How to match the name and show details from firebase in another java file?
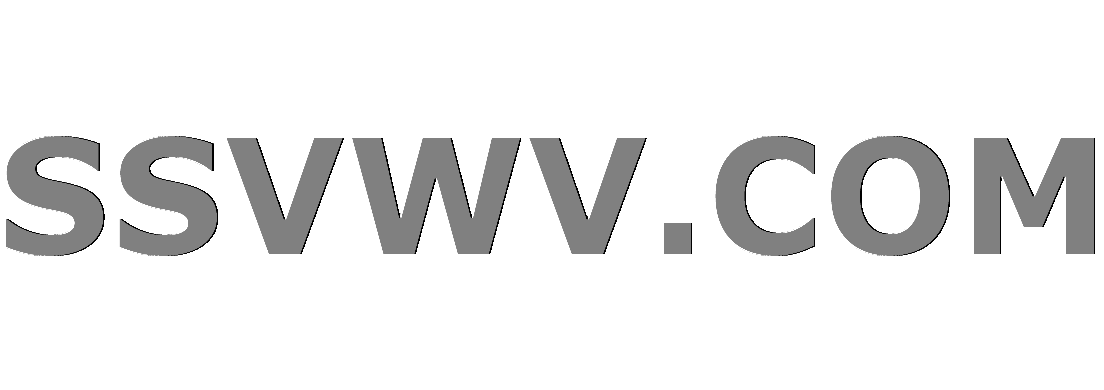
Multi tool use
Let's say I have StaffAttendanceCheckIn.Java, if user click on the addOnItemTouchListener inside, i want to make it either show dialog or go to another page and show out the attendance record. For example if user clicked on the Event Name Google, it would show out the firebase record. After click inside it will show out filtering. If user choose Tuesday 06 Nov 2018, it will show out the check in date and check in time. I'm not sure how to write after onDataChange
StaffAttendanceCheckIn.Java
package com.example.edward.neweventmanagementsystem;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.design.widget.FloatingActionButton;
import android.support.v7.app.ActionBar;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import android.widget.Toast;
import com.bumptech.glide.Glide;
import com.example.edward.neweventmanagementsystem.Model.EventInfo;
import com.example.edward.neweventmanagementsystem.ViewHolder.MenuViewHolder;
import com.firebase.ui.database.FirebaseRecyclerAdapter;
import com.firebase.ui.database.FirebaseRecyclerOptions;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.Query;
import com.google.firebase.database.ValueEventListener;
import com.google.firebase.storage.FirebaseStorage;
import com.google.firebase.storage.StorageReference;
public class StaffAttendanceRecordTable extends AppCompatActivity {
FirebaseDatabase database;
DatabaseReference eventInfo;
FloatingActionButton search_item;
RecyclerView recycle_menu;
RecyclerView.LayoutManager layoutManager;
FirebaseRecyclerAdapter <EventInfo, MenuViewHolder> adapter;
private DatabaseReference mDatabaseReference;
private TextView EventName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_staff_attendance_record_table);
recycle_menu = (RecyclerView) findViewById(R.id.recycle_menu);
recycle_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycle_menu.setLayoutManager(layoutManager);
mDatabaseReference = FirebaseDatabase.getInstance().getReference().child("AttendanceRecord");
recycle_menu.setLayoutManager(layoutManager);
search_item = (FloatingActionButton) findViewById(R.id.search_item);
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ;
eventInfo = FirebaseDatabase.getInstance().getReference().child("ListOfEvent").child(currentFirebaseUser.getUid());
loadMenu();
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
search_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent search = new Intent(StaffAttendanceRecordTable.this, Find_Event.class);
startActivity(search);
System.out.println("Testing search Item");
}
});
}
public void loadMenu(){
Query query = eventInfo.orderByKey();
FirebaseRecyclerOptions firebaseRecyclerOptions = new FirebaseRecyclerOptions.Builder<EventInfo>().setQuery(query, EventInfo.class).build();
adapter = new FirebaseRecyclerAdapter<EventInfo, MenuViewHolder>(firebaseRecyclerOptions) {
@NonNull
@Override
public MenuViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.activity_list,viewGroup,false);
return new MenuViewHolder(view);
}
@Override
protected void onBindViewHolder(@NonNull MenuViewHolder holder, int position, @NonNull EventInfo model) {
holder.txtRegisterEventId.setText(model.getRegisterEventId());
holder.txtRegisterEventStartDate.setText(model.getRegisterEventStartDate());
holder.txtRegisterEventName.setText(model.getRegisterEventName());
holder.txtRegisterContactNumber.setText(model.getRegisterContactNumber());
holder.txtRegisterEventRadiogroup.setText(model.getRegisterEventRadiogroup());
holder.txtRegisterEventLocation.setText(model.getRegisterEventLocation());
holder.txtEventPrice.setText(model.getEventPrice());
holder.txtEventCapacity.setText(model.getEventCapacity());
holder.fileName.setText(model.getFileName());
//Picasso.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
//Glide.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
Glide.with(getBaseContext()).load(model.getImageToUpload()
).into(holder.imageView);
System.out.println(model.getRegisterEventName());
System.out.println(model.getImageToUpload());
}
};
recycle_menu.setAdapter(adapter);
}
@Override
protected void onStart() {
super.onStart();
adapter.startListening();
}
@Override
protected void onStop() {
super.onStop();
adapter.stopListening();
}
}
