Sub-document in an array saves as an empty array item if no value is provided on document creation
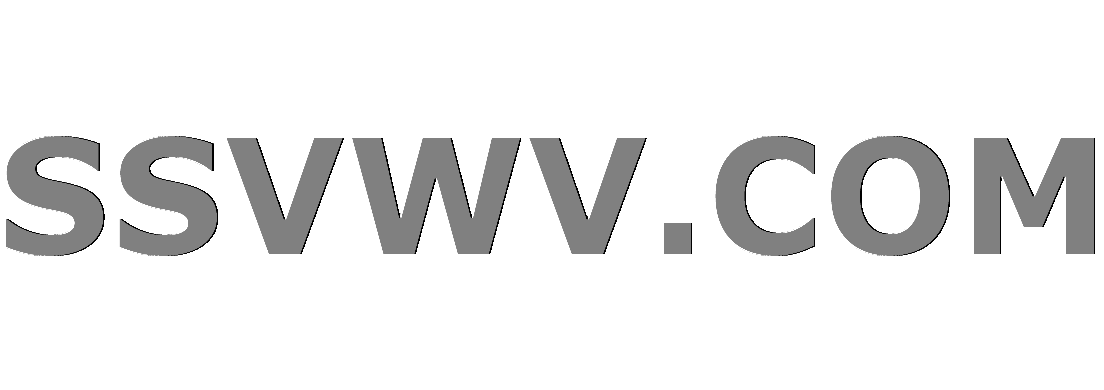
Multi tool use
What I want is for a particular field in my schema to be an array with items in it.
When I create the document in question, I will not have any array items. Therefore, I expect my document to look like:
{
notes:
}
The problem is, I'm getting an array that looks like:
{
notes: ['']
}
Querying the notes.length
, I get 1, which is problematic for me, because it's essentially an empty array item.
This is the code I'm working with:
const SubDocumentSchema = function () {
return new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
}
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema()]
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
arrays mongodb mongoose mongoose-schema
add a comment |
What I want is for a particular field in my schema to be an array with items in it.
When I create the document in question, I will not have any array items. Therefore, I expect my document to look like:
{
notes:
}
The problem is, I'm getting an array that looks like:
{
notes: ['']
}
Querying the notes.length
, I get 1, which is problematic for me, because it's essentially an empty array item.
This is the code I'm working with:
const SubDocumentSchema = function () {
return new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
}
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema()]
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
arrays mongodb mongoose mongoose-schema
1
What isNotesSchema
? Not in your code. It also probably should betype: [NotesSchema]
since the()
implies invoking a function, not assigning a "schema type" as you should be doing here.
– Neil Lunn
Nov 14 '18 at 4:02
Sorry, I fixed that. I actually changed the schema to justnew....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.
– Modermo
Nov 14 '18 at 4:33
1
Again you don't donew
. Just do it like I already ( and as the documentation also does ) showed you.type: [SubDocumentSchema]
. Nonew
and no brackets()
. You use it there just like the second argument in yourmongoose.model
call.model('DocumentSchema', DocumentSchema)
where again, nonew
and no brackets()
.
– Neil Lunn
Nov 14 '18 at 4:37
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
1
You're misunderstanding.const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So nofunction()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html
– Neil Lunn
Nov 14 '18 at 5:11
add a comment |
What I want is for a particular field in my schema to be an array with items in it.
When I create the document in question, I will not have any array items. Therefore, I expect my document to look like:
{
notes:
}
The problem is, I'm getting an array that looks like:
{
notes: ['']
}
Querying the notes.length
, I get 1, which is problematic for me, because it's essentially an empty array item.
This is the code I'm working with:
const SubDocumentSchema = function () {
return new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
}
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema()]
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
arrays mongodb mongoose mongoose-schema
What I want is for a particular field in my schema to be an array with items in it.
When I create the document in question, I will not have any array items. Therefore, I expect my document to look like:
{
notes:
}
The problem is, I'm getting an array that looks like:
{
notes: ['']
}
Querying the notes.length
, I get 1, which is problematic for me, because it's essentially an empty array item.
This is the code I'm working with:
const SubDocumentSchema = function () {
return new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
}
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema()]
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
arrays mongodb mongoose mongoose-schema
arrays mongodb mongoose mongoose-schema
edited Nov 14 '18 at 4:30
Modermo
asked Nov 14 '18 at 3:57
ModermoModermo
557414
557414
1
What isNotesSchema
? Not in your code. It also probably should betype: [NotesSchema]
since the()
implies invoking a function, not assigning a "schema type" as you should be doing here.
– Neil Lunn
Nov 14 '18 at 4:02
Sorry, I fixed that. I actually changed the schema to justnew....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.
– Modermo
Nov 14 '18 at 4:33
1
Again you don't donew
. Just do it like I already ( and as the documentation also does ) showed you.type: [SubDocumentSchema]
. Nonew
and no brackets()
. You use it there just like the second argument in yourmongoose.model
call.model('DocumentSchema', DocumentSchema)
where again, nonew
and no brackets()
.
– Neil Lunn
Nov 14 '18 at 4:37
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
1
You're misunderstanding.const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So nofunction()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html
– Neil Lunn
Nov 14 '18 at 5:11
add a comment |
1
What isNotesSchema
? Not in your code. It also probably should betype: [NotesSchema]
since the()
implies invoking a function, not assigning a "schema type" as you should be doing here.
