How to get all nested iframes on document?
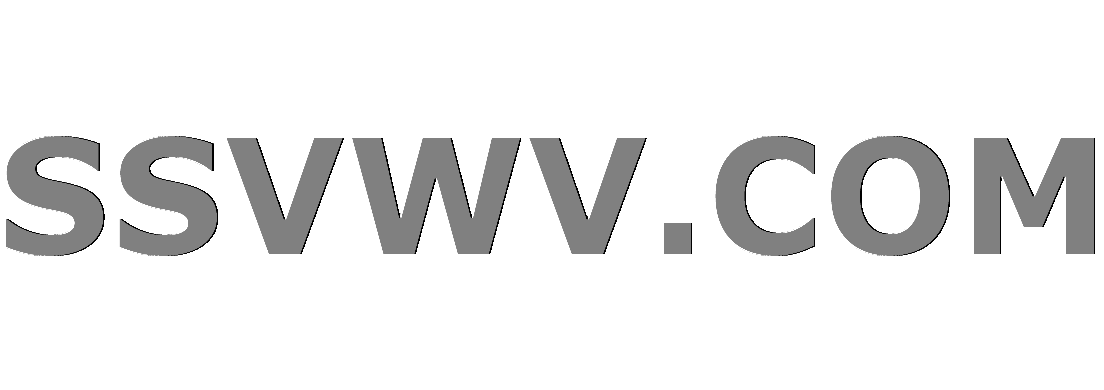
Multi tool use
up vote
0
down vote
favorite
I found many related questions but none of them had a solution that worked for me, so apologies if this is a dupe.
I have the following HTML structure (simplified) :
<html>
<head>
</head>
<body style="">
<div>
<div>
<div>
<iframe>
<html>
<head></head>
<body>
<div></div>
<iframe>
<html>
<head></head>
<body>
<div>
<iframe src="about:blank">
<html>
<head></head>
<body>
<img />
<iframe id="some_random_id">
<html>
<head></head>
<body>
<div>
<!-- main content -->
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</div>
</div>
</body>
</html>
And I would like to retrieve all the iframes, ideally in an array.
I have tried the following:
document.getElementsByTagName('iframe')
But that returns an array of size 1 : [iframe]
window.frames.length
give me 1
I thought about doing something like :
var a = document.getElementsByTagName('iframe')[0]
var b = a.getElementsByTagName('iframe')[0]
// b is undefined
var b = a.contentDocument.getElementsByTagName('iframe')[0]
// b is undefined
Is there any way to retrieve all iframe on the page? Alternatively, just getting the last one (the one with the id some_random_id
) would works as fine, but I can't use the id to select it since the html is created by a third party.
Edit: I don't think my question is a duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
because the accepted answer in this question use:
for( j=0; j<m; j++) {
...
}
Where m
is document.getElementsByTagName('iframe').length
. But in my case it would have the value 1
and thus I couldn't access the nested iframes.
javascript html iframe
add a comment |
up vote
0
down vote
favorite
I found many related questions but none of them had a solution that worked for me, so apologies if this is a dupe.
I have the following HTML structure (simplified) :
<html>
<head>
</head>
<body style="">
<div>
<div>
<div>
<iframe>
<html>
<head></head>
<body>
<div></div>
<iframe>
<html>
<head></head>
<body>
<div>
<iframe src="about:blank">
<html>
<head></head>
<body>
<img />
<iframe id="some_random_id">
<html>
<head></head>
<body>
<div>
<!-- main content -->
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</div>
</div>
</body>
</html>
And I would like to retrieve all the iframes, ideally in an array.
I have tried the following:
document.getElementsByTagName('iframe')
But that returns an array of size 1 : [iframe]
window.frames.length
give me 1
I thought about doing something like :
var a = document.getElementsByTagName('iframe')[0]
var b = a.getElementsByTagName('iframe')[0]
// b is undefined
var b = a.contentDocument.getElementsByTagName('iframe')[0]
// b is undefined
Is there any way to retrieve all iframe on the page? Alternatively, just getting the last one (the one with the id some_random_id
) would works as fine, but I can't use the id to select it since the html is created by a third party.
Edit: I don't think my question is a duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
because the accepted answer in this question use:
for( j=0; j<m; j++) {
...
