Audio recording using ALSA in PCM format
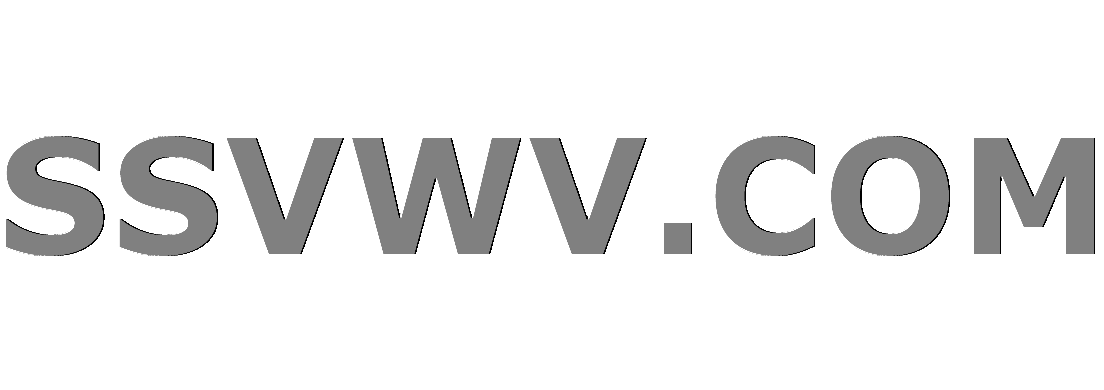
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am working on audio capturing using ALSA in linux platform.
I am able to capture audio using below code where I am passing "default" device into argument, and it will dump audio data into in.pcm
file.
However, when I tried to play in.pcm
file, I hear only noise. I am trying to play audio using below command:
ffplay -autoexit -f f32le -ac 1 -ar 44100 in.pcm
Code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocatedn");
FILE *fp = fopen("in.pcm", "a+");
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
fwrite(buffer, 1, buffer_frames, fp);
fprintf(stdout, "read %d donen", i);
}
fclose(fp);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
Can some one tell me what is issue ?
Thanks in advance.
c linux audio audio-recording alsa
|
show 1 more comment
I am working on audio capturing using ALSA in linux platform.
I am able to capture audio using below code where I am passing "default" device into argument, and it will dump audio data into in.pcm
file.
However, when I tried to play in.pcm
file, I hear only noise. I am trying to play audio using below command:
ffplay -autoexit -f f32le -ac 1 -ar 44100 in.pcm
Code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocatedn");
FILE *fp = fopen("in.pcm", "a+");
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
fwrite(buffer, 1, buffer_frames, fp);
fprintf(stdout, "read %d donen", i);
}
fclose(fp);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
Can some one tell me what is issue ?
Thanks in advance.
c linux audio audio-recording alsa
snd_pcm_readi
counts in frames,fwrite
, in bytes.
– CL.
Nov 25 '18 at 13:16
1
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
1
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
1
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09
|
show 1 more comment
I am working on audio capturing using ALSA in linux platform.
I am able to capture audio using below code where I am passing "default" device into argument, and it will dump audio data into in.pcm
file.
However, when I tried to play in.pcm
file, I hear only noise. I am trying to play audio using below command:
ffplay -autoexit -f f32le -ac 1 -ar 44100 in.pcm
Code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocatedn");
FILE *fp = fopen("in.pcm", "a+");
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
fwrite(buffer, 1, buffer_frames, fp);
fprintf(stdout, "read %d donen", i);
}
fclose(fp);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
Can some one tell me what is issue ?
Thanks in advance.
c linux audio audio-recording alsa
I am working on audio capturing using ALSA in linux platform.
I am able to capture audio using below code where I am passing "default" device into argument, and it will dump audio data into in.pcm
file.
However, when I tried to play in.pcm
file, I hear only noise. I am trying to play audio using below command:
ffplay -autoexit -f f32le -ac 1 -ar 44100 in.pcm
Code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocatedn");
FILE *fp = fopen("in.pcm", "a+");
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
fwrite(buffer, 1, buffer_frames, fp);
fprintf(stdout, "read %d donen", i);
}
fclose(fp);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
Can some one tell me what is issue ?
Thanks in advance.
c linux audio audio-recording alsa
c linux audio audio-recording alsa
edited Nov 25 '18 at 19:07


jww
54.6k42240523
54.6k42240523
asked Nov 25 '18 at 10:50
Harshil MakwanaHarshil Makwana
195
195
snd_pcm_readi
counts in frames,fwrite
, in bytes.
– CL.
Nov 25 '18 at 13:16
1
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
1
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
1
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09
|
show 1 more comment
snd_pcm_readi
counts in frames,fwrite
, in bytes.
– CL.
