Scroll horizontally in programmatically created ScrollView
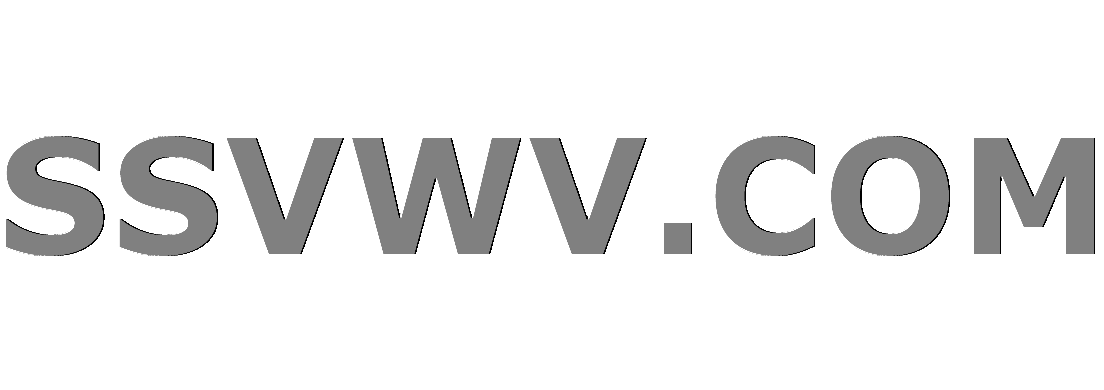
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm creating a ScrollView, nesting in a RelativeLayout (which contains tables). Everything is properly added to the layout. The problem is: vertically scrolling works fine, but horizontal scrolling does NOT work at all. I already tested various combinations of MATCH_PARENT
, WRAP_CONTENT
and FILL_PARENT
. No try even worked close. So my question is: What am I missing here?
Here is the code:
public class PlayerView extends View {
private RelativeLayout relativeLayout;
private TableLayout tableLayout;
private int player_loop;
private int player_count;
public PlayerView(Context context, int player_count){
super(context);
setPlayer_count(player_count);
}
public ScrollView create_scrollView(){
ScrollView scrollView = new ScrollView(getContext());
ScrollView.LayoutParams scroll_params = new ScrollView.LayoutParams(
ScrollView.LayoutParams.MATCH_PARENT,
ScrollView.LayoutParams.MATCH_PARENT
);
scrollView.setLayoutParams(scroll_params);
scrollView.addView(create_relativeLayout());
return scrollView;
}
public RelativeLayout create_relativeLayout(){
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
return relativeLayout;
}
public void create_Player_Table_Layout(){
for(player_loop = 1; player_loop <= player_count; player_loop++){
relativeLayout.addView(player_table(getResources().getString(R.string.dummy_player_name) + player_loop, player_loop));
}
}
public TableLayout player_table(String playername, int playernumber){
tableLayout = new TableLayout(getContext());
tableLayout.setId(playernumber * 1000);
if (playernumber > 1) {
//TABLE PLACEMENT
RelativeLayout.LayoutParams tbl_params_New = new RelativeLayout.LayoutParams(RelativeLayout.LayoutParams.WRAP_CONTENT, RelativeLayout.LayoutParams.WRAP_CONTENT);
tbl_params_New.addRule(RelativeLayout.RIGHT_OF, (playernumber - 1) * 1000);
tableLayout.setLayoutParams(tbl_params_New);
}
//Add Playername
TableRow row_playername = new TableRow(getContext());
TextView view_name = new TextView(getContext());
TableRow.LayoutParams view_name_params = new TableRow.LayoutParams();
view_name_params.setMargins(20,20,20,20);
view_name.setLayoutParams(view_name_params);
view_name.setGravity(Gravity.CENTER);
view_name.setText(playername);
view_name.setTextSize(20);
view_name.setId(playernumber * 100);
row_playername.addView(view_name);
tableLayout.addView(row_playername);
//Add Lifepoints
TableRow row_lifepoints = new TableRow(getContext());
TextView view_lifepoints = new TextView(getContext());
TableRow.LayoutParams view_lifepoints_params = new TableRow.LayoutParams();
view_lifepoints_params.setMargins(20, 0, 20, 20);
view_lifepoints.setText("40");
view_lifepoints.setTextSize(40);
view_lifepoints.setGravity(Gravity.CENTER);
view_lifepoints.setId(playernumber * 100 + 10);
view_lifepoints.setLayoutParams(view_lifepoints_params);
row_lifepoints.addView(view_lifepoints);
tableLayout.addView(row_lifepoints);
Log.d("Test", "Player count:" + player_count);
for(int opponent_loop = 1; opponent_loop <= player_count; opponent_loop++){
tableLayout.addView(commander_damage_from_player(player_loop, opponent_loop));
}
return tableLayout;
}
private TableRow commander_damage_from_player(int player_loop, int opponent_loop){
TableRow row = new TableRow(getContext());
TextView textView = new TextView(getContext());
TableRow.LayoutParams layoutParams = new TableRow.LayoutParams();
layoutParams.setMargins(20, 0, 40, 20);
textView.setLayoutParams(layoutParams);
textView.setText("0 | " + getResources().getString(R.string.dummy_player_name)+ " " + opponent_loop);
textView.setTextSize(20);
textView.setId(player_loop + 100 + opponent_loop);
row.addView(textView);
return row;
}
private void setPlayer_count(int player_count){
this.player_count = player_count;
}
}
And for documentation the calling class:
public class Home extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
PlayerView playerView = new PlayerView(this, 8);
setContentView(playerView.create_scrollView());
}
}



add a comment |
I'm creating a ScrollView, nesting in a RelativeLayout (which contains tables). Everything is properly added to the layout. The problem is: vertically scrolling works fine, but horizontal scrolling does NOT work at all. I already tested various combinations of MATCH_PARENT
, WRAP_CONTENT
and FILL_PARENT
. No try even worked close. So my question is: What am I missing here?
