
(Push adds an element to an array and returns the new length)
Two-liner:
var a = ;
while(a.push() < 10);
It will generate an array a of the length 10, filled with arrays.
(Push adds an element to an array and returns the new length)
answered May 26 '14 at 10:00
domenukk
7191121
7191121
12
One-liner:for (var a=; a.push()<10;);
?
– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
@StinkyCat: Yes, that's howvar
works. It's always function-scoped.
– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
|
show 1 more comment
12
One-liner:for (var a=; a.push()<10;);
?
– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
@StinkyCat: Yes, that's howvar
works. It's always function-scoped.
– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
12
12
One-liner:
for (var a=; a.push()<10;);
?– Bergi
Jul 7 '14 at 22:07
One-liner:
for (var a=; a.push()<10;);
?– Bergi
Jul 7 '14 at 22:07
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
@Bergi will the a variable still be defined in the next line..?
– StinkyCat
Apr 11 '16 at 10:48
1
1
@StinkyCat: Yes, that's how
var
works. It's always function-scoped.– Bergi
Apr 11 '16 at 10:51
@StinkyCat: Yes, that's how
var
works. It's always function-scoped.– Bergi
Apr 11 '16 at 10:51
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
I know, therefore your one-liner is useless in this case: you cannot "access its members" (check question)
– StinkyCat
Apr 11 '16 at 11:05
1
1
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
domenukk and @Bergi, you're both correct. I tried it out and I can access a after the for. I apologize! and thank you, may this be a lesson to me ;)
– StinkyCat
Apr 13 '16 at 14:06
|
show 1 more comment
up vote
19
down vote
The sanest answer seems to be
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
Note we can't directly fill with the rows since fill uses shallow copy constructor, therefore all rows would share the same memory...here is example which demonstrates how each row would be shared (taken from other answers):
// DON'T do this: each row in arr, is shared
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo'; // also modifies arr[1][0]
console.info(arr);
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar usingArray.apply(null, Array(nrows))
but this is much more elegant.
– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support forfill
. This won't work on a majority of browsers.
– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:Array(nrows).map(() => 0)
, or,Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
add a comment |
up vote
19
down vote
The sanest answer seems to be
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
Note we can't directly fill with the rows since fill uses shallow copy constructor, therefore all rows would share the same memory...here is example which demonstrates how each row would be shared (taken from other answers):
// DON'T do this: each row in arr, is shared
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo'; // also modifies arr[1][0]
console.info(arr);
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar usingArray.apply(null, Array(nrows))
but this is much more elegant.
– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support forfill
. This won't work on a majority of browsers.
– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:Array(nrows).map(() => 0)
, or,Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
add a comment |
up vote
19
down vote
up vote
19
down vote
The sanest answer seems to be
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
Note we can't directly fill with the rows since fill uses shallow copy constructor, therefore all rows would share the same memory...here is example which demonstrates how each row would be shared (taken from other answers):
// DON'T do this: each row in arr, is shared
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo'; // also modifies arr[1][0]
console.info(arr);
The sanest answer seems to be
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
Note we can't directly fill with the rows since fill uses shallow copy constructor, therefore all rows would share the same memory...here is example which demonstrates how each row would be shared (taken from other answers):
// DON'T do this: each row in arr, is shared
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo'; // also modifies arr[1][0]
console.info(arr);
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
var nrows = ~~(Math.random() * 10);
var ncols = ~~(Math.random() * 10);
console.log(`rows:${nrows}`);
console.log(`cols:${ncols}`);
var matrix = new Array(nrows).fill(0).map(row => new Array(ncols).fill(0));
console.log(matrix);
edited Jul 28 at 11:00
giorgim
13.8k31957
13.8k31957
answered Feb 15 '16 at 4:19


Francesco Dondi
783515
783515
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar usingArray.apply(null, Array(nrows))
but this is much more elegant.
– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support forfill
. This won't work on a majority of browsers.
– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:Array(nrows).map(() => 0)
, or,Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
add a comment |
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar usingArray.apply(null, Array(nrows))
but this is much more elegant.
– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support forfill
. This won't work on a majority of browsers.
– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:Array(nrows).map(() => 0)
, or,Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
It works. jsfiddle.net/trdnhy9q
– VladSavitsky
Mar 15 '16 at 15:26
This should be at the very top. I did something similar using
Array.apply(null, Array(nrows))
but this is much more elegant.– dimiguel
Mar 25 '16 at 6:05
This should be at the very top. I did something similar using
Array.apply(null, Array(nrows))
but this is much more elegant.– dimiguel
Mar 25 '16 at 6:05
This regard my last comment... Internet Explorer and Opera don't have support for
fill
. This won't work on a majority of browsers.– dimiguel
Mar 25 '16 at 20:50
This regard my last comment... Internet Explorer and Opera don't have support for
fill
. This won't work on a majority of browsers.– dimiguel
Mar 25 '16 at 20:50
@dimgl Fill can be emulated in this instance with a constant map:
Array(nrows).map(() => 0)
, or, Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
@dimgl Fill can be emulated in this instance with a constant map:
Array(nrows).map(() => 0)
, or, Array(nrows).map(function(){ return 0; });
– Conor O'Brien
Jan 17 '17 at 18:56
add a comment |
up vote
17
down vote
How to create an empty two dimensional array (one-line)
Array.from(Array(2), () => new Array(4))
2 and 4 being first and second dimensions respectively.
We are making use of Array.from
, which can take an array-like param and an optional mapping for each of the elements.
Array.from(arrayLike[, mapFn[, thisArg]])
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
The same trick can be used to Create a JavaScript array containing 1...N
Alternatively (but more inefficient 12% with n = 10,000
)
Array(2).fill(null).map(() => Array(4))
The performance decrease comes with the fact that we have to have the first dimension values initialized to run .map
. Remember that Array
will not allocate the positions until you order it to through .fill
or direct value assignment.
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
Follow up
Why doesn't this work?
Array(2).fill(Array(4));
While it does return the apparently desired two dimensional array ([ [ <4 empty items> ], [ <4 empty items> ] ]
), there a catch: first dimension arrays have been copied by reference. That means a arr[0][0] = 'foo'
would actually change two rows instead of one.
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
I suggest this:Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
add a comment |
up vote
17
down vote
How to create an empty two dimensional array (one-line)
Array.from(Array(2), () => new Array(4))
2 and 4 being first and second dimensions respectively.
We are making use of Array.from
, which can take an array-like param and an optional mapping for each of the elements.
Array.from(arrayLike[, mapFn[, thisArg]])
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
The same trick can be used to Create a JavaScript array containing 1...N
Alternatively (but more inefficient 12% with n = 10,000
)
Array(2).fill(null).map(() => Array(4))
The performance decrease comes with the fact that we have to have the first dimension values initialized to run .map
. Remember that Array
will not allocate the positions until you order it to through .fill
or direct value assignment.
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
Follow up
Why doesn't this work?
