C++ const "and the object has type qualifiers that are not compatible with the member
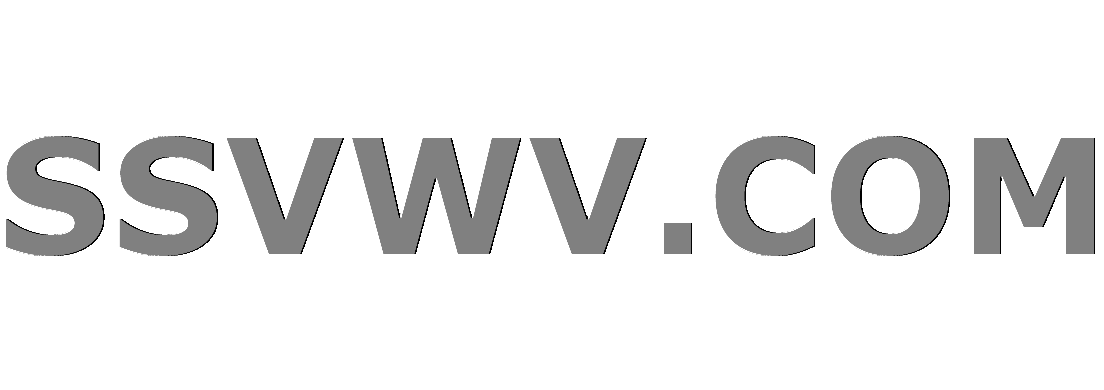
Multi tool use
up vote
0
down vote
favorite
I'm new to C++ programming and in my OPP class we were requested to create a phone book.
Now, in the lecture the Professor said something about that if you want to make sure that your variable that is being injected to a method doesn't get changed you must put const on it.
here is my code so far.
private:
static int phoneCount;
char* name;
char* family;
int phone;
Phone* nextPhone;
public:
int compare(const Phone&other) const;
const char* getFamily();
const char* getName();
and in Phone.cpp
int Phone::compare(const Phone & other) const
{
int result = 0;
result = strcmp(this->family, other.getFamily());
if (result == 0) {
result = strcmp(this->name, other.getName);
}
return 0;
}
I keep getting "the object has type qualifiers that are not compatible with the member"
when I try to call to strcmp inside my compare function.
I know that I can just remove the const in the function declaration and it will go away, but I still doesn't understand why it's showing in the first place.
Help would be greatly appreciated.
c++ const strcmp
add a comment |
up vote
0
down vote
favorite
I'm new to C++ programming and in my OPP class we were requested to create a phone book.
Now, in the lecture the Professor said something about that if you want to make sure that your variable that is being injected to a method doesn't get changed you must put const on it.
here is my code so far.
private:
static int phoneCount;
char* name;
char* family;
int phone;
Phone* nextPhone;
public:
int compare(const Phone&other) const;
const char* getFamily();
const char* getName();
and in Phone.cpp
int Phone::compare(const Phone & other) const
{
int result = 0;
result = strcmp(this->family, other.getFamily());
if (result == 0) {
result = strcmp(this->name, other.getName);
}
return 0;
}
I keep getting "the object has type qualifiers that are not compatible with the member"
when I try to call to strcmp inside my compare function.
I know that I can just remove the const in the function declaration and it will go away, but I still doesn't understand why it's showing in the first place.
Help would be greatly appreciated.
c++ const strcmp
1
As an aside, usestd::string
, and don't mix defining a collection ofPhone
s with definingPhone
– Caleth
Nov 8 at 11:28
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm new to C++ programming and in my OPP class we were requested to create a phone book.
Now, in the lecture the Professor said something about that if you want to make sure that your variable that is being injected to a method doesn't get changed you must put const on it.
here is my code so far.
private:
static int phoneCount;
char* name;
char* family;
int phone;
Phone* nextPhone;
public:
int compare(const Phone&other) const;
const char* getFamily();
const char* getName();
and in Phone.cpp
int Phone::compare(const Phone & other) const
{
int result = 0;
result = strcmp(this->family, other.getFamily());
if (result == 0) {
result = strcmp(this->name, other.getName);
}
return 0;
}
I keep getting "the object has type qualifiers that are not compatible with the member"
when I try to call to strcmp inside my compare function.
I know that I can just remove the const in the function declaration and it will go away, but I still doesn't understand why it's showing in the first place.
Help would be greatly appreciated.
c++ const strcmp
I'm new to C++ programming and in my OPP class we were requested to create a phone book.
Now, in the lecture the Professor said something about that if you want to make sure that your variable that is being injected to a method doesn't get changed you must put const on it.
here is my code so far.
private:
static int phoneCount;
char* name;
char* family;
int phone;
Phone* nextPhone;
public:
int compare(const Phone&other) const;
const char* getFamily();
const char* getName();
and in Phone.cpp
int Phone::compare(const Phone & other) const
{
int result = 0;
result = strcmp(this->family, other.getFamily());
if (result == 0) {
result = strcmp(this->name, other.getName);
}
return 0;
}
I keep getting "the object has type qualifiers that are not compatible with the member"
when I try to call to strcmp inside my compare function.
I know that I can just remove the const in the function declaration and it will go away, but I still doesn't understand why it's showing in the first place.