Here is the code:
public class PlayerView extends View {
private RelativeLayout relativeLayout;
private TableLayout tableLayout;
private int player_loop;
private int player_count;
public PlayerView(Context context, int player_count){
super(context);
setPlayer_count(player_count);
}
public ScrollView create_scrollView(){
ScrollView scrollView = new ScrollView(getContext());
ScrollView.LayoutParams scroll_params = new ScrollView.LayoutParams(
ScrollView.LayoutParams.MATCH_PARENT,
ScrollView.LayoutParams.MATCH_PARENT
);
scrollView.setLayoutParams(scroll_params);
scrollView.addView(create_relativeLayout());
return scrollView;
}
public RelativeLayout create_relativeLayout(){
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
return relativeLayout;
}
public void create_Player_Table_Layout(){
for(player_loop = 1; player_loop <= player_count; player_loop++){
relativeLayout.addView(player_table(getResources().getString(R.string.dummy_player_name) + player_loop, player_loop));
}
}
public TableLayout player_table(String playername, int playernumber){
tableLayout = new TableLayout(getContext());
tableLayout.setId(playernumber * 1000);
if (playernumber > 1) {
//TABLE PLACEMENT
RelativeLayout.LayoutParams tbl_params_New = new RelativeLayout.LayoutParams(RelativeLayout.LayoutParams.WRAP_CONTENT, RelativeLayout.LayoutParams.WRAP_CONTENT);
tbl_params_New.addRule(RelativeLayout.RIGHT_OF, (playernumber - 1) * 1000);
tableLayout.setLayoutParams(tbl_params_New);
}
//Add Playername
TableRow row_playername = new TableRow(getContext());
TextView view_name = new TextView(getContext());
TableRow.LayoutParams view_name_params = new TableRow.LayoutParams();
view_name_params.setMargins(20,20,20,20);
view_name.setLayoutParams(view_name_params);
view_name.setGravity(Gravity.CENTER);
view_name.setText(playername);
view_name.setTextSize(20);
view_name.setId(playernumber * 100);
row_playername.addView(view_name);
tableLayout.addView(row_playername);
//Add Lifepoints
TableRow row_lifepoints = new TableRow(getContext());
TextView view_lifepoints = new TextView(getContext());
TableRow.LayoutParams view_lifepoints_params = new TableRow.LayoutParams();
view_lifepoints_params.setMargins(20, 0, 20, 20);
view_lifepoints.setText("40");
view_lifepoints.setTextSize(40);
view_lifepoints.setGravity(Gravity.CENTER);
view_lifepoints.setId(playernumber * 100 + 10);
view_lifepoints.setLayoutParams(view_lifepoints_params);
row_lifepoints.addView(view_lifepoints);
tableLayout.addView(row_lifepoints);
Log.d("Test", "Player count:" + player_count);
for(int opponent_loop = 1; opponent_loop <= player_count; opponent_loop++){
tableLayout.addView(commander_damage_from_player(player_loop, opponent_loop));
}
return tableLayout;
}
private TableRow commander_damage_from_player(int player_loop, int opponent_loop){
TableRow row = new TableRow(getContext());
TextView textView = new TextView(getContext());
TableRow.LayoutParams layoutParams = new TableRow.LayoutParams();
layoutParams.setMargins(20, 0, 40, 20);
textView.setLayoutParams(layoutParams);
textView.setText("0 | " + getResources().getString(R.string.dummy_player_name)+ " " + opponent_loop);
textView.setTextSize(20);
textView.setId(player_loop + 100 + opponent_loop);
row.addView(textView);
return row;
}
private void setPlayer_count(int player_count){
this.player_count = player_count;
}
}
And for documentation the calling class:
public class Home extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
PlayerView playerView = new PlayerView(this, 8);
setContentView(playerView.create_scrollView());
}
}



"What am I missing here?" – Nothing.ScrollView
only scrolls vertically.
– Mike M.
Nov 24 '18 at 10:11
It sounds like aRecyclerView
may be better suited to your needs since you can scroll in both directions.
– Paul
Nov 24 '18 at 10:12
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58
add a comment |
I'm creating a ScrollView, nesting in a RelativeLayout (which contains tables). Everything is properly added to the layout. The problem is: vertically scrolling works fine, but horizontal scrolling does NOT work at all. I already tested various combinations of MATCH_PARENT
, WRAP_CONTENT
and FILL_PARENT
. No try even worked close. So my question is: What am I missing here?
Here is the code:
public class PlayerView extends View {
private RelativeLayout relativeLayout;
private TableLayout tableLayout;
private int player_loop;
private int player_count;
public PlayerView(Context context, int player_count){
super(context);
setPlayer_count(player_count);
}
public ScrollView create_scrollView(){
ScrollView scrollView = new ScrollView(getContext());
ScrollView.LayoutParams scroll_params = new ScrollView.LayoutParams(
ScrollView.LayoutParams.MATCH_PARENT,
ScrollView.LayoