How to get the uri of an uploaded image
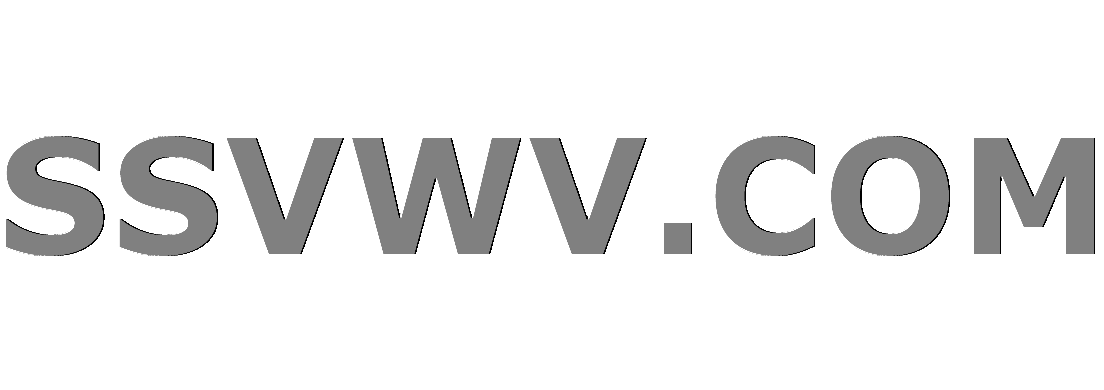
Multi tool use
I'm using Firebase Storage to create and send image url's to Firebase Storage Bucket. I have this working well when I have the upload right and a Firebase Storage config set. But, how do I grab the actual URI from the image and put it to be viewed? I've seen some possible answers to this in JS. But not react-native. Here's my code:
submitFormHandler = () => {
console.log("FormHandler: " + this.state.message)
let isFormValid = true;
let dataToSubmit = {};
const formCopy = this.state.form;
var user = firebase.auth().currentUser;
for (let key in formCopy) {
isFormValid = isFormValid && formCopy[key].valid;
dataToSubmit[key] = this.state.form[key].value;
}
if (isFormValid, user) {
this.setState({
loading: true
});
getTokens((value) => {
const dateNow = new Date();
const expiration = dateNow.getTime();
const form = {
...dataToSubmit,
uid: value[3][1]
}
if (expiration > value[2][1]) {
this.props.autoSignIn(value[1][1]).then(() => {
setTokens(this.props.User.userData, () => {
this.props.addArticle(form, this.props.User.userData.token).then(() => {
this.setState({ modalSuccess: true })
})
})
})
} else {
this.props.addArticle(form, value[0][1]).then(() => {
this.setState({ modalSuccess: true })
})
}
})
} else {
let errorsArray = ;
for (let key in formCopy) {
if (!formCopy[key].valid) {
errorsArray.push(formCopy[key].errorMsg)
}
}
this.setState({
loading: false,
upload: true,
hasErrors: true,
modalVisible: true,
errorsArray
})
}
}
showErrorsArray = (errors) => (
errors ?
errors.map((item, i) => (
<Text key={i} style={styles.errorItem}> - {item}</Text>
))
: null
)
clearErrors = () => {
this.setState({
hasErrors: false,
modalVisible: false,
errorsArray:
})
}
resetDenEventScreen = () => {
const formCopy = this.state.form;
for (let key in formCopy) {
formCopy[key].valid = false;
formCopy[key].value = "";
}
this.setState({
modalSuccess: false,
hasErrors: false,
errorsArray: ,
loading: false
})
this.props.resetArticle();
}
The image url is then passed to Firebase and sets it as a key of 'imageSource' and a value of '.jpg'. With the hardcoding, this works fine, but for only if i have a URI set for the image added from the user (or folder in Firebase Storage). I'm aware of how Firebase Realtime Database will assign it's own id number to an item as in:
pickImage = () => {
console.log('onPickImage');
this.setState({ imageLoading: true });
ImagePicker.showImagePicker(null, (response) => {
console.log('Response = ', response);
if (response.didCancel) {
console.log('User cancelled image picker');
this.setState({ imageLoading: false });
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
this.setState({ imageLoading: false });
} else {
const source = {
uri: response.uri,
type: response.type,
};
this.setState({ localImageSource: source.uri });
}
});
}
uploadImage(imageSource) {
console.log("starting upload image...");
return new Promise((resolve, reject) => {
const uploadUri = Platform.OS === 'ios' ? imageSource.uri.replace('file://', '') : imageSource.uri;
let uploadBlob = null;
const imageRef = firebase.storage().ref('images/').child(imageSource.uri)
fs.readFile(uploadUri, 'base64')
.then((data) => {
console.log("building blob...");
return Blob.build(data, { type: '${mime};BASE64' });
})
.then((blob) => {
uploadBlob = blob;
console.log("uploading blob...");
return imageRef.put(uploadUri, { contentType: imageSource.type });
})
.then(() => {
uploadBlob.close();
console.log("getting blob downloadUrl...");
return imageRef.getDownloadURL();
})
.then((url) => {
console.log('Image URL: ', url);
console.log("resolving...")
