jupyter notebook vs jupyter console: display of markdown (and latex, html, etc) objects
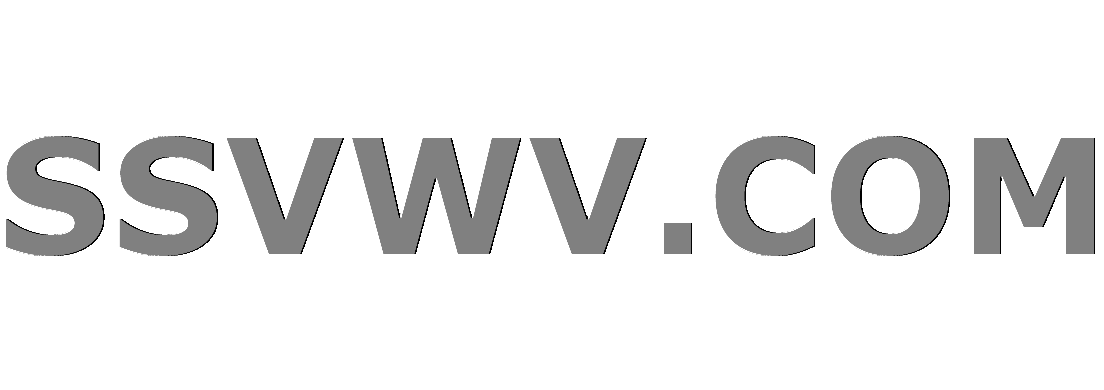
Multi tool use
i would like to be able to run a jupyter notebook as a regular python file (using a standard python interpreter) as
well. the problem i'm facing is that in python i can not render markdown objects in a usable from:
running the code below renders as it should in the notebook but prints <IPython.core.display.Markdown object>
in the
when run using just python.
from IPython.display import Markdown, display
display(Markdown('# Hello World!'))
i was trying to come up with a way to make that work and found this ugly work-around:
from IPython.display import Markdown, display
from IPython import get_ipython
from IPython.core.displaypub import DisplayPublisher
from ipykernel.zmqshell import ZMQDisplayPublisher
display_pub_class = get_ipython().display_pub_class()
def displaymd(strg):
if isinstance(display_pub_class, ZMQDisplayPublisher):
display(Markdown(strg))
elif isinstance(display_pub_class, DisplayPublisher):
print(strg)
else:
# ??
display(strg)
displaymd('# Hello World!')
that seems very hacky! is there a simpler way to get a reasonable display
of markdown objects? or at least a simpler way to know whether display
is capable of rendering markdown?
the same question goes for latex, html and similar objects.
just found out a silghtly simpler way to check if i am on ipython:
def on_ipython():
if 'get_ipython' in globals():
return True
else:
return False
def displaymd(strg):
if on_ipython():
display(Markdown(strg))
else:
print(strg)
still this is not very nice...
python python-3.x jupyter-notebook jupyter sage
add a comment |
i would like to be able to run a jupyter notebook as a regular python file (using a standard python interpreter) as
well. the problem i'm facing is that in python i can not render markdown objects in a usable from:
running the code below renders as it should in the notebook but prints <IPython.core.display.Markdown object>
in the
when run using just python.
from IPython.display import Markdown, display
display(Markdown('# Hello World!'))
i was trying to come up with a way to make that work and found this ugly work-around:
from IPython.display import Markdown, display
from IPython import get_ipython
from IPython.core.displaypub import DisplayPublisher
from ipykernel.zmqshell import ZMQDisplayPublisher
display_pub_class = get_ipython().display_pub_class()
def displaymd(strg):
if isinstance(display_pub_class, ZMQDisplayPublisher):
display(Markdown(strg))
elif isinstance(display_pub_class, DisplayPublisher):
print(strg)
else:
# ??
display(strg)
displaymd('# Hello World!')
that seems very hacky! is there a simpler way to get a reasonable display
of markdown objects? or at least a simpler way to know whether display
is capable of rendering markdown?
