Howcan I start @Scheduled method with concrete scheduler?
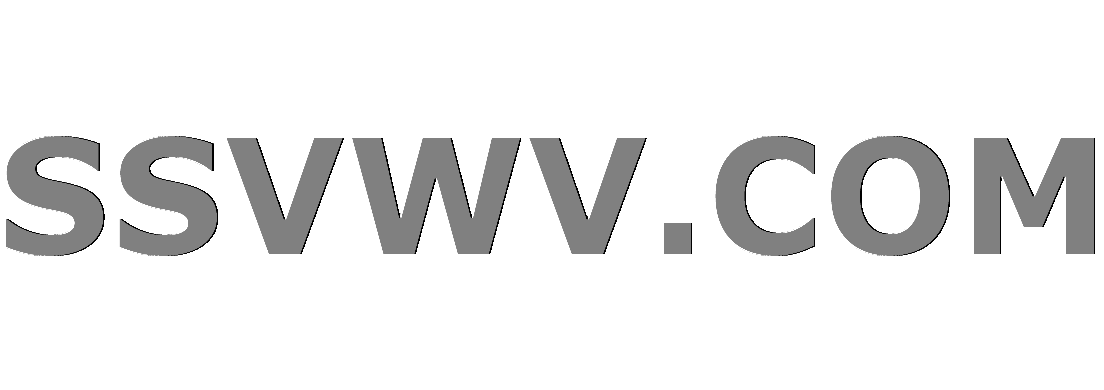
Multi tool use
I have scheduler:
@Bean("one")
ThreadPoolTaskScheduler taskScheduler(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(5);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test-");
return threadPoolTaskScheduler;
}
@Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
And method:
@Scheduled(fixedRate = 1000L)
public void test() {
And Second method:
@Scheduled(fixedRate = 1000L)
public void test2() {
How can I configure each @Scheduled
method with concrete scheduler?
If I implement it like this:
@Slf4j
@Component
public class MyScheduler {
private final ThreadPoolTaskScheduler taskSchedulerFirst;
private final ThreadPoolTaskScheduler taskSchedulerSecond;
private final TestBean testBean;
public MyScheduler(@Qualifier("first") ThreadPoolTaskScheduler taskSchedulerFirst, @Qualifier("second")ThreadPoolTaskScheduler taskSchedulerSecond, TestBean testBean) {
this.taskSchedulerFirst = taskSchedulerFirst;
this.taskSchedulerSecond = taskSchedulerSecond;
this.testBean = testBean;
}
@PostConstruct
public void test() {
taskSchedulerFirst.scheduleAtFixedRate(testBean::test, 1000L);
taskSchedulerSecond.scheduleAtFixedRate(testBean::test2, 1000L);
}
Bouth schedulers not used and used TaskExecutor:
2018-09-05 11:10:30.812 INFO 10724 --- [TaskExecutor-41] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.747 INFO 10724 --- [TaskExecutor-43] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.748 INFO 10724 --- [TaskExecutor-46] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:32.747 INFO 10724 --- [TaskExecutor-45] com.example.scheduling.TestBean : hz
2018-09-05 11:10:32.748 INFO 10724 --- [TaskExecutor-48] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:33.747 INFO 10724 --- [TaskExecutor-47]
But used TaskExecutor
why?
java spring scheduler
add a comment |
I have scheduler:
@Bean("one")
ThreadPoolTaskScheduler taskScheduler(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(5);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test-");
return threadPoolTaskScheduler;
}
@Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
And method:
@Scheduled(fixedRate = 1000L)
public void test() {
And Second method:
@Scheduled(fixedRate = 1000L)
public void test2() {
How can I configure each @Scheduled
method with concrete scheduler?