resolve(url);
})
.catch((error) => {
console.log("rejecting promise..." + error);
reject(error)
});
// this.handlePickedImage(pickerResult);
});
}
where item.key is generated by Firebase, thus it doesn't have to be hardcoded in. Is it possible to achieve this in Firebase Storage for both the image and the 'article'? And do I really need to assign new key to add an entry in Firebase storage since the mobile app only is uploading the url to the Firebase, and now i need to get the URI from the image.
handlePickedImage = pickerResult => {
try {
this.setState({ uploading: true });
if (!pickerResult.cancelled) {
uploadUrl = awaituploadImage(pickerResult.uri);
this.setState({ image: uploadUrl });
}
} catch (e) {
console.log(e);
alert('Upload failed, sorry : (');
} finally {
this.setState({ uploading: false });
}
};
getImageLocal() {
console.log('Attempting to get localImageSource: ' + this.state.localImageSource);
if (this.state.localImageSource == null) {
console.log("localImageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.localImageSource }} />
);
}
getImage() {
console.log('Attempting to get imageSource: ' + this.state.imageSource);
if (this.state.imageSource == null) {
console.log("imageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.imageSource }} />
);
}
I know i need put something like 'firebase.storage().ref().getDowloadUrl()' or it is more simple as adding 'form.image.value.getDownloadUrl()' and sorry for asking 'where'? Thanks, i'm new and look for many documentation, but i'm not realy understanding on who to put or some logic to work...
ios firebase react-native react-native-ios
add a comment |
I'm using Firebase Storage to create and send image url's to Firebase Storage Bucket. I have this working well when I have the upload right and a Firebase Storage config set. But, how do I grab the actual URI from the image and put it to be viewed? I've seen some possible answers to this in JS. But not react-native. Here's my code:
submitFormHandler = () => {
console.log("FormHandler: " + this.state.message)
let isFormValid = true;
let dataToSubmit = {};
const formCopy = this.state.form;
var user = firebase.auth().currentUser;
for (let key in formCopy) {
isFormValid = isFormValid && formCopy[key].valid;
dataToSubmit[key] = this.state.form[key].value;
}
if (isFormValid, user) {
this.setState({
loading: true
});
getTokens((value) => {
const dateNow = new Date();
const expiration = dateNow.getTime();
const form = {
...dataToSubmit,
uid: value[3][1]
}
if (expiration > value[2][1]) {
this.props.autoSignIn(value[1][1]).then(() => {
setTokens(this.props.User.userData, () => {
this.props.addArticle(form, this.props.User.userData.token).then(() => {
this.setState({ modalSuccess: true })
})
})
})
} else {
this.props.addArticle(form, value[0][1]).then(() => {
this.setState({ modalSuccess: true })
})
}
})
} else {
let errorsArray = ;
for (let key in formCopy) {
if (!formCopy[key].valid) {
errorsArray.push(formCopy[key].errorMsg)
}
}
this.setState({
loading: false,
upload: true,
hasErrors: true,
modalVisible: true,
errorsArray
})
}
}
showErrorsArray = (errors) => (
errors ?