Array(2).fill(Array(4));
While it does return the apparently desired two dimensional array ([ [ <4 empty items> ], [ <4 empty items> ] ]
), there a catch: first dimension arrays have been copied by reference. That means a arr[0][0] = 'foo'
would actually change two rows instead of one.
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
I suggest this:Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
add a comment |
up vote
17
down vote
up vote
17
down vote
How to create an empty two dimensional array (one-line)
Array.from(Array(2), () => new Array(4))
2 and 4 being first and second dimensions respectively.
We are making use of Array.from
, which can take an array-like param and an optional mapping for each of the elements.
Array.from(arrayLike[, mapFn[, thisArg]])
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
The same trick can be used to Create a JavaScript array containing 1...N
Alternatively (but more inefficient 12% with n = 10,000
)
Array(2).fill(null).map(() => Array(4))
The performance decrease comes with the fact that we have to have the first dimension values initialized to run .map
. Remember that Array
will not allocate the positions until you order it to through .fill
or direct value assignment.
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
Follow up
Why doesn't this work?
Array(2).fill(Array(4));
While it does return the apparently desired two dimensional array ([ [ <4 empty items> ], [ <4 empty items> ] ]
), there a catch: first dimension arrays have been copied by reference. That means a arr[0][0] = 'foo'
would actually change two rows instead of one.
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
How to create an empty two dimensional array (one-line)
Array.from(Array(2), () => new Array(4))
2 and 4 being first and second dimensions respectively.
We are making use of Array.from
, which can take an array-like param and an optional mapping for each of the elements.
Array.from(arrayLike[, mapFn[, thisArg]])
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
The same trick can be used to Create a JavaScript array containing 1...N
Alternatively (but more inefficient 12% with n = 10,000
)
Array(2).fill(null).map(() => Array(4))
The performance decrease comes with the fact that we have to have the first dimension values initialized to run .map
. Remember that Array
will not allocate the positions until you order it to through .fill
or direct value assignment.
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
Follow up
Why doesn't this work?
Array(2).fill(Array(4));
While it does return the apparently desired two dimensional array ([ [ <4 empty items> ], [ <4 empty items> ] ]
), there a catch: first dimension arrays have been copied by reference. That means a arr[0][0] = 'foo'
would actually change two rows instead of one.
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
var arr = Array.from(Array(2), () => new Array(4));
arr[0][0] = 'foo';
console.info(arr);
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
var arr = Array(2).fill(null).map(() => Array(4));
arr[0][0] = 'foo';
console.info(arr);
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
var arr = Array(2).fill(Array(4));
arr[0][0] = 'foo';
console.info(arr);
console.info(arr[0][0], arr[1][0]);
edited Mar 9 at 22:38
answered Mar 9 at 19:53


zurfyx
12.2k106797
12.2k106797
I suggest this:Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
add a comment |
I suggest this:Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
I suggest this:
Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
I suggest this:
Array.from({length:5}, () => )
– vsync
Aug 29 at 9:11
add a comment |
up vote
15
down vote
The easiest way:
var arr = ;
var arr1 = ['00','01'];
var arr2 = ['10','11'];
var arr3 = ['20','21'];
arr.push(arr1);
arr.push(arr2);
arr.push(arr3);
alert(arr[0][1]); // '01'
alert(arr[1][1]); // '11'
alert(arr[2][0]); // '20'
add a comment |
up vote
15
down vote
The easiest way:
var arr = ;
var arr1 = ['00','01'];
var arr2 = ['10','11'];
var arr3 = ['20','21'];
arr.push(arr1);
arr.push(arr2);
arr.push(arr3);
alert(arr[0][1]); // '01'
alert(arr[1][1]); // '11'
alert(arr[2][0]); // '20'
add a comment |
up vote
15
down vote
up vote
15
down vote
The easiest way:
var arr = ;
var arr1 = ['00','01'];
var arr2 = ['10','11'];
var arr3 = ['20','21'];
arr.push(arr1);
arr.push(arr2);
arr.push(arr3);
alert(arr[0][1]); // '01'
alert(arr[1][1]); // '11'
alert(arr[2][0]); // '20'
The easiest way:
var arr = ;
var arr1 = ['00','01'];
var arr2 = ['10','11'];
var arr3 = ['20','21'];
arr.push(arr1);
arr.push(arr2);
arr.push(arr3);
alert(arr[0][1]); // '01'
alert(arr[1][1]); // '11'
alert(arr[2][0]); // '20'
edited Dec 27 '13 at 13:32
answered Dec 27 '13 at 8:59


Chicharito
85752547
85752547
add a comment |
add a comment |
up vote
15
down vote
This is what i achieved :
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
This spelled me bineeshkumar
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
ButappVar[1][4] = "bineesh";
is wrong, how to solve it?
– Gank
May 20 at 13:50
add a comment |
up vote
15
down vote
This is what i achieved :
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
This spelled me bineeshkumar
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
ButappVar[1][4] = "bineesh";
is wrong, how to solve it?
– Gank
May 20 at 13:50
add a comment |
up vote
15
down vote
up vote
15
down vote
This is what i achieved :
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
This spelled me bineeshkumar
This is what i achieved :
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
This spelled me bineeshkumar
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
var appVar = [];
appVar[0][4] = "bineesh";
appVar[0][5] = "kumar";
console.log(appVar[0][4] + appVar[0][5]);
console.log(appVar);
edited Sep 10 '16 at 6:35
Ruslan López
2,79811325
2,79811325
answered May 18 '16 at 15:16
Bineesh
348316
348316
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
ButappVar[1][4] = "bineesh";
is wrong, how to solve it?
– Gank
May 20 at 13:50
add a comment |
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
ButappVar[1][4] = "bineesh";
is wrong, how to solve it?
– Gank
May 20 at 13:50
1
1
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
Notice how you can only access the 0 index of the parent array. This isn't as useful as something which allows you to set, for example, appVar[5][9] = 10; ... you would get 'Unable to set property "9" of undefined' with this.
– RaisinBranCrunch
Jul 30 '17 at 16:38
But
appVar[1][4] = "bineesh";
is wrong, how to solve it?– Gank
May 20 at 13:50
But
appVar[1][4] = "bineesh";
is wrong, how to solve it?– Gank
May 20 at 13:50
add a comment |
up vote
13
down vote
Two dimensional arrays are created the same way single dimensional arrays are. And you access them like array[0][1]
.
var arr = [1, 2, [3, 4], 5];
alert (arr[2][1]); //alerts "4"
add a comment |
up vote
13
down vote
Two dimensional arrays are created the same way single dimensional arrays are. And you access them like array[0][1]
.
var arr = [1, 2, [3, 4], 5];
alert (arr[2][1]); //alerts "4"
add a comment |
up vote
13
down vote
up vote
13
down vote
Two dimensional arrays are created the same way single dimensional arrays are. And you access them like array[0][1]
.
var arr = [1, 2, [3, 4], 5];
alert (arr[2][1]); //alerts "4"
Two dimensional arrays are created the same way single dimensional arrays are. And you access them like array[0][1]
.
var arr = [1, 2, [3, 4], 5];
alert (arr[2][1]); //alerts "4"
answered Jun 8 '09 at 18:27
tj111
18k65273
18k65273
add a comment |
add a comment |
up vote
11
down vote
I'm not sure if anyone has answered this but I found this worked for me pretty well -
var array = [[,],[,]]
eg:
var a = [[1,2],[3,4]]
For a 2 dimensional array, for instance.
