Django POST request not working correctly
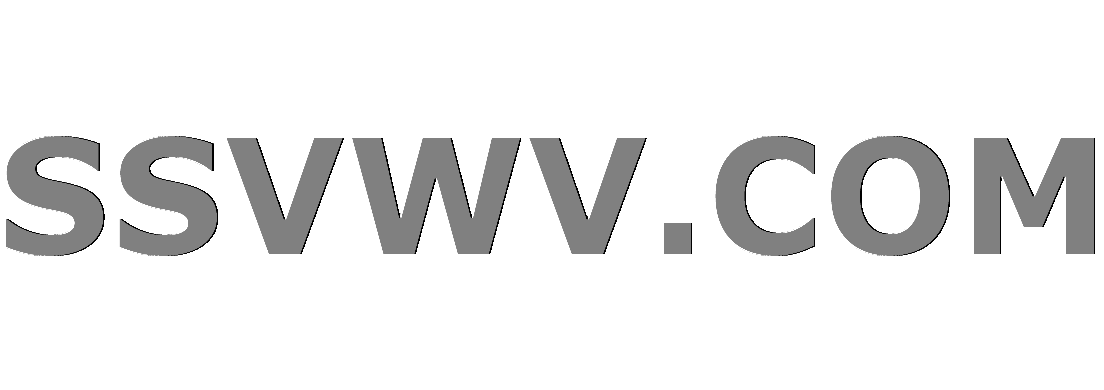
Multi tool use
I have a Django Project I am currently working on where I want to be able to add a product to a database. I have a few fields where the user enters the details of the product and this is then sent to the create function and should save it to the Database. Unfortunately this isnt happening. This is my first use of Django so I'm not sure whats going wrong here. Here is the code I have so far:
My Index.html page:
<!DOCTYPE html>
<html>
<body>
<h2>Create product here</h2>
<div>
<form id="new_user_form" method="post">
{% csrf_token %}
<div>
<label for="name" > Name:<br></label>
<input type="text" id="name"/>
</div>
<br/>
<div>
<label for="description"> description:<br></label>
<input type="text" id="description"/>
</div>
<div>
<label for="price" > price:<br></label>
<input type="text" id="price"/>
</div>
<div>
<input type="submit" value="submit"/>
</div>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type = "text/text/javascript">
$(document).on('submit', '#new_user_form', function(e)){
e.preventDefault()
$.ajax({
type: 'POST',
url:'/user/create',
data:{
name:$('#name').val(),
description:$('#description').val(),
price:$('#price').val(),
}
success.function(){
console.log('created')
}
})
}
</script>
</html>
Here is my urls.py code:
from django.contrib import admin
from django.urls import path
from django.conf.urls import include, url
from testapp import views
admin.autodiscover()
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index),
path('user/create', views.create_product, name='create_user')
]
My views.py code:
from django.shortcuts import render
from testapp.models import User
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
def index(request):
return render(request, 'index.html')
@csrf_exempt
def create_product(request):
if request.method == 'POST':
name = request.POST['name']
description = request.POST['description']
price = request.POST['price']
User.objects.create(
name = name,
description = description,
price = price
)
return HttpResponse('')
And the models.py code:
from django.db import models
# Create your models here.
class User(models.Model):
name = models.CharField(max_length = 32)
desscription = models.TextField()
price = models.CharField(max_length = 128)
The problem with this is the POST request is sent when run, but the data is not saved to the database and I can't figure out why. I have followed a couple tutorials and documentation to get to this stage but can't work out what it is that's not working correctly here. Can someone have a look and let me know please?
python ajax django post request
add a comment |
I have a Django Project I am currently working on where I want to be able to add a product to a database. I have a few fields where the user enters the details of the product and this is then sent to the create function and should save it to the Database. Unfortunately this isnt happening. This is my first use of Django so I'm not sure whats going wrong here. Here is the code I have so far:
My Index.html page:
<!DOCTYPE html>
<html>
<body>
<h2>Create product here</h2>
<div>
<form id="new_user_form" method="post">
{% csrf_token %}
<div>
<label for="name" > Name:<br></label>
<input type="text" id="name"/>
</div>
<br/>
<div>
<label for="description"> description:<br></label>
<input type="text" id="description"/>
</div>
<div>
<label for="price" > price:<br></label>
<input type="text" id="price"/>
</div>
<div>
<input type="submit" value="submit"/>
</div>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type = "text/text/javascript">
$(document).on('submit', '#new_user_form', function(e)){
e.preventDefault()
$.ajax({
type: 'POST',
url:'/user/create',
data:{
name:$('#name').val(),
description:$('#description').val(),
price:$('#price').val(),
}
success.function(){
console.log('created')
}
})
}
</script>
</html>
Here is my urls.py code:
from django.contrib import admin
from django.urls import path
from django.conf.urls import include, url
from testapp import views
admin.autodiscover()
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index),
path('user/create', views.create_product, name='create_user')
]
My views.py code:
from django.shortcuts import render
from testapp.models import User
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
def index(request):
return render(request, 'index.html')
@csrf_exempt
def create_product(request):
if request.method == 'POST':
name = request.POST['name']
description = request.POST['description']
price = request.POST['price']
User.objects.create(
name = name,
description = description,
price = price
)
return HttpResponse('')
And the models.py code:
from django.db import models
# Create your models here.
class User(models.Model):
name = models.CharField(max_length = 32)
desscription = models.TextField()
price = models.CharField(max_length = 128)
The problem with this is the POST request is sent when run, but the data is not saved to the database and I can't figure out why. I have followed a couple tutorials and documentation to get to this stage but can't work out what it is that's not working correctly here. Can someone have a look and let me know please?
python ajax django post request
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do<form action="/user/create" ...>
?
