Jackson deserialize nulls to empty list with no access to entity
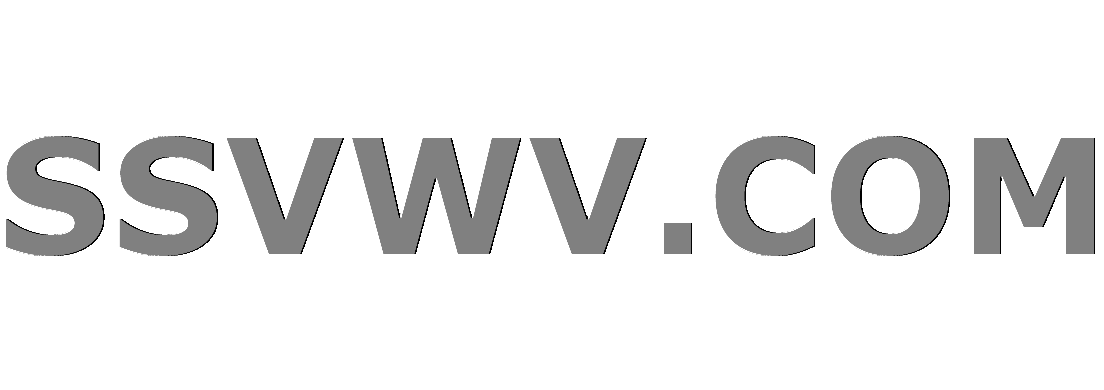
Multi tool use
up vote
0
down vote
favorite
What I am trying to achieve:
to deserialize null values to empty lists
Note: I cannot change/annotate the entities, because they do come from a jar.
I have the following code:
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
public class MyJsonMapperList extends ObjectMapper{
private static final MyJsonMapperList jsonMapper = new MyJsonMapperList();
public MyJsonMapperList() {
SimpleModule sm = new SimpleModule();
sm.addDeserializer(List.class, new NullToEmptyListDeserializer());
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
private static class NullToEmptyListDeserializer extends JsonDeserializer<List> {
@Override
public List deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper mapper = new ObjectMapper();
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forContentNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY, Nulls.AS_EMPTY));
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
}
public static <T> T fromJson(String json, Class<T> tClass) throws IOException {
if (json == null) { return null; }
return jsonMapper.readerFor(tClass).readValue(json);
}
public static String toJson(Object bean) throws JsonProcessingException {
if (bean == null) { return null; }
return jsonMapper.writer().writeValueAsString(bean);
}
The source for this code is from here.
Did I interpret something incorrectly from the Kotlin code provided by shyiko?
*Note I've tried also directly with setDeserializerModifier like:
public MyJsonMapperList () {
SimpleModule sm = new SimpleModule();
// sm.addDeserializer(CollectionType.class, new NullToEmptyListDeserializer ());
sm.setDeserializerModifier(new BeanDeserializerModifier() {
@Override
public JsonDeserializer<?> modifyCollectionDeserializer(DeserializationConfig config, CollectionType type, BeanDescription beanDesc, JsonDeserializer<?> deserializer) {
deserializer = new JsonDeserializer<List<?>>() {
@Override
public List<?> deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
};
return deserializer;
}
});
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
The error which I get:
java.lang.IllegalArgumentException: Cannot handle managed/back reference 'defaultReference': type: value deserializer of type MyJsonMapperList$NullToEmptyListDeserializer does not support them
at com.fasterxml.jackson.databind.JsonDeserializer.findBackReference(JsonDeserializer.java:365)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase._resolveManagedReferenceProperty(BeanDeserializerBase.java:764)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.resolve(BeanDeserializerBase.java:474)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCache2(DeserializerCache.java:293)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCacheValueDeserializer(DeserializerCache.java:244)
at com.fasterxml.jackson.databind.deser.DeserializerCache.findValueDeserializer(DeserializerCache.java:142)
at com.fasterxml.jackson.databind.DeserializationContext.findRootValueDeserializer(DeserializationContext.java:477)
at com.fasterxml.jackson.databind.ObjectReader._prefetchRootDeserializer(ObjectReader.java:1935)
at com.fasterxml.jackson.databind.ObjectReader.<init>(ObjectReader.java:189)
at com.fasterxml.jackson.databind.ObjectMapper._newReader(ObjectMapper.java:658)
at com.fasterxml.jackson.databind.ObjectMapper.readerFor(ObjectMapper.java:3517)
at ...MyJsonMapperList.fromJson(MyJsonMapperList.java:47)
Entities do not have any @JsonManagedReference and @JsonBackReference annotations (even if they had, could not modify them)
Jackson dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.0</version>
</dependency>
What am I missing?