If I implement it like this:
@Slf4j
@Component
public class MyScheduler {
private final ThreadPoolTaskScheduler taskSchedulerFirst;
private final ThreadPoolTaskScheduler taskSchedulerSecond;
private final TestBean testBean;
public MyScheduler(@Qualifier("first") ThreadPoolTaskScheduler taskSchedulerFirst, @Qualifier("second")ThreadPoolTaskScheduler taskSchedulerSecond, TestBean testBean) {
this.taskSchedulerFirst = taskSchedulerFirst;
this.taskSchedulerSecond = taskSchedulerSecond;
this.testBean = testBean;
}
@PostConstruct
public void test() {
taskSchedulerFirst.scheduleAtFixedRate(testBean::test, 1000L);
taskSchedulerSecond.scheduleAtFixedRate(testBean::test2, 1000L);
}
Bouth schedulers not used and used TaskExecutor:
2018-09-05 11:10:30.812 INFO 10724 --- [TaskExecutor-41] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.747 INFO 10724 --- [TaskExecutor-43] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.748 INFO 10724 --- [TaskExecutor-46] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:32.747 INFO 10724 --- [TaskExecutor-45] com.example.scheduling.TestBean : hz
2018-09-05 11:10:32.748 INFO 10724 --- [TaskExecutor-48] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:33.747 INFO 10724 --- [TaskExecutor-47]
But used TaskExecutor
why?
java spring scheduler
Did you remove the@Scheduled
annotation from the bean methods after your edit?
– dpr
Sep 5 at 8:49
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
You should not need the@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.
– dpr
Sep 5 at 9:23
add a comment |
I have scheduler:
@Bean("one")
ThreadPoolTaskScheduler taskScheduler(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(5);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test-");
return threadPoolTaskScheduler;
}
@Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
And method:
@Scheduled(fixedRate = 1000L)
public void test() {
And Second method:
@Scheduled(fixedRate = 1000L)
public void test2() {
How can I configure each @Scheduled
method with concrete scheduler?
If I implement it like this:
@Slf4j
@Component
public class MyScheduler {
private final ThreadPoolTaskScheduler taskSchedulerFirst;
private final ThreadPoolTaskScheduler taskSchedulerSecond;
private final TestBean testBean;
public MyScheduler(@Qualifier("first") ThreadPoolTaskScheduler taskSchedulerFirst, @Qualifier("second")ThreadPoolTaskScheduler taskSchedulerSecond, TestBean testBean) {
this.taskSchedulerFirst = taskSchedulerFirst;
this.taskSchedulerSecond = taskSchedulerSecond;
this.testBean = testBean;
}
@PostConstruct
public void test() {
taskSchedulerFirst.scheduleAtFixedRate(testBean::test, 1000L);
taskSchedulerSecond.scheduleAtFixedRate(testBean::test2, 1000L);
}
Bouth schedulers not used and used TaskExecutor:
2018-09-05 11:10:30.812 INFO 10724 --- [TaskExecutor-41] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.747 INFO 10724 --- [TaskExecutor-43] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.748 INFO 10724 --- [TaskExecutor-46] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:32.747 INFO 10724 --- [TaskExecutor-45] com.example.scheduling.TestBean : hz
2018-09-05 11:10:32.748 INFO 10724 --- [TaskExecutor-48] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:33.747 INFO 10724 --- [TaskExecutor-47]
But used TaskExecutor
why?
java spring scheduler
I have scheduler:
@Bean("one")
ThreadPoolTaskScheduler taskScheduler(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(5);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test-");
return threadPoolTaskScheduler;
}
@Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
And method:
@Scheduled(fixedRate = 1000L)
public void test() {
And Second method:
@Scheduled(fixedRate = 1000L)
public void test2() {
How can I configure each @Scheduled
method with concrete scheduler?
