Disable a part of Java Program during JUnit testing
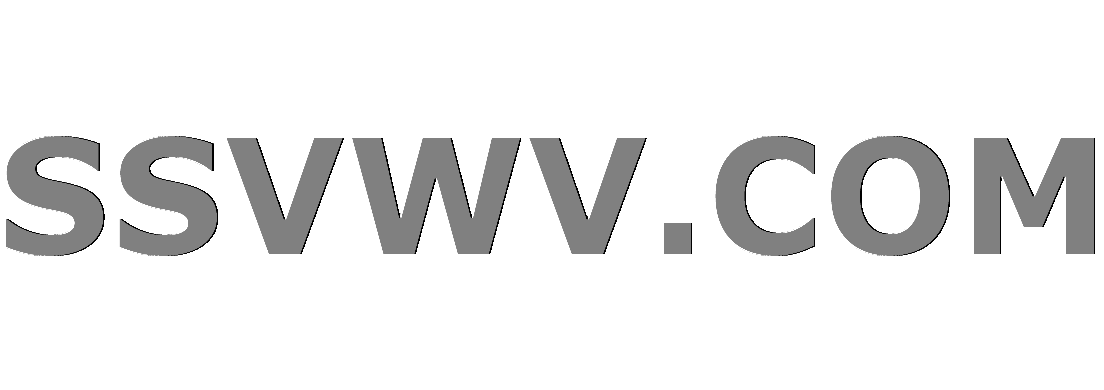
Multi tool use
I am testing my Java program with JUnit.
The program includes some JavaFX GUI interface and other logging code. However, during testing, I don't want these to be tested. Is there any way to switch the code between testing and development?
The description can be abstract, I'll use an example:
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
}
In this example, if I only want to test the correct number of greetingCnt but not want to have anything printed or any extra computation to be performed. But during actual program execution, there is no influence on the function of the program. May I know if there is any way to do it?
Thank you!
java unit-testing testing junit
add a comment |
I am testing my Java program with JUnit.
The program includes some JavaFX GUI interface and other logging code. However, during testing, I don't want these to be tested. Is there any way to switch the code between testing and development?
The description can be abstract, I'll use an example:
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
}
In this example, if I only want to test the correct number of greetingCnt but not want to have anything printed or any extra computation to be performed. But during actual program execution, there is no influence on the function of the program. May I know if there is any way to do it?
Thank you!
java unit-testing testing junit
1
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
1
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17
add a comment |
I am testing my Java program with JUnit.
The program includes some JavaFX GUI interface and other logging code. However, during testing, I don't want these to be tested. Is there any way to switch the code between testing and development?
The description can be abstract, I'll use an example:
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
}
In this example, if I only want to test the correct number of greetingCnt but not want to have anything printed or any extra computation to be performed. But during actual program execution, there is no influence on the function of the program. May I know if there is any way to do it?
Thank you!
java unit-testing testing junit
I am testing my Java program with JUnit.
The program includes some JavaFX GUI interface and other logging code. However, during testing, I don't want these to be tested. Is there any way to switch the code between testing and development?
The description can be abstract, I'll use an example:
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
}
In this example, if I only want to test the correct number of greetingCnt but not want to have anything printed or any extra computation to be performed. But during actual program execution, there is no influence on the function of the program. May I know if there is any way to do it?
Thank you!
java unit-testing testing junit
java unit-testing testing junit
edited Nov 21 '18 at 9:33


Federico klez Culloca
16k134380
16k134380
asked Nov 21 '18 at 9:21
EugeneHEugeneH
63
63
1
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
1
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17
add a comment |
1
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
1
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17
1
1
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
1
1
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17
add a comment |
3 Answers
3
active
oldest
votes
It is impossible without additional effort from you.
Computer simply have no way to tell which computation affect what.
You can turn off specific features like printing to console by replacing default PrintWriter
, but there is no generic solution.
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code withif
s is an elegant and maintainable solution
– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
add a comment |
For your specific case : you could use PowerMock in order to mock System.out
, but the be honest I ignore the whole side effects it might have.
More generally : what you are looking for is called Mock object, and basically allows an instance of an Object
to do nothing be the just minimum to run through your code.
For your case, mocking System.out
would allow you to run through the calls to System.out.println()
without actually calling those.
So as a result, your code would execute as if it was :
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
// System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
// System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
I could go further in explaining how it really occurs, but I guess this is enough for your answer. If you are curious, you could look at runtime execution of your tests to inspect the real type of your mocked objects.
add a comment |
You would first need to make your Java program configurable.
One way to do this is using Java property files. You can then use specific properties to disable parts of your program.
During testing you can then use one property file, and during normal execution another.
The property file is typically a resource on the Java class path. Then you can run the tests with a classpath containing the property files describing the test configuration.
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53408784%2fdisable-a-part-of-java-program-during-junit-testing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
It is impossible without additional effort from you.
Computer simply have no way to tell which computation affect what.
You can turn off specific features like printing to console by replacing default PrintWriter
, but there is no generic solution.
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code withif
s is an elegant and maintainable solution
– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
add a comment |
It is impossible without additional effort from you.
Computer simply have no way to tell which computation affect what.
You can turn off specific features like printing to console by replacing default PrintWriter
, but there is no generic solution.
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code withif
s is an elegant and maintainable solution
– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
add a comment |
It is impossible without additional effort from you.
Computer simply have no way to tell which computation affect what.
You can turn off specific features like printing to console by replacing default PrintWriter
, but there is no generic solution.
It is impossible without additional effort from you.
Computer simply have no way to tell which computation affect what.
You can turn off specific features like printing to console by replacing default PrintWriter
, but there is no generic solution.
answered Nov 21 '18 at 9:27
talextalex
11.4k11648
11.4k11648
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code withif
s is an elegant and maintainable solution
– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
add a comment |
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code withif
s is an elegant and maintainable solution
– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
hi talex, thanks so much for your answer. the helloworld program is just a example of course. I was thinking if there is anything like a generic switch for the whole program so I can toggle between testing mode and normal development mode.
– EugeneH
Nov 21 '18 at 9:47
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code with
if
s is an elegant and maintainable solution– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH you can take inspiration from what logging frameworks do. You set a logging level and when you call the logging method you specify at which level it should and should not log. I highly doubt that spraying your code with
if
s is an elegant and maintainable solution– Federico klez Culloca
Nov 21 '18 at 9:49
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
@EugeneH no there is no such switch because it is theoretically impossible to implement it.
– talex
Nov 21 '18 at 9:51
add a comment |
For your specific case : you could use PowerMock in order to mock System.out
, but the be honest I ignore the whole side effects it might have.
More generally : what you are looking for is called Mock object, and basically allows an instance of an Object
to do nothing be the just minimum to run through your code.
For your case, mocking System.out
would allow you to run through the calls to System.out.println()
without actually calling those.
So as a result, your code would execute as if it was :
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
// System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
// System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
I could go further in explaining how it really occurs, but I guess this is enough for your answer. If you are curious, you could look at runtime execution of your tests to inspect the real type of your mocked objects.
add a comment |
For your specific case : you could use PowerMock in order to mock System.out
, but the be honest I ignore the whole side effects it might have.
More generally : what you are looking for is called Mock object, and basically allows an instance of an Object
to do nothing be the just minimum to run through your code.
For your case, mocking System.out
would allow you to run through the calls to System.out.println()
without actually calling those.
So as a result, your code would execute as if it was :
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
// System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
// System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
I could go further in explaining how it really occurs, but I guess this is enough for your answer. If you are curious, you could look at runtime execution of your tests to inspect the real type of your mocked objects.
add a comment |
For your specific case : you could use PowerMock in order to mock System.out
, but the be honest I ignore the whole side effects it might have.
More generally : what you are looking for is called Mock object, and basically allows an instance of an Object
to do nothing be the just minimum to run through your code.