java


add a comment |
Let's say I have StaffAttendanceCheckIn.Java, if user click on the addOnItemTouchListener inside, i want to make it either show dialog or go to another page and show out the attendance record. For example if user clicked on the Event Name Google, it would show out the firebase record. After click inside it will show out filtering. If user choose Tuesday 06 Nov 2018, it will show out the check in date and check in time. I'm not sure how to write after onDataChange
StaffAttendanceCheckIn.Java
package com.example.edward.neweventmanagementsystem;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.design.widget.FloatingActionButton;
import android.support.v7.app.ActionBar;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import android.widget.Toast;
import com.bumptech.glide.Glide;
import com.example.edward.neweventmanagementsystem.Model.EventInfo;
import com.example.edward.neweventmanagementsystem.ViewHolder.MenuViewHolder;
import com.firebase.ui.database.FirebaseRecyclerAdapter;
import com.firebase.ui.database.FirebaseRecyclerOptions;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.Query;
import com.google.firebase.database.ValueEventListener;
import com.google.firebase.storage.FirebaseStorage;
import com.google.firebase.storage.StorageReference;
public class StaffAttendanceRecordTable extends AppCompatActivity {
FirebaseDatabase database;
DatabaseReference eventInfo;
FloatingActionButton search_item;
RecyclerView recycle_menu;
RecyclerView.LayoutManager layoutManager;
FirebaseRecyclerAdapter <EventInfo, MenuViewHolder> adapter;
private DatabaseReference mDatabaseReference;
private TextView EventName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_staff_attendance_record_table);
recycle_menu = (RecyclerView) findViewById(R.id.recycle_menu);
recycle_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycle_menu.setLayoutManager(layoutManager);
mDatabaseReference = FirebaseDatabase.getInstance().getReference().child("AttendanceRecord");
recycle_menu.setLayoutManager(layoutManager);
search_item = (FloatingActionButton) findViewById(R.id.search_item);
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ;
eventInfo = FirebaseDatabase.getInstance().getReference().child("ListOfEvent").child(currentFirebaseUser.getUid());
loadMenu();
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
search_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent search = new Intent(StaffAttendanceRecordTable.this, Find_Event.class);
startActivity(search);
System.out.println("Testing search Item");
}
});
}
public void loadMenu(){
Query query = eventInfo.orderByKey();
FirebaseRecyclerOptions firebaseRecyclerOptions = new FirebaseRecyclerOptions.Builder<EventInfo>().setQuery(query, EventInfo.class).build();
adapter = new FirebaseRecyclerAdapter<EventInfo, MenuViewHolder>(firebaseRecyclerOptions) {
@NonNull
@Override
public MenuViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.activity_list,viewGroup,false);
return new MenuViewHolder(view);
}
@Override
protected void onBindViewHolder(@NonNull MenuViewHolder holder, int position, @NonNull EventInfo model) {
holder.txtRegisterEventId.setText(model.getRegisterEventId());
holder.txtRegisterEventStartDate.setText(model.getRegisterEventStartDate());
holder.txtRegisterEventName.setText(model.getRegisterEventName());
holder.txtRegisterContactNumber.setText(model.getRegisterContactNumber());
holder.txtRegisterEventRadiogroup.setText(model.getRegisterEventRadiogroup());
holder.txtRegisterEventLocation.setText(model.getRegisterEventLocation());
holder.txtEventPrice.setText(model.getEventPrice());
holder.txtEventCapacity.setText(model.getEventCapacity());
holder.fileName.setText(model.getFileName());
//Picasso.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
//Glide.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
Glide.with(getBaseContext()).load(model.getImageToUpload()
).into(holder.imageView);
System.out.println(model.getRegisterEventName());
System.out.println(model.getImageToUpload());
}
};
recycle_menu.setAdapter(adapter);
}
@Override
protected void onStart() {
super.onStart();
adapter.startListening();
}
@Override
protected void onStop() {
super.onStop();
adapter.stopListening();
}
}
java


add a comment |
