How can i sort my list of names alphabetically?
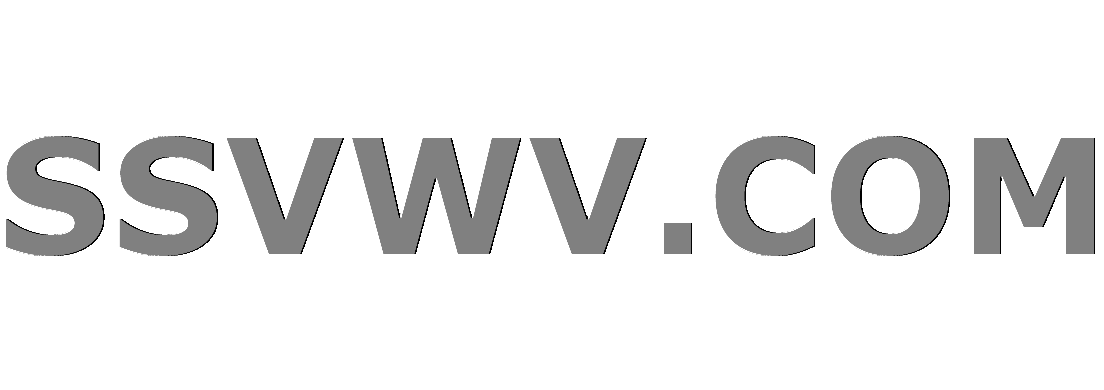
Multi tool use
I would like to know, how can i sort the names and surenames alphabetically in my list.
I'm not sure, but i googled and i'm guessing it only sort's by the name.
public void FilterParticipants(List<string> players, PlayerContainer allPlayers)
{
for (int i = 0; i < allPlayers.Count; i++)
{
if (!players.Contains(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i)))
{
players.Add(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i));
}
}
players.Sort();
}
c#
|
show 2 more comments
I would like to know, how can i sort the names and surenames alphabetically in my list.
I'm not sure, but i googled and i'm guessing it only sort's by the name.
public void FilterParticipants(List<string> players, PlayerContainer allPlayers)
{
for (int i = 0; i < allPlayers.Count; i++)
{
if (!players.Contains(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i)))
{
players.Add(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i));
}
}
players.Sort();
}
c#
5
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
4
The short answer is that you must have aList<Player>
rather thanList<string>
. Then you will do something likevar players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to aList<string>
in the order that you want it.
– mjwills
Nov 14 '18 at 20:27
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
2
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But addingref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.
– mjwills
Nov 14 '18 at 20:31
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33
|
show 2 more comments
I would like to know, how can i sort the names and surenames alphabetically in my list.
I'm not sure, but i googled and i'm guessing it only sort's by the name.
public void FilterParticipants(List<string> players, PlayerContainer allPlayers)
{
for (int i = 0; i < allPlayers.Count; i++)
{
if (!players.Contains(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i)))
{
players.Add(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i));
}
}
players.Sort();
}
c#
I would like to know, how can i sort the names and surenames alphabetically in my list.
I'm not sure, but i googled and i'm guessing it only sort's by the name.
public void FilterParticipants(List<string> players, PlayerContainer allPlayers)
{
for (int i = 0; i < allPlayers.Count; i++)
{
if (!players.Contains(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i)))
{
players.Add(allPlayers.FindName(i) + " " + allPlayers.FindSurname(i));
}
}
players.Sort();
}
c#
c#
asked Nov 14 '18 at 20:23
DomDom
12
12
5
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
4
The short answer is that you must have aList<Player>
rather thanList<string>
. Then you will do something likevar players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to aList<string>
in the order that you want it.
– mjwills
Nov 14 '18 at 20:27
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
2
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But addingref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.
– mjwills
Nov 14 '18 at 20:31
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33
|
show 2 more comments
5
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
4
The short answer is that you must have aList<Player>
rather thanList<string>
. Then you will do something likevar players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to aList<string>
in the order that you want it.
– mjwills
Nov 14 '18 at 20:27
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
2
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But addingref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.
– mjwills
Nov 14 '18 at 20:31
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33
5
5
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
4
4
The short answer is that you must have a
List<Player>
rather than List<string>
. Then you will do something like var players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to a List<string>
in the order that you want it.– mjwills
Nov 14 '18 at 20:27
The short answer is that you must have a
List<Player>
rather than List<string>
. Then you will do something like var players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to a List<string>
in the order that you want it.– mjwills
Nov 14 '18 at 20:27
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
2
2
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But adding
ref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.– mjwills
Nov 14 '18 at 20:31
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But adding
ref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.– mjwills
Nov 14 '18 at 20:31
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33
|
show 2 more comments
1 Answer
1
active
oldest
votes
If you want to sort your player names by Surname and then Name and cannot change your design to have a List<Player>
passed in, then here's one solution.
