Issue with identification of Twitter object type
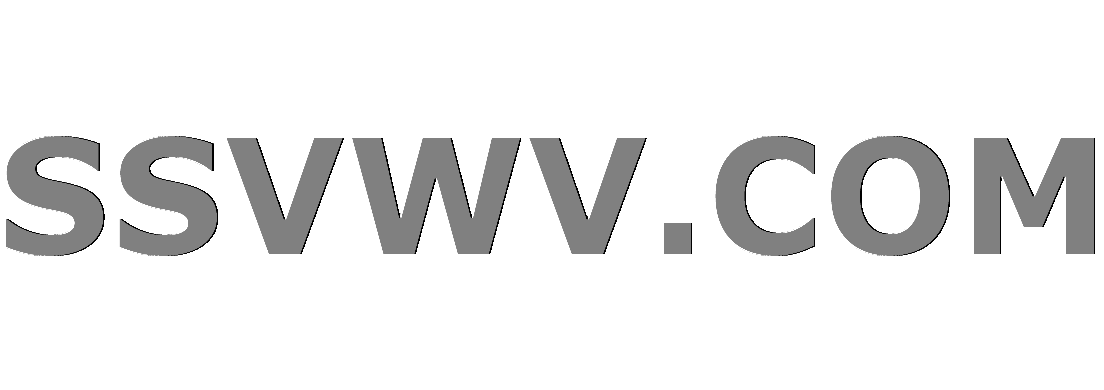
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
As per Twitter documentation. There are 3 kind of Status objects (Tweet, Retweets and Quote Tweets).
I am searching for related tweets based on tweet id and find there are cases where both retweeted_status (used to identify Retweets) and quoted_status (used to identify Quote Tweets) missing.
So wanted to know what these Tweet objects represent.
Code Snippet:
#Imports
import tweepy
import pandas as pd
import json
#Variables that contains the user credentials to access Twitter API
access_token = ACCESS_TOKEN
access_token_secret = ACCESS_TOKEN_SECRET
consumer_key = CONSUMER_KEY
consumer_secret = CONSUMER_SECRET
#Authenticate Twitter API
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
#Entity refers to person or thing who's tweets we are interested in
entity = api.get_user('realDonaldTrump')
#Get recent status/tweet of entity
set_result = api.user_timeline(id=entity.id, count=1)
# tweet is a tweepy.models.Status object
# https://developer.twitter.com/en/docs/tweets/data-dictionary/overview/tweet-object
tweet = set_result[0]
#Fetch related tweets
related_tweets =
for status in tweepy.Cursor(api.search,
q=tweet.id_str,
#lang='en',
tweet_mode='extended').items(500):
related_tweets.append(status)
tweetdf = pd.DataFrame()
#Build the dataframe with key information
...
tweetdf['retweeted'] = list(map(
lambda tweet: int(hasattr(tweet,'retweeted_status')),
related_tweets))
tweetdf['quoted'] = list(map(
lambda tweet: int(hasattr(tweet,'quoted_status')),
related_tweets))
print(len(tweetdf[(tweetdf["retweeted"] == 0) & (tweetdf["quoted"] == 0)]))
twitter tweepy
add a comment |
As per Twitter documentation. There are 3 kind of Status objects (Tweet, Retweets and Quote Tweets).
I am searching for related tweets based on tweet id and find there are cases where both retweeted_status (used to identify Retweets) and quoted_status (used to identify Quote Tweets) missing.
So wanted to know what these Tweet objects represent.
Code Snippet:
#Imports
import tweepy
import pandas as pd
import json
#Variables that contains the user credentials to access Twitter API
access_token = ACCESS_TOKEN
access_token_secret = ACCESS_TOKEN_SECRET
consumer_key = CONSUMER_KEY
consumer_secret = CONSUMER_SECRET
#Authenticate Twitter API
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
#Entity refers to person or thing who's tweets we are interested in
entity = api.get_user('realDonaldTrump')
#Get recent status/tweet of entity
set_result = api.user_timeline(id=entity.id, count=1)
# tweet is a tweepy.models.Status object
# https://developer.twitter.com/en/docs/tweets/data-dictionary/overview/tweet-object
tweet = set_result[0]
#Fetch related tweets
related_tweets =
for status in tweepy.Cursor(api.search,
q=tweet.id_str,
#lang='en',
tweet_mode='extended').items(500):
related_tweets.append(status)
tweetdf = pd.DataFrame()
#Build the dataframe with key information
...
tweetdf['retweeted'] = list(map(
lambda tweet: int(hasattr(tweet,'retweeted_status')),
related_tweets))
tweetdf['quoted'] = list(map(
lambda tweet: int(hasattr(tweet,'quoted_status')),
related_tweets))
print(len(tweetdf[(tweetdf["retweeted"] == 0) & (tweetdf["quoted"] == 0)]))
twitter tweepy
add a comment |
As per Twitter documentation. There are 3 kind of Status objects (Tweet, Retweets and Quote Tweets).
