Databinding to object - How to cancel datasource changes
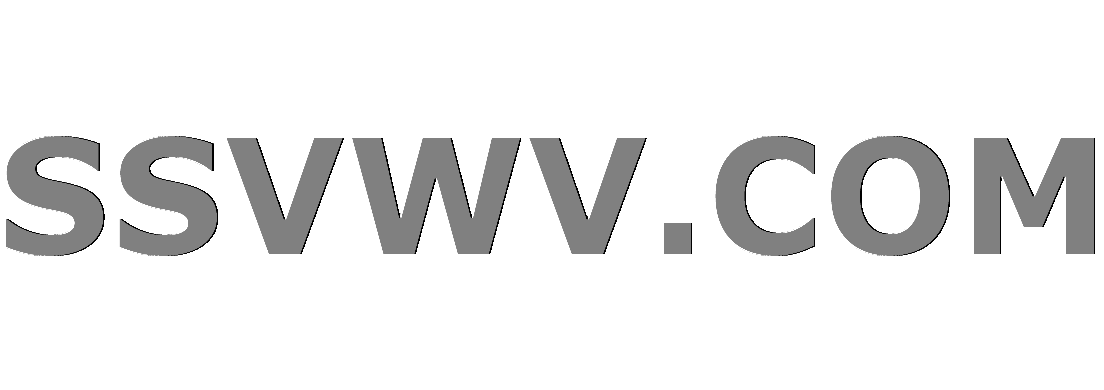
Multi tool use
Here is the scenario:
I have an Edit Dialog form with a BindingSource
and some data bound text boxes on it:
I pass an entity to the form constructor and it gets loaded into BindingSource.DataSource
which causes the data bound controls to show the values of properties.
The problem is as the user edits the values in TextBox
controls and Validating
events get passed, the data source gets changed though it is not applying to DB but it still can confuses the user as he sees the edited values on the List Form, till next application restart.
so the question is: How to prevent binding source from reflecting changes instantly or how to roll them back?
I inherited the binding source and created a new binding source like this:
public class SuperBindingSource:BindingSource
{
#region Properties
public object DataSourceBeforeChange { get; private set; }
#endregion
#region Methods
public void ResetChanges()
{
this.DataSource = this.DataSourceBeforeChange;
}
#endregion
protected override void OnDataSourceChanged(EventArgs e)
{
base.OnDataSourceChanged(e);
DataSourceBeforeChange=this.DataSource.DeepClone();
}
}
Though I am not sure if it is a good approach.
c# .net winforms data-binding bindingsource
add a comment |
Here is the scenario:
I have an Edit Dialog form with a BindingSource
and some data bound text boxes on it:
I pass an entity to the form constructor and it gets loaded into BindingSource.DataSource
which causes the data bound controls to show the values of properties.
The problem is as the user edits the values in TextBox
controls and Validating
events get passed, the data source gets changed though it is not applying to DB but it still can confuses the user as he sees the edited values on the List Form, till next application restart.
so the question is: How to prevent binding source from reflecting changes instantly or how to roll them back?
I inherited the binding source and created a new binding source like this:
public class SuperBindingSource:BindingSource
{
#region Properties
public object DataSourceBeforeChange { get; private set; }
#endregion
#region Methods
public void ResetChanges()
{
this.DataSource = this.DataSourceBeforeChange;
}
#endregion
protected override void OnDataSourceChanged(EventArgs e)
{
base.OnDataSourceChanged(e);
DataSourceBeforeChange=this.DataSource.DeepClone();
}
}
Though I am not sure if it is a good approach.
c# .net winforms data-binding bindingsource
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18
add a comment |
Here is the scenario:
I have an Edit Dialog form with a BindingSource
and some data bound text boxes on it:
I pass an entity to the form constructor and it gets loaded into BindingSource.DataSource
which causes the data bound controls to show the values of properties.
The problem is as the user edits the values in TextBox
controls and Validating
events get passed, the data source gets changed though it is not applying to DB but it still can confuses the user as he sees the edited values on the List Form, till next application restart.
so the question is: How to prevent binding source from reflecting changes instantly or how to roll them back?
I inherited the binding source and created a new binding source like this:
public class SuperBindingSource:BindingSource
{
#region Properties
public object DataSourceBeforeChange { get; private set; }
#endregion
#region Methods
public void ResetChanges()
{
this.DataSource = this.DataSourceBeforeChange;
}
#endregion
protected override void OnDataSourceChanged(EventArgs e)
{
base.OnDataSourceChanged(e);
DataSourceBeforeChange=this.DataSource.DeepClone();
}
}
Though I am not sure if it is a good approach.
c# .net winforms data-binding bindingsource
Here is the scenario:
I have an Edit Dialog form with a BindingSource
and some data bound text boxes on it:
I pass an entity to the form constructor and it gets loaded into BindingSource.DataSource
which causes the data bound controls to show the values of properties.
The problem is as the user edits the values in TextBox
controls and Validating
events get passed, the data source gets changed though it is not applying to DB but it still can confuses the user as he sees the edited values on the List Form, till next application restart.
so the question is: How to prevent binding source from reflecting changes instantly or how to roll them back?
I inherited the binding source and created a new binding source like this:
public class SuperBindingSource:BindingSource
{
#region Properties
public object DataSourceBeforeChange { get; private set; }
#endregion
#region Methods
public void ResetChanges()
{
this.DataSource = this.DataSourceBeforeChange;
}
#endregion
protected override void OnDataSourceChanged(EventArgs e)
{
base.OnDataSourceChanged(e);
DataSourceBeforeChange=this.DataSource.DeepClone();
}
}
Though I am not sure if it is a good approach.
c# .net winforms data-binding bindingsource
c# .net winforms data-binding bindingsource
edited Nov 21 '18 at 9:27
Reza Aghaei
68k857171
68k857171
asked Feb 6 '18 at 9:14


Sabouei AlirezaSabouei Alireza
16118
16118
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18
add a comment |
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18
add a comment |
2 Answers
2
active
oldest
votes
As an option, when setting up data-bindings, you can set them to update data source never.
Then at the point that you want to apply changes, for example when pressing OK
button, you can set data-bindings to update data source on property change and then call end edit method of the binding source.
For Cancel
button, you don't need to do anything, because the data source is not updated.
Example
In form load event:
this.BindingContext[bindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b=>b.DataSourceUpdateMode= DataSourceUpdateMode.Never);
When pressing OK:
this.BindingContext[productBindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b => b.DataSourceUpdateMode = DataSourceUpdateMode.OnPropertyChanged);
productBindingSource.EndEdit();
You can download/clone the full source code:
- r-aghaei/SuspendDataBindingExample
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standardBindingSource
.
– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is aClass
and classes are reference type, so resettingthis.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.
– Reza Aghaei
Feb 6 '18 at 11:56
|
show 6 more comments
You can use the SuspendBinding
method after the values are loaded.
After that the values should not update the source until you call ResumeBinding
:
SuspendBinding and ResumeBinding are two methods that allow the temporary suspension and resumption of data binding in a simple-binding scenario. You would typically suspend data binding if the user must be allowed to make several edits to data fields before validation occurs. For example, if one field must be changed in accordance with a second, but where validating the first field would cause the second field to be in error.
According to the documentation you should be able to use this with your textboxes. If the user clicks Ok
to save the values you resume the binding and if he cancels you don't.
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f48639312%2fdatabinding-to-object-how-to-cancel-datasource-changes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As an option, when setting up data-bindings, you can set them to update data source never.
Then at the point that you want to apply changes, for example when pressing OK
button, you can set data-bindings to update data source on property change and then call end edit method of the binding source.
For Cancel
button, you don't need to do anything, because the data source is not updated.