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
You don't need extra commasvar array = [,]
is adequate.
– Kaya Toast
Jan 31 '15 at 7:29
add a comment |
up vote
11
down vote
I'm not sure if anyone has answered this but I found this worked for me pretty well -
var array = [[,],[,]]
eg:
var a = [[1,2],[3,4]]
For a 2 dimensional array, for instance.
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
You don't need extra commasvar array = [,]
is adequate.
– Kaya Toast
Jan 31 '15 at 7:29
add a comment |
up vote
11
down vote
up vote
11
down vote
I'm not sure if anyone has answered this but I found this worked for me pretty well -
var array = [[,],[,]]
eg:
var a = [[1,2],[3,4]]
For a 2 dimensional array, for instance.
I'm not sure if anyone has answered this but I found this worked for me pretty well -
var array = [[,],[,]]
eg:
var a = [[1,2],[3,4]]
For a 2 dimensional array, for instance.
edited Jul 1 '12 at 6:50
Nikson Kanti Paul
2,77412344
2,77412344
answered Jul 1 '12 at 3:28
Uchenna
11912
11912
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
You don't need extra commasvar array = [,]
is adequate.
– Kaya Toast
Jan 31 '15 at 7:29
add a comment |
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
You don't need extra commasvar array = [,]
is adequate.
– Kaya Toast
Jan 31 '15 at 7:29
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
How can I do this dynamically? I want the inner arrays with different sizes.
– alap
Jan 19 '14 at 16:48
3
3
You don't need extra commas
var array = [,]
is adequate.– Kaya Toast
Jan 31 '15 at 7:29
You don't need extra commas
var array = [,]
is adequate.– Kaya Toast
Jan 31 '15 at 7:29
add a comment |
up vote
10
down vote
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns.
function Create2DArray(rows,columns) {
var x = new Array(rows);
for (var i = 0; i < rows; i++) {
x[i] = new Array(columns);
}
return x;
}
to create an Array use this method as below.
var array = Create2DArray(10,20);
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
add a comment |
up vote
10
down vote
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns.
function Create2DArray(rows,columns) {
var x = new Array(rows);
for (var i = 0; i < rows; i++) {
x[i] = new Array(columns);
}
return x;
}
to create an Array use this method as below.
var array = Create2DArray(10,20);
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
add a comment |
up vote
10
down vote
up vote
10
down vote
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns.
function Create2DArray(rows,columns) {
var x = new Array(rows);
for (var i = 0; i < rows; i++) {
x[i] = new Array(columns);
}
return x;
}
to create an Array use this method as below.
var array = Create2DArray(10,20);
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns.
function Create2DArray(rows,columns) {
var x = new Array(rows);
for (var i = 0; i < rows; i++) {
x[i] = new Array(columns);
}
return x;
}
to create an Array use this method as below.
var array = Create2DArray(10,20);
edited Jun 26 '14 at 6:56
answered Jun 25 '14 at 11:38
prime
4,44664073
4,44664073
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
add a comment |
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
2
2
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
Please would you add some explanatory information to your ansdwer showing how it works, and why it solves the problem. This will help others who find this page in the future
– Our Man in Bananas
Jun 25 '14 at 12:16
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
When would you need an Array that is preinitialized with a certain number of colums in Javascript? You can access the n-th element of a array as well.
– domenukk
Jul 8 '14 at 14:49
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
I noticed the function starts with capital C, which (by certain conventions) suggest it would be a Function constructor and you would use it with the new keyword. A very minor and somewhat opinionated maybe, but I would still suggest un-capitalized word.
– Hachi
Aug 24 '14 at 5:53
add a comment |
up vote
8
down vote
Use Array Comprehensions
In JavaScript 1.7 and higher you can use array comprehensions to create two dimensional arrays. You can also filter and/or manipulate the entries while filling the array and don't have to use loops.
var rows = [1, 2, 3];
var cols = ["a", "b", "c", "d"];
var grid = [ for (r of rows) [ for (c of cols) r+c ] ];
/*
grid = [
["1a","1b","1c","1d"],
["2a","2b","2c","2d"],
["3a","3b","3c","3d"]
]
*/
You can create any n x m
array you want and fill it with a default value by calling
var default = 0; // your 2d array will be filled with this value
var n_dim = 2;
var m_dim = 7;
var arr = [ for (n of Array(n_dim)) [ for (m of Array(m_dim) default ]]
/*
arr = [
[0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0],
]
*/
More examples and documentation can be found here.
Please note that this is not a standard feature yet.
A quick google check here... yup... thefor
statement is still a loop...
– Pimp Trizkit
Mar 10 at 15:25
add a comment |
up vote
8
down vote
Use Array Comprehensions
In JavaScript 1.7 and higher you can use array comprehensions to create two dimensional arrays. You can also filter and/or manipulate the entries while filling the array and don't have to use loops.
var rows = [1, 2, 3];
var cols = ["a", "b", "c", "d"];
var grid = [ for (r of rows) [ for (c of cols) r+c ] ];
/*
grid = [
["1a","1b","1c","1d"],
["2a","2b","2c","2d"],
["3a","3b","3c","3d"]
]
*/
You can create any n x m
array you want and fill it with a default value by calling
var default = 0; // your 2d array will be filled with this value
var n_dim = 2;
var m_dim = 7;
var arr = [ for (n of Array(n_dim)) [ for (m of Array(m_dim) default ]]
/*
arr = [
[0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0],
]
*/
More examples and documentation can be found here.
Please note that this is not a standard feature yet.
A quick google check here... yup... thefor
statement is still a loop...
– Pimp Trizkit
Mar 10 at 15:25
add a comment |
up vote
8
down vote
up vote
8
down vote
Use Array Comprehensions
In JavaScript 1.7 and higher you can use array comprehensions to create two dimensional arrays. You can also filter and/or manipulate the entries while filling the array and don't have to use loops.
var rows = [1, 2, 3];
var cols = ["a", "b", "c", "d"];
var grid = [ for (r of rows) [ for (c of cols) r+c ] ];
/*
grid = [
["1a","1b","1c","1d"],
["2a","2b","2c","2d"],
["3a","3b","3c","3d"]
]
*/
You can create any n x m
array you want and fill it with a default value by calling
var default = 0; // your 2d array will be filled with this value
var n_dim = 2;
var m_dim = 7;
var arr = [ for (n of Array(n_dim)) [ for (m of Array(m_dim) default ]]
/*
arr = [
[0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0],
]
*/
More examples and documentation can be found here.