I am searching for related tweets based on tweet id and find there are cases where both retweeted_status (used to identify Retweets) and quoted_status (used to identify Quote Tweets) missing.
So wanted to know what these Tweet objects represent.
Code Snippet:
#Imports
import tweepy
import pandas as pd
import json
#Variables that contains the user credentials to access Twitter API
access_token = ACCESS_TOKEN
access_token_secret = ACCESS_TOKEN_SECRET
consumer_key = CONSUMER_KEY
consumer_secret = CONSUMER_SECRET
#Authenticate Twitter API
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
#Entity refers to person or thing who's tweets we are interested in
entity = api.get_user('realDonaldTrump')
#Get recent status/tweet of entity
set_result = api.user_timeline(id=entity.id, count=1)
# tweet is a tweepy.models.Status object
# https://developer.twitter.com/en/docs/tweets/data-dictionary/overview/tweet-object
tweet = set_result[0]
#Fetch related tweets
related_tweets =
for status in tweepy.Cursor(api.search,
q=tweet.id_str,
#lang='en',
tweet_mode='extended').items(500):
related_tweets.append(status)
tweetdf = pd.DataFrame()
#Build the dataframe with key information
...
tweetdf['retweeted'] = list(map(
lambda tweet: int(hasattr(tweet,'retweeted_status')),
related_tweets))
tweetdf['quoted'] = list(map(
lambda tweet: int(hasattr(tweet,'quoted_status')),
related_tweets))
print(len(tweetdf[(tweetdf["retweeted"] == 0) & (tweetdf["quoted"] == 0)]))
twitter tweepy
As per Twitter documentation. There are 3 kind of Status objects (Tweet, Retweets and Quote Tweets).
I am searching for related tweets based on tweet id and find there are cases where both retweeted_status (used to identify Retweets) and quoted_status (used to identify Quote Tweets) missing.
So wanted to know what these Tweet objects represent.
Code Snippet:
#Imports
import tweepy
import pandas as pd
import json
#Variables that contains the user credentials to access Twitter API
access_token = ACCESS_TOKEN
access_token_secret = ACCESS_TOKEN_SECRET
consumer_key = CONSUMER_KEY
consumer_secret = CONSUMER_SECRET
#Authenticate Twitter API
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
#Entity refers to person or thing who's tweets we are interested in
entity = api.get_user('realDonaldTrump')
#Get recent status/tweet of entity
set_result = api.user_timeline(id=entity.id, count=1)
# tweet is a tweepy.models.Status object
# https://developer.twitter.com/en/docs/tweets/data-dictionary/overview/tweet-object
tweet = set_result[0]
#Fetch related tweets
related_tweets =
for status in tweepy.Cursor(api.search,
q=tweet.id_str,
#lang='en',
tweet_mode='extended').items(500):
related_tweets.append(status)
tweetdf = pd.DataFrame()
#Build the dataframe with key information
...
tweetdf['retweeted'] = list(map(
lambda tweet: int(hasattr(tweet,'retweeted_status')),
related_tweets))
tweetdf['quoted'] = list(map(
lambda tweet: int(hasattr(tweet,'quoted_status')),
related_tweets))
print(len(tweetdf[(tweetdf["retweeted"] == 0) & (tweetdf["quoted"] == 0)]))
twitter tweepy
twitter tweepy
asked Nov 24 '18 at 9:49
Shreyas JShreyas J
11
11
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456980%2fissue-with-identification-of-twitter-object-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456980%2fissue-with-identification-of-twitter-object-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JfdVaO,BUWnGZ0l3A27Xb,cDbWCQepY53,MkW2x3e3drA5 mq1MwjGq49qXVaQdWsKt8,Yp7z6eaBscoJP