Example
In form load event:
this.BindingContext[bindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b=>b.DataSourceUpdateMode= DataSourceUpdateMode.Never);
When pressing OK:
this.BindingContext[productBindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b => b.DataSourceUpdateMode = DataSourceUpdateMode.OnPropertyChanged);
productBindingSource.EndEdit();
You can download/clone the full source code:
- r-aghaei/SuspendDataBindingExample
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standardBindingSource
.
– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is aClass
and classes are reference type, so resettingthis.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.
– Reza Aghaei
Feb 6 '18 at 11:56
|
show 6 more comments
As an option, when setting up data-bindings, you can set them to update data source never.
Then at the point that you want to apply changes, for example when pressing OK
button, you can set data-bindings to update data source on property change and then call end edit method of the binding source.
For Cancel
button, you don't need to do anything, because the data source is not updated.
Example
In form load event:
this.BindingContext[bindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b=>b.DataSourceUpdateMode= DataSourceUpdateMode.Never);
When pressing OK:
this.BindingContext[productBindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b => b.DataSourceUpdateMode = DataSourceUpdateMode.OnPropertyChanged);
productBindingSource.EndEdit();
You can download/clone the full source code:
- r-aghaei/SuspendDataBindingExample
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standardBindingSource
.
– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is aClass
and classes are reference type, so resettingthis.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.
– Reza Aghaei
Feb 6 '18 at 11:56
|
show 6 more comments
As an option, when setting up data-bindings, you can set them to update data source never.
Then at the point that you want to apply changes, for example when pressing OK
button, you can set data-bindings to update data source on property change and then call end edit method of the binding source.
For Cancel
button, you don't need to do anything, because the data source is not updated.
Example
In form load event:
this.BindingContext[bindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b=>b.DataSourceUpdateMode= DataSourceUpdateMode.Never);
When pressing OK:
this.BindingContext[productBindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b => b.DataSourceUpdateMode = DataSourceUpdateMode.OnPropertyChanged);
productBindingSource.EndEdit();
You can download/clone the full source code:
- r-aghaei/SuspendDataBindingExample
As an option, when setting up data-bindings, you can set them to update data source never.
Then at the point that you want to apply changes, for example when pressing OK
button, you can set data-bindings to update data source on property change and then call end edit method of the binding source.
For Cancel
button, you don't need to do anything, because the data source is not updated.
Example
In form load event:
this.BindingContext[bindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b=>b.DataSourceUpdateMode= DataSourceUpdateMode.Never);
When pressing OK:
this.BindingContext[productBindingSource].Bindings.Cast<Binding>().ToList()
.ForEach(b => b.DataSourceUpdateMode = DataSourceUpdateMode.OnPropertyChanged);
productBindingSource.EndEdit();
You can download/clone the full source code:
- r-aghaei/SuspendDataBindingExample
edited Feb 6 '18 at 15:51
answered Feb 6 '18 at 10:02
Reza AghaeiReza Aghaei
68k857171
68k857171
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standardBindingSource
.
– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is aClass
and classes are reference type, so resettingthis.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.
– Reza Aghaei
Feb 6 '18 at 11:56
|
show 6 more comments
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standardBindingSource
.
– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is aClass
and classes are reference type, so resettingthis.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.
– Reza Aghaei
Feb 6 '18 at 11:56
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
Thanks so much for the answer. Well isn't there a cleaner way to do it? The thing is if I wanna do it, it would be better if I go manual approch and set controls one by one. like :txtname.text=myObject.name etc. Actually I am looking for a cleaner approach.
– Sabouei Alireza
Feb 6 '18 at 10:25
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
You don't need to do anything manually. You will use data-binding to show data in controls, but the updates will not be written back to data-source until you press OK button.
– Reza Aghaei
Feb 6 '18 at 10:39
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standard
BindingSource
.– Reza Aghaei
Feb 6 '18 at 11:00
In fact, with the new update which I added to the answer, you just need to add those code to form load event and click event of the OK button, without any change in your existing code. Just use a standard
BindingSource
.– Reza Aghaei
Feb 6 '18 at 11:00
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Thank u so much. I marked Your answer as correct. I have a final question. Which approach is cleaner in your idea? mine or yours?