Let's say I have StaffAttendanceCheckIn.Java, if user click on the addOnItemTouchListener inside, i want to make it either show dialog or go to another page and show out the attendance record. For example if user clicked on the Event Name Google, it would show out the firebase record. After click inside it will show out filtering. If user choose Tuesday 06 Nov 2018, it will show out the check in date and check in time. I'm not sure how to write after onDataChange
StaffAttendanceCheckIn.Java
package com.example.edward.neweventmanagementsystem;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.design.widget.FloatingActionButton;
import android.support.v7.app.ActionBar;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import android.widget.Toast;
import com.bumptech.glide.Glide;
import com.example.edward.neweventmanagementsystem.Model.EventInfo;
import com.example.edward.neweventmanagementsystem.ViewHolder.MenuViewHolder;
import com.firebase.ui.database.FirebaseRecyclerAdapter;
import com.firebase.ui.database.FirebaseRecyclerOptions;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.Query;
import com.google.firebase.database.ValueEventListener;
import com.google.firebase.storage.FirebaseStorage;
import com.google.firebase.storage.StorageReference;
public class StaffAttendanceRecordTable extends AppCompatActivity {
FirebaseDatabase database;
DatabaseReference eventInfo;
FloatingActionButton search_item;
RecyclerView recycle_menu;
RecyclerView.LayoutManager layoutManager;
FirebaseRecyclerAdapter <EventInfo, MenuViewHolder> adapter;
private DatabaseReference mDatabaseReference;
private TextView EventName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_staff_attendance_record_table);
recycle_menu = (RecyclerView) findViewById(R.id.recycle_menu);
recycle_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycle_menu.setLayoutManager(layoutManager);
mDatabaseReference = FirebaseDatabase.getInstance().getReference().child("AttendanceRecord");
recycle_menu.setLayoutManager(layoutManager);
search_item = (FloatingActionButton) findViewById(R.id.search_item);
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ;
eventInfo = FirebaseDatabase.getInstance().getReference().child("ListOfEvent").child(currentFirebaseUser.getUid());
loadMenu();
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
search_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent search = new Intent(StaffAttendanceRecordTable.this, Find_Event.class);
startActivity(search);
System.out.println("Testing search Item");
}
});
}
public void loadMenu(){
Query query = eventInfo.orderByKey();
FirebaseRecyclerOptions firebaseRecyclerOptions = new FirebaseRecyclerOptions.Builder<EventInfo>().setQuery(query, EventInfo.class).build();
adapter = new FirebaseRecyclerAdapter<EventInfo, MenuViewHolder>(firebaseRecyclerOptions) {
@NonNull
@Override
public MenuViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.activity_list,viewGroup,false);
return new MenuViewHolder(view);
}
@Override
protected void onBindViewHolder(@NonNull MenuViewHolder holder, int position, @NonNull EventInfo model) {
holder.txtRegisterEventId.setText(model.getRegisterEventId());
holder.txtRegisterEventStartDate.setText(model.getRegisterEventStartDate());
holder.txtRegisterEventName.setText(model.getRegisterEventName());
holder.txtRegisterContactNumber.setText(model.getRegisterContactNumber());
holder.txtRegisterEventRadiogroup.setText(model.getRegisterEventRadiogroup());
holder.txtRegisterEventLocation.setText(model.getRegisterEventLocation());
holder.txtEventPrice.setText(model.getEventPrice());
holder.txtEventCapacity.setText(model.getEventCapacity());
holder.fileName.setText(model.getFileName());
//Picasso.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
//Glide.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
Glide.with(getBaseContext()).load(model.getImageToUpload()
).into(holder.imageView);
System.out.println(model.getRegisterEventName());
System.out.println(model.getImageToUpload());
}
};
recycle_menu.setAdapter(adapter);
}
@Override
protected void onStart() {
super.onStart();
adapter.startListening();
}
@Override
protected void onStop() {
super.onStop();
adapter.stopListening();
}
}
java


