How to properly display DatePickerDialog using MaterialComponents?
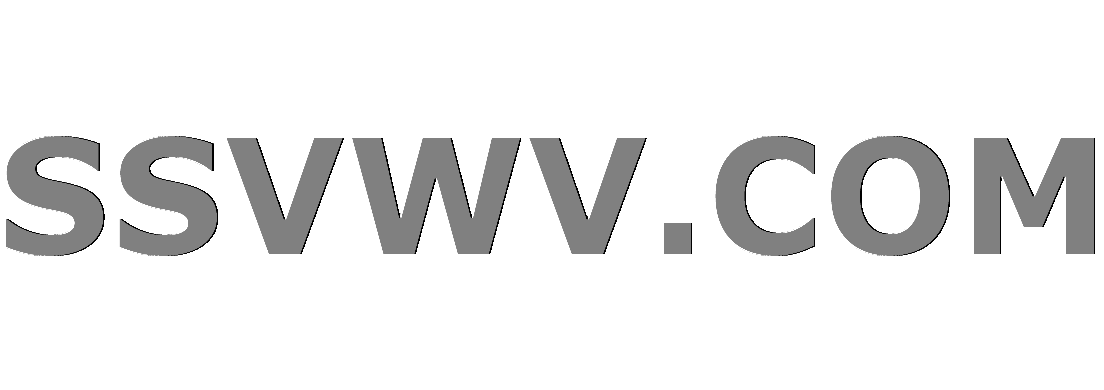
Multi tool use
I'm using MaterialComponents for Android from material.io.
I have two themes (light and dark). I want to show an DatePickerDialog.
But this is the result:
Here is the code how i show my dialog:
private fun selectDeadline() {
val dialog = DateDialog()
val bundle = Bundle()
bundle.putInt("themeId", themeId)
dialog.arguments = bundle
dialog.show(supportFragmentManager, "")
}
class DateDialog : DialogFragment(), DatePickerDialog.OnDateSetListener {
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog {
val date = DateTime.now()
val themeId = arguments!!.getInt("themeId")
return DatePickerDialog(activity!!, themeId, this, date.year, date.monthOfYear - 1, date.dayOfMonth)
}
override fun onDateSet(view: DatePicker?, year: Int, month: Int, day: Int) {
deadlineTitle.text = "$year, $month, $day"
}
}
And last but not least, my themes:
<resources>
<!-- Dark application theme. -->
<style name="AppTheme.Dark" parent="Theme.MaterialComponents.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/darkBackground</item>
<item name="toolbarStyle">@style/AppTheme.Dark.Toolbar</item>
<item name="iconColor">#fff</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_dark</item>
<item name="android:statusBarColor">@android:color/black</item>
</style>
<style name="AppTheme.Dark.Toolbar" parent="Widget.AppCompat.Toolbar">
<item name="android:background">@color/darkBackground</item>
<item name="android:elevation">8dp</item>
</style>
<!-- Light application theme. -->
<style name="AppTheme.Light" parent="Theme.MaterialComponents.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/lightBackground</item>
<item name="toolbarStyle">@style/AppTheme.Light.Toolbar</item>
<item name="iconColor">#000</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_bright</item>
<item name="android:datePickerDialogTheme">@style/AppTheme.Light.DatePicker</item>
<item name="android:statusBarColor">@color/colorPrimaryDark</item>
</style>
<style name="AppTheme.Light.DatePicker" parent="ThemeOverlay.MaterialComponents.Dialog">
<item name="materialButtonStyle">?android:attr/borderlessButtonStyle</item>
</style>
<style name="AppTheme.Light.Toolbar" parent="Widget.MaterialComponents.Toolbar">
<item name="android:background">@color/colorPrimary</item>
<item name="android:elevation">8dp</item>
</style>
If i don't give themeId as parameter it shows properly on light theme but on dark theme it shows just the light version with white text which is invisible for the user to see because of the white background.
What is the proper way to solve this problem?

