App crashing using collection.add() with Firestore
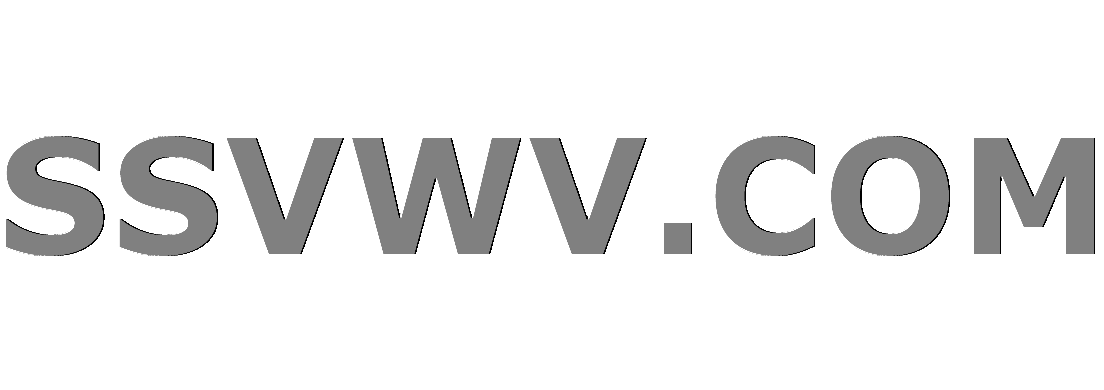
Multi tool use
I'm a newbie on Android development, so please forgive me if this sounds quite trivial or it's too long, but I got stuck trying to add items to a collection in Firestore.
I read somewhere in a tutorial's comments section this occured below API 25 (I'm using 24), but I'm not sure why.
Here's part of my code:
FirebaseFirestore db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_registro);
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
db = FirebaseFirestore.getInstance();
}
public void sendInfo(View view){
CollectionReference dbArtists = db.collection("artistas");
Artist a = new Artist("0", "A", 10);
dbArtists.add(a)
}
sendInfo is associated to a button that works fine. The line that crashes the app is
dbArtists.add(a)
So the logcat had a warning stating:
com.esiete.anapptitle W/Firestore: (0.6.6-dev) [Firestore]: The behavior for java.util.Date objects stored in Firestore is going to change AND YOUR APP MAY BREAK.
To hide this warning and ensure your app does not break, you need to add the following code to your app before calling any other Cloud Firestore methods:
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
With this change, timestamps stored in Cloud Firestore will be read back as com.google.firebase.Timestamp objects instead of as system java.util.Date objects. So you will also need to update code expecting a java.util.Date to instead expect a Timestamp. For example:
// Old:
java.util.Date date = snapshot.getDate("created_at");
// New:
Timestamp timestamp = snapshot.getTimestamp("created_at");
java.util.Date date = timestamp.toDate();
Please audit all existing usages of java.util.Date when you enable the new behavior. In a future release, the behavior will be changed to the new behavior, so if you do not follow these steps, YOUR APP MAY BREAK.
Crashes still remain after adding the code as shown above. Logcat shows:
8-11-13 21:44:09.290 30030-30030/com.esiete.anapptitle D/AndroidRuntime: Shutting down VM
2018-11-13 21:44:09.294 30030-30030/com.esiete.anapptitle E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.esiete.anapptitle, PID: 30030
java.lang.IllegalStateException: Could not execute method for android:onClick
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:390)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.reflect.InvocationTargetException
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.RuntimeException: No properties to serialize found on class com.esiete.anapptitle.Artist
at com.google.firebase.firestore.g.zzg$zza.<init>(SourceFile:643)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:331)
at com.google.firebase.firestore.g.zzg.zzb(SourceFile:152)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:1085)
at com.google.firebase.firestore.UserDataConverter.convertPOJO(SourceFile:421)
at com.google.firebase.firestore.CollectionReference.add(SourceFile:125)
at com.esiete.anapptitle.RegistroActivity.enviarRegistro(RegistroActivity.java:82)
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
2018-11-13 21:44:09.298 4088-5186/? W/ActivityManager: Force finishing activity com.esiete.anapptitle/.RegistroActivity
2018-11-13 21:44:09.306 4088-30868/? W/DropBoxManagerService: Dropping: data_app_crash (1752 > 0 bytes)
Any help or word of advice is greatly appreciated.
EDIT: Adding Artist class code.
package com.esiete.anapptitle;
public class Artist {
private String artistID;
private String name;
private int sales;
public Artist() {
}
public Artist(String artistID, String name, int sales) {
this.artistID = artistID;
this.name = name;
this.sales = sales;
}
}

add a comment |
I'm a newbie on Android development, so please forgive me if this sounds quite trivial or it's too long, but I got stuck trying to add items to a collection in Firestore.