Help would be greatly appreciated.
c++ const strcmp
c++ const strcmp
asked Nov 8 at 11:17
Yoni hodeffi
62
62
1
As an aside, usestd::string
, and don't mix defining a collection ofPhone
s with definingPhone
– Caleth
Nov 8 at 11:28
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32
add a comment |
1
As an aside, usestd::string
, and don't mix defining a collection ofPhone
s with definingPhone
– Caleth
Nov 8 at 11:28
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32
1
1
As an aside, use
std::string
, and don't mix defining a collection of Phone
s with defining Phone
– Caleth
Nov 8 at 11:28
As an aside, use
std::string
, and don't mix defining a collection of Phone
s with defining Phone
– Caleth
Nov 8 at 11:28
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32
add a comment |
3 Answers
3
active
oldest
votes
up vote
6
down vote
You need to add const
qualifier for getters const char* getFamily() const;
. This way these getters can be invoked on objects of type const Phone &
that you pass into function.
Also other.getName
should be other.getName()
.
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
add a comment |
up vote
2
down vote
In addition to the other answers that correctly suggest const
qualifying your getters, you can access the data members of other
directly, avoiding those calls.
int Phone::compare(const Phone & other) const
{
int result = strcmp(family, other.family);
if (result == 0) {
result = strcmp(name, other.name);
}
return result;
}
add a comment |
up vote
1
down vote
Your signature
int Phone::compare(const Phone & other) const
means inside that function you need to ensure you don't change the Phone
instance.
At the moment, your function calls const char* getFamily()
(and getName
, which you've missed the ()
call from). Neither of these functions are const
, hence the error.
If you mark these as const too, it will be ok.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
6
down vote
You need to add const
qualifier for getters const char* getFamily() const;
. This way these getters can be invoked on objects of type const Phone &
that you pass into function.
Also other.getName
should be other.getName()
.
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
add a comment |
up vote
6
down vote
You need to add const
qualifier for getters const char* getFamily() const;
. This way these getters can be invoked on objects of type const Phone &
that you pass into function.
Also other.getName
should be other.getName()
.
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
add a comment |
up vote
6
down vote
up vote
6
down vote
You need to add const
qualifier for getters const char* getFamily() const;
. This way these getters can be invoked on objects of type const Phone &
that you pass into function.
Also other.getName
should be other.getName()
.
You need to add const
qualifier for getters const char* getFamily() const;
. This way these getters can be invoked on objects of type const Phone &
that you pass into function.
Also other.getName
should be other.getName()
.
answered Nov 8 at 11:19


VTT
23.4k42345
23.4k42345
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
add a comment |
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
2
2
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
Thank you, that so obvious in retrospective
– Yoni hodeffi
Nov 8 at 11:23
add a comment |
up vote
2
down vote
In addition to the other answers that correctly suggest const
qualifying your getters, you can access the data members of other
directly, avoiding those calls.
int Phone::compare(const Phone & other) const
{
int result = strcmp(family, other.family);
if (result == 0) {
result = strcmp(name, other.name);
}
return result;
}
add a comment |
up vote
2
down vote
In addition to the other answers that correctly suggest const
qualifying your getters, you can access the data members of other
directly, avoiding those calls.
int Phone::compare(const Phone & other) const
{
int result = strcmp(family, other.family);
if (result == 0) {
result = strcmp(name, other.name);
}
return result;
}
add a comment |
up vote
2
down vote
up vote
2
down vote
In addition to the other answers that correctly suggest const
qualifying your getters, you can access the data members of other
directly, avoiding those calls.
int Phone::compare(const Phone & other) const
{
int result = strcmp(family, other.family);
if (result == 0) {
result = strcmp(name, other.name);
}
return result;
}
In addition to the other answers that correctly suggest const
qualifying your getters, you can access the data members of other
directly, avoiding those calls.
int Phone::compare(const Phone & other) const
{
int result = strcmp(family, other.family);
if (result == 0) {
result = strcmp(name, other.name);
}
return result;
}
answered Nov 8 at 11:26
Caleth
15.5k22037
15.5k22037
add a comment |
add a comment |
up vote
1
down vote
Your signature
int Phone::compare(const Phone & other) const
means inside that function you need to ensure you don't change the Phone
instance.
At the moment, your function calls const char* getFamily()
(and getName
, which you've missed the ()
call from). Neither of these functions are const
, hence the error.
If you mark these as const too, it will be ok.
add a comment |
up vote
1
down vote
Your signature
int Phone::compare(const Phone & other) const
means inside that function you need to ensure you don't change the Phone
instance.
At the moment, your function calls const char* getFamily()
(and getName
, which you've missed the ()
call from). Neither of these functions are const
, hence the error.
If you mark these as const too, it will be ok.
add a comment |
up vote
1
down vote
up vote
1
down vote
Your signature
int Phone::compare(const Phone & other) const
means inside that function you need to ensure you don't change the Phone
instance.
At the moment, your function calls const char* getFamily()
(and getName
, which you've missed the ()
call from). Neither of these functions are const
, hence the error.
If you mark these as const too, it will be ok.
Your signature
int Phone::compare(const Phone & other) const
means inside that function you need to ensure you don't change the Phone
instance.
At the moment, your function calls const char* getFamily()
(and getName
, which you've missed the ()
call from). Neither of these functions are const
, hence the error.
If you mark these as const too, it will be ok.
answered Nov 8 at 11:21


doctorlove
14.4k22950
14.4k22950
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206678%2fc-const-and-the-object-has-type-qualifiers-that-are-not-compatible-with-the-m%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N8V 2,zH59fw90Db5QVZj9pFfYZtrq45O7LrSYjj9NirFs8fQRbXdOfuCL
1
As an aside, use
std::string
, and don't mix defining a collection ofPhone
s with definingPhone
– Caleth
Nov 8 at 11:28
@Caleth Intrusive lists are not necessarily an antipattern (in fact they used to be extraordinarily common) though I would tend to agree that for modern production C++ it's a bit ew
– Lightness Races in Orbit
Nov 8 at 11:32