add a comment |
I'm using MaterialComponents for Android from material.io.
I have two themes (light and dark). I want to show an DatePickerDialog.
But this is the result:
Here is the code how i show my dialog:
private fun selectDeadline() {
val dialog = DateDialog()
val bundle = Bundle()
bundle.putInt("themeId", themeId)
dialog.arguments = bundle
dialog.show(supportFragmentManager, "")
}
class DateDialog : DialogFragment(), DatePickerDialog.OnDateSetListener {
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog {
val date = DateTime.now()
val themeId = arguments!!.getInt("themeId")
return DatePickerDialog(activity!!, themeId, this, date.year, date.monthOfYear - 1, date.dayOfMonth)
}
override fun onDateSet(view: DatePicker?, year: Int, month: Int, day: Int) {
deadlineTitle.text = "$year, $month, $day"
}
}
And last but not least, my themes:
<resources>
<!-- Dark application theme. -->
<style name="AppTheme.Dark" parent="Theme.MaterialComponents.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/darkBackground</item>
<item name="toolbarStyle">@style/AppTheme.Dark.Toolbar</item>
<item name="iconColor">#fff</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_dark</item>
<item name="android:statusBarColor">@android:color/black</item>
</style>
<style name="AppTheme.Dark.Toolbar" parent="Widget.AppCompat.Toolbar">
<item name="android:background">@color/darkBackground</item>
<item name="android:elevation">8dp</item>
</style>
<!-- Light application theme. -->
<style name="AppTheme.Light" parent="Theme.MaterialComponents.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/lightBackground</item>
<item name="toolbarStyle">@style/AppTheme.Light.Toolbar</item>
<item name="iconColor">#000</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_bright</item>
<item name="android:datePickerDialogTheme">@style/AppTheme.Light.DatePicker</item>
<item name="android:statusBarColor">@color/colorPrimaryDark</item>
</style>
<style name="AppTheme.Light.DatePicker" parent="ThemeOverlay.MaterialComponents.Dialog">
<item name="materialButtonStyle">?android:attr/borderlessButtonStyle</item>
</style>
<style name="AppTheme.Light.Toolbar" parent="Widget.MaterialComponents.Toolbar">
<item name="android:background">@color/colorPrimary</item>
<item name="android:elevation">8dp</item>
</style>
If i don't give themeId as parameter it shows properly on light theme but on dark theme it shows just the light version with white text which is invisible for the user to see because of the white background.
What is the proper way to solve this problem?

add a comment |
I'm using MaterialComponents for Android from material.io.
I have two themes (light and dark). I want to show an DatePickerDialog.
But this is the result:
Here is the code how i show my dialog:
private fun selectDeadline() {
val dialog = DateDialog()
val bundle = Bundle()
bundle.putInt("themeId", themeId)
dialog.arguments = bundle
dialog.show(supportFragmentManager, "")
}
class DateDialog : DialogFragment(), DatePickerDialog.OnDateSetListener {
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog {
val date = DateTime.now()
val themeId = arguments!!.getInt("themeId")
return DatePickerDialog(activity!!, themeId, this, date.year, date.monthOfYear - 1, date.dayOfMonth)
}
override fun onDateSet(view: DatePicker?, year: Int, month: Int, day: Int) {
deadlineTitle.text = "$year, $month, $day"
}
}
And last but not least, my themes:
<resources>
<!-- Dark application theme. -->
<style name="AppTheme.Dark" parent="Theme.MaterialComponents.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/darkBackground</item>
<item name="toolbarStyle">@style/AppTheme.Dark.Toolbar</item>
<item name="iconColor">#fff</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_dark</item>
<item name="android:statusBarColor">@android:color/black</item>
</style>
<style name="AppTheme.Dark.Toolbar" parent="Widget.AppCompat.Toolbar">
<item name="android:background">@color/darkBackground</item>
<item name="android:elevation">8dp</item>
</style>
<!-- Light application theme. -->
<style name="AppTheme.Light" parent="Theme.MaterialComponents.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/lightBackground</item>
<item name="toolbarStyle">@style/AppTheme.Light.Toolbar</item>
<item name="iconColor">#000</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_bright</item>
<item name="android:datePickerDialogTheme">@style/AppTheme.Light.DatePicker</item>
<item name="android:statusBarColor">@color/colorPrimaryDark</item>
</style>
<style name="AppTheme.Light.DatePicker" parent="ThemeOverlay.MaterialComponents.Dialog">
<item name="materialButtonStyle">?android:attr/borderlessButtonStyle</item>
</style>
<style name="AppTheme.Light.Toolbar" parent="Widget.MaterialComponents.Toolbar">
<item name="android:background">@color/colorPrimary</item>
<item name="android:elevation">8dp</item>
</style>
If i don't give themeId as parameter it shows properly on light theme but on dark theme it shows just the light version with white text which is invisible for the user to see because of the white background.
What is the proper way to solve this problem?