I read somewhere in a tutorial's comments section this occured below API 25 (I'm using 24), but I'm not sure why.
Here's part of my code:
FirebaseFirestore db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_registro);
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
db = FirebaseFirestore.getInstance();
}
public void sendInfo(View view){
CollectionReference dbArtists = db.collection("artistas");
Artist a = new Artist("0", "A", 10);
dbArtists.add(a)
}
sendInfo is associated to a button that works fine. The line that crashes the app is
dbArtists.add(a)
So the logcat had a warning stating:
com.esiete.anapptitle W/Firestore: (0.6.6-dev) [Firestore]: The behavior for java.util.Date objects stored in Firestore is going to change AND YOUR APP MAY BREAK.
To hide this warning and ensure your app does not break, you need to add the following code to your app before calling any other Cloud Firestore methods:
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
With this change, timestamps stored in Cloud Firestore will be read back as com.google.firebase.Timestamp objects instead of as system java.util.Date objects. So you will also need to update code expecting a java.util.Date to instead expect a Timestamp. For example:
// Old:
java.util.Date date = snapshot.getDate("created_at");
// New:
Timestamp timestamp = snapshot.getTimestamp("created_at");
java.util.Date date = timestamp.toDate();
Please audit all existing usages of java.util.Date when you enable the new behavior. In a future release, the behavior will be changed to the new behavior, so if you do not follow these steps, YOUR APP MAY BREAK.
Crashes still remain after adding the code as shown above. Logcat shows:
8-11-13 21:44:09.290 30030-30030/com.esiete.anapptitle D/AndroidRuntime: Shutting down VM
2018-11-13 21:44:09.294 30030-30030/com.esiete.anapptitle E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.esiete.anapptitle, PID: 30030
java.lang.IllegalStateException: Could not execute method for android:onClick
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:390)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.reflect.InvocationTargetException
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.RuntimeException: No properties to serialize found on class com.esiete.anapptitle.Artist
at com.google.firebase.firestore.g.zzg$zza.<init>(SourceFile:643)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:331)
at com.google.firebase.firestore.g.zzg.zzb(SourceFile:152)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:1085)
at com.google.firebase.firestore.UserDataConverter.convertPOJO(SourceFile:421)
at com.google.firebase.firestore.CollectionReference.add(SourceFile:125)
at com.esiete.anapptitle.RegistroActivity.enviarRegistro(RegistroActivity.java:82)
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
2018-11-13 21:44:09.298 4088-5186/? W/ActivityManager: Force finishing activity com.esiete.anapptitle/.RegistroActivity
2018-11-13 21:44:09.306 4088-30868/? W/DropBoxManagerService: Dropping: data_app_crash (1752 > 0 bytes)
Any help or word of advice is greatly appreciated.
EDIT: Adding Artist class code.
package com.esiete.anapptitle;
public class Artist {
private String artistID;
private String name;
private int sales;
public Artist() {
}
public Artist(String artistID, String name, int sales) {
this.artistID = artistID;
this.name = name;
this.sales = sales;
}
}

2
The error message is complaining about your classArtist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.
– Doug Stevenson
Nov 14 '18 at 4:02
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18
add a comment |
I'm a newbie on Android development, so please forgive me if this sounds quite trivial or it's too long, but I got stuck trying to add items to a collection in Firestore.
I read somewhere in a tutorial's comments section this occured below API 25 (I'm using 24), but I'm not sure why.
Here's part of my code:
FirebaseFirestore db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_registro);
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
db = FirebaseFirestore.getInstance();
}
public void sendInfo(View view){
CollectionReference dbArtists = db.collection("artistas");
Artist a = new Artist("0", "A", 10);
dbArtists.add(a)
}
sendInfo is associated to a button that works fine. The line that crashes the app is
dbArtists.add(a)
So the logcat had a warning stating:
com.esiete.anapptitle W/Firestore: (0.6.6-dev) [Firestore]: The behavior for java.util.Date objects stored in Firestore is going to change AND YOUR APP MAY BREAK.
To hide this warning and ensure your app does not break, you need to add the following code to your app before calling any other Cloud Firestore methods:
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
With this change, timestamps stored in Cloud Firestore will be read back as com.google.firebase.Timestamp objects instead of as system java.util.Date objects. So you will also need to update code expecting a java.util.Date to instead expect a Timestamp. For example:
// Old:
java.util.Date date = snapshot.getDate("created_at");
// New:
Timestamp timestamp = snapshot.getTimestamp("created_at");
java.util.Date date = timestamp.toDate();
Please audit all existing usages of java.util.Date when you enable the new behavior. In a future release, the behavior will be changed to the new behavior, so if you do not follow these steps, YOUR APP MAY BREAK.