the same question goes for latex, html and similar objects.
just found out a silghtly simpler way to check if i am on ipython:
def on_ipython():
if 'get_ipython' in globals():
return True
else:
return False
def displaymd(strg):
if on_ipython():
display(Markdown(strg))
else:
print(strg)
still this is not very nice...
python python-3.x jupyter-notebook jupyter sage
add a comment |
i would like to be able to run a jupyter notebook as a regular python file (using a standard python interpreter) as
well. the problem i'm facing is that in python i can not render markdown objects in a usable from:
running the code below renders as it should in the notebook but prints <IPython.core.display.Markdown object>
in the
when run using just python.
from IPython.display import Markdown, display
display(Markdown('# Hello World!'))
i was trying to come up with a way to make that work and found this ugly work-around:
from IPython.display import Markdown, display
from IPython import get_ipython
from IPython.core.displaypub import DisplayPublisher
from ipykernel.zmqshell import ZMQDisplayPublisher
display_pub_class = get_ipython().display_pub_class()
def displaymd(strg):
if isinstance(display_pub_class, ZMQDisplayPublisher):
display(Markdown(strg))
elif isinstance(display_pub_class, DisplayPublisher):
print(strg)
else:
# ??
display(strg)
displaymd('# Hello World!')
that seems very hacky! is there a simpler way to get a reasonable display
of markdown objects? or at least a simpler way to know whether display
is capable of rendering markdown?
the same question goes for latex, html and similar objects.
just found out a silghtly simpler way to check if i am on ipython:
def on_ipython():
if 'get_ipython' in globals():
return True
else:
return False
def displaymd(strg):
if on_ipython():
display(Markdown(strg))
else:
print(strg)
still this is not very nice...
python python-3.x jupyter-notebook jupyter sage
i would like to be able to run a jupyter notebook as a regular python file (using a standard python interpreter) as
well. the problem i'm facing is that in python i can not render markdown objects in a usable from:
running the code below renders as it should in the notebook but prints <IPython.core.display.Markdown object>
in the
when run using just python.
from IPython.display import Markdown, display
display(Markdown('# Hello World!'))
i was trying to come up with a way to make that work and found this ugly work-around:
from IPython.display import Markdown, display
from IPython import get_ipython
from IPython.core.displaypub import DisplayPublisher
from ipykernel.zmqshell import ZMQDisplayPublisher
display_pub_class = get_ipython().display_pub_class()
def displaymd(strg):
if isinstance(display_pub_class, ZMQDisplayPublisher):
display(Markdown(strg))
elif isinstance(display_pub_class, DisplayPublisher):
print(strg)
else:
# ??
display(strg)
displaymd('# Hello World!')
that seems very hacky! is there a simpler way to get a reasonable display
of markdown objects? or at least a simpler way to know whether display
is capable of rendering markdown?
the same question goes for latex, html and similar objects.
just found out a silghtly simpler way to check if i am on ipython:
def on_ipython():
if 'get_ipython' in globals():
return True
else:
return False
def displaymd(strg):
if on_ipython():
display(Markdown(strg))
else:
print(strg)
still this is not very nice...
python python-3.x jupyter-notebook jupyter sage
python python-3.x jupyter-notebook jupyter sage
edited Nov 19 '18 at 15:40
hiro protagonist
asked Nov 19 '18 at 15:26


hiro protagonisthiro protagonist
18.6k64060
18.6k64060
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Option 1: dicts with both 'text/plain' and 'text/markdown' entries
You can pass a dict containing different MIME types to IPython's display(..., raw=True)
: Jupyter Notebook will use the rich representation, and an IPython or plain Python frontend will fall back to the text/plain
representation.