I'm using MaterialComponents for Android from material.io.
I have two themes (light and dark). I want to show an DatePickerDialog.
But this is the result:
Here is the code how i show my dialog:
private fun selectDeadline() {
val dialog = DateDialog()
val bundle = Bundle()
bundle.putInt("themeId", themeId)
dialog.arguments = bundle
dialog.show(supportFragmentManager, "")
}
class DateDialog : DialogFragment(), DatePickerDialog.OnDateSetListener {
override fun onCreateDialog(savedInstanceState: Bundle?): Dialog {
val date = DateTime.now()
val themeId = arguments!!.getInt("themeId")
return DatePickerDialog(activity!!, themeId, this, date.year, date.monthOfYear - 1, date.dayOfMonth)
}
override fun onDateSet(view: DatePicker?, year: Int, month: Int, day: Int) {
deadlineTitle.text = "$year, $month, $day"
}
}
And last but not least, my themes:
<resources>
<!-- Dark application theme. -->
<style name="AppTheme.Dark" parent="Theme.MaterialComponents.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/darkBackground</item>
<item name="toolbarStyle">@style/AppTheme.Dark.Toolbar</item>
<item name="iconColor">#fff</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_dark</item>
<item name="android:statusBarColor">@android:color/black</item>
</style>
<style name="AppTheme.Dark.Toolbar" parent="Widget.AppCompat.Toolbar">
<item name="android:background">@color/darkBackground</item>
<item name="android:elevation">8dp</item>
</style>
<!-- Light application theme. -->
<style name="AppTheme.Light" parent="Theme.MaterialComponents.Light.NoActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="toolbarBackground">@color/lightBackground</item>
<item name="toolbarStyle">@style/AppTheme.Light.Toolbar</item>
<item name="iconColor">#000</item>
<item name="dividerBackground">@android:drawable/divider_horizontal_bright</item>
<item name="android:datePickerDialogTheme">@style/AppTheme.Light.DatePicker</item>
<item name="android:statusBarColor">@color/colorPrimaryDark</item>
</style>
<style name="AppTheme.Light.DatePicker" parent="ThemeOverlay.MaterialComponents.Dialog">
<item name="materialButtonStyle">?android:attr/borderlessButtonStyle</item>
</style>
<style name="AppTheme.Light.Toolbar" parent="Widget.MaterialComponents.Toolbar">
<item name="android:background">@color/colorPrimary</item>
<item name="android:elevation">8dp</item>
</style>
If i don't give themeId as parameter it shows properly on light theme but on dark theme it shows just the light version with white text which is invisible for the user to see because of the white background.
What is the proper way to solve this problem?


asked Nov 13 '18 at 12:04


Daniel DäschleDaniel Däschle
11010
11010
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Thank you for the question. The Material Components library currently doesn't support dark mode for date and time pickers. We plan to add support for this, please stay tuned.
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280662%2fhow-to-properly-display-datepickerdialog-using-materialcomponents%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thank you for the question. The Material Components library currently doesn't support dark mode for date and time pickers. We plan to add support for this, please stay tuned.
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
add a comment |
Thank you for the question. The Material Components library currently doesn't support dark mode for date and time pickers. We plan to add support for this, please stay tuned.
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
add a comment |
Thank you for the question. The Material Components library currently doesn't support dark mode for date and time pickers. We plan to add support for this, please stay tuned.
Thank you for the question. The Material Components library currently doesn't support dark mode for date and time pickers. We plan to add support for this, please stay tuned.
answered Nov 14 '18 at 18:28


wcshiwcshi
513
513
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
add a comment |
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
Nice, thank you. My solution was to use the MaterialComponents Bridge Theme in combination with MaterialDateTimePicker.
– Daniel Däschle
Nov 15 '18 at 8:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280662%2fhow-to-properly-display-datepickerdialog-using-materialcomponents%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
r U82VcJ qX0 9oexC6 yUngQCzSXbQ8W PtiXBRw9w Zrnj4,y93r6 sjY,n qWUZj,J,iPrhy0Ukx1U