errors.map((item, i) => (
<Text key={i} style={styles.errorItem}> - {item}</Text>
))
: null
)
clearErrors = () => {
this.setState({
hasErrors: false,
modalVisible: false,
errorsArray:
})
}
resetDenEventScreen = () => {
const formCopy = this.state.form;
for (let key in formCopy) {
formCopy[key].valid = false;
formCopy[key].value = "";
}
this.setState({
modalSuccess: false,
hasErrors: false,
errorsArray: ,
loading: false
})
this.props.resetArticle();
}
The image url is then passed to Firebase and sets it as a key of 'imageSource' and a value of '.jpg'. With the hardcoding, this works fine, but for only if i have a URI set for the image added from the user (or folder in Firebase Storage). I'm aware of how Firebase Realtime Database will assign it's own id number to an item as in:
pickImage = () => {
console.log('onPickImage');
this.setState({ imageLoading: true });
ImagePicker.showImagePicker(null, (response) => {
console.log('Response = ', response);
if (response.didCancel) {
console.log('User cancelled image picker');
this.setState({ imageLoading: false });
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
this.setState({ imageLoading: false });
} else {
const source = {
uri: response.uri,
type: response.type,
};
this.setState({ localImageSource: source.uri });
}
});
}
uploadImage(imageSource) {
console.log("starting upload image...");
return new Promise((resolve, reject) => {
const uploadUri = Platform.OS === 'ios' ? imageSource.uri.replace('file://', '') : imageSource.uri;
let uploadBlob = null;
const imageRef = firebase.storage().ref('images/').child(imageSource.uri)
fs.readFile(uploadUri, 'base64')
.then((data) => {
console.log("building blob...");
return Blob.build(data, { type: '${mime};BASE64' });
})
.then((blob) => {
uploadBlob = blob;
console.log("uploading blob...");
return imageRef.put(uploadUri, { contentType: imageSource.type });
})
.then(() => {
uploadBlob.close();
console.log("getting blob downloadUrl...");
return imageRef.getDownloadURL();
})
.then((url) => {
console.log('Image URL: ', url);
console.log("resolving...")
resolve(url);
})
.catch((error) => {
console.log("rejecting promise..." + error);
reject(error)
});
// this.handlePickedImage(pickerResult);
});
}
where item.key is generated by Firebase, thus it doesn't have to be hardcoded in. Is it possible to achieve this in Firebase Storage for both the image and the 'article'? And do I really need to assign new key to add an entry in Firebase storage since the mobile app only is uploading the url to the Firebase, and now i need to get the URI from the image.
handlePickedImage = pickerResult => {
try {
this.setState({ uploading: true });
if (!pickerResult.cancelled) {
uploadUrl = awaituploadImage(pickerResult.uri);
this.setState({ image: uploadUrl });
}
} catch (e) {
console.log(e);
alert('Upload failed, sorry : (');
} finally {
this.setState({ uploading: false });
}
};
getImageLocal() {
console.log('Attempting to get localImageSource: ' + this.state.localImageSource);
if (this.state.localImageSource == null) {
console.log("localImageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.localImageSource }} />
);
}
getImage() {
console.log('Attempting to get imageSource: ' + this.state.imageSource);
if (this.state.imageSource == null) {
console.log("imageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.imageSource }} />
);
}
I know i need put something like 'firebase.storage().ref().getDowloadUrl()' or it is more simple as adding 'form.image.value.getDownloadUrl()' and sorry for asking 'where'? Thanks, i'm new and look for many documentation, but i'm not realy understanding on who to put or some logic to work...
ios firebase react-native react-native-ios
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51
add a comment |
I'm using Firebase Storage to create and send image url's to Firebase Storage Bucket. I have this working well when I have the upload right and a Firebase Storage config set. But, how do I grab the actual URI from the image and put it to be viewed? I've seen some possible answers to this in JS. But not react-native. Here's my code:
submitFormHandler = () => {
console.log("FormHandler: " + this.state.message)
let isFormValid = true;
let dataToSubmit = {};
const formCopy = this.state.form;
var user = firebase.auth().currentUser;
for (let key in formCopy) {
isFormValid = isFormValid && formCopy[key].valid;
dataToSubmit[key] = this.state.form[key].value;
}
if (isFormValid, user) {
this.setState({
loading: true
});
getTokens((value) => {
const dateNow = new Date();
const expiration = dateNow.getTime();
const form = {
...dataToSubmit,
uid: value[3][1]
}
if (expiration > value[2][1]) {
this.props.autoSignIn(value[1][1]).then(() => {
setTokens(this.props.User.userData, () => {
this.props.addArticle(form, this.props.User.userData.token).then(() => {
this.setState({ modalSuccess: true })
})
})
})
} else {
this.props.addArticle(form, value[0][1]).then(() => {
this.setState({ modalSuccess: true })
})
}
})
} else {
let errorsArray = ;
for (let key in formCopy) {
if (!formCopy[key].valid) {
errorsArray.push(formCopy[key].errorMsg)
}
}
this.setState({
loading: false,
upload: true,
hasErrors: true,
modalVisible: true,
errorsArray
})
}
}
showErrorsArray = (errors) => (
errors ?
