How to append new Array in JSON file stored in android
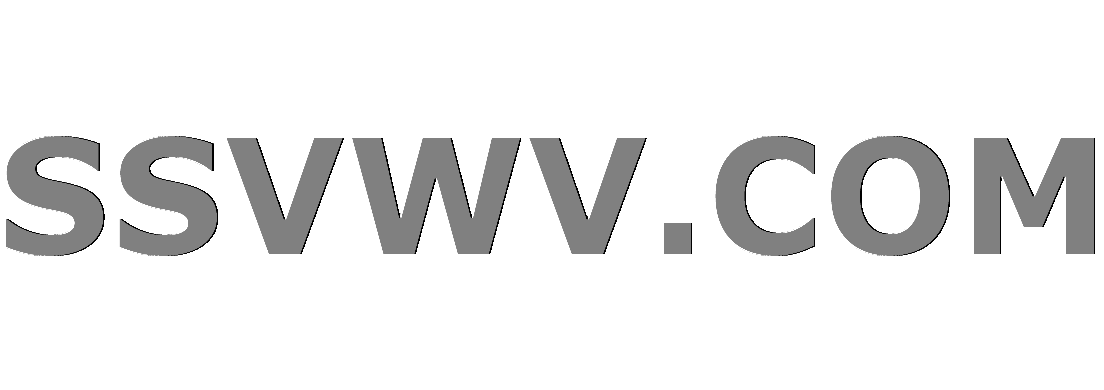
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have one JSON file in my app's data folder.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
]
}
Now I want to add a new user at runtime like this.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
],
"user": [
{
"identifier": "2",
"name": "abc",
"contact": [
{
"contact": "445"
},
{
"contact": "789"
}
]
}
]
}
For that, I have this code but it simply overrides the existing user.
String lastObject = getStringFronFile(new FileInputStream(file));
try {
JSONObject prevJSONObj = new JSONObject(lastObject);
prevJSONObj.put("sticker_packs",newUserArray);
writeJsonFile(file,prevJSONObj);
}
catch (Exception e) {
Log.d("Json Error", e.toString());
}
writeJsonFile
is a method for writing in the file.
Any help will be appreciated.


add a comment |
I have one JSON file in my app's data folder.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
]
}
Now I want to add a new user at runtime like this.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
],
"user": [
{
"identifier": "2",
"name": "abc",
"contact": [
{
"contact": "445"
},
{
"contact": "789"
}
]
}
]
}
For that, I have this code but it simply overrides the existing user.
String lastObject = getStringFronFile(new FileInputStream(file));
try {
JSONObject prevJSONObj = new JSONObject(lastObject);
prevJSONObj.put("sticker_packs",newUserArray);
writeJsonFile(file,prevJSONObj);
}
catch (Exception e) {
Log.d("Json Error", e.toString());
}
writeJsonFile
is a method for writing in the file.
Any help will be appreciated.


add a comment |
I have one JSON file in my app's data folder.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
]
}
Now I want to add a new user at runtime like this.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
],
"user": [
{
"identifier": "2",
"name": "abc",
"contact": [
{
"contact": "445"
},
{
"contact": "789"
}
]
}
]
}
For that, I have this code but it simply overrides the existing user.
String lastObject = getStringFronFile(new FileInputStream(file));
try {
JSONObject prevJSONObj = new JSONObject(lastObject);
prevJSONObj.put("sticker_packs",newUserArray);
writeJsonFile(file,prevJSONObj);
}
catch (Exception e) {
Log.d("Json Error", e.toString());
}
writeJsonFile
is a method for writing in the file.
Any help will be appreciated.


I have one JSON file in my app's data folder.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
]
}
Now I want to add a new user at runtime like this.
{
"user": [
{
"identifier": "1",
"name": "xyz",
"contact": [
{
"contact": "123"
},
{
"contact": "456"
}
]
}
],
"user": [
{
"identifier": "2",
"name": "abc",
"contact": [
{
"contact": "445"
},
{
"contact": "789"
}
]
}
]
}
For that, I have this code but it simply overrides the existing user.
String lastObject = getStringFronFile(new FileInputStream(file));
try {
JSONObject prevJSONObj = new JSONObject(lastObject);
prevJSONObj.put("sticker_packs",newUserArray);
writeJsonFile(file,prevJSONObj);
}
catch (Exception e) {
Log.d("Json Error", e.toString());
}
writeJsonFile
is a method for writing in the file.
Any help will be appreciated.