Are there other possibilities which I ommitted?
This answer sounded promising, but you don't have access to objectMapperBuilder because of the protected access.
java json jackson deserialization jackson-databind
add a comment |
up vote
0
down vote
favorite
What I am trying to achieve:
to deserialize null values to empty lists
Note: I cannot change/annotate the entities, because they do come from a jar.
I have the following code:
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
public class MyJsonMapperList extends ObjectMapper{
private static final MyJsonMapperList jsonMapper = new MyJsonMapperList();
public MyJsonMapperList() {
SimpleModule sm = new SimpleModule();
sm.addDeserializer(List.class, new NullToEmptyListDeserializer());
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
private static class NullToEmptyListDeserializer extends JsonDeserializer<List> {
@Override
public List deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper mapper = new ObjectMapper();
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forContentNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY, Nulls.AS_EMPTY));
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
}
public static <T> T fromJson(String json, Class<T> tClass) throws IOException {
if (json == null) { return null; }
return jsonMapper.readerFor(tClass).readValue(json);
}
public static String toJson(Object bean) throws JsonProcessingException {
if (bean == null) { return null; }
return jsonMapper.writer().writeValueAsString(bean);
}
The source for this code is from here.
Did I interpret something incorrectly from the Kotlin code provided by shyiko?
*Note I've tried also directly with setDeserializerModifier like:
public MyJsonMapperList () {
SimpleModule sm = new SimpleModule();
// sm.addDeserializer(CollectionType.class, new NullToEmptyListDeserializer ());
sm.setDeserializerModifier(new BeanDeserializerModifier() {
@Override
public JsonDeserializer<?> modifyCollectionDeserializer(DeserializationConfig config, CollectionType type, BeanDescription beanDesc, JsonDeserializer<?> deserializer) {
deserializer = new JsonDeserializer<List<?>>() {
@Override
public List<?> deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
};
return deserializer;
}
});
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
The error which I get:
java.lang.IllegalArgumentException: Cannot handle managed/back reference 'defaultReference': type: value deserializer of type MyJsonMapperList$NullToEmptyListDeserializer does not support them
at com.fasterxml.jackson.databind.JsonDeserializer.findBackReference(JsonDeserializer.java:365)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase._resolveManagedReferenceProperty(BeanDeserializerBase.java:764)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.resolve(BeanDeserializerBase.java:474)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCache2(DeserializerCache.java:293)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCacheValueDeserializer(DeserializerCache.java:244)
at com.fasterxml.jackson.databind.deser.DeserializerCache.findValueDeserializer(DeserializerCache.java:142)
at com.fasterxml.jackson.databind.DeserializationContext.findRootValueDeserializer(DeserializationContext.java:477)
at com.fasterxml.jackson.databind.ObjectReader._prefetchRootDeserializer(ObjectReader.java:1935)
at com.fasterxml.jackson.databind.ObjectReader.<init>(ObjectReader.java:189)
at com.fasterxml.jackson.databind.ObjectMapper._newReader(ObjectMapper.java:658)
at com.fasterxml.jackson.databind.ObjectMapper.readerFor(ObjectMapper.java:3517)
at ...MyJsonMapperList.fromJson(MyJsonMapperList.java:47)
Entities do not have any @JsonManagedReference and @JsonBackReference annotations (even if they had, could not modify them)
Jackson dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.0</version>
</dependency>
What am I missing?