errors.map((item, i) => (
<Text key={i} style={styles.errorItem}> - {item}</Text>
))
: null
)
clearErrors = () => {
this.setState({
hasErrors: false,
modalVisible: false,
errorsArray:
})
}
resetDenEventScreen = () => {
const formCopy = this.state.form;
for (let key in formCopy) {
formCopy[key].valid = false;
formCopy[key].value = "";
}
this.setState({
modalSuccess: false,
hasErrors: false,
errorsArray: ,
loading: false
})
this.props.resetArticle();
}
The image url is then passed to Firebase and sets it as a key of 'imageSource' and a value of '.jpg'. With the hardcoding, this works fine, but for only if i have a URI set for the image added from the user (or folder in Firebase Storage). I'm aware of how Firebase Realtime Database will assign it's own id number to an item as in:
pickImage = () => {
console.log('onPickImage');
this.setState({ imageLoading: true });
ImagePicker.showImagePicker(null, (response) => {
console.log('Response = ', response);
if (response.didCancel) {
console.log('User cancelled image picker');
this.setState({ imageLoading: false });
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
this.setState({ imageLoading: false });
} else {
const source = {
uri: response.uri,
type: response.type,
};
this.setState({ localImageSource: source.uri });
}
});
}
uploadImage(imageSource) {
console.log("starting upload image...");
return new Promise((resolve, reject) => {
const uploadUri = Platform.OS === 'ios' ? imageSource.uri.replace('file://', '') : imageSource.uri;
let uploadBlob = null;
const imageRef = firebase.storage().ref('images/').child(imageSource.uri)
fs.readFile(uploadUri, 'base64')
.then((data) => {
console.log("building blob...");
return Blob.build(data, { type: '${mime};BASE64' });
})
.then((blob) => {
uploadBlob = blob;
console.log("uploading blob...");
return imageRef.put(uploadUri, { contentType: imageSource.type });
})
.then(() => {
uploadBlob.close();
console.log("getting blob downloadUrl...");
return imageRef.getDownloadURL();
})
.then((url) => {
console.log('Image URL: ', url);
console.log("resolving...")
resolve(url);
})
.catch((error) => {
console.log("rejecting promise..." + error);
reject(error)
});
// this.handlePickedImage(pickerResult);
});
}
where item.key is generated by Firebase, thus it doesn't have to be hardcoded in. Is it possible to achieve this in Firebase Storage for both the image and the 'article'? And do I really need to assign new key to add an entry in Firebase storage since the mobile app only is uploading the url to the Firebase, and now i need to get the URI from the image.
handlePickedImage = pickerResult => {
try {
this.setState({ uploading: true });
if (!pickerResult.cancelled) {
uploadUrl = awaituploadImage(pickerResult.uri);
this.setState({ image: uploadUrl });
}
} catch (e) {
console.log(e);
alert('Upload failed, sorry : (');
} finally {
this.setState({ uploading: false });
}
};
getImageLocal() {
console.log('Attempting to get localImageSource: ' + this.state.localImageSource);
if (this.state.localImageSource == null) {
console.log("localImageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.localImageSource }} />
);
}
getImage() {
console.log('Attempting to get imageSource: ' + this.state.imageSource);
if (this.state.imageSource == null) {
console.log("imageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.imageSource }} />
);
}
I know i need put something like 'firebase.storage().ref().getDowloadUrl()' or it is more simple as adding 'form.image.value.getDownloadUrl()' and sorry for asking 'where'? Thanks, i'm new and look for many documentation, but i'm not realy understanding on who to put or some logic to work...
ios firebase react-native react-native-ios
I'm using Firebase Storage to create and send image url's to Firebase Storage Bucket. I have this working well when I have the upload right and a Firebase Storage config set. But, how do I grab the actual URI from the image and put it to be viewed? I've seen some possible answers to this in JS. But not react-native. Here's my code:
submitFormHandler = () => {
console.log("FormHandler: " + this.state.message)
let isFormValid = true;
let dataToSubmit = {};
const formCopy = this.state.form;
var user = firebase.auth().currentUser;
for (let key in formCopy) {
isFormValid = isFormValid && formCopy[key].valid;
dataToSubmit[key] = this.state.form[key].value;
}
if (isFormValid, user) {
this.setState({
loading: true
});
getTokens((value) => {
const dateNow = new Date();
const expiration = dateNow.getTime();
const form = {
...dataToSubmit,
uid: value[3][1]
}
if (expiration > value[2][1]) {
this.props.autoSignIn(value[1][1]).then(() => {
setTokens(this.props.User.userData, () => {
this.props.addArticle(form, this.props.User.userData.token).then(() => {
this.setState({ modalSuccess: true })
})
})
})
} else {
this.props.addArticle(form, value[0][1]).then(() => {
this.setState({ modalSuccess: true })
})
}
})
} else {
let errorsArray = ;
for (let key in formCopy) {
if (!formCopy[key].valid) {
errorsArray.push(formCopy[key].errorMsg)
}
}
this.setState({
loading: false,
upload: true,
hasErrors: true,
modalVisible: true,
errorsArray
})
}
}
showErrorsArray = (errors) => (
errors ?