asked Nov 25 '18 at 7:22
PATEL MEETPATEL MEET
206
206
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The structure you defined above is wrong, you cannot define two node with the same name user
in a JSONObject.
If you want to store a list of user in to a file, you may store it like:
[
{
"name":"Jack",
"age" :20
},
{
"name":"Tom",
"age" :20
}
]
By the way, why not use SQLite database?
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
add a comment |
Gson simplifies this work. Add the gson dependency https://github.com/google/gson or retrofit's gson (both are same)
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
. From your details constructed the following pojo classes :
public class Example {
@SerializedName("user")
@Expose
private List<User> user = null;
public List<User> getUser() {
return user;
}
public void setUser(List<User> user) {
this.user = user;
}
}
public class User {
@SerializedName("identifier")
@Expose
private String identifier;
@SerializedName("name")
@Expose
private String name;
@SerializedName("contact")
@Expose
private List<Contact> contact = null;
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Contact> getContact() {
return contact;
}
public void setContact(List<Contact> contact) {
this.contact = contact;
}
}
public class Contact {
@SerializedName("contact")
@Expose
private String contact;
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
}
You can use the following snippet to get details from string and append your dynamic data and update the file.
String lastObject = getStringFromFile(new FileInputStream(file));
Example newJsonToReplace = new Example();
List<User> usersList = new ArrayList();
try{
Type typeOfObject = new TypeToken<Example>() {
}.getType(); // type you will be getting from string
Example example = new Gson().fromJson(lastObject, typeOfObject); // converting string to pojo using gson
if(example != null){
usersList.addAll(example.getUser());
}
usersList.add(yourYourToAdd); // add your user object
newJsonToReplace.setUser(usersList);
String updatedList = new Gson().toJson(newJsonToReplace, typeOfObject); // again converting to json to string
writeJsonFile(updatedList); // will replace the existing content
}catch(Exception exception){
Log.d("Json Error", e.toString());
}
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465478%2fhow-to-append-new-array-in-json-file-stored-in-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The structure you defined above is wrong, you cannot define two node with the same name user
in a JSONObject.
If you want to store a list of user in to a file, you may store it like:
[
{
"name":"Jack",
"age" :20
},
{
"name":"Tom",
"age" :20
}
]
By the way, why not use SQLite database?
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
add a comment |
The structure you defined above is wrong, you cannot define two node with the same name user
in a JSONObject.
If you want to store a list of user in to a file, you may store it like:
[
{
"name":"Jack",
"age" :20
},
{
"name":"Tom",
"age" :20
}
]
By the way, why not use SQLite database?
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
add a comment |
The structure you defined above is wrong, you cannot define two node with the same name user
in a JSONObject.
If you want to store a list of user in to a file, you may store it like:
[
{
"name":"Jack",
"age" :20
},
{
"name":"Tom",
"age" :20
}
]
By the way, why not use SQLite database?
The structure you defined above is wrong, you cannot define two node with the same name user
in a JSONObject.
If you want to store a list of user in to a file, you may store it like:
[
{
"name":"Jack",
"age" :20
},
{
"name":"Tom",
"age" :20
}
]
By the way, why not use SQLite database?
answered Nov 25 '18 at 7:37