Are there other possibilities which I ommitted?
This answer sounded promising, but you don't have access to objectMapperBuilder because of the protected access.
java json jackson deserialization jackson-databind
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
What I am trying to achieve:
to deserialize null values to empty lists
Note: I cannot change/annotate the entities, because they do come from a jar.
I have the following code:
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
public class MyJsonMapperList extends ObjectMapper{
private static final MyJsonMapperList jsonMapper = new MyJsonMapperList();
public MyJsonMapperList() {
SimpleModule sm = new SimpleModule();
sm.addDeserializer(List.class, new NullToEmptyListDeserializer());
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
private static class NullToEmptyListDeserializer extends JsonDeserializer<List> {
@Override
public List deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper mapper = new ObjectMapper();
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forContentNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY, Nulls.AS_EMPTY));
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
}
public static <T> T fromJson(String json, Class<T> tClass) throws IOException {
if (json == null) { return null; }
return jsonMapper.readerFor(tClass).readValue(json);
}
public static String toJson(Object bean) throws JsonProcessingException {
if (bean == null) { return null; }
return jsonMapper.writer().writeValueAsString(bean);
}
The source for this code is from here.
Did I interpret something incorrectly from the Kotlin code provided by shyiko?
*Note I've tried also directly with setDeserializerModifier like:
public MyJsonMapperList () {
SimpleModule sm = new SimpleModule();
// sm.addDeserializer(CollectionType.class, new NullToEmptyListDeserializer ());
sm.setDeserializerModifier(new BeanDeserializerModifier() {
@Override
public JsonDeserializer<?> modifyCollectionDeserializer(DeserializationConfig config, CollectionType type, BeanDescription beanDesc, JsonDeserializer<?> deserializer) {
deserializer = new JsonDeserializer<List<?>>() {
@Override
public List<?> deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
};
return deserializer;
}
});
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
The error which I get:
java.lang.IllegalArgumentException: Cannot handle managed/back reference 'defaultReference': type: value deserializer of type MyJsonMapperList$NullToEmptyListDeserializer does not support them
at com.fasterxml.jackson.databind.JsonDeserializer.findBackReference(JsonDeserializer.java:365)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase._resolveManagedReferenceProperty(BeanDeserializerBase.java:764)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.resolve(BeanDeserializerBase.java:474)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCache2(DeserializerCache.java:293)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCacheValueDeserializer(DeserializerCache.java:244)
at com.fasterxml.jackson.databind.deser.DeserializerCache.findValueDeserializer(DeserializerCache.java:142)
at com.fasterxml.jackson.databind.DeserializationContext.findRootValueDeserializer(DeserializationContext.java:477)
at com.fasterxml.jackson.databind.ObjectReader._prefetchRootDeserializer(ObjectReader.java:1935)
at com.fasterxml.jackson.databind.ObjectReader.<init>(ObjectReader.java:189)
at com.fasterxml.jackson.databind.ObjectMapper._newReader(ObjectMapper.java:658)
at com.fasterxml.jackson.databind.ObjectMapper.readerFor(ObjectMapper.java:3517)
at ...MyJsonMapperList.fromJson(MyJsonMapperList.java:47)
Entities do not have any @JsonManagedReference and @JsonBackReference annotations (even if they had, could not modify them)
Jackson dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.0</version>
</dependency>
What am I missing?
Are there other possibilities which I ommitted?
This answer sounded promising, but you don't have access to objectMapperBuilder because of the protected access.
java json jackson deserialization jackson-databind
What I am trying to achieve:
to deserialize null values to empty lists
Note: I cannot change/annotate the entities, because they do come from a jar.