Note there's a slight design change, as it's usually better to return a new list rather than modifying the input list. Also, the method name is a little misleading. "Filter" implies that you're reducing the set of items based on some criteria, but in this case we're adding items if they don't exist, so I renamed it to GetCombinedParticipants
.
Given that, here's one way you could implement it. Note that this design uses Substring
to find the last space in the name, which is used as a delimeter between the first name and the last name (which therefore assumes that there are no spaces in the last name). If there are, then I don't know how you could possibly identify them from a List<string>
, which is another good reason to create a Player
class with separate FirstName
and Surname
poperties...
public List<string> GetCombinedParticipants(List<string> players,
PlayerContainer allPlayers)
{
// Make a copy of the input list
var results = players.ToList();
for (int i = 0; i < allPlayers.Count; i++)
{
var fullName = $"{allPlayers.FindName(i)} {allPlayers.FindSurname(i)}";
if (!results.Contains(fullName)) results.Add(fullName);
}
// Order by last name, then by first name
return results
.OrderBy(name => name.Substring(name.LastIndexOf(" ") + 1))
.ThenBy(name => name.Substring(0, name.LastIndexOf(" ")))
.ToList();
}
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
|
show 4 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308207%2fhow-can-i-sort-my-list-of-names-alphabetically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to sort your player names by Surname and then Name and cannot change your design to have a List<Player>
passed in, then here's one solution.
Note there's a slight design change, as it's usually better to return a new list rather than modifying the input list. Also, the method name is a little misleading. "Filter" implies that you're reducing the set of items based on some criteria, but in this case we're adding items if they don't exist, so I renamed it to GetCombinedParticipants
.
Given that, here's one way you could implement it. Note that this design uses Substring
to find the last space in the name, which is used as a delimeter between the first name and the last name (which therefore assumes that there are no spaces in the last name). If there are, then I don't know how you could possibly identify them from a List<string>
, which is another good reason to create a Player
class with separate FirstName
and Surname
poperties...
public List<string> GetCombinedParticipants(List<string> players,
PlayerContainer allPlayers)
{
// Make a copy of the input list
var results = players.ToList();
for (int i = 0; i < allPlayers.Count; i++)
{
var fullName = $"{allPlayers.FindName(i)} {allPlayers.FindSurname(i)}";
if (!results.Contains(fullName)) results.Add(fullName);
}
// Order by last name, then by first name
return results
.OrderBy(name => name.Substring(name.LastIndexOf(" ") + 1))
.ThenBy(name => name.Substring(0, name.LastIndexOf(" ")))
.ToList();
}
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
|
show 4 more comments
If you want to sort your player names by Surname and then Name and cannot change your design to have a List<Player>
passed in, then here's one solution.
Note there's a slight design change, as it's usually better to return a new list rather than modifying the input list. Also, the method name is a little misleading. "Filter" implies that you're reducing the set of items based on some criteria, but in this case we're adding items if they don't exist, so I renamed it to GetCombinedParticipants
.
Given that, here's one way you could implement it. Note that this design uses Substring
to find the last space in the name, which is used as a delimeter between the first name and the last name (which therefore assumes that there are no spaces in the last name). If there are, then I don't know how you could possibly identify them from a List<string>
, which is another good reason to create a Player
class with separate FirstName
and Surname
poperties...
public List<string> GetCombinedParticipants(List<string> players,
PlayerContainer allPlayers)
{
// Make a copy of the input list
var results = players.ToList();
for (int i = 0; i < allPlayers.Count; i++)
{
var fullName = $"{allPlayers.FindName(i)} {allPlayers.FindSurname(i)}";
if (!results.Contains(fullName)) results.Add(fullName);
}
// Order by last name, then by first name
return results
.OrderBy(name => name.Substring(name.LastIndexOf(" ") + 1))
.ThenBy(name => name.Substring(0, name.LastIndexOf(" ")))
.ToList();
}
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
|
show 4 more comments
If you want to sort your player names by Surname and then Name and cannot change your design to have a List<Player>
passed in, then here's one solution.
Note there's a slight design change, as it's usually better to return a new list rather than modifying the input list. Also, the method name is a little misleading. "Filter" implies that you're reducing the set of items based on some criteria, but in this case we're adding items if they don't exist, so I renamed it to GetCombinedParticipants
.