Let's say I have StaffAttendanceCheckIn.Java, if user click on the addOnItemTouchListener inside, i want to make it either show dialog or go to another page and show out the attendance record. For example if user clicked on the Event Name Google, it would show out the firebase record. After click inside it will show out filtering. If user choose Tuesday 06 Nov 2018, it will show out the check in date and check in time. I'm not sure how to write after onDataChange
StaffAttendanceCheckIn.Java
package com.example.edward.neweventmanagementsystem;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.design.widget.FloatingActionButton;
import android.support.v7.app.ActionBar;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import android.widget.Toast;
import com.bumptech.glide.Glide;
import com.example.edward.neweventmanagementsystem.Model.EventInfo;
import com.example.edward.neweventmanagementsystem.ViewHolder.MenuViewHolder;
import com.firebase.ui.database.FirebaseRecyclerAdapter;
import com.firebase.ui.database.FirebaseRecyclerOptions;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.Query;
import com.google.firebase.database.ValueEventListener;
import com.google.firebase.storage.FirebaseStorage;
import com.google.firebase.storage.StorageReference;
public class StaffAttendanceRecordTable extends AppCompatActivity {
FirebaseDatabase database;
DatabaseReference eventInfo;
FloatingActionButton search_item;
RecyclerView recycle_menu;
RecyclerView.LayoutManager layoutManager;
FirebaseRecyclerAdapter <EventInfo, MenuViewHolder> adapter;
private DatabaseReference mDatabaseReference;
private TextView EventName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_staff_attendance_record_table);
recycle_menu = (RecyclerView) findViewById(R.id.recycle_menu);
recycle_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycle_menu.setLayoutManager(layoutManager);
mDatabaseReference = FirebaseDatabase.getInstance().getReference().child("AttendanceRecord");
recycle_menu.setLayoutManager(layoutManager);
search_item = (FloatingActionButton) findViewById(R.id.search_item);
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ;
eventInfo = FirebaseDatabase.getInstance().getReference().child("ListOfEvent").child(currentFirebaseUser.getUid());
loadMenu();
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
search_item.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent search = new Intent(StaffAttendanceRecordTable.this, Find_Event.class);
startActivity(search);
System.out.println("Testing search Item");
}
});
}
public void loadMenu(){
Query query = eventInfo.orderByKey();
FirebaseRecyclerOptions firebaseRecyclerOptions = new FirebaseRecyclerOptions.Builder<EventInfo>().setQuery(query, EventInfo.class).build();
adapter = new FirebaseRecyclerAdapter<EventInfo, MenuViewHolder>(firebaseRecyclerOptions) {
@NonNull
@Override
public MenuViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.activity_list,viewGroup,false);
return new MenuViewHolder(view);
}
@Override
protected void onBindViewHolder(@NonNull MenuViewHolder holder, int position, @NonNull EventInfo model) {
holder.txtRegisterEventId.setText(model.getRegisterEventId());
holder.txtRegisterEventStartDate.setText(model.getRegisterEventStartDate());
holder.txtRegisterEventName.setText(model.getRegisterEventName());
holder.txtRegisterContactNumber.setText(model.getRegisterContactNumber());
holder.txtRegisterEventRadiogroup.setText(model.getRegisterEventRadiogroup());
holder.txtRegisterEventLocation.setText(model.getRegisterEventLocation());
holder.txtEventPrice.setText(model.getEventPrice());
holder.txtEventCapacity.setText(model.getEventCapacity());
holder.fileName.setText(model.getFileName());
//Picasso.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
//Glide.with(getBaseContext()).load(model.getImageToUpload()).into(viewHolder.imageView);
Glide.with(getBaseContext()).load(model.getImageToUpload()
).into(holder.imageView);
System.out.println(model.getRegisterEventName());
System.out.println(model.getImageToUpload());
}
};
recycle_menu.setAdapter(adapter);
}
@Override
protected void onStart() {
super.onStart();
adapter.startListening();
}
@Override
protected void onStop() {
super.onStop();
adapter.stopListening();
}
}
java


java


edited Nov 12 '18 at 5:39


Alireza Noorali
1,419633
1,419633
asked Nov 12 '18 at 4:12
Edward Liew
135
135
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
As you said when clicked on addOnItemTouchListener
then you want to fetch all events.
You can use this code. Little modified your code.