I have the following code:
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
public class MyJsonMapperList extends ObjectMapper{
private static final MyJsonMapperList jsonMapper = new MyJsonMapperList();
public MyJsonMapperList() {
SimpleModule sm = new SimpleModule();
sm.addDeserializer(List.class, new NullToEmptyListDeserializer());
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
private static class NullToEmptyListDeserializer extends JsonDeserializer<List> {
@Override
public List deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
ObjectMapper mapper = new ObjectMapper();
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forContentNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY));
mapper.configOverride(List.class).setSetterInfo(JsonSetter.Value.forValueNulls(Nulls.AS_EMPTY, Nulls.AS_EMPTY));
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
}
public static <T> T fromJson(String json, Class<T> tClass) throws IOException {
if (json == null) { return null; }
return jsonMapper.readerFor(tClass).readValue(json);
}
public static String toJson(Object bean) throws JsonProcessingException {
if (bean == null) { return null; }
return jsonMapper.writer().writeValueAsString(bean);
}
The source for this code is from here.
Did I interpret something incorrectly from the Kotlin code provided by shyiko?
*Note I've tried also directly with setDeserializerModifier like:
public MyJsonMapperList () {
SimpleModule sm = new SimpleModule();
// sm.addDeserializer(CollectionType.class, new NullToEmptyListDeserializer ());
sm.setDeserializerModifier(new BeanDeserializerModifier() {
@Override
public JsonDeserializer<?> modifyCollectionDeserializer(DeserializationConfig config, CollectionType type, BeanDescription beanDesc, JsonDeserializer<?> deserializer) {
deserializer = new JsonDeserializer<List<?>>() {
@Override
public List<?> deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException {
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jsonParser, new TypeReference<List>(){});
}
@Override
public List getNullValue(DeserializationContext ctx) {
return Collections.emptyList();
}
};
return deserializer;
}
});
super.registerModule(sm);
// setSerializationInclusion(JsonInclude.Include.NON_NULL);
// disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
}
The error which I get:
java.lang.IllegalArgumentException: Cannot handle managed/back reference 'defaultReference': type: value deserializer of type MyJsonMapperList$NullToEmptyListDeserializer does not support them
at com.fasterxml.jackson.databind.JsonDeserializer.findBackReference(JsonDeserializer.java:365)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase._resolveManagedReferenceProperty(BeanDeserializerBase.java:764)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.resolve(BeanDeserializerBase.java:474)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCache2(DeserializerCache.java:293)
at com.fasterxml.jackson.databind.deser.DeserializerCache._createAndCacheValueDeserializer(DeserializerCache.java:244)
at com.fasterxml.jackson.databind.deser.DeserializerCache.findValueDeserializer(DeserializerCache.java:142)
at com.fasterxml.jackson.databind.DeserializationContext.findRootValueDeserializer(DeserializationContext.java:477)
at com.fasterxml.jackson.databind.ObjectReader._prefetchRootDeserializer(ObjectReader.java:1935)
at com.fasterxml.jackson.databind.ObjectReader.<init>(ObjectReader.java:189)
at com.fasterxml.jackson.databind.ObjectMapper._newReader(ObjectMapper.java:658)
at com.fasterxml.jackson.databind.ObjectMapper.readerFor(ObjectMapper.java:3517)
at ...MyJsonMapperList.fromJson(MyJsonMapperList.java:47)
Entities do not have any @JsonManagedReference and @JsonBackReference annotations (even if they had, could not modify them)
Jackson dependency:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.0</version>
</dependency>
What am I missing?
Are there other possibilities which I ommitted?
This answer sounded promising, but you don't have access to objectMapperBuilder because of the protected access.
java json jackson deserialization jackson-databind
java json jackson deserialization jackson-databind
edited Nov 7 at 13:49
asked Nov 7 at 13:43


David Laci
437
437
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53190680%2fjackson-deserialize-nulls-to-empty-list-with-no-access-to-entity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6yAdSCspQ9p H0,tLXT,UienGQhKwybIWy XX4 IyI RfSrKC9DJC1GpAD,8BK7N55Iy4Fq7 2FtKh