Please note that this is not a standard feature yet.
Use Array Comprehensions
In JavaScript 1.7 and higher you can use array comprehensions to create two dimensional arrays. You can also filter and/or manipulate the entries while filling the array and don't have to use loops.
var rows = [1, 2, 3];
var cols = ["a", "b", "c", "d"];
var grid = [ for (r of rows) [ for (c of cols) r+c ] ];
/*
grid = [
["1a","1b","1c","1d"],
["2a","2b","2c","2d"],
["3a","3b","3c","3d"]
]
*/
You can create any n x m
array you want and fill it with a default value by calling
var default = 0; // your 2d array will be filled with this value
var n_dim = 2;
var m_dim = 7;
var arr = [ for (n of Array(n_dim)) [ for (m of Array(m_dim) default ]]
/*
arr = [
[0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0],
]
*/
More examples and documentation can be found here.
Please note that this is not a standard feature yet.
answered Aug 11 '16 at 17:33
Tim Hallyburton
1,5981225
1,5981225
A quick google check here... yup... thefor
statement is still a loop...
– Pimp Trizkit
Mar 10 at 15:25
add a comment |
A quick google check here... yup... thefor
statement is still a loop...
– Pimp Trizkit
Mar 10 at 15:25
A quick google check here... yup... the
for
statement is still a loop...– Pimp Trizkit
Mar 10 at 15:25
A quick google check here... yup... the
for
statement is still a loop...– Pimp Trizkit
Mar 10 at 15:25
add a comment |
up vote
7
down vote
For one liner lovers Array.from()
// creates 8x8 array filed with "0"
const arr2d = Array.from({ length: 8 }, () => Array.from({ length: 8 }, () => "0"));
Another one (from comment by dmitry_romanov) use Array().fill()
// creates 8x8 array filed with "0"
const arr2d = Array(8).fill(0).map(() => Array(8).fill("0"));
2
we can remove0
in the firstfill()
function:const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
add a comment |
up vote
7
down vote
For one liner lovers Array.from()
// creates 8x8 array filed with "0"
const arr2d = Array.from({ length: 8 }, () => Array.from({ length: 8 }, () => "0"));
Another one (from comment by dmitry_romanov) use Array().fill()
// creates 8x8 array filed with "0"
const arr2d = Array(8).fill(0).map(() => Array(8).fill("0"));
2
we can remove0
in the firstfill()
function:const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
add a comment |
up vote
7
down vote
up vote
7
down vote
For one liner lovers Array.from()
// creates 8x8 array filed with "0"
const arr2d = Array.from({ length: 8 }, () => Array.from({ length: 8 }, () => "0"));
Another one (from comment by dmitry_romanov) use Array().fill()
// creates 8x8 array filed with "0"
const arr2d = Array(8).fill(0).map(() => Array(8).fill("0"));
For one liner lovers Array.from()
// creates 8x8 array filed with "0"
const arr2d = Array.from({ length: 8 }, () => Array.from({ length: 8 }, () => "0"));
Another one (from comment by dmitry_romanov) use Array().fill()
// creates 8x8 array filed with "0"
const arr2d = Array(8).fill(0).map(() => Array(8).fill("0"));
answered Jul 1 '17 at 20:00
my-
9317
9317
2
we can remove0
in the firstfill()
function:const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
add a comment |
2
we can remove0
in the firstfill()
function:const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
2
2
we can remove
0
in the first fill()
function: const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
we can remove
0
in the first fill()
function: const arr2d = Array(8).fill().map(() => Array(8).fill("0"));
– Jinsong Li
Nov 21 '17 at 14:38
add a comment |
up vote
7
down vote
My approach is very similar to @Bineesh answer but with a more general approach.
You can declare the double array as follows:
var myDoubleArray = [];
And the storing and accessing the contents in the following manner:
var testArray1 = [9,8]
var testArray2 = [3,5,7,9,10]
var testArray3 = {"test":123}
var index = 0;
myDoubleArray[index++] = testArray1;
myDoubleArray[index++] = testArray2;
myDoubleArray[index++] = testArray3;
console.log(myDoubleArray[0],myDoubleArray[1][3], myDoubleArray[2]['test'],)
This will print the expected output
[ 9, 8 ] 9 123
add a comment |
up vote
7
down vote
My approach is very similar to @Bineesh answer but with a more general approach.
You can declare the double array as follows:
var myDoubleArray = [];
And the storing and accessing the contents in the following manner:
var testArray1 = [9,8]
var testArray2 = [3,5,7,9,10]
var testArray3 = {"test":123}
var index = 0;
myDoubleArray[index++] = testArray1;
myDoubleArray[index++] = testArray2;
myDoubleArray[index++] = testArray3;
console.log(myDoubleArray[0],myDoubleArray[1][3], myDoubleArray[2]['test'],)
This will print the expected output
[ 9, 8 ] 9 123
add a comment |
up vote
7
down vote
up vote
7
down vote
My approach is very similar to @Bineesh answer but with a more general approach.
You can declare the double array as follows:
var myDoubleArray = [];
And the storing and accessing the contents in the following manner:
var testArray1 = [9,8]
var testArray2 = [3,5,7,9,10]
var testArray3 = {"test":123}
var index = 0;
myDoubleArray[index++] = testArray1;
myDoubleArray[index++] = testArray2;
myDoubleArray[index++] = testArray3;
console.log(myDoubleArray[0],myDoubleArray[1][3], myDoubleArray[2]['test'],)
This will print the expected output
[ 9, 8 ] 9 123
My approach is very similar to @Bineesh answer but with a more general approach.
You can declare the double array as follows:
var myDoubleArray = [];
And the storing and accessing the contents in the following manner:
var testArray1 = [9,8]
var testArray2 = [3,5,7,9,10]
var testArray3 = {"test":123}
var index = 0;
myDoubleArray[index++] = testArray1;
myDoubleArray[index++] = testArray2;
myDoubleArray[index++] = testArray3;
console.log(myDoubleArray[0],myDoubleArray[1][3], myDoubleArray[2]['test'],)
This will print the expected output
[ 9, 8 ] 9 123
edited Jan 14 at 0:25
answered Dec 4 '16 at 4:36
Alexander
196213
196213
add a comment |
add a comment |
up vote
5
down vote
I found below is the simplest way:
var array1 = [];
array1[0][100] = 5;
alert(array1[0][100]);
alert(array1.length);
alert(array1[0].length);
add a comment |
up vote
5
down vote
I found below is the simplest way:
var array1 = [];
array1[0][100] = 5;
alert(array1[0][100]);
alert(array1.length);
alert(array1[0].length);
add a comment |
up vote
5
down vote
up vote
5
down vote
I found below is the simplest way:
var array1 = [];
array1[0][100] = 5;
alert(array1[0][100]);
alert(array1.length);
alert(array1[0].length);
I found below is the simplest way:
var array1 = [];
array1[0][100] = 5;
alert(array1[0][100]);
alert(array1.length);
alert(array1[0].length);
answered Apr 15 '14 at 9:14
GMsoF
3,16495392
3,16495392
add a comment |
add a comment |
up vote
4
down vote
I had to make a flexible array function to add "records" to it as i needed and to be able to update them and do whatever calculations e needed before i sent it to a database for further processing. Here's the code, hope it helps :).