Crashes still remain after adding the code as shown above. Logcat shows:
8-11-13 21:44:09.290 30030-30030/com.esiete.anapptitle D/AndroidRuntime: Shutting down VM
2018-11-13 21:44:09.294 30030-30030/com.esiete.anapptitle E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.esiete.anapptitle, PID: 30030
java.lang.IllegalStateException: Could not execute method for android:onClick
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:390)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.reflect.InvocationTargetException
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.RuntimeException: No properties to serialize found on class com.esiete.anapptitle.Artist
at com.google.firebase.firestore.g.zzg$zza.<init>(SourceFile:643)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:331)
at com.google.firebase.firestore.g.zzg.zzb(SourceFile:152)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:1085)
at com.google.firebase.firestore.UserDataConverter.convertPOJO(SourceFile:421)
at com.google.firebase.firestore.CollectionReference.add(SourceFile:125)
at com.esiete.anapptitle.RegistroActivity.enviarRegistro(RegistroActivity.java:82)
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
2018-11-13 21:44:09.298 4088-5186/? W/ActivityManager: Force finishing activity com.esiete.anapptitle/.RegistroActivity
2018-11-13 21:44:09.306 4088-30868/? W/DropBoxManagerService: Dropping: data_app_crash (1752 > 0 bytes)
Any help or word of advice is greatly appreciated.
EDIT: Adding Artist class code.
package com.esiete.anapptitle;
public class Artist {
private String artistID;
private String name;
private int sales;
public Artist() {
}
public Artist(String artistID, String name, int sales) {
this.artistID = artistID;
this.name = name;
this.sales = sales;
}
}

I'm a newbie on Android development, so please forgive me if this sounds quite trivial or it's too long, but I got stuck trying to add items to a collection in Firestore.
I read somewhere in a tutorial's comments section this occured below API 25 (I'm using 24), but I'm not sure why.
Here's part of my code:
FirebaseFirestore db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_registro);
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
db = FirebaseFirestore.getInstance();
}
public void sendInfo(View view){
CollectionReference dbArtists = db.collection("artistas");
Artist a = new Artist("0", "A", 10);
dbArtists.add(a)
}
sendInfo is associated to a button that works fine. The line that crashes the app is
dbArtists.add(a)
So the logcat had a warning stating:
com.esiete.anapptitle W/Firestore: (0.6.6-dev) [Firestore]: The behavior for java.util.Date objects stored in Firestore is going to change AND YOUR APP MAY BREAK.
To hide this warning and ensure your app does not break, you need to add the following code to your app before calling any other Cloud Firestore methods:
FirebaseFirestore firestore = FirebaseFirestore.getInstance();
FirebaseFirestoreSettings settings = new FirebaseFirestoreSettings.Builder()
.setTimestampsInSnapshotsEnabled(true)
.build();
firestore.setFirestoreSettings(settings);
With this change, timestamps stored in Cloud Firestore will be read back as com.google.firebase.Timestamp objects instead of as system java.util.Date objects. So you will also need to update code expecting a java.util.Date to instead expect a Timestamp. For example:
// Old:
java.util.Date date = snapshot.getDate("created_at");
// New:
Timestamp timestamp = snapshot.getTimestamp("created_at");
java.util.Date date = timestamp.toDate();
Please audit all existing usages of java.util.Date when you enable the new behavior. In a future release, the behavior will be changed to the new behavior, so if you do not follow these steps, YOUR APP MAY BREAK.