Here's a minimal complete example; try running it in an IPython terminal and in a Jupyter notebook, and you'll see it renders properly in both.
from IPython.display import display
my_markdown_string = '''
# Heading one
This is
* a
* list
'''
display({'text/plain': my_markdown_string,
'text/markdown': my_markdown_string},
raw=True)
Option 2: Define a custom text/plain formatter for objects of class Markdown
Example is based on the 'define a new int
formatter' example from the IPython display
docs. You'll want to run it in IPython to see its effect.
from IPython.display import display, Markdown
def md_formatter(md, pp, cycle):
pp.text(md.data)
text_plain = get_ipython().display_formatter.formatters['text/plain']
text_plain.for_type(Markdown, md_formatter)
display(Markdown('x **x** x'))
# x **x** x
del text_plain.type_printers[Markdown]
display(Markdown('x **x** x'))
# <IPython.core.display.Markdown object>
Appendix: list of MIME types Jupyter/IPython knows about
Taken from the DisplayFormatter docs:
See the
display_data
message in the messaging documentation for
more details about this message type.
The following MIME types are currently implemented:
- text/plain
- text/html
- text/markdown
- text/latex
- application/json
- application/javascript
- image/png
- image/jpeg
- image/svg+xml
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377801%2fjupyter-notebook-vs-jupyter-console-display-of-markdown-and-latex-html-etc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Option 1: dicts with both 'text/plain' and 'text/markdown' entries
You can pass a dict containing different MIME types to IPython's display(..., raw=True)
: Jupyter Notebook will use the rich representation, and an IPython or plain Python frontend will fall back to the text/plain
representation.
Here's a minimal complete example; try running it in an IPython terminal and in a Jupyter notebook, and you'll see it renders properly in both.
from IPython.display import display
my_markdown_string = '''
# Heading one
This is
* a
* list
'''
display({'text/plain': my_markdown_string,
'text/markdown': my_markdown_string},
raw=True)
Option 2: Define a custom text/plain formatter for objects of class Markdown
Example is based on the 'define a new int
formatter' example from the IPython display
docs. You'll want to run it in IPython to see its effect.
from IPython.display import display, Markdown
def md_formatter(md, pp, cycle):
pp.text(md.data)
text_plain = get_ipython().display_formatter.formatters['text/plain']
text_plain.for_type(Markdown, md_formatter)
display(Markdown('x **x** x'))
# x **x** x
del text_plain.type_printers[Markdown]
display(Markdown('x **x** x'))
# <IPython.core.display.Markdown object>
Appendix: list of MIME types Jupyter/IPython knows about
Taken from the DisplayFormatter docs:
See the
display_data
message in the messaging documentation for
more details about this message type.
The following MIME types are currently implemented:
- text/plain
- text/html
- text/markdown
- text/latex
- application/json
- application/javascript
- image/png
- image/jpeg
- image/svg+xml
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
add a comment |
Option 1: dicts with both 'text/plain' and 'text/markdown' entries
You can pass a dict containing different MIME types to IPython's display(..., raw=True)
: Jupyter Notebook will use the rich representation, and an IPython or plain Python frontend will fall back to the text/plain
representation.
Here's a minimal complete example; try running it in an IPython terminal and in a Jupyter notebook, and you'll see it renders properly in both.
from IPython.display import display
my_markdown_string = '''
# Heading one
This is
* a
* list
'''
display({'text/plain': my_markdown_string,
'text/markdown': my_markdown_string},
raw=True)
Option 2: Define a custom text/plain formatter for objects of class Markdown
Example is based on the 'define a new int
formatter' example from the IPython display
docs. You'll want to run it in IPython to see its effect.
from IPython.display import display, Markdown
def md_formatter(md, pp, cycle):
pp.text(md.data)
text_plain = get_ipython().display_formatter.formatters['text/plain']
text_plain.for_type(Markdown, md_formatter)
display(Markdown('x **x** x'))
# x **x** x
del text_plain.type_printers[Markdown]
display(Markdown('x **x** x'))
# <IPython.core.display.Markdown object>
Appendix: list of MIME types Jupyter/IPython knows about
Taken from the DisplayFormatter docs:
See the
display_data
message in the messaging documentation for
more details about this message type.