function Add2List(clmn1, clmn2, clmn3) {
aColumns.push(clmn1,clmn2,clmn3); // Creates array with "record"
aLine.splice(aPos, 0,aColumns); // Inserts new "record" at position aPos in main array
aColumns = ; // Resets temporary array
aPos++ // Increments position not to overlap previous "records"
}
Feel free to optimize and / or point out any bugs :)
How about justaLine.push([clmn1, clmn2, clmn3]);
?
– Pimp Trizkit
Mar 10 at 15:55
add a comment |
up vote
4
down vote
I had to make a flexible array function to add "records" to it as i needed and to be able to update them and do whatever calculations e needed before i sent it to a database for further processing. Here's the code, hope it helps :).
function Add2List(clmn1, clmn2, clmn3) {
aColumns.push(clmn1,clmn2,clmn3); // Creates array with "record"
aLine.splice(aPos, 0,aColumns); // Inserts new "record" at position aPos in main array
aColumns = ; // Resets temporary array
aPos++ // Increments position not to overlap previous "records"
}
Feel free to optimize and / or point out any bugs :)
How about justaLine.push([clmn1, clmn2, clmn3]);
?
– Pimp Trizkit
Mar 10 at 15:55
add a comment |
up vote
4
down vote
up vote
4
down vote
I had to make a flexible array function to add "records" to it as i needed and to be able to update them and do whatever calculations e needed before i sent it to a database for further processing. Here's the code, hope it helps :).
function Add2List(clmn1, clmn2, clmn3) {
aColumns.push(clmn1,clmn2,clmn3); // Creates array with "record"
aLine.splice(aPos, 0,aColumns); // Inserts new "record" at position aPos in main array
aColumns = ; // Resets temporary array
aPos++ // Increments position not to overlap previous "records"
}
Feel free to optimize and / or point out any bugs :)
I had to make a flexible array function to add "records" to it as i needed and to be able to update them and do whatever calculations e needed before i sent it to a database for further processing. Here's the code, hope it helps :).
function Add2List(clmn1, clmn2, clmn3) {
aColumns.push(clmn1,clmn2,clmn3); // Creates array with "record"
aLine.splice(aPos, 0,aColumns); // Inserts new "record" at position aPos in main array
aColumns = ; // Resets temporary array
aPos++ // Increments position not to overlap previous "records"
}
Feel free to optimize and / or point out any bugs :)
answered Oct 12 '12 at 10:59


CJ Mendes
159210
159210
How about justaLine.push([clmn1, clmn2, clmn3]);
?
– Pimp Trizkit
Mar 10 at 15:55
add a comment |
How about justaLine.push([clmn1, clmn2, clmn3]);
?
– Pimp Trizkit
Mar 10 at 15:55
How about just
aLine.push([clmn1, clmn2, clmn3]);
?– Pimp Trizkit
Mar 10 at 15:55
How about just
aLine.push([clmn1, clmn2, clmn3]);
?– Pimp Trizkit
Mar 10 at 15:55
add a comment |
up vote
4
down vote
Javascript does not support two dimensional arrays, instead we store an array inside another array and fetch the data from that array depending on what position of that array you want to access. Remember array numeration starts at ZERO.
Code Example:
/* Two dimensional array that's 5 x 5
C0 C1 C2 C3 C4
R0[1][1][1][1][1]
R1[1][1][1][1][1]
R2[1][1][1][1][1]
R3[1][1][1][1][1]
R4[1][1][1][1][1]
*/
var row0 = [1,1,1,1,1],
row1 = [1,1,1,1,1],
row2 = [1,1,1,1,1],
row3 = [1,1,1,1,1],
row4 = [1,1,1,1,1];
var table = [row0,row1,row2,row3,row4];
console.log(table[0][0]); // Get the first item in the array
add a comment |
up vote
4
down vote
Javascript does not support two dimensional arrays, instead we store an array inside another array and fetch the data from that array depending on what position of that array you want to access. Remember array numeration starts at ZERO.
Code Example:
/* Two dimensional array that's 5 x 5
C0 C1 C2 C3 C4
R0[1][1][1][1][1]
R1[1][1][1][1][1]
R2[1][1][1][1][1]
R3[1][1][1][1][1]
R4[1][1][1][1][1]
*/
var row0 = [1,1,1,1,1],
row1 = [1,1,1,1,1],
row2 = [1,1,1,1,1],
row3 = [1,1,1,1,1],
row4 = [1,1,1,1,1];
var table = [row0,row1,row2,row3,row4];
console.log(table[0][0]); // Get the first item in the array
add a comment |
up vote
4
down vote
up vote
4
down vote
Javascript does not support two dimensional arrays, instead we store an array inside another array and fetch the data from that array depending on what position of that array you want to access. Remember array numeration starts at ZERO.
Code Example:
/* Two dimensional array that's 5 x 5
C0 C1 C2 C3 C4
R0[1][1][1][1][1]
R1[1][1][1][1][1]
R2[1][1][1][1][1]
R3[1][1][1][1][1]
R4[1][1][1][1][1]
*/
var row0 = [1,1,1,1,1],
row1 = [1,1,1,1,1],
row2 = [1,1,1,1,1],
row3 = [1,1,1,1,1],
row4 = [1,1,1,1,1];
var table = [row0,row1,row2,row3,row4];
console.log(table[0][0]); // Get the first item in the array
Javascript does not support two dimensional arrays, instead we store an array inside another array and fetch the data from that array depending on what position of that array you want to access. Remember array numeration starts at ZERO.