Crashes still remain after adding the code as shown above. Logcat shows:
8-11-13 21:44:09.290 30030-30030/com.esiete.anapptitle D/AndroidRuntime: Shutting down VM
2018-11-13 21:44:09.294 30030-30030/com.esiete.anapptitle E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.esiete.anapptitle, PID: 30030
java.lang.IllegalStateException: Could not execute method for android:onClick
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:390)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.reflect.InvocationTargetException
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
Caused by: java.lang.RuntimeException: No properties to serialize found on class com.esiete.anapptitle.Artist
at com.google.firebase.firestore.g.zzg$zza.<init>(SourceFile:643)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:331)
at com.google.firebase.firestore.g.zzg.zzb(SourceFile:152)
at com.google.firebase.firestore.g.zzg.zza(SourceFile:1085)
at com.google.firebase.firestore.UserDataConverter.convertPOJO(SourceFile:421)
at com.google.firebase.firestore.CollectionReference.add(SourceFile:125)
at com.esiete.anapptitle.RegistroActivity.enviarRegistro(RegistroActivity.java:82)
at java.lang.reflect.Method.invoke(Native Method)
at android.support.v7.app.AppCompatViewInflater$DeclaredOnClickListener.onClick(AppCompatViewInflater.java:385)
at android.view.View.performClick(View.java:5612)
at android.view.View$PerformClick.run(View.java:22285)
at android.os.Handler.handleCallback(Handler.java:751)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6123)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:867)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:757)
2018-11-13 21:44:09.298 4088-5186/? W/ActivityManager: Force finishing activity com.esiete.anapptitle/.RegistroActivity
2018-11-13 21:44:09.306 4088-30868/? W/DropBoxManagerService: Dropping: data_app_crash (1752 > 0 bytes)
Any help or word of advice is greatly appreciated.
EDIT: Adding Artist class code.
package com.esiete.anapptitle;
public class Artist {
private String artistID;
private String name;
private int sales;
public Artist() {
}
public Artist(String artistID, String name, int sales) {
this.artistID = artistID;
this.name = name;
this.sales = sales;
}
}


edited Nov 14 '18 at 4:16
Frank van Puffelen
230k28376400
230k28376400
asked Nov 14 '18 at 4:00


Uriel TapiaUriel Tapia
52
52
2
The error message is complaining about your classArtist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.
– Doug Stevenson
Nov 14 '18 at 4:02
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18
add a comment |
2
The error message is complaining about your classArtist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.
– Doug Stevenson
Nov 14 '18 at 4:02
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18
2
2
The error message is complaining about your class
Artist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.– Doug Stevenson
Nov 14 '18 at 4:02
The error message is complaining about your class
Artist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.– Doug Stevenson
Nov 14 '18 at 4:02
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18
add a comment |
1 Answer
1
active
oldest
votes
Your Artist
class has no information that Firebase knows how to serialize to and from the database. In order of it to know this, the class needs to either have public fields, or public getter and setter methods.
Say that an Artist
document in your database has these three fields:
artistId: "0"
name: "A"
sales: 10
That means that you need either this Java class:
public class Artist {
public String artistId;
public String name;
public long sales;
}
Each public field here matches the exact field name of a document in the database, which is how the Firebase client can read/write the correct values.
Alternatively you can have this Java class:
public class Artist {
String artistId;
String name;
long sales;
public Artist() {} // Empty constructor is required
public Artist(String artistId, String name, long sales) {
this.artistId = artistId;
this.name = name;
this.sales = sales;
}
public String getArtistId() { return this.artistId; }
public void setArtistId(String artistId) { this.artistId = artistId; }
public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public long getRanking() { return this.ranking; }
public void setRanking(long ranking) { this.ranking = ranking; }
}
This second class follow JavaBean naming conventions, meaning that getId/setId
define a property id
, which matches the exact field name in the database again.
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last twoString
statements should actually belong
ones.
– Uriel Tapia
Nov 14 '18 at 4:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293013%2fapp-crashing-using-collection-add-with-firestore%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your Artist
class has no information that Firebase knows how to serialize to and from the database. In order of it to know this, the class needs to either have public fields, or public getter and setter methods.
Say that an Artist
document in your database has these three fields:
artistId: "0"
name: "A"
sales: 10
That means that you need either this Java class:
public class Artist {
public String artistId;
public String name;
public long sales;
}
Each public field here matches the exact field name of a document in the database, which is how the Firebase client can read/write the correct values.
Alternatively you can have this Java class:
public class Artist {
String artistId;
String name;
long sales;
public Artist() {} // Empty constructor is required
public Artist(String artistId, String name, long sales) {
this.artistId = artistId;
this.name = name;
this.sales = sales;
}
public String getArtistId() { return this.artistId; }
public void setArtistId(String artistId) { this.artistId = artistId; }
public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public long getRanking() { return this.ranking; }
public void setRanking(long ranking) { this.ranking = ranking; }
}
This second class follow JavaBean naming conventions, meaning that getId/setId
define a property id
, which matches the exact field name in the database again.
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last twoString
statements should actually belong
ones.
– Uriel Tapia
Nov 14 '18 at 4:26
add a comment |
Your Artist
class has no information that Firebase knows how to serialize to and from the database. In order of it to know this, the class needs to either have public fields, or public getter and setter methods.
Say that an Artist
document in your database has these three fields:
artistId: "0"
name: "A"
sales: 10
That means that you need either this Java class:
public class Artist {
public String artistId;
public String name;
public long sales;
}
Each public field here matches the exact field name of a document in the database, which is how the Firebase client can read/write the correct values.