Using this code you will get All Event Names inside Attendance Record,
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
DatabaseReference mDatabaseReference;
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
ArrayList<String> eventNames = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventNames.add(snapshot.getKey());
//Now you have all Event Names in List
// you can show them in a Custom Alert Dialog
//Or pass it to next Activity.
}
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
Lets say you have displayed these codes in your Activity/Alert Dialog and user clicked on any event.
You can fetch all days inside that event using below code.
databaseReference.child("clicked_item").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
ArrayList<String> eventDays = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventDays.add(snapshot.getKey());
//Now you have all days that exist inside Clicked Item (Google)
//Same as previous you can show them in Alert Dialog or New Activity,
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
The Last part.. As you have all the Days and you have displayed these days to user and User clicked on any day.
Now you want to fetch checkInDate & checkInTime. you can use below code to fetch this data.
databaseReference.child("clciked_Day").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
String checkInDate = snapshot.child("checkInDate").getValue().toString();
String checkInTime = snapshot.child("checkInTime").getValue().toString();
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
Hope this will help you !!
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned heredisplayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass theArraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?
– Ali Ahmed
Nov 12 '18 at 6:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255878%2fhow-to-match-the-name-and-show-details-from-firebase-in-another-java-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As you said when clicked on addOnItemTouchListener
then you want to fetch all events.
You can use this code. Little modified your code.
Using this code you will get All Event Names inside Attendance Record,
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
DatabaseReference mDatabaseReference;
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
ArrayList<String> eventNames = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventNames.add(snapshot.getKey());
//Now you have all Event Names in List
// you can show them in a Custom Alert Dialog
//Or pass it to next Activity.
}
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
Lets say you have displayed these codes in your Activity/Alert Dialog and user clicked on any event.
You can fetch all days inside that event using below code.
databaseReference.child("clicked_item").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
ArrayList<String> eventDays = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventDays.add(snapshot.getKey());
//Now you have all days that exist inside Clicked Item (Google)
//Same as previous you can show them in Alert Dialog or New Activity,
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
The Last part.. As you have all the Days and you have displayed these days to user and User clicked on any day.
Now you want to fetch checkInDate & checkInTime. you can use below code to fetch this data.
databaseReference.child("clciked_Day").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
String checkInDate = snapshot.child("checkInDate").getValue().toString();
String checkInTime = snapshot.child("checkInTime").getValue().toString();
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
Hope this will help you !!
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned heredisplayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass theArraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?
– Ali Ahmed
Nov 12 '18 at 6:26
add a comment |
As you said when clicked on addOnItemTouchListener
then you want to fetch all events.
You can use this code. Little modified your code.
Using this code you will get All Event Names inside Attendance Record,
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
DatabaseReference mDatabaseReference;
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
ArrayList<String> eventNames = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventNames.add(snapshot.getKey());
//Now you have all Event Names in List
// you can show them in a Custom Alert Dialog
//Or pass it to next Activity.
}
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
Lets say you have displayed these codes in your Activity/Alert Dialog and user clicked on any event.
You can fetch all days inside that event using below code.
databaseReference.child("clicked_item").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
ArrayList<String> eventDays = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventDays.add(snapshot.getKey());
//Now you have all days that exist inside Clicked Item (Google)
//Same as previous you can show them in Alert Dialog or New Activity,
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
The Last part.. As you have all the Days and you have displayed these days to user and User clicked on any day.
Now you want to fetch checkInDate & checkInTime. you can use below code to fetch this data.
databaseReference.child("clciked_Day").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
String checkInDate = snapshot.child("checkInDate").getValue().toString();
String checkInTime = snapshot.child("checkInTime").getValue().toString();
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
Hope this will help you !!
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned heredisplayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass theArraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?
– Ali Ahmed
Nov 12 '18 at 6:26
add a comment |
As you said when clicked on addOnItemTouchListener
then you want to fetch all events.
You can use this code. Little modified your code.
Using this code you will get All Event Names inside Attendance Record,
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
DatabaseReference mDatabaseReference;
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
ArrayList<String> eventNames = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventNames.add(snapshot.getKey());
//Now you have all Event Names in List
// you can show them in a Custom Alert Dialog
//Or pass it to next Activity.
}
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
Lets say you have displayed these codes in your Activity/Alert Dialog and user clicked on any event.
You can fetch all days inside that event using below code.
databaseReference.child("clicked_item").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
ArrayList<String> eventDays = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventDays.add(snapshot.getKey());
//Now you have all days that exist inside Clicked Item (Google)
//Same as previous you can show them in Alert Dialog or New Activity,
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
The Last part.. As you have all the Days and you have displayed these days to user and User clicked on any day.