Code Example:
/* Two dimensional array that's 5 x 5
C0 C1 C2 C3 C4
R0[1][1][1][1][1]
R1[1][1][1][1][1]
R2[1][1][1][1][1]
R3[1][1][1][1][1]
R4[1][1][1][1][1]
*/
var row0 = [1,1,1,1,1],
row1 = [1,1,1,1,1],
row2 = [1,1,1,1,1],
row3 = [1,1,1,1,1],
row4 = [1,1,1,1,1];
var table = [row0,row1,row2,row3,row4];
console.log(table[0][0]); // Get the first item in the array
answered Jul 30 '15 at 23:53
Rick
5,60522831
5,60522831
add a comment |
add a comment |
up vote
4
down vote
Below one, creates a 5x5 matrix and fill them with null
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
3
This answer is wrong. It will create an array with same array filling in its slots.md[1][0] = 3
and all the rest of elements are updated too
– Qiang
Nov 15 '16 at 6:33
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
add a comment |
up vote
4
down vote
Below one, creates a 5x5 matrix and fill them with null
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
3
This answer is wrong. It will create an array with same array filling in its slots.md[1][0] = 3
and all the rest of elements are updated too
– Qiang
Nov 15 '16 at 6:33
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
add a comment |
up vote
4
down vote
up vote
4
down vote
Below one, creates a 5x5 matrix and fill them with null
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
Below one, creates a 5x5 matrix and fill them with null
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
var md = ;
for(var i=0; i<5; i++) {
md.push(new Array(5).fill(null));
}
console.log(md);
edited Aug 29 at 6:53


Javascript Lover - SKT
1,2431525
1,2431525
answered Oct 23 '16 at 5:33
zeah
1255
1255
3
This answer is wrong. It will create an array with same array filling in its slots.md[1][0] = 3
and all the rest of elements are updated too
– Qiang
Nov 15 '16 at 6:33
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
add a comment |
3
This answer is wrong. It will create an array with same array filling in its slots.md[1][0] = 3
and all the rest of elements are updated too
– Qiang
Nov 15 '16 at 6:33
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
3
3
This answer is wrong. It will create an array with same array filling in its slots.
md[1][0] = 3
and all the rest of elements are updated too– Qiang
Nov 15 '16 at 6:33
This answer is wrong. It will create an array with same array filling in its slots.
md[1][0] = 3
and all the rest of elements are updated too– Qiang
Nov 15 '16 at 6:33
1
1
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
@Qiang - yes you are right. Edited my post.
– zeah
Nov 21 '16 at 5:42
add a comment |
up vote
3
down vote
Here's a quick way I've found to make a two dimensional array.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(e => Array(y));
}
You can easily turn this function into an ES5 function as well.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(function(e) {
return Array(y);
});
}
Why this works: the new Array(n)
constructor creates an object with a prototype of Array.prototype
and then assigns the object's length
, resulting in an unpopulated array. Due to its lack of actual members we can't run the Array.prototype.map
function on it.
However, when you provide more than one argument to the constructor, such as when you do Array(1, 2, 3, 4)
, the constructor will use the arguments
object to instantiate and populate an Array
object correctly.
For this reason, we can use Array.apply(null, Array(x))
, because the apply
function will spread the arguments into the constructor. For clarification, doing Array.apply(null, Array(3))
is equivalent to doing Array(null, null, null)
.
Now that we've created an actual populated array, all we need to do is call map
and create the second layer (y
).
add a comment |
up vote
3
down vote
Here's a quick way I've found to make a two dimensional array.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(e => Array(y));
}
You can easily turn this function into an ES5 function as well.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(function(e) {
return Array(y);
});
}
Why this works: the new Array(n)
constructor creates an object with a prototype of Array.prototype
and then assigns the object's length
, resulting in an unpopulated array. Due to its lack of actual members we can't run the Array.prototype.map
function on it.
However, when you provide more than one argument to the constructor, such as when you do Array(1, 2, 3, 4)
, the constructor will use the arguments
object to instantiate and populate an Array
object correctly.
For this reason, we can use Array.apply(null, Array(x))
, because the apply
function will spread the arguments into the constructor. For clarification, doing Array.apply(null, Array(3))
is equivalent to doing Array(null, null, null)
.
Now that we've created an actual populated array, all we need to do is call map
and create the second layer (y
).
add a comment |
up vote
3
down vote
up vote
3
down vote
Here's a quick way I've found to make a two dimensional array.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(e => Array(y));
}
You can easily turn this function into an ES5 function as well.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(function(e) {
return Array(y);
});
}
Why this works: the new Array(n)
constructor creates an object with a prototype of Array.prototype
and then assigns the object's length
, resulting in an unpopulated array. Due to its lack of actual members we can't run the Array.prototype.map
function on it.
However, when you provide more than one argument to the constructor, such as when you do Array(1, 2, 3, 4)
, the constructor will use the arguments
object to instantiate and populate an Array
object correctly.
For this reason, we can use Array.apply(null, Array(x))
, because the apply
function will spread the arguments into the constructor. For clarification, doing Array.apply(null, Array(3))
is equivalent to doing Array(null, null, null)
.
Now that we've created an actual populated array, all we need to do is call map
and create the second layer (y
).
Here's a quick way I've found to make a two dimensional array.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(e => Array(y));
}
You can easily turn this function into an ES5 function as well.
function createArray(x, y) {
return Array.apply(null, Array(x)).map(function(e) {
return Array(y);
});
}
Why this works: the new Array(n)
constructor creates an object with a prototype of Array.prototype
and then assigns the object's length
, resulting in an unpopulated array. Due to its lack of actual members we can't run the Array.prototype.map
function on it.
However, when you provide more than one argument to the constructor, such as when you do Array(1, 2, 3, 4)
, the constructor will use the arguments
object to instantiate and populate an Array
object correctly.
For this reason, we can use Array.apply(null, Array(x))
, because the apply
function will spread the arguments into the constructor. For clarification, doing Array.apply(null, Array(3))
is equivalent to doing Array(null, null, null)
.
Now that we've created an actual populated array, all we need to do is call map
and create the second layer (y
).
answered Mar 25 '16 at 5:56


dimiguel
702724
702724
add a comment |
add a comment |
up vote
3
down vote
To create a non-sparse "2D" array (x,y) with all indices addressable and values set to null:
let 2Darray = new Array(x).fill(null).map(item =>(new Array(y).fill(null)))
bonus "3D" Array (x,y,z)
let 3Darray = new Array(x).fill(null).map(item=>(new Array(y).fill(null)).map(item=>Array(z).fill(null)))
Variations and corrections on this have been mentioned in comments and at various points in response to this question but not as an actual answer so I am adding it here.
It should be noted that (similar to most other answers) this has O(x*y) time complexity so it probably not suitable for very large arrays.
add a comment |
up vote
3
down vote
To create a non-sparse "2D" array (x,y) with all indices addressable and values set to null:
let 2Darray = new Array(x).fill(null).map(item =>(new Array(y).fill(null)))
bonus "3D" Array (x,y,z)
let 3Darray = new Array(x).fill(null).map(item=>(new Array(y).fill(null)).map(item=>Array(z).fill(null)))
Variations and corrections on this have been mentioned in comments and at various points in response to this question but not as an actual answer so I am adding it here.
It should be noted that (similar to most other answers) this has O(x*y) time complexity so it probably not suitable for very large arrays.
add a comment |
up vote
3
down vote
up vote
3
down vote
To create a non-sparse "2D" array (x,y) with all indices addressable and values set to null:
let 2Darray = new Array(x).fill(null).map(item =>(new Array(y).fill(null)))
bonus "3D" Array (x,y,z)
let 3Darray = new Array(x).fill(null).map(item=>(new Array(y).fill(null)).map(item=>Array(z).fill(null)))
Variations and corrections on this have been mentioned in comments and at various points in response to this question but not as an actual answer so I am adding it here.
