MVC .NET CORE - DataAnnotation's Required(ErrorMessage=“Err”) doesn't show up on server-side validation
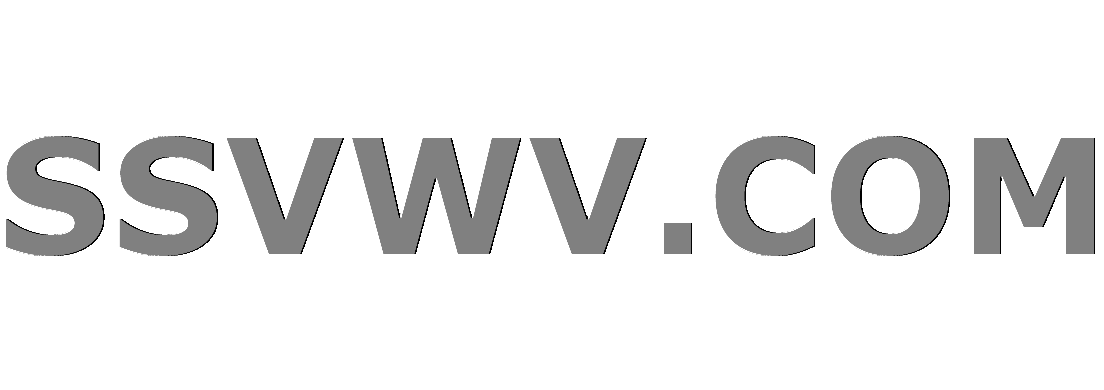
Multi tool use
I have created a web application using .Net MVC Core, with Code First approach, and generated the Views/Controllers through scaffolding.
For validation, I'm using Fluent API and Data Annotations, and everything seems to be working just fine.
However my DataAnnotation's custom error messages, show up only on the client-side validation, and when I block my browser's JS to test my server-side validation, I get the following error message instead: "The value '' is invalid."
I tried to use [DataType(DataType.DateTime, ErrorMessage = "Invalid date!")] but it didn't work as well!
My Model:
public class MyModel
{
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Departure Date")]
public DateTime DateDeparture { get; set; }
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Arrival Date")]
public DateTime DateArrival { get; set; }
}
My View:
public IActionResult Create()
{
return View();
}
My Controller:
@model App.Models.MyModel
@{
ViewData["Title"] = "Create";
}
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="DateDeparture" class="control-label"></label>
<input asp-for="DateDeparture" class="form-control" />
<span asp-validation-for="DateDeparture" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="DateArrival" class="control-label"></label>
<input asp-for="DateArrival" class="form-control" />
<span asp-validation-for="DateArrival" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</form>
Client-Side Validation
Server-Side Validation
According to the suggested solution here for a similar scenario (Thread) I have to make my field nullable!
Which doesn't seem to be a proper solution for all cases! And I already have another form with Non-Nullable string, that generates the same custom error message for both client & server sides validations, that's why I believe the issue is more related to the scalar values rather than Non/Nullable type options, but I can't figure out the reason, to be able to override that server-side validation message!
Any help would be appreciated…
.net ef-code-first asp.net-core-mvc data-annotations
add a comment |
I have created a web application using .Net MVC Core, with Code First approach, and generated the Views/Controllers through scaffolding.
For validation, I'm using Fluent API and Data Annotations, and everything seems to be working just fine.
However my DataAnnotation's custom error messages, show up only on the client-side validation, and when I block my browser's JS to test my server-side validation, I get the following error message instead: "The value '' is invalid."
I tried to use [DataType(DataType.DateTime, ErrorMessage = "Invalid date!")] but it didn't work as well!
My Model:
public class MyModel
{
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Departure Date")]
public DateTime DateDeparture { get; set; }
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Arrival Date")]
public DateTime DateArrival { get; set; }
}
My View:
public IActionResult Create()
{
return View();
}
My Controller:
@model App.Models.MyModel
@{
ViewData["Title"] = "Create";
}
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="DateDeparture" class="control-label"></label>
<input asp-for="DateDeparture" class="form-control" />
<span asp-validation-for="DateDeparture" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="DateArrival" class="control-label"></label>
<input asp-for="DateArrival" class="form-control" />
<span asp-validation-for="DateArrival" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</form>
Client-Side Validation
Server-Side Validation
According to the suggested solution here for a similar scenario (Thread) I have to make my field nullable!
Which doesn't seem to be a proper solution for all cases! And I already have another form with Non-Nullable string, that generates the same custom error message for both client & server sides validations, that's why I believe the issue is more related to the scalar values rather than Non/Nullable type options, but I can't figure out the reason, to be able to override that server-side validation message!
Any help would be appreciated…
.net ef-code-first asp.net-core-mvc data-annotations
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
As a side note, the answer you linked to is wrong. ADateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575
– user3559349
Nov 13 '18 at 22:18
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02
add a comment |
I have created a web application using .Net MVC Core, with Code First approach, and generated the Views/Controllers through scaffolding.