errors.map((item, i) => (
<Text key={i} style={styles.errorItem}> - {item}</Text>
))
: null
)
clearErrors = () => {
this.setState({
hasErrors: false,
modalVisible: false,
errorsArray:
})
}
resetDenEventScreen = () => {
const formCopy = this.state.form;
for (let key in formCopy) {
formCopy[key].valid = false;
formCopy[key].value = "";
}
this.setState({
modalSuccess: false,
hasErrors: false,
errorsArray: ,
loading: false
})
this.props.resetArticle();
}
The image url is then passed to Firebase and sets it as a key of 'imageSource' and a value of '.jpg'. With the hardcoding, this works fine, but for only if i have a URI set for the image added from the user (or folder in Firebase Storage). I'm aware of how Firebase Realtime Database will assign it's own id number to an item as in:
pickImage = () => {
console.log('onPickImage');
this.setState({ imageLoading: true });
ImagePicker.showImagePicker(null, (response) => {
console.log('Response = ', response);
if (response.didCancel) {
console.log('User cancelled image picker');
this.setState({ imageLoading: false });
} else if (response.error) {
console.log('ImagePicker Error: ', response.error);
this.setState({ imageLoading: false });
} else {
const source = {
uri: response.uri,
type: response.type,
};
this.setState({ localImageSource: source.uri });
}
});
}
uploadImage(imageSource) {
console.log("starting upload image...");
return new Promise((resolve, reject) => {
const uploadUri = Platform.OS === 'ios' ? imageSource.uri.replace('file://', '') : imageSource.uri;
let uploadBlob = null;
const imageRef = firebase.storage().ref('images/').child(imageSource.uri)
fs.readFile(uploadUri, 'base64')
.then((data) => {
console.log("building blob...");
return Blob.build(data, { type: '${mime};BASE64' });
})
.then((blob) => {
uploadBlob = blob;
console.log("uploading blob...");
return imageRef.put(uploadUri, { contentType: imageSource.type });
})
.then(() => {
uploadBlob.close();
console.log("getting blob downloadUrl...");
return imageRef.getDownloadURL();
})
.then((url) => {
console.log('Image URL: ', url);
console.log("resolving...")
resolve(url);
})
.catch((error) => {
console.log("rejecting promise..." + error);
reject(error)
});
// this.handlePickedImage(pickerResult);
});
}
where item.key is generated by Firebase, thus it doesn't have to be hardcoded in. Is it possible to achieve this in Firebase Storage for both the image and the 'article'? And do I really need to assign new key to add an entry in Firebase storage since the mobile app only is uploading the url to the Firebase, and now i need to get the URI from the image.
handlePickedImage = pickerResult => {
try {
this.setState({ uploading: true });
if (!pickerResult.cancelled) {
uploadUrl = awaituploadImage(pickerResult.uri);
this.setState({ image: uploadUrl });
}
} catch (e) {
console.log(e);
alert('Upload failed, sorry : (');
} finally {
this.setState({ uploading: false });
}
};
getImageLocal() {
console.log('Attempting to get localImageSource: ' + this.state.localImageSource);
if (this.state.localImageSource == null) {
console.log("localImageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.localImageSource }} />
);
}
getImage() {
console.log('Attempting to get imageSource: ' + this.state.imageSource);
if (this.state.imageSource == null) {
console.log("imageSource was not available, aborting...");
return;
}
return (
<Image
style={styles.imageStyle}
source={{ uri: this.state.imageSource }} />
);
}
I know i need put something like 'firebase.storage().ref().getDowloadUrl()' or it is more simple as adding 'form.image.value.getDownloadUrl()' and sorry for asking 'where'? Thanks, i'm new and look for many documentation, but i'm not realy understanding on who to put or some logic to work...
ios firebase react-native react-native-ios
ios firebase react-native react-native-ios
asked Nov 19 '18 at 15:23
J. LopesJ. Lopes
12
12
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51
add a comment |
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377751%2fhow-to-get-the-uri-of-an-uploaded-image%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377751%2fhow-to-get-the-uri-of-an-uploaded-image%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ij61cGbVSHB,0y n 3n Nn0nUQiUml
I must have a lot unnecessary lines in this hardcoded, but i'm already tired out...
– J. Lopes
Nov 19 '18 at 15:51