Given that, here's one way you could implement it. Note that this design uses Substring
to find the last space in the name, which is used as a delimeter between the first name and the last name (which therefore assumes that there are no spaces in the last name). If there are, then I don't know how you could possibly identify them from a List<string>
, which is another good reason to create a Player
class with separate FirstName
and Surname
poperties...
public List<string> GetCombinedParticipants(List<string> players,
PlayerContainer allPlayers)
{
// Make a copy of the input list
var results = players.ToList();
for (int i = 0; i < allPlayers.Count; i++)
{
var fullName = $"{allPlayers.FindName(i)} {allPlayers.FindSurname(i)}";
if (!results.Contains(fullName)) results.Add(fullName);
}
// Order by last name, then by first name
return results
.OrderBy(name => name.Substring(name.LastIndexOf(" ") + 1))
.ThenBy(name => name.Substring(0, name.LastIndexOf(" ")))
.ToList();
}
If you want to sort your player names by Surname and then Name and cannot change your design to have a List<Player>
passed in, then here's one solution.
Note there's a slight design change, as it's usually better to return a new list rather than modifying the input list. Also, the method name is a little misleading. "Filter" implies that you're reducing the set of items based on some criteria, but in this case we're adding items if they don't exist, so I renamed it to GetCombinedParticipants
.
Given that, here's one way you could implement it. Note that this design uses Substring
to find the last space in the name, which is used as a delimeter between the first name and the last name (which therefore assumes that there are no spaces in the last name). If there are, then I don't know how you could possibly identify them from a List<string>
, which is another good reason to create a Player
class with separate FirstName
and Surname
poperties...
public List<string> GetCombinedParticipants(List<string> players,
PlayerContainer allPlayers)
{
// Make a copy of the input list
var results = players.ToList();
for (int i = 0; i < allPlayers.Count; i++)
{
var fullName = $"{allPlayers.FindName(i)} {allPlayers.FindSurname(i)}";
if (!results.Contains(fullName)) results.Add(fullName);
}
// Order by last name, then by first name
return results
.OrderBy(name => name.Substring(name.LastIndexOf(" ") + 1))
.ThenBy(name => name.Substring(0, name.LastIndexOf(" ")))
.ToList();
}
edited Nov 14 '18 at 22:23
answered Nov 14 '18 at 21:02


Rufus LRufus L
17.7k31531
17.7k31531
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
|
show 4 more comments
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
1
1
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
This relies on people having no spaces in their first or surname.
– mjwills
Nov 14 '18 at 21:11
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
@mjwills Isn't that a fair assumption? Unless I am seriously culture blind here
– Cubemaster
Nov 14 '18 at 21:18
1
1
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
@Cubemaster w3.org/International/questions/qa-personal-names "because some people consider a space-separated sequence of characters to be a single name, eg. Rose Marie." brians.wsu.edu/2016/05/25/multipart-names could be even worse for surnames.
– mjwills
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
I like that you are trying to anticipate their needs, and yet hesitant to like a solution that doesn't properly structure the data. For instance: what if two players share a name? How will you differentiate the surname? It looks like you are relying upon the list of player names being synchronized with the index that they appear in the PlayerContainer, but that doesn't sound like a sound solution to me. Since this is supposed to serve as a filter, it would be bad to assume that the indeces of the player names you are filtering by correspond with the player indeces in the larger PlayerContainer
– Ryan Pierce Williams
Nov 14 '18 at 21:20
1
1
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
Well would you look at that. There's a standard for this stuff.
– Cubemaster
Nov 14 '18 at 21:21
|
show 4 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308207%2fhow-can-i-sort-my-list-of-names-alphabetically%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NTj6oJRWpW4 bKsblHu9mfBKd5Gm260qSb,8T 8YqFHs,KtPPDt,7,ESO
5
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 20:25
4
The short answer is that you must have a
List<Player>
rather thanList<string>
. Then you will do something likevar players = playerList.OrderBy(z -> z.FirstName).ThenBy(z => z.Surname).Select(z => z.FirstName + " " + z.Surname).ToList();
to convert that to aList<string>
in the order that you want it.– mjwills
Nov 14 '18 at 20:27
it's usually bad form to modify inputs in a method without requiring the ref keyword.
– Felix Castor
Nov 14 '18 at 20:28
2
@FelixCastor Is sorting the inputs a bad idea? Yes, yes it is. But adding
ref
would make it even more confusing, since it would imply that the called code isn't sorting the data in place but is instead assigning a new array to it - which it isn't.– mjwills
Nov 14 '18 at 20:31
@mjwills fair point.
– Felix Castor
Nov 14 '18 at 20:33