Alternatively you can have this Java class:
public class Artist {
String artistId;
String name;
long sales;
public Artist() {} // Empty constructor is required
public Artist(String artistId, String name, long sales) {
this.artistId = artistId;
this.name = name;
this.sales = sales;
}
public String getArtistId() { return this.artistId; }
public void setArtistId(String artistId) { this.artistId = artistId; }
public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public long getRanking() { return this.ranking; }
public void setRanking(long ranking) { this.ranking = ranking; }
}
This second class follow JavaBean naming conventions, meaning that getId/setId
define a property id
, which matches the exact field name in the database again.
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last twoString
statements should actually belong
ones.
– Uriel Tapia
Nov 14 '18 at 4:26
add a comment |
Your Artist
class has no information that Firebase knows how to serialize to and from the database. In order of it to know this, the class needs to either have public fields, or public getter and setter methods.
Say that an Artist
document in your database has these three fields:
artistId: "0"
name: "A"
sales: 10
That means that you need either this Java class:
public class Artist {
public String artistId;
public String name;
public long sales;
}
Each public field here matches the exact field name of a document in the database, which is how the Firebase client can read/write the correct values.
Alternatively you can have this Java class:
public class Artist {
String artistId;
String name;
long sales;
public Artist() {} // Empty constructor is required
public Artist(String artistId, String name, long sales) {
this.artistId = artistId;
this.name = name;
this.sales = sales;
}
public String getArtistId() { return this.artistId; }
public void setArtistId(String artistId) { this.artistId = artistId; }
public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public long getRanking() { return this.ranking; }
public void setRanking(long ranking) { this.ranking = ranking; }
}
This second class follow JavaBean naming conventions, meaning that getId/setId
define a property id
, which matches the exact field name in the database again.
Your Artist
class has no information that Firebase knows how to serialize to and from the database. In order of it to know this, the class needs to either have public fields, or public getter and setter methods.
Say that an Artist
document in your database has these three fields:
artistId: "0"
name: "A"
sales: 10
That means that you need either this Java class:
public class Artist {
public String artistId;
public String name;
public long sales;
}
Each public field here matches the exact field name of a document in the database, which is how the Firebase client can read/write the correct values.
Alternatively you can have this Java class:
public class Artist {
String artistId;
String name;
long sales;
public Artist() {} // Empty constructor is required
public Artist(String artistId, String name, long sales) {
this.artistId = artistId;
this.name = name;
this.sales = sales;
}
public String getArtistId() { return this.artistId; }
public void setArtistId(String artistId) { this.artistId = artistId; }
public String getName() { return this.name; }
public void setName(String name) { this.name = name; }
public long getRanking() { return this.ranking; }
public void setRanking(long ranking) { this.ranking = ranking; }
}
This second class follow JavaBean naming conventions, meaning that getId/setId
define a property id
, which matches the exact field name in the database again.
edited Nov 14 '18 at 4:29
answered Nov 14 '18 at 4:16
Frank van PuffelenFrank van Puffelen
230k28376400
230k28376400
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last twoString
statements should actually belong
ones.
– Uriel Tapia
Nov 14 '18 at 4:26
add a comment |
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last twoString
statements should actually belong
ones.
– Uriel Tapia
Nov 14 '18 at 4:26
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last two
String
statements should actually be long
ones.– Uriel Tapia
Nov 14 '18 at 4:26
Thank you, this solved it instantly! I'll keep it in mind. I'll edit your answer just a bit; last two
String
statements should actually be long
ones.– Uriel Tapia
Nov 14 '18 at 4:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293013%2fapp-crashing-using-collection-add-with-firestore%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8,or4XGlqWuWNI9aCxAAKpPH2CgTC7cr,0OtUnJkqMslA9YzMhlh4cZqWVJhcfPiboKDJ,DppOFG,wxj 2,XiG5kqEtfXZ5IDgeJl
2
The error message is complaining about your class
Artist
: "No properties to serialize found on class com.esiete.anapptitle.Artist". Please edit your question to show the code for that class.– Doug Stevenson
Nov 14 '18 at 4:02
have you initialized a new instance of the list before using the add() function?
– Anjani Mittal
Nov 14 '18 at 4:09
@DougStevenson done. I tried making my variables public as suggested here, but got no luck.
– Uriel Tapia
Nov 14 '18 at 4:16
@AnjaniMittal no. I'm sorry, what list are you talking about? Do you mean one at FireStore? Yes, I have.
– Uriel Tapia
Nov 14 '18 at 4:18