– Sabouei Alireza
Feb 6 '18 at 11:41
Just one point about your solution,
this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is a Class
and classes are reference type, so resetting this.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.– Reza Aghaei
Feb 6 '18 at 11:56
Just one point about your solution,
this.DataSource = this.DataSourceBeforeChange
, it seems it has a pitfall. The entity which you are editing is a Class
and classes are reference type, so resetting this.DataSource
to a new instance of object doesn't mean you reset changes. The instance is edited. You just decided to put another instance in data source. Take a look at this question.– Reza Aghaei
Feb 6 '18 at 11:56
|
show 6 more comments
You can use the SuspendBinding
method after the values are loaded.
After that the values should not update the source until you call ResumeBinding
:
SuspendBinding and ResumeBinding are two methods that allow the temporary suspension and resumption of data binding in a simple-binding scenario. You would typically suspend data binding if the user must be allowed to make several edits to data fields before validation occurs. For example, if one field must be changed in accordance with a second, but where validating the first field would cause the second field to be in error.
According to the documentation you should be able to use this with your textboxes. If the user clicks Ok
to save the values you resume the binding and if he cancels you don't.
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
add a comment |
You can use the SuspendBinding
method after the values are loaded.
After that the values should not update the source until you call ResumeBinding
:
SuspendBinding and ResumeBinding are two methods that allow the temporary suspension and resumption of data binding in a simple-binding scenario. You would typically suspend data binding if the user must be allowed to make several edits to data fields before validation occurs. For example, if one field must be changed in accordance with a second, but where validating the first field would cause the second field to be in error.
According to the documentation you should be able to use this with your textboxes. If the user clicks Ok
to save the values you resume the binding and if he cancels you don't.
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
add a comment |
You can use the SuspendBinding
method after the values are loaded.
After that the values should not update the source until you call ResumeBinding
:
SuspendBinding and ResumeBinding are two methods that allow the temporary suspension and resumption of data binding in a simple-binding scenario. You would typically suspend data binding if the user must be allowed to make several edits to data fields before validation occurs. For example, if one field must be changed in accordance with a second, but where validating the first field would cause the second field to be in error.
According to the documentation you should be able to use this with your textboxes. If the user clicks Ok
to save the values you resume the binding and if he cancels you don't.
You can use the SuspendBinding
method after the values are loaded.
After that the values should not update the source until you call ResumeBinding
:
SuspendBinding and ResumeBinding are two methods that allow the temporary suspension and resumption of data binding in a simple-binding scenario. You would typically suspend data binding if the user must be allowed to make several edits to data fields before validation occurs. For example, if one field must be changed in accordance with a second, but where validating the first field would cause the second field to be in error.
According to the documentation you should be able to use this with your textboxes. If the user clicks Ok
to save the values you resume the binding and if he cancels you don't.
edited Feb 6 '18 at 9:26
answered Feb 6 '18 at 9:22
ChrFinChrFin
17.8k75391
17.8k75391
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
add a comment |
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
Thanks for the help. I used SuspendBinding but it clears the loaded values from textboxes. Am i using it in a wrong way?
– Sabouei Alireza
Feb 6 '18 at 9:23
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
@SaboueiAlireza Are you sure it "clears the values" and you are not simply calling the suspend to early? Because the method should not clear any values according to the documentation...
– ChrFin
Feb 6 '18 at 9:24
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
I put a button on the form which calls the Suspend method. The form loads the data perfectly but as soon as I press the button, it clears all the textboxes.
– Sabouei Alireza
Feb 6 '18 at 9:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f48639312%2fdatabinding-to-object-how-to-cancel-datasource-changes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VkwzVfwgNu7by ZN7,skUFXJGWbF5YBOdC0KnP 8w5X,O,IAkex b,fZ1NgkjPlI,A,85tKbYZF7mqPujr,lh9Z3vd
DeepClone() is coming from DeepCloner Nuget package which creates a clone.
– Sabouei Alireza
Feb 6 '18 at 9:18