}
Where m
is document.getElementsByTagName('iframe').length
. But in my case it would have the value 1
and thus I couldn't access the nested iframes.
javascript html iframe
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
1
OK. Then you need to get inside each content window first before usinggetElementsByTagName
. Every iframe has it's ownwindow
which is different than it's parent window
– charlietfl
Nov 8 at 11:49
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I found many related questions but none of them had a solution that worked for me, so apologies if this is a dupe.
I have the following HTML structure (simplified) :
<html>
<head>
</head>
<body style="">
<div>
<div>
<div>
<iframe>
<html>
<head></head>
<body>
<div></div>
<iframe>
<html>
<head></head>
<body>
<div>
<iframe src="about:blank">
<html>
<head></head>
<body>
<img />
<iframe id="some_random_id">
<html>
<head></head>
<body>
<div>
<!-- main content -->
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</div>
</div>
</body>
</html>
And I would like to retrieve all the iframes, ideally in an array.
I have tried the following:
document.getElementsByTagName('iframe')
But that returns an array of size 1 : [iframe]
window.frames.length
give me 1
I thought about doing something like :
var a = document.getElementsByTagName('iframe')[0]
var b = a.getElementsByTagName('iframe')[0]
// b is undefined
var b = a.contentDocument.getElementsByTagName('iframe')[0]
// b is undefined
Is there any way to retrieve all iframe on the page? Alternatively, just getting the last one (the one with the id some_random_id
) would works as fine, but I can't use the id to select it since the html is created by a third party.
Edit: I don't think my question is a duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
because the accepted answer in this question use:
for( j=0; j<m; j++) {
...
}
Where m
is document.getElementsByTagName('iframe').length
. But in my case it would have the value 1
and thus I couldn't access the nested iframes.
javascript html iframe
I found many related questions but none of them had a solution that worked for me, so apologies if this is a dupe.
I have the following HTML structure (simplified) :
<html>
<head>
</head>
<body style="">
<div>
<div>
<div>
<iframe>
<html>
<head></head>
<body>
<div></div>
<iframe>
<html>
<head></head>
<body>
<div>
<iframe src="about:blank">
<html>
<head></head>
<body>
<img />
<iframe id="some_random_id">
<html>
<head></head>
<body>
<div>
<!-- main content -->
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</body>
</html>
</iframe>
</body>
</html>
</iframe>
</div>
</div>
</div>
</body>
</html>
And I would like to retrieve all the iframes, ideally in an array.
I have tried the following:
document.getElementsByTagName('iframe')
But that returns an array of size 1 : [iframe]
window.frames.length
give me 1
I thought about doing something like :
var a = document.getElementsByTagName('iframe')[0]
var b = a.getElementsByTagName('iframe')[0]
// b is undefined
var b = a.contentDocument.getElementsByTagName('iframe')[0]
// b is undefined
Is there any way to retrieve all iframe on the page? Alternatively, just getting the last one (the one with the id some_random_id
) would works as fine, but I can't use the id to select it since the html is created by a third party.
Edit: I don't think my question is a duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
because the accepted answer in this question use:
for( j=0; j<m; j++) {
...
}
Where m
is document.getElementsByTagName('iframe').length
. But in my case it would have the value 1
and thus I couldn't access the nested iframes.
javascript html iframe
javascript html iframe
edited Nov 8 at 16:37


Bharata
6,86541030
6,86541030
asked Nov 8 at 11:36
L. Faros
16110
16110
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
1
OK. Then you need to get inside each content window first before usinggetElementsByTagName
. Every iframe has it's ownwindow
which is different than it's parent window
– charlietfl
Nov 8 at 11:49
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53
add a comment |
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
1
OK. Then you need to get inside each content window first before usinggetElementsByTagName
. Every iframe has it's ownwindow
which is different than it's parent window
– charlietfl
Nov 8 at 11:49
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
1
1
OK. Then you need to get inside each content window first before using
getElementsByTagName
. Every iframe has it's own window
which is different than it's parent window– charlietfl
Nov 8 at 11:49
OK. Then you need to get inside each content window first before using
getElementsByTagName
. Every iframe has it's own window
which is different than it's parent window– charlietfl
Nov 8 at 11:49
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You are using the <iframe>
tag absolutely wrong! You would like to read the documentation from <iframe>
tag:
Permitted content: Fallback content, i.e. content that is normally not rendered, but that browsers not supporting the
<iframe>
element will render.
In other words the content between <iframe>
and </iframe>
tags will be rendered, if the browser do not support the <iframe>
element.
You have two possibilities to use <iframe>
tag:
- In the
src
attribute from<iframe>
tag you could write a path to HTML file. - In the
src
attribute from<iframe>
tag you could write"about:blank"
and then using JS you could add the content to this<iframe>
.
If you want find some elements or manipulate the content from this iframes you could use the following code:
var iframe = document.getElementById('iframeId'),
innerDoc = iframe.contentDocument ? iframe.contentDocument
: iframe.contentWindow.document;
You should be sure that you have an access to your <iframe>
.
Please read Cross-origin resource sharing (CORS) article about it.
If you want to get the count of all nested iframes on document you have to find it for each <iframe>
separatelly and to add this count to your global iframe count variable. And do not forget about the CORS (see above).
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use thedocument.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working
– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same likecharlietfl
in his comments: 1. CORS (same domain). 2.iframe.contentDocument
oriframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.
– Bharata
Nov 8 at 17:05
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You are using the <iframe>
tag absolutely wrong! You would like to read the documentation from <iframe>
tag:
Permitted content: Fallback content, i.e. content that is normally not rendered, but that browsers not supporting the
<iframe>
element will render.
In other words the content between <iframe>
and </iframe>
tags will be rendered, if the browser do not support the <iframe>
element.
You have two possibilities to use <iframe>
tag:
- In the
src
attribute from<iframe>
tag you could write a path to HTML file. - In the
src
attribute from<iframe>
tag you could write"about:blank"
and then using JS you could add the content to this<iframe>
.
If you want find some elements or manipulate the content from this iframes you could use the following code:
var iframe = document.getElementById('iframeId'),
innerDoc = iframe.contentDocument ? iframe.contentDocument
: iframe.contentWindow.document;
You should be sure that you have an access to your <iframe>
.
Please read Cross-origin resource sharing (CORS) article about it.
If you want to get the count of all nested iframes on document you have to find it for each <iframe>
separatelly and to add this count to your global iframe count variable. And do not forget about the CORS (see above).
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use thedocument.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working
– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same likecharlietfl
in his comments: 1. CORS (same domain). 2.iframe.contentDocument
oriframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.
– Bharata
Nov 8 at 17:05
add a comment |
up vote
0
down vote
accepted
You are using the <iframe>
tag absolutely wrong! You would like to read the documentation from <iframe>
tag:
Permitted content: Fallback content, i.e. content that is normally not rendered, but that browsers not supporting the
<iframe>
element will render.
In other words the content between <iframe>
and </iframe>
tags will be rendered, if the browser do not support the <iframe>
element.
You have two possibilities to use <iframe>
tag:
- In the
src
attribute from<iframe>
tag you could write a path to HTML file. - In the
src
attribute from<iframe>
tag you could write"about:blank"
and then using JS you could add the content to this<iframe>
.
If you want find some elements or manipulate the content from this iframes you could use the following code:
var iframe = document.getElementById('iframeId'),
innerDoc = iframe.contentDocument ? iframe.contentDocument
: iframe.contentWindow.document;
You should be sure that you have an access to your <iframe>
.
Please read Cross-origin resource sharing (CORS) article about it.
If you want to get the count of all nested iframes on document you have to find it for each <iframe>
separatelly and to add this count to your global iframe count variable. And do not forget about the CORS (see above).
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use thedocument.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working
– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same likecharlietfl
in his comments: 1. CORS (same domain). 2.iframe.contentDocument
oriframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.
– Bharata
Nov 8 at 17:05
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You are using the <iframe>
tag absolutely wrong! You would like to read the documentation from <iframe>
tag:
Permitted content: Fallback content, i.e. content that is normally not rendered, but that browsers not supporting the
<iframe>
element will render.
In other words the content between <iframe>
and </iframe>
tags will be rendered, if the browser do not support the <iframe>
element.
You have two possibilities to use <iframe>
tag:
- In the
src
attribute from<iframe>
tag you could write a path to HTML file. - In the
src
attribute from<iframe>
tag you could write"about:blank"
and then using JS you could add the content to this<iframe>
.
If you want find some elements or manipulate the content from this iframes you could use the following code:
var iframe = document.getElementById('iframeId'),
innerDoc = iframe.contentDocument ? iframe.contentDocument
: iframe.contentWindow.document;
You should be sure that you have an access to your <iframe>
.
Please read Cross-origin resource sharing (CORS) article about it.
If you want to get the count of all nested iframes on document you have to find it for each <iframe>
separatelly and to add this count to your global iframe count variable. And do not forget about the CORS (see above).
You are using the <iframe>
tag absolutely wrong! You would like to read the documentation from <iframe>
tag:
Permitted content: Fallback content, i.e. content that is normally not rendered, but that browsers not supporting the
<iframe>
element will render.
In other words the content between <iframe>
and </iframe>
tags will be rendered, if the browser do not support the <iframe>
element.
You have two possibilities to use <iframe>
tag:
- In the
src
attribute from<iframe>
tag you could write a path to HTML file. - In the
src
attribute from<iframe>
tag you could write"about:blank"
and then using JS you could add the content to this<iframe>
.
If you want find some elements or manipulate the content from this iframes you could use the following code:
var iframe = document.getElementById('iframeId'),
innerDoc = iframe.contentDocument ? iframe.contentDocument
: iframe.contentWindow.document;
You should be sure that you have an access to your <iframe>
.
Please read Cross-origin resource sharing (CORS) article about it.
If you want to get the count of all nested iframes on document you have to find it for each <iframe>
separatelly and to add this count to your global iframe count variable. And do not forget about the CORS (see above).
answered Nov 8 at 16:31


Bharata
6,86541030
6,86541030
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use thedocument.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working
– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same likecharlietfl
in his comments: 1. CORS (same domain). 2.iframe.contentDocument
oriframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.
– Bharata
Nov 8 at 17:05
add a comment |
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use thedocument.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working
– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same likecharlietfl
in his comments: 1. CORS (same domain). 2.iframe.contentDocument
oriframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.
– Bharata
Nov 8 at 17:05
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
CORS doesn't help with iframes.
– Quentin
Nov 8 at 16:39
Well as I said in the question I can't use the
document.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working– L. Faros
Nov 8 at 16:40
Well as I said in the question I can't use the
document.getElementById
because the id is random. And FYI the DOM structure I posted is the simplified version of what some js third party insert in one of my pages, I needed to get access to the html in the most nested iframe. Anyway I went with the method suggested by charlietfl in comments which is working– L. Faros
Nov 8 at 16:40
@L.Faros if you read my answer again then you will see that I wrote the same like
charlietfl
in his comments: 1. CORS (same domain). 2. iframe.contentDocument
or iframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.– Bharata
Nov 8 at 17:05
@L.Faros if you read my answer again then you will see that I wrote the same like
charlietfl
in his comments: 1. CORS (same domain). 2. iframe.contentDocument
or iframe.contentWindow.document
. My answer is detailed – so each user could understand it. And if this works, then you could mark my answer as accepted, please.– Bharata
Nov 8 at 17:05
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53206970%2fhow-to-get-all-nested-iframes-on-document%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0ubChCUgQwy5TuxWRZmPHnbTRiULh
Possible duplicate of using document.getElementsByTagName on a page with iFrames - elements inside the iframe are not being picked up
– Muhammet Can TONBUL
Nov 8 at 11:40
You can only do this if all the iframes are from same domain. Is that true?
– charlietfl
Nov 8 at 11:41
None of the iframe have a domain except for the one with ` src="about:blank"` but I don't think ` about:blank` is a domain right ?
– L. Faros
Nov 8 at 11:42
1
OK. Then you need to get inside each content window first before using
getElementsByTagName
. Every iframe has it's ownwindow
which is different than it's parent window– charlietfl
Nov 8 at 11:49
Not sure on how to do that, isn't it what I tried in the last code snippet I put ? (the one before the Edit)
– L. Faros
Nov 8 at 11:53