For validation, I'm using Fluent API and Data Annotations, and everything seems to be working just fine.
However my DataAnnotation's custom error messages, show up only on the client-side validation, and when I block my browser's JS to test my server-side validation, I get the following error message instead: "The value '' is invalid."
I tried to use [DataType(DataType.DateTime, ErrorMessage = "Invalid date!")] but it didn't work as well!
My Model:
public class MyModel
{
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Departure Date")]
public DateTime DateDeparture { get; set; }
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Arrival Date")]
public DateTime DateArrival { get; set; }
}
My View:
public IActionResult Create()
{
return View();
}
My Controller:
@model App.Models.MyModel
@{
ViewData["Title"] = "Create";
}
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="DateDeparture" class="control-label"></label>
<input asp-for="DateDeparture" class="form-control" />
<span asp-validation-for="DateDeparture" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="DateArrival" class="control-label"></label>
<input asp-for="DateArrival" class="form-control" />
<span asp-validation-for="DateArrival" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</form>
Client-Side Validation
Server-Side Validation
According to the suggested solution here for a similar scenario (Thread) I have to make my field nullable!
Which doesn't seem to be a proper solution for all cases! And I already have another form with Non-Nullable string, that generates the same custom error message for both client & server sides validations, that's why I believe the issue is more related to the scalar values rather than Non/Nullable type options, but I can't figure out the reason, to be able to override that server-side validation message!
Any help would be appreciated…
.net ef-code-first asp.net-core-mvc data-annotations
I have created a web application using .Net MVC Core, with Code First approach, and generated the Views/Controllers through scaffolding.
For validation, I'm using Fluent API and Data Annotations, and everything seems to be working just fine.
However my DataAnnotation's custom error messages, show up only on the client-side validation, and when I block my browser's JS to test my server-side validation, I get the following error message instead: "The value '' is invalid."
I tried to use [DataType(DataType.DateTime, ErrorMessage = "Invalid date!")] but it didn't work as well!
My Model:
public class MyModel
{
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Departure Date")]
public DateTime DateDeparture { get; set; }
[Required(ErrorMessage = "Required field!")]
[Display(Name = "Arrival Date")]
public DateTime DateArrival { get; set; }
}
My View:
public IActionResult Create()
{
return View();
}
My Controller:
@model App.Models.MyModel
@{
ViewData["Title"] = "Create";
}
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="DateDeparture" class="control-label"></label>
<input asp-for="DateDeparture" class="form-control" />
<span asp-validation-for="DateDeparture" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="DateArrival" class="control-label"></label>
<input asp-for="DateArrival" class="form-control" />
<span asp-validation-for="DateArrival" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</form>
Client-Side Validation
Server-Side Validation
According to the suggested solution here for a similar scenario (Thread) I have to make my field nullable!
Which doesn't seem to be a proper solution for all cases! And I already have another form with Non-Nullable string, that generates the same custom error message for both client & server sides validations, that's why I believe the issue is more related to the scalar values rather than Non/Nullable type options, but I can't figure out the reason, to be able to override that server-side validation message!
Any help would be appreciated…
.net ef-code-first asp.net-core-mvc data-annotations
.net ef-code-first asp.net-core-mvc data-annotations
edited Nov 14 '18 at 9:03
user3559349
asked Nov 13 '18 at 11:42
Odeh KhanfarOdeh Khanfar
12
12
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
As a side note, the answer you linked to is wrong. ADateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575
– user3559349
Nov 13 '18 at 22:18
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02
add a comment |
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
As a side note, the answer you linked to is wrong. ADateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575
– user3559349
Nov 13 '18 at 22:18
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
As a side note, the answer you linked to is wrong. A
DateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575– user3559349
Nov 13 '18 at 22:18
As a side note, the answer you linked to is wrong. A
DateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575– user3559349
Nov 13 '18 at 22:18
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280311%2fmvc-net-core-dataannotations-requirederrormessage-err-doesnt-show-up-on%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280311%2fmvc-net-core-dataannotations-requirederrormessage-err-doesnt-show-up-on%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
r 5 k6ZVZp1m0Xe,V,t ZVydYw,Zpd5aweEfOlh 424qmwTo fxOiK,4sPtvyntSP1C,L,vkZUzKNT9RmzLKCVT
Both your images are identical. Its not clear what you are asking
– user3559349
Nov 13 '18 at 22:15
As a side note, the answer you linked to is wrong. A
DateTime
is required by default (although its recommended that reference types be nullable in your view model to protect against under-posting attacks - refer https://stackoverflow.com/questions/43688968/what-does-it-mean-for-a-property-to-be-required-and-nullable/43689575#43689575– user3559349
Nov 13 '18 at 22:18
Updated.. Thank you @StephenMuecke
– Odeh Khanfar
Nov 14 '18 at 9:02