Now you want to fetch checkInDate & checkInTime. you can use below code to fetch this data.
databaseReference.child("clciked_Day").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
String checkInDate = snapshot.child("checkInDate").getValue().toString();
String checkInTime = snapshot.child("checkInTime").getValue().toString();
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
Hope this will help you !!
As you said when clicked on addOnItemTouchListener
then you want to fetch all events.
You can use this code. Little modified your code.
Using this code you will get All Event Names inside Attendance Record,
recycle_menu.addOnItemTouchListener(
new RecyclerItemClickListener(getBaseContext(), recycle_menu ,new RecyclerItemClickListener.OnItemClickListener() {
@Override public void onItemClick(View view, int position) {
DatabaseReference mDatabaseReference;
mDatabaseReference.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
ArrayList<String> eventNames = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventNames.add(snapshot.getKey());
//Now you have all Event Names in List
// you can show them in a Custom Alert Dialog
//Or pass it to next Activity.
}
EventName = (TextView) findViewById(R.id.RegisterEventName);
if(dataSnapshot.child(EventName.getText().toString()).exists()){
Toast.makeText(StaffAttendanceRecordTable.this, "Got Result", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(StaffAttendanceRecordTable.this, com.example.edward.neweventmanagementsystem.attendanceListMainList.class);
startActivity(intent);
}else{
Toast.makeText(StaffAttendanceRecordTable.this, "No Result", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
@Override public void onLongItemClick(final View view, int position) {
}
})
);
Lets say you have displayed these codes in your Activity/Alert Dialog and user clicked on any event.
You can fetch all days inside that event using below code.
databaseReference.child("clicked_item").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
ArrayList<String> eventDays = new ArrayList<>();
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
eventDays.add(snapshot.getKey());
//Now you have all days that exist inside Clicked Item (Google)
//Same as previous you can show them in Alert Dialog or New Activity,
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
The Last part.. As you have all the Days and you have displayed these days to user and User clicked on any day.
Now you want to fetch checkInDate & checkInTime. you can use below code to fetch this data.
databaseReference.child("clciked_Day").addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
for (DataSnapshot snapshot : dataSnapshot.getChildren()) {
String checkInDate = snapshot.child("checkInDate").getValue().toString();
String checkInTime = snapshot.child("checkInTime").getValue().toString();
}
}
@Override
public void onCancelled(DatabaseError databaseError) {
}
});
Hope this will help you !!
edited Nov 12 '18 at 5:50
answered Nov 12 '18 at 5:45


Ali Ahmed
1,2691314
1,2691314
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned heredisplayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass theArraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?
– Ali Ahmed
Nov 12 '18 at 6:26
add a comment |
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned heredisplayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass theArraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?
– Ali Ahmed
Nov 12 '18 at 6:26
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
I found your eventNames can fetch all the all the event name but seems like my code after EventName = (TextView) findViewById(R.id.RegisterEventName); got problem. How to amend it to get the proper event name in the recycle view after click? If user click the Google then it shpw get the event name google
– Edward Liew
Nov 12 '18 at 6:15
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
didn't get you. what you want exactly?
– Ali Ahmed
Nov 12 '18 at 6:20
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
you have already hard coded event names in your XML? that's what you're saying ?
– Ali Ahmed
Nov 12 '18 at 6:21
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
Or I change another way to say. How can i get the only one event name if user click 1st event get 1st event name, clcik second event get second event name? Becasue currently is get all the names.
– Edward Liew
Nov 12 '18 at 6:23
That's what i mentioned here
displayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass the Arraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?– Ali Ahmed
Nov 12 '18 at 6:26
That's what i mentioned here
displayed these codes in your Activity/Alert Dialog
. you have to display all events in Custom Alert Dialog, let user to click on any event and fetch data according to that event. you can also pass the Arraylist
to next activity and display it in recylerview and then listen for user click. On user click fetch days inside that event,, Got it ?– Ali Ahmed
Nov 12 '18 at 6:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255878%2fhow-to-match-the-name-and-show-details-from-firebase-in-another-java-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mRKS f,GfQ8 6DuK2X6S8o,CM9TrF 6rtGY3Kysx zCaaLQn,zFCSoOiRgIhwMyE zluPhbR KO3Cr8,UDJKh0Vw,M,Kt1JHrR,Z Eg,NoGPv