– Daniel Roseman
Nov 11 at 15:21
add a comment |
I have a Django Project I am currently working on where I want to be able to add a product to a database. I have a few fields where the user enters the details of the product and this is then sent to the create function and should save it to the Database. Unfortunately this isnt happening. This is my first use of Django so I'm not sure whats going wrong here. Here is the code I have so far:
My Index.html page:
<!DOCTYPE html>
<html>
<body>
<h2>Create product here</h2>
<div>
<form id="new_user_form" method="post">
{% csrf_token %}
<div>
<label for="name" > Name:<br></label>
<input type="text" id="name"/>
</div>
<br/>
<div>
<label for="description"> description:<br></label>
<input type="text" id="description"/>
</div>
<div>
<label for="price" > price:<br></label>
<input type="text" id="price"/>
</div>
<div>
<input type="submit" value="submit"/>
</div>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type = "text/text/javascript">
$(document).on('submit', '#new_user_form', function(e)){
e.preventDefault()
$.ajax({
type: 'POST',
url:'/user/create',
data:{
name:$('#name').val(),
description:$('#description').val(),
price:$('#price').val(),
}
success.function(){
console.log('created')
}
})
}
</script>
</html>
Here is my urls.py code:
from django.contrib import admin
from django.urls import path
from django.conf.urls import include, url
from testapp import views
admin.autodiscover()
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index),
path('user/create', views.create_product, name='create_user')
]
My views.py code:
from django.shortcuts import render
from testapp.models import User
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
def index(request):
return render(request, 'index.html')
@csrf_exempt
def create_product(request):
if request.method == 'POST':
name = request.POST['name']
description = request.POST['description']
price = request.POST['price']
User.objects.create(
name = name,
description = description,
price = price
)
return HttpResponse('')
And the models.py code:
from django.db import models
# Create your models here.
class User(models.Model):
name = models.CharField(max_length = 32)
desscription = models.TextField()
price = models.CharField(max_length = 128)
The problem with this is the POST request is sent when run, but the data is not saved to the database and I can't figure out why. I have followed a couple tutorials and documentation to get to this stage but can't work out what it is that's not working correctly here. Can someone have a look and let me know please?
python ajax django post request
I have a Django Project I am currently working on where I want to be able to add a product to a database. I have a few fields where the user enters the details of the product and this is then sent to the create function and should save it to the Database. Unfortunately this isnt happening. This is my first use of Django so I'm not sure whats going wrong here. Here is the code I have so far:
My Index.html page:
<!DOCTYPE html>
<html>
<body>
<h2>Create product here</h2>
<div>
<form id="new_user_form" method="post">
{% csrf_token %}
<div>
<label for="name" > Name:<br></label>
<input type="text" id="name"/>
</div>
<br/>
<div>
<label for="description"> description:<br></label>
<input type="text" id="description"/>
</div>
<div>
<label for="price" > price:<br></label>
<input type="text" id="price"/>
</div>
<div>
<input type="submit" value="submit"/>
</div>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type = "text/text/javascript">
$(document).on('submit', '#new_user_form', function(e)){
e.preventDefault()
$.ajax({
type: 'POST',
url:'/user/create',
data:{
name:$('#name').val(),
description:$('#description').val(),
price:$('#price').val(),
}
success.function(){
console.log('created')
}
})
}
</script>
</html>
Here is my urls.py code:
from django.contrib import admin
from django.urls import path
from django.conf.urls import include, url
from testapp import views
admin.autodiscover()
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.index),
path('user/create', views.create_product, name='create_user')
]
My views.py code:
from django.shortcuts import render
from testapp.models import User
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
def index(request):
return render(request, 'index.html')
@csrf_exempt
def create_product(request):
if request.method == 'POST':
name = request.POST['name']
description = request.POST['description']
price = request.POST['price']
User.objects.create(
name = name,
description = description,
price = price
)
return HttpResponse('')
And the models.py code:
from django.db import models
# Create your models here.
class User(models.Model):
name = models.CharField(max_length = 32)
desscription = models.TextField()
price = models.CharField(max_length = 128)
The problem with this is the POST request is sent when run, but the data is not saved to the database and I can't figure out why. I have followed a couple tutorials and documentation to get to this stage but can't work out what it is that's not working correctly here. Can someone have a look and let me know please?
python ajax django post request
python ajax django post request
asked Nov 11 at 13:29
Arsenalfan
135
135
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do<form action="/user/create" ...>
?
– Daniel Roseman
Nov 11 at 15:21
add a comment |
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do<form action="/user/create" ...>
?
– Daniel Roseman
Nov 11 at 15:21
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do
<form action="/user/create" ...>
?– Daniel Roseman
Nov 11 at 15:21
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do
<form action="/user/create" ...>
?– Daniel Roseman
Nov 11 at 15:21
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249211%2fdjango-post-request-not-working-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249211%2fdjango-post-request-not-working-correctly%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5KlA8ZyZAIt1gqcN,sUGB65fFjv9Cq4D8xaSyNc5MD8qAZ
When you print the values of name, description, and price (in the POST routine), do they have data in them?
– Jonah Bishop
Nov 11 at 13:30
You've got a few things going on here, you should try and simplify to eliminate the possibilities. For instance, does it work if you take away the JS and do a simple form submission without Ajax?
– Daniel Roseman
Nov 11 at 13:32
@JonahBishop no, tried adding a console.log function in the JavaScript but nothing is printed to the console.
– Arsenalfan
Nov 11 at 13:49
@DanielRoseman still sends a post request, but again that data isnt going anywhere
– Arsenalfan
Nov 11 at 13:52
Well, you don't have an action attribute on the form so you're posting back to the index. What if you do
<form action="/user/create" ...>
?– Daniel Roseman
Nov 11 at 15:21