For your case, mocking System.out
would allow you to run through the calls to System.out.println()
without actually calling those.
So as a result, your code would execute as if it was :
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
// System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
// System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
I could go further in explaining how it really occurs, but I guess this is enough for your answer. If you are curious, you could look at runtime execution of your tests to inspect the real type of your mocked objects.
For your specific case : you could use PowerMock in order to mock System.out
, but the be honest I ignore the whole side effects it might have.
More generally : what you are looking for is called Mock object, and basically allows an instance of an Object
to do nothing be the just minimum to run through your code.
For your case, mocking System.out
would allow you to run through the calls to System.out.println()
without actually calling those.
So as a result, your code would execute as if it was :
public class Helloworld {
/**
* @param args the command line arguments
*/
public static int greetingCnt = 0;
public static void main(String args) {
Helloworld helloword = new Helloworld();
helloword.greetWorld();
helloword.greetWorld();
helloword.greetWorld();
// System.out.println("Greating count: " + greetingCnt);
}
public void greetWorld() {
// System.out.println("Hello World!");
++greetingCnt;
//some other computation...
}
I could go further in explaining how it really occurs, but I guess this is enough for your answer. If you are curious, you could look at runtime execution of your tests to inspect the real type of your mocked objects.
answered Nov 21 '18 at 9:45
Vincent C.Vincent C.
13
13
add a comment |
add a comment |
You would first need to make your Java program configurable.
One way to do this is using Java property files. You can then use specific properties to disable parts of your program.
During testing you can then use one property file, and during normal execution another.
The property file is typically a resource on the Java class path. Then you can run the tests with a classpath containing the property files describing the test configuration.
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
add a comment |
You would first need to make your Java program configurable.
One way to do this is using Java property files. You can then use specific properties to disable parts of your program.
During testing you can then use one property file, and during normal execution another.
The property file is typically a resource on the Java class path. Then you can run the tests with a classpath containing the property files describing the test configuration.
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
add a comment |
You would first need to make your Java program configurable.
One way to do this is using Java property files. You can then use specific properties to disable parts of your program.
During testing you can then use one property file, and during normal execution another.
The property file is typically a resource on the Java class path. Then you can run the tests with a classpath containing the property files describing the test configuration.
You would first need to make your Java program configurable.
One way to do this is using Java property files. You can then use specific properties to disable parts of your program.
During testing you can then use one property file, and during normal execution another.
The property file is typically a resource on the Java class path. Then you can run the tests with a classpath containing the property files describing the test configuration.
answered Nov 21 '18 at 21:26
avandeursenavandeursen
6,61923144
6,61923144
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
add a comment |
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
hi @avandeursen thanks so much for the answer. So from what I understand, the property files is like loading configurations, however, after getting the configurations, how can I implement the separation of testing and dev code? Shall I use if statement or switch/case statement? Or there is another more systematic way of doing it? Really appreciate your help :)
– EugeneH
Nov 22 '18 at 2:20
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53408784%2fdisable-a-part-of-java-program-during-junit-testing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e270hVt4lw8uZRLl xn,xLXBBLjjUqL8qIMYlRBg 3SWUM,4SH8ffs5jUnsS zL3 zqN77b3vvzpsLgQyG 6KFK 4
1
The problem seems to be in the way the code is structured, that is the printing and the increment are done in the same place. I'm not sure how this can be fixed in this example (maybe increment elsewhere and test that function?) but maybe it can be done in your actual case.
– Federico klez Culloca
Nov 21 '18 at 9:25
Hi Federico, thanks for the comments. What i am thinking is if there is anything equivalent to #define or preprocessor in Java, so that the program is able to change behavior a little bit according to different scenarios.
– EugeneH
Nov 21 '18 at 9:45
1
abstraction is the key to writing testable code - separate your concerns then write actual unit tests. SOLID is your friend.
– Boris the Spider
Nov 21 '18 at 10:17