– Neil Lunn
Nov 14 '18 at 4:02
Sorry, I fixed that. I actually changed the schema to justnew....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.
– Modermo
Nov 14 '18 at 4:33
1
Again you don't donew
. Just do it like I already ( and as the documentation also does ) showed you.type: [SubDocumentSchema]
. Nonew
and no brackets()
. You use it there just like the second argument in yourmongoose.model
call.model('DocumentSchema', DocumentSchema)
where again, nonew
and no brackets()
.
– Neil Lunn
Nov 14 '18 at 4:37
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
1
You're misunderstanding.const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So nofunction()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html
– Neil Lunn
Nov 14 '18 at 5:11
1
1
What is
NotesSchema
? Not in your code. It also probably should be type: [NotesSchema]
since the ()
implies invoking a function, not assigning a "schema type" as you should be doing here.– Neil Lunn
Nov 14 '18 at 4:02
What is
NotesSchema
? Not in your code. It also probably should be type: [NotesSchema]
since the ()
implies invoking a function, not assigning a "schema type" as you should be doing here.– Neil Lunn
Nov 14 '18 at 4:02
Sorry, I fixed that. I actually changed the schema to just
new....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.– Modermo
Nov 14 '18 at 4:33
Sorry, I fixed that. I actually changed the schema to just
new....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.– Modermo
Nov 14 '18 at 4:33
1
1
Again you don't do
new
. Just do it like I already ( and as the documentation also does ) showed you. type: [SubDocumentSchema]
. No new
and no brackets ()
. You use it there just like the second argument in your mongoose.model
call .model('DocumentSchema', DocumentSchema)
where again, no new
and no brackets ()
.– Neil Lunn
Nov 14 '18 at 4:37
Again you don't do
new
. Just do it like I already ( and as the documentation also does ) showed you. type: [SubDocumentSchema]
. No new
and no brackets ()
. You use it there just like the second argument in your mongoose.model
call .model('DocumentSchema', DocumentSchema)
where again, no new
and no brackets ()
.– Neil Lunn
Nov 14 '18 at 4:37
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
1
1
You're misunderstanding.
const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So no function()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html– Neil Lunn
Nov 14 '18 at 5:11
You're misunderstanding.
const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So no function()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html– Neil Lunn
Nov 14 '18 at 5:11
add a comment |
1 Answer
1
active
oldest
votes
You can specify empty array as the default value for notes. And you don't need to return a function for the SubDocumentSchema. Try the below edited code.
const SubDocumentSchema = new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema],
default:
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292993%2fsub-document-in-an-array-saves-as-an-empty-array-item-if-no-value-is-provided-on%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can specify empty array as the default value for notes. And you don't need to return a function for the SubDocumentSchema. Try the below edited code.
const SubDocumentSchema = new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema],
default:
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
add a comment |
You can specify empty array as the default value for notes. And you don't need to return a function for the SubDocumentSchema. Try the below edited code.
const SubDocumentSchema = new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema],
default:
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
add a comment |
You can specify empty array as the default value for notes. And you don't need to return a function for the SubDocumentSchema. Try the below edited code.
const SubDocumentSchema = new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema],
default:
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
You can specify empty array as the default value for notes. And you don't need to return a function for the SubDocumentSchema. Try the below edited code.
const SubDocumentSchema = new mongoose.Schema({
content: {
type: String,
trim: true
},
date: {
type: Date,
default: Date.now
}
})
const DocumentSchema = new mongoose.Schema({
notes: {
type: [SubDocumentSchema],
default:
}
});
const Document = mongooseConnection.model('DocumentSchema', DocumentSchema)
const t = new Document()
t.save()
answered Nov 14 '18 at 7:06


Karthik SamyakKarthik Samyak
121110
121110
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292993%2fsub-document-in-an-array-saves-as-an-empty-array-item-if-no-value-is-provided-on%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cF brkQ5HFelQ8s u33,PcSUJ9,JLJ 1JnawxxCRcwKCuc9SWswU5qpdnmhVpXWSeKWPipzaRo82sUEZn77Y
1
What is
NotesSchema
? Not in your code. It also probably should betype: [NotesSchema]
since the()
implies invoking a function, not assigning a "schema type" as you should be doing here.– Neil Lunn
Nov 14 '18 at 4:02
Sorry, I fixed that. I actually changed the schema to just
new....
without being returned from a function, but I'm still get an array with an empty first value. I hope that makes sense.– Modermo
Nov 14 '18 at 4:33
1
Again you don't do
new
. Just do it like I already ( and as the documentation also does ) showed you.type: [SubDocumentSchema]
. Nonew
and no brackets()
. You use it there just like the second argument in yourmongoose.model
call.model('DocumentSchema', DocumentSchema)
where again, nonew
and no brackets()
.– Neil Lunn
Nov 14 '18 at 4:37
I could be misunderstanding, but the Mongoose docs do specify instantiating a new schema sub-doc.
– Modermo
Nov 14 '18 at 5:07
1
You're misunderstanding.
const SubDocumentSchema = new Schema({ ...
It's the same for "all schema" whether it's the root of the model, or in an array or just a property. So nofunction()
, no returning a function and certainly no invoking. The "invocation" is actually what is creating the "empty string". See also mongoosejs.com/docs/subdocs.html– Neil Lunn
Nov 14 '18 at 5:11