XieEDeHeiShouXieEDeHeiShou
1008
1008
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
add a comment |
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
Thanks for giving you valuable answer. I am new to android so I ma learning and I am surely use SQLite database in future.
– PATEL MEET
Nov 25 '18 at 7:54
add a comment |
Gson simplifies this work. Add the gson dependency https://github.com/google/gson or retrofit's gson (both are same)
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
. From your details constructed the following pojo classes :
public class Example {
@SerializedName("user")
@Expose
private List<User> user = null;
public List<User> getUser() {
return user;
}
public void setUser(List<User> user) {
this.user = user;
}
}
public class User {
@SerializedName("identifier")
@Expose
private String identifier;
@SerializedName("name")
@Expose
private String name;
@SerializedName("contact")
@Expose
private List<Contact> contact = null;
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Contact> getContact() {
return contact;
}
public void setContact(List<Contact> contact) {
this.contact = contact;
}
}
public class Contact {
@SerializedName("contact")
@Expose
private String contact;
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
}
You can use the following snippet to get details from string and append your dynamic data and update the file.
String lastObject = getStringFromFile(new FileInputStream(file));
Example newJsonToReplace = new Example();
List<User> usersList = new ArrayList();
try{
Type typeOfObject = new TypeToken<Example>() {
}.getType(); // type you will be getting from string
Example example = new Gson().fromJson(lastObject, typeOfObject); // converting string to pojo using gson
if(example != null){
usersList.addAll(example.getUser());
}
usersList.add(yourYourToAdd); // add your user object
newJsonToReplace.setUser(usersList);
String updatedList = new Gson().toJson(newJsonToReplace, typeOfObject); // again converting to json to string
writeJsonFile(updatedList); // will replace the existing content
}catch(Exception exception){
Log.d("Json Error", e.toString());
}
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
add a comment |
Gson simplifies this work. Add the gson dependency https://github.com/google/gson or retrofit's gson (both are same)
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
. From your details constructed the following pojo classes :
public class Example {
@SerializedName("user")
@Expose
private List<User> user = null;
public List<User> getUser() {
return user;
}
public void setUser(List<User> user) {
this.user = user;
}
}
public class User {
@SerializedName("identifier")
@Expose
private String identifier;
@SerializedName("name")
@Expose
private String name;
@SerializedName("contact")
@Expose
private List<Contact> contact = null;
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Contact> getContact() {
return contact;
}
public void setContact(List<Contact> contact) {
this.contact = contact;
}
}
public class Contact {
@SerializedName("contact")
@Expose
private String contact;
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
}
You can use the following snippet to get details from string and append your dynamic data and update the file.
String lastObject = getStringFromFile(new FileInputStream(file));
Example newJsonToReplace = new Example();
List<User> usersList = new ArrayList();
try{
Type typeOfObject = new TypeToken<Example>() {
}.getType(); // type you will be getting from string
Example example = new Gson().fromJson(lastObject, typeOfObject); // converting string to pojo using gson
if(example != null){
usersList.addAll(example.getUser());
}
usersList.add(yourYourToAdd); // add your user object
newJsonToReplace.setUser(usersList);
String updatedList = new Gson().toJson(newJsonToReplace, typeOfObject); // again converting to json to string
writeJsonFile(updatedList); // will replace the existing content
}catch(Exception exception){
Log.d("Json Error", e.toString());
}
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
add a comment |
Gson simplifies this work. Add the gson dependency https://github.com/google/gson or retrofit's gson (both are same)
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
. From your details constructed the following pojo classes :
public class Example {
@SerializedName("user")
@Expose
private List<User> user = null;
public List<User> getUser() {
return user;
}
public void setUser(List<User> user) {
this.user = user;
}
}
public class User {
@SerializedName("identifier")
@Expose
private String identifier;
@SerializedName("name")
@Expose
private String name;
@SerializedName("contact")
@Expose
private List<Contact> contact = null;
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Contact> getContact() {
return contact;
}
public void setContact(List<Contact> contact) {
this.contact = contact;
}
}
public class Contact {
@SerializedName("contact")
@Expose
private String contact;
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
}
You can use the following snippet to get details from string and append your dynamic data and update the file.
String lastObject = getStringFromFile(new FileInputStream(file));
Example newJsonToReplace = new Example();
List<User> usersList = new ArrayList();
try{
Type typeOfObject = new TypeToken<Example>() {
}.getType(); // type you will be getting from string
Example example = new Gson().fromJson(lastObject, typeOfObject); // converting string to pojo using gson
if(example != null){
usersList.addAll(example.getUser());
}
usersList.add(yourYourToAdd); // add your user object
newJsonToReplace.setUser(usersList);
String updatedList = new Gson().toJson(newJsonToReplace, typeOfObject); // again converting to json to string
writeJsonFile(updatedList); // will replace the existing content
}catch(Exception exception){
Log.d("Json Error", e.toString());
}
Gson simplifies this work. Add the gson dependency https://github.com/google/gson or retrofit's gson (both are same)
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
. From your details constructed the following pojo classes :
public class Example {
@SerializedName("user")
@Expose
private List<User> user = null;
public List<User> getUser() {
return user;
}
public void setUser(List<User> user) {
this.user = user;
}
}
public class User {
@SerializedName("identifier")
@Expose
private String identifier;
@SerializedName("name")
@Expose
private String name;
@SerializedName("contact")
@Expose
private List<Contact> contact = null;
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Contact> getContact() {
return contact;
}
public void setContact(List<Contact> contact) {
this.contact = contact;
}
}
public class Contact {
@SerializedName("contact")
@Expose
private String contact;
public String getContact() {
return contact;
}
public void setContact(String contact) {
this.contact = contact;
}
}
You can use the following snippet to get details from string and append your dynamic data and update the file.
String lastObject = getStringFromFile(new FileInputStream(file));
Example newJsonToReplace = new Example();
List<User> usersList = new ArrayList();
try{
Type typeOfObject = new TypeToken<Example>() {
}.getType(); // type you will be getting from string
Example example = new Gson().fromJson(lastObject, typeOfObject); // converting string to pojo using gson
if(example != null){
usersList.addAll(example.getUser());
}
usersList.add(yourYourToAdd); // add your user object
newJsonToReplace.setUser(usersList);
String updatedList = new Gson().toJson(newJsonToReplace, typeOfObject); // again converting to json to string
writeJsonFile(updatedList); // will replace the existing content
}catch(Exception exception){
Log.d("Json Error", e.toString());
}
answered Nov 25 '18 at 8:20
RajRaj
15016
15016
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
add a comment |
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
Thanks for your valuable answer it helps me a lot.
– PATEL MEET
Nov 25 '18 at 9:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465478%2fhow-to-append-new-array-in-json-file-stored-in-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h48AP7Mklb 40sUB9wN dMbiFHfbhwL w4aukMt2vJni G5MnUCRW,3RijSyVqzn4ms8VUX1 NDW4POzC9WyL5xKgY6RMyeb4Wh,Q