If I implement it like this:
@Slf4j
@Component
public class MyScheduler {
private final ThreadPoolTaskScheduler taskSchedulerFirst;
private final ThreadPoolTaskScheduler taskSchedulerSecond;
private final TestBean testBean;
public MyScheduler(@Qualifier("first") ThreadPoolTaskScheduler taskSchedulerFirst, @Qualifier("second")ThreadPoolTaskScheduler taskSchedulerSecond, TestBean testBean) {
this.taskSchedulerFirst = taskSchedulerFirst;
this.taskSchedulerSecond = taskSchedulerSecond;
this.testBean = testBean;
}
@PostConstruct
public void test() {
taskSchedulerFirst.scheduleAtFixedRate(testBean::test, 1000L);
taskSchedulerSecond.scheduleAtFixedRate(testBean::test2, 1000L);
}
Bouth schedulers not used and used TaskExecutor:
2018-09-05 11:10:30.812 INFO 10724 --- [TaskExecutor-41] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.747 INFO 10724 --- [TaskExecutor-43] com.example.scheduling.TestBean : hz
2018-09-05 11:10:31.748 INFO 10724 --- [TaskExecutor-46] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:32.747 INFO 10724 --- [TaskExecutor-45] com.example.scheduling.TestBean : hz
2018-09-05 11:10:32.748 INFO 10724 --- [TaskExecutor-48] com.example.scheduling.TestBean : hz2
2018-09-05 11:10:33.747 INFO 10724 --- [TaskExecutor-47]
But used TaskExecutor
why?
java spring scheduler
java spring scheduler
edited Nov 11 at 13:23
Cœur
17.3k9102143
17.3k9102143
asked Sep 5 at 7:59
ip696
1,14111135
1,14111135
Did you remove the@Scheduled
annotation from the bean methods after your edit?
– dpr
Sep 5 at 8:49
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
You should not need the@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.
– dpr
Sep 5 at 9:23
add a comment |
Did you remove the@Scheduled
annotation from the bean methods after your edit?
– dpr
Sep 5 at 8:49
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
You should not need the@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.
– dpr
Sep 5 at 9:23
Did you remove the
@Scheduled
annotation from the bean methods after your edit?– dpr
Sep 5 at 8:49
Did you remove the
@Scheduled
annotation from the bean methods after your edit?– dpr
Sep 5 at 8:49
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
You should not need the
@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.– dpr
Sep 5 at 9:23
You should not need the
@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.– dpr
Sep 5 at 9:23
add a comment |
3 Answers
3
active
oldest
votes
TL;DR No
According to Spring scheduling they are different implementations of TaskScheduler abstraction
ThreadPoolTaskScheduler as implementation:
ThreadPoolTaskScheduler, can be used whenever external thread management is not a requirement. Internally, it delegates to a ScheduledExecutorService instance. ThreadPoolTaskScheduler actually implements Spring's TaskExecutor interface as well, so that a single instance can be used for asynchronous execution as soon as possible as well as scheduled, and potentially recurring, executions.
@Scheduled as annotation support for task scheduling
The @Scheduled annotation can be added to a method along with trigger metadata.
See also answer for best way to schedule task , most voted:
The simplest way to schedule tasks in Spring is to create method annotated by @Scheduled in spring managed bean.
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
add a comment |
When using the @Scheduled
annotation there is no out-of-the-box support to use different thread pools for different beans. You can configure the thread pool to be used by implementing SchedulingConfigurer
in your @Configuration
class.
I think the implementation after your edit should work. You probably only need to call threadPoolTaskScheduler.initialize()
directly after creating the scheduler like this:
Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.initialize(); // initialize scheduler
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
This will create the scheduler's internal executor, that is used the actually execute stuff.
add a comment |
You need to implement the SchedulingConfigurer
in config class and override it's configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar)
method. In the overridden method register your ThreadPoolTaskScheduler
:
@Override
public void configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar) {
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
...
scheduledTaskRegistrar.setTaskScheduler(threadPoolTaskScheduler);
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52180040%2fhowcan-i-start-scheduled-method-with-concrete-scheduler%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
TL;DR No
According to Spring scheduling they are different implementations of TaskScheduler abstraction
ThreadPoolTaskScheduler as implementation:
ThreadPoolTaskScheduler, can be used whenever external thread management is not a requirement. Internally, it delegates to a ScheduledExecutorService instance. ThreadPoolTaskScheduler actually implements Spring's TaskExecutor interface as well, so that a single instance can be used for asynchronous execution as soon as possible as well as scheduled, and potentially recurring, executions.