The following MIME types are currently implemented:
- text/plain
- text/html
- text/markdown
- text/latex
- application/json
- application/javascript
- image/png
- image/jpeg
- image/svg+xml
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
add a comment |
Option 1: dicts with both 'text/plain' and 'text/markdown' entries
You can pass a dict containing different MIME types to IPython's display(..., raw=True)
: Jupyter Notebook will use the rich representation, and an IPython or plain Python frontend will fall back to the text/plain
representation.
Here's a minimal complete example; try running it in an IPython terminal and in a Jupyter notebook, and you'll see it renders properly in both.
from IPython.display import display
my_markdown_string = '''
# Heading one
This is
* a
* list
'''
display({'text/plain': my_markdown_string,
'text/markdown': my_markdown_string},
raw=True)
Option 2: Define a custom text/plain formatter for objects of class Markdown
Example is based on the 'define a new int
formatter' example from the IPython display
docs. You'll want to run it in IPython to see its effect.
from IPython.display import display, Markdown
def md_formatter(md, pp, cycle):
pp.text(md.data)
text_plain = get_ipython().display_formatter.formatters['text/plain']
text_plain.for_type(Markdown, md_formatter)
display(Markdown('x **x** x'))
# x **x** x
del text_plain.type_printers[Markdown]
display(Markdown('x **x** x'))
# <IPython.core.display.Markdown object>
Appendix: list of MIME types Jupyter/IPython knows about
Taken from the DisplayFormatter docs:
See the
display_data
message in the messaging documentation for
more details about this message type.
The following MIME types are currently implemented:
- text/plain
- text/html
- text/markdown
- text/latex
- application/json
- application/javascript
- image/png
- image/jpeg
- image/svg+xml
Option 1: dicts with both 'text/plain' and 'text/markdown' entries
You can pass a dict containing different MIME types to IPython's display(..., raw=True)
: Jupyter Notebook will use the rich representation, and an IPython or plain Python frontend will fall back to the text/plain
representation.
Here's a minimal complete example; try running it in an IPython terminal and in a Jupyter notebook, and you'll see it renders properly in both.
from IPython.display import display
my_markdown_string = '''
# Heading one
This is
* a
* list
'''
display({'text/plain': my_markdown_string,
'text/markdown': my_markdown_string},
raw=True)
Option 2: Define a custom text/plain formatter for objects of class Markdown
Example is based on the 'define a new int
formatter' example from the IPython display
docs. You'll want to run it in IPython to see its effect.
from IPython.display import display, Markdown
def md_formatter(md, pp, cycle):
pp.text(md.data)
text_plain = get_ipython().display_formatter.formatters['text/plain']
text_plain.for_type(Markdown, md_formatter)
display(Markdown('x **x** x'))
# x **x** x
del text_plain.type_printers[Markdown]
display(Markdown('x **x** x'))
# <IPython.core.display.Markdown object>
Appendix: list of MIME types Jupyter/IPython knows about
Taken from the DisplayFormatter docs:
See the
display_data
message in the messaging documentation for
more details about this message type.
The following MIME types are currently implemented:
- text/plain
- text/html
- text/markdown
- text/latex
- application/json
- application/javascript
- image/png
- image/jpeg
- image/svg+xml
edited Dec 21 '18 at 22:20
answered Dec 21 '18 at 16:59
EsteisEsteis
1,47921730
1,47921730
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
add a comment |
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
that looks promising! will try that. thanks!
– hiro protagonist
Dec 21 '18 at 19:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377801%2fjupyter-notebook-vs-jupyter-console-display-of-markdown-and-latex-html-etc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1feEN4,RAgDZOVIEC btVS6V,zVvv,SQvFmtB9L,d,YZqFMwRc t NnZLVxk,O,n0wIyHGJdt 68 CwFvlCucX,39,9