Nov 25 '18 at 13:16
1
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
1
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
1
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09
snd_pcm_readi
counts in frames, fwrite
, in bytes.– CL.
Nov 25 '18 at 13:16
snd_pcm_readi
counts in frames, fwrite
, in bytes.– CL.
Nov 25 '18 at 13:16
1
1
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
1
1
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
1
1
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09
|
show 1 more comment
1 Answer
1
active
oldest
votes
I fixed this issue by changing buffer size of write function, attaching my working code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocated %dn", snd_pcm_format_width(format) / 8 * 2);
int fd = open("in.pcm", O_CREAT | O_RDWR, 0666);
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
//snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
write(fd, buffer, 128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "read %d donen", i);
}
close(fd);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53466720%2faudio-recording-using-alsa-in-pcm-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I fixed this issue by changing buffer size of write function, attaching my working code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocated %dn", snd_pcm_format_width(format) / 8 * 2);
int fd = open("in.pcm", O_CREAT | O_RDWR, 0666);
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
//snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
write(fd, buffer, 128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "read %d donen", i);
}
close(fd);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
add a comment |
I fixed this issue by changing buffer size of write function, attaching my working code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocated %dn", snd_pcm_format_width(format) / 8 * 2);
int fd = open("in.pcm", O_CREAT | O_RDWR, 0666);
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
//snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
write(fd, buffer, 128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "read %d donen", i);
}
close(fd);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
add a comment |
I fixed this issue by changing buffer size of write function, attaching my working code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocated %dn", snd_pcm_format_width(format) / 8 * 2);
int fd = open("in.pcm", O_CREAT | O_RDWR, 0666);
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
//snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
write(fd, buffer, 128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "read %d donen", i);
}
close(fd);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
I fixed this issue by changing buffer size of write function, attaching my working code:
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
main (int argc, char *argv)
{
int i;
int err;
char *buffer;
int buffer_frames = 128;
unsigned int rate = 44100;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
snd_pcm_format_t format = SND_PCM_FORMAT_FLOAT;
// snd_pcm_format_t format = SND_PCM_FORMAT_S16_LE;
if ((err = snd_pcm_open (&capture_handle, argv[1], SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)n",
argv[1],
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface openedn");
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params allocatedn");
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params initializedn");
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params access settedn");
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, format)) < 0) {
fprintf (stderr, "cannot set sample format (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params format settedn");
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params rate settedn");
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, 2)) < 0) {
fprintf (stderr, "cannot set channel count (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params channels settedn");
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "hw_params settedn");
snd_pcm_hw_params_free (hw_params);
fprintf(stdout, "hw_params freedn");
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)n",
snd_strerror (err));
exit (1);
}
fprintf(stdout, "audio interface preparedn");
buffer = malloc(128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "buffer allocated %dn", snd_pcm_format_width(format) / 8 * 2);
int fd = open("in.pcm", O_CREAT | O_RDWR, 0666);
//for (i = 0; i < 10; ++i) {
i = 0;
while(++i) {
//snd_pcm_wait(capture_handle, 1000);
if ((err = snd_pcm_readi (capture_handle, buffer, buffer_frames)) != buffer_frames) {
fprintf (stderr, "read from audio interface failed (%s)n",
snd_strerror (err));
exit (1);
}
write(fd, buffer, 128 * snd_pcm_format_width(format) / 8 * 2);
fprintf(stdout, "read %d donen", i);
}
close(fd);
free(buffer);
fprintf(stdout, "buffer freedn");
snd_pcm_close (capture_handle);
fprintf(stdout, "audio interface closedn");
exit (0);
}
answered Nov 27 '18 at 3:01
Harshil MakwanaHarshil Makwana
195
195
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
add a comment |
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
You can expand it with playing sound captured in c code.
– EsmaeelE
Nov 27 '18 at 12:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53466720%2faudio-recording-using-alsa-in-pcm-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ya sSarGb9Yup61 Vx2c8,ryAUdcvFV96Fd7jNscLvBpxoBZZiu xsM40p
snd_pcm_readi
counts in frames,fwrite
, in bytes.– CL.
Nov 25 '18 at 13:16
1
@CL so in 2nd argument of fwrite function, I need to pass 128 * format size / 8 * 2, right?
– Harshil Makwana
Nov 25 '18 at 17:08
1
Thanks @CL, After changing size I am getting correct Audio , I updated size to format size / 8 * 2.
– Harshil Makwana
Nov 25 '18 at 17:42
1
This appears to be a very good question from a new user. I am surprised someone down-voted it.
– jww
Nov 25 '18 at 19:08
@Harshil - In a couple of days, you can add an answer in an Answer block. Later, you can accept your own Answer. Also see How does accepting an answer work?
– jww
Nov 25 '18 at 19:09