@Scheduled as annotation support for task scheduling
The @Scheduled annotation can be added to a method along with trigger metadata.
See also answer for best way to schedule task , most voted:
The simplest way to schedule tasks in Spring is to create method annotated by @Scheduled in spring managed bean.
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
add a comment |
TL;DR No
According to Spring scheduling they are different implementations of TaskScheduler abstraction
ThreadPoolTaskScheduler as implementation:
ThreadPoolTaskScheduler, can be used whenever external thread management is not a requirement. Internally, it delegates to a ScheduledExecutorService instance. ThreadPoolTaskScheduler actually implements Spring's TaskExecutor interface as well, so that a single instance can be used for asynchronous execution as soon as possible as well as scheduled, and potentially recurring, executions.
@Scheduled as annotation support for task scheduling
The @Scheduled annotation can be added to a method along with trigger metadata.
See also answer for best way to schedule task , most voted:
The simplest way to schedule tasks in Spring is to create method annotated by @Scheduled in spring managed bean.
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
add a comment |
TL;DR No
According to Spring scheduling they are different implementations of TaskScheduler abstraction
ThreadPoolTaskScheduler as implementation:
ThreadPoolTaskScheduler, can be used whenever external thread management is not a requirement. Internally, it delegates to a ScheduledExecutorService instance. ThreadPoolTaskScheduler actually implements Spring's TaskExecutor interface as well, so that a single instance can be used for asynchronous execution as soon as possible as well as scheduled, and potentially recurring, executions.
@Scheduled as annotation support for task scheduling
The @Scheduled annotation can be added to a method along with trigger metadata.
See also answer for best way to schedule task , most voted:
The simplest way to schedule tasks in Spring is to create method annotated by @Scheduled in spring managed bean.
TL;DR No
According to Spring scheduling they are different implementations of TaskScheduler abstraction
ThreadPoolTaskScheduler as implementation:
ThreadPoolTaskScheduler, can be used whenever external thread management is not a requirement. Internally, it delegates to a ScheduledExecutorService instance. ThreadPoolTaskScheduler actually implements Spring's TaskExecutor interface as well, so that a single instance can be used for asynchronous execution as soon as possible as well as scheduled, and potentially recurring, executions.
@Scheduled as annotation support for task scheduling
The @Scheduled annotation can be added to a method along with trigger metadata.
See also answer for best way to schedule task , most voted:
The simplest way to schedule tasks in Spring is to create method annotated by @Scheduled in spring managed bean.
edited Sep 5 at 8:18
answered Sep 5 at 8:10


user7294900
20.4k103258
20.4k103258
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
add a comment |
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
I did not understand anything from the answer. somehow all smeared. Can I set concrete tasckScheduler to method with @Scheduled annotation or not?
– ip696
Sep 5 at 8:16
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
@ip696 TL;DR No, they are different implementation of scheduling
– user7294900
Sep 5 at 8:17
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
but if I configure 1 tasckScheduler and one @Scheduled method - this method use this tasckScheduler.
– ip696
Sep 5 at 8:23
add a comment |
When using the @Scheduled
annotation there is no out-of-the-box support to use different thread pools for different beans. You can configure the thread pool to be used by implementing SchedulingConfigurer
in your @Configuration
class.
I think the implementation after your edit should work. You probably only need to call threadPoolTaskScheduler.initialize()
directly after creating the scheduler like this:
Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.initialize(); // initialize scheduler
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
This will create the scheduler's internal executor, that is used the actually execute stuff.
add a comment |
When using the @Scheduled
annotation there is no out-of-the-box support to use different thread pools for different beans. You can configure the thread pool to be used by implementing SchedulingConfigurer
in your @Configuration
class.
I think the implementation after your edit should work. You probably only need to call threadPoolTaskScheduler.initialize()
directly after creating the scheduler like this:
Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.initialize(); // initialize scheduler
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
This will create the scheduler's internal executor, that is used the actually execute stuff.
add a comment |
When using the @Scheduled
annotation there is no out-of-the-box support to use different thread pools for different beans. You can configure the thread pool to be used by implementing SchedulingConfigurer
in your @Configuration
class.
I think the implementation after your edit should work. You probably only need to call threadPoolTaskScheduler.initialize()
directly after creating the scheduler like this:
Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.initialize(); // initialize scheduler
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
This will create the scheduler's internal executor, that is used the actually execute stuff.
When using the @Scheduled
annotation there is no out-of-the-box support to use different thread pools for different beans. You can configure the thread pool to be used by implementing SchedulingConfigurer
in your @Configuration
class.
I think the implementation after your edit should work. You probably only need to call threadPoolTaskScheduler.initialize()
directly after creating the scheduler like this:
Bean("two")
ThreadPoolTaskScheduler taskScheduler2(){
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
threadPoolTaskScheduler.initialize(); // initialize scheduler
threadPoolTaskScheduler.setPoolSize(50);
threadPoolTaskScheduler.setAwaitTerminationSeconds(60);
threadPoolTaskScheduler.setThreadNamePrefix("Test2-");
return threadPoolTaskScheduler;
}
This will create the scheduler's internal executor, that is used the actually execute stuff.
answered Sep 5 at 9:21


dpr
4,39611644
4,39611644
add a comment |
add a comment |
You need to implement the SchedulingConfigurer
in config class and override it's configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar)
method. In the overridden method register your ThreadPoolTaskScheduler
:
@Override
public void configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar) {
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
...
scheduledTaskRegistrar.setTaskScheduler(threadPoolTaskScheduler);
}
add a comment |
You need to implement the SchedulingConfigurer
in config class and override it's configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar)
method. In the overridden method register your ThreadPoolTaskScheduler
:
@Override
public void configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar) {
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
...
scheduledTaskRegistrar.setTaskScheduler(threadPoolTaskScheduler);
}
add a comment |
You need to implement the SchedulingConfigurer
in config class and override it's configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar)
method. In the overridden method register your ThreadPoolTaskScheduler
:
@Override
public void configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar) {
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
...
scheduledTaskRegistrar.setTaskScheduler(threadPoolTaskScheduler);
}
You need to implement the SchedulingConfigurer
in config class and override it's configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar)
method. In the overridden method register your ThreadPoolTaskScheduler
:
@Override
public void configureTasks(ScheduledTaskRegistrar scheduledTaskRegistrar) {
ThreadPoolTaskScheduler threadPoolTaskScheduler = new ThreadPoolTaskScheduler();
...
scheduledTaskRegistrar.setTaskScheduler(threadPoolTaskScheduler);
}
answered Sep 5 at 9:13
Rohit
1,64211019
1,64211019
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52180040%2fhowcan-i-start-scheduled-method-with-concrete-scheduler%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
05quGc9T fevJylwkBxV Il,sI4A7R,2whmh2CLNO 1,L 6n,YNIaPOSx MxiOyBo26obKV5aiHjC
Did you remove the
@Scheduled
annotation from the bean methods after your edit?– dpr
Sep 5 at 8:49
bean methods after not @Scheduled befour and after edit. Only Async
– ip696
Sep 5 at 9:11
You should not need the
@Async
annotation. This will make spring use a different thread pool for execution. Simply remove this annotation an you should be fine.– dpr
Sep 5 at 9:23