It should be noted that (similar to most other answers) this has O(x*y) time complexity so it probably not suitable for very large arrays.
To create a non-sparse "2D" array (x,y) with all indices addressable and values set to null:
let 2Darray = new Array(x).fill(null).map(item =>(new Array(y).fill(null)))
bonus "3D" Array (x,y,z)
let 3Darray = new Array(x).fill(null).map(item=>(new Array(y).fill(null)).map(item=>Array(z).fill(null)))
Variations and corrections on this have been mentioned in comments and at various points in response to this question but not as an actual answer so I am adding it here.
It should be noted that (similar to most other answers) this has O(x*y) time complexity so it probably not suitable for very large arrays.
answered Sep 8 at 18:31
Justin Ohms
2,1371932
2,1371932
add a comment |
add a comment |
up vote
2
down vote
You could allocate an array of rows, where each row is an array of the same length. Or you could allocate a one-dimensional array with rows*columns elements and define methods to map row/column coordinates to element indices.
Whichever implementation you pick, if you wrap it in an object you can define the accessor methods in a prototype to make the API easy to use.
add a comment |
up vote
2
down vote
You could allocate an array of rows, where each row is an array of the same length. Or you could allocate a one-dimensional array with rows*columns elements and define methods to map row/column coordinates to element indices.
Whichever implementation you pick, if you wrap it in an object you can define the accessor methods in a prototype to make the API easy to use.
add a comment |
up vote
2
down vote
up vote
2
down vote
You could allocate an array of rows, where each row is an array of the same length. Or you could allocate a one-dimensional array with rows*columns elements and define methods to map row/column coordinates to element indices.
Whichever implementation you pick, if you wrap it in an object you can define the accessor methods in a prototype to make the API easy to use.
You could allocate an array of rows, where each row is an array of the same length. Or you could allocate a one-dimensional array with rows*columns elements and define methods to map row/column coordinates to element indices.
Whichever implementation you pick, if you wrap it in an object you can define the accessor methods in a prototype to make the API easy to use.
answered Jun 8 '09 at 18:32
Nat
8,76032532
8,76032532
add a comment |
add a comment |
up vote
2
down vote
I found that this code works for me:
var map = [
];
mapWidth = 50;
mapHeight = 50;
fillEmptyMap(map, mapWidth, mapHeight);
...
function fillEmptyMap(array, width, height) {
for (var x = 0; x < width; x++) {
array[x] = ;
for (var y = 0; y < height; y++) {
array[x][y] = [0];
}
}
}
add a comment |
up vote
2
down vote
I found that this code works for me:
var map = [
];
mapWidth = 50;
mapHeight = 50;
fillEmptyMap(map, mapWidth, mapHeight);
...
function fillEmptyMap(array, width, height) {
for (var x = 0; x < width; x++) {
array[x] = ;
for (var y = 0; y < height; y++) {
array[x][y] = [0];
}
}
}
add a comment |
up vote
2
down vote
up vote
2
down vote
I found that this code works for me:
var map = [
];
mapWidth = 50;
mapHeight = 50;
fillEmptyMap(map, mapWidth, mapHeight);
...
function fillEmptyMap(array, width, height) {
for (var x = 0; x < width; x++) {
array[x] = ;
for (var y = 0; y < height; y++) {
array[x][y] = [0];
}
}
}
I found that this code works for me:
var map = [
];
mapWidth = 50;
mapHeight = 50;
fillEmptyMap(map, mapWidth, mapHeight);
...
function fillEmptyMap(array, width, height) {
for (var x = 0; x < width; x++) {
array[x] = ;
for (var y = 0; y < height; y++) {
array[x][y] = [0];
}
}
}
answered Jun 14 '14 at 15:46


Fabced
437
437
add a comment |
add a comment |
up vote
2
down vote
A simplified example:
var blocks = ;
blocks[0] = ;
blocks[0][0] = 7;
add a comment |
up vote
2
down vote
A simplified example:
var blocks = ;
blocks[0] = ;
blocks[0][0] = 7;
add a comment |
up vote
2
down vote
up vote
2
down vote
A simplified example:
var blocks = ;
blocks[0] = ;
blocks[0][0] = 7;
A simplified example:
var blocks = ;
blocks[0] = ;
blocks[0][0] = 7;
edited Sep 21 '15 at 7:05


Anders
4,57052951
4,57052951
answered Sep 21 '15 at 5:17


rickatech
42148
42148
add a comment |
add a comment |
up vote
2
down vote
One liner to create a m*n 2 dimensional array filled with 0.
new Array(m).fill(new Array(n).fill(0));
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
Yes, I confirm the gotcha. Quick fix:a = Array(m).fill(0).map(() => Array(n).fill(0))
?map
will untie reference and create unique array per slot.
– dmitry_romanov
Apr 15 '17 at 4:28
add a comment |
up vote
2
down vote
One liner to create a m*n 2 dimensional array filled with 0.
new Array(m).fill(new Array(n).fill(0));
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
Yes, I confirm the gotcha. Quick fix:a = Array(m).fill(0).map(() => Array(n).fill(0))
?map
will untie reference and create unique array per slot.
– dmitry_romanov
Apr 15 '17 at 4:28
add a comment |
up vote
2
down vote
up vote
2
down vote
One liner to create a m*n 2 dimensional array filled with 0.
new Array(m).fill(new Array(n).fill(0));
One liner to create a m*n 2 dimensional array filled with 0.
new Array(m).fill(new Array(n).fill(0));
answered Jan 3 '17 at 1:07
geniuscarrier
3,57911515
3,57911515
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
Yes, I confirm the gotcha. Quick fix:a = Array(m).fill(0).map(() => Array(n).fill(0))
?map
will untie reference and create unique array per slot.
– dmitry_romanov
Apr 15 '17 at 4:28
add a comment |
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
Yes, I confirm the gotcha. Quick fix:a = Array(m).fill(0).map(() => Array(n).fill(0))
?map
will untie reference and create unique array per slot.
– dmitry_romanov
Apr 15 '17 at 4:28
3
3
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
Actually, this will create only two arrays. Second dimensions is going to be the same array in every index.
– Pijusn
Mar 7 '17 at 19:07
6
6
Yes, I confirm the gotcha. Quick fix:
a = Array(m).fill(0).map(() => Array(n).fill(0))
? map
will untie reference and create unique array per slot.– dmitry_romanov
Apr 15 '17 at 4:28
Yes, I confirm the gotcha. Quick fix:
a = Array(m).fill(0).map(() => Array(n).fill(0))
? map
will untie reference and create unique array per slot.– dmitry_romanov
Apr 15 '17 at 4:28
add a comment |
up vote
2
down vote
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
add a comment |
up vote
2
down vote
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
add a comment |
up vote
2
down vote
up vote
2
down vote
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
var playList = [
['I Did It My Way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springsteen'],
['Louie Louie', 'The Kingsmen'],
['Maybellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs( songs ) {
var listHTML = '<ol>';
for ( var i = 0; i < songs.length; i += 1) {
listHTML += '<li>' + songs[i][0] + ' by ' + songs[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
answered Oct 3 '17 at 15:22
antelove
65747
65747
add a comment |
add a comment |
up vote
1
down vote
I've made a modification of Matthew Crumley's answer for creating a multidimensional array function. I've added the dimensions of the array to be passed as array variable and there will be another variable - value
, which will be used to set the values of the elements of the last arrays in the multidimensional array.
/*
* Function to create an n-dimensional array
*
* @param array dimensions
* @param any type value
*
* @return array array
*/
function createArray(dimensions, value) {
// Create new array
var array = new Array(dimensions[0] || 0);
var i = dimensions[0];
// If dimensions array's length is bigger than 1
// we start creating arrays in the array elements with recursions
// to achieve multidimensional array
if (dimensions.length > 1) {
// Remove the first value from the array
var args = Array.prototype.slice.call(dimensions, 1);
// For each index in the created array create a new array with recursion
while(i--) {
array[dimensions[0]-1 - i] = createArray(args, value);
}
// If there is only one element left in the dimensions array
// assign value to each of the new array's elements if value is set as param
} else {
if (typeof value !== 'undefined') {
while(i--) {
array[dimensions[0]-1 - i] = value;
}
}
}
return array;
}
createArray(); // or new Array()
createArray([2], 'empty'); // ['empty', 'empty']
createArray([3, 2], 0); // [[0, 0],
// [0, 0],
// [0, 0]]
add a comment |
up vote
1
down vote
I've made a modification of Matthew Crumley's answer for creating a multidimensional array function. I've added the dimensions of the array to be passed as array variable and there will be another variable - value
, which will be used to set the values of the elements of the last arrays in the multidimensional array.
/*
* Function to create an n-dimensional array
*
* @param array dimensions
* @param any type value
*
* @return array array
*/
function createArray(dimensions, value) {
// Create new array
var array = new Array(dimensions[0] || 0);
var i = dimensions[0];
// If dimensions array's length is bigger than 1
// we start creating arrays in the array elements with recursions
// to achieve multidimensional array
if (dimensions.length > 1) {
// Remove the first value from the array
var args = Array.prototype.slice.call(dimensions, 1);
// For each index in the created array create a new array with recursion
while(i--) {
array[dimensions[0]-1 - i] = createArray(args, value);
}
// If there is only one element left in the dimensions array
// assign value to each of the new array's elements if value is set as param
} else {
if (typeof value !== 'undefined') {
while(i--) {
array[dimensions[0]-1 - i] = value;
}
}
}
return array;
}
createArray(); // or new Array()
createArray([2], 'empty'); // ['empty', 'empty']
createArray([3, 2], 0); // [[0, 0],
// [0, 0],
// [0, 0]]
add a comment |
up vote
1
down vote
up vote
1
down vote
I've made a modification of Matthew Crumley's answer for creating a multidimensional array function. I've added the dimensions of the array to be passed as array variable and there will be another variable - value
, which will be used to set the values of the elements of the last arrays in the multidimensional array.
/*
* Function to create an n-dimensional array
*
* @param array dimensions
* @param any type value
*
* @return array array
*/
function createArray(dimensions, value) {
// Create new array
var array = new Array(dimensions[0] || 0);
var i = dimensions[0];
// If dimensions array's length is bigger than 1
// we start creating arrays in the array elements with recursions
// to achieve multidimensional array
if (dimensions.length > 1) {
// Remove the first value from the array
var args = Array.prototype.slice.call(dimensions, 1);
// For each index in the created array create a new array with recursion
while(i--) {
array[dimensions[0]-1 - i] = createArray(args, value);
}
// If there is only one element left in the dimensions array
// assign value to each of the new array's elements if value is set as param
} else {
if (typeof value !== 'undefined') {
while(i--) {
array[dimensions[0]-1 - i] = value;
}
}
}
return array;
}
createArray(); // or new Array()
createArray([2], 'empty'); // ['empty', 'empty']
createArray([3, 2], 0); // [[0, 0],
// [0, 0],
// [0, 0]]
I've made a modification of Matthew Crumley's answer for creating a multidimensional array function. I've added the dimensions of the array to be passed as array variable and there will be another variable - value
, which will be used to set the values of the elements of the last arrays in the multidimensional array.
/*
* Function to create an n-dimensional array
*
* @param array dimensions
* @param any type value
*
* @return array array
*/
function createArray(dimensions, value) {
// Create new array
var array = new Array(dimensions[0] || 0);
var i = dimensions[0];
// If dimensions array's length is bigger than 1
// we start creating arrays in the array elements with recursions
// to achieve multidimensional array
if (dimensions.length > 1) {
// Remove the first value from the array
var args = Array.prototype.slice.call(dimensions, 1);
// For each index in the created array create a new array with recursion
while(i--) {
array[dimensions[0]-1 - i] = createArray(args, value);
}
// If there is only one element left in the dimensions array
// assign value to each of the new array's elements if value is set as param
} else {
if (typeof value !== 'undefined') {
while(i--) {
array[dimensions[0]-1 - i] = value;
}
}
}
return array;
}
createArray(); // or new Array()
createArray([2], 'empty'); // ['empty', 'empty']
createArray([3, 2], 0); // [[0, 0],
// [0, 0],
// [0, 0]]
edited May 23 '17 at 12:03
Community♦
11
11
answered Feb 20 '15 at 9:31


Hristo Enev
1,544919
1,544919
add a comment |
add a comment |
1 2
next
protected by Mysticial Jul 24 '14 at 5:56
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
usn5wWW6D,W
13
Assuming a somewhat pedantic definition, it is technically impossible to create a 2d array in javascript. But you can create an array of arrays, which is tantamount to the same.
– I. J. Kennedy
Jul 29 '14 at 5:05
Duplicate of - stackoverflow.com/q/6495187/104380
– vsync
Mar 26 '16 at 16:55
For a 5x3 2D array I would do like
var arr2D = new Array(5).fill(new Array(3));
besides if you don't want the cells to be "undefined" you can do likevar arr2D = new Array(5).fill(new Array(3).fill("hey"));
– Redu
May 12 '16 at 16:25
4
FYI... when you fill an array with more arrays using
var arr2D = new Array(5).fill(new Array(3));
, each element of Array(5) will point to the same Array(3). So it's best to use a for loop to dynamically populate sub arrays.– Josh Stribling
May 23 '16 at 8:51
22
a = Array(5).fill(0).map(x => Array(10).fill(0))
– Longfei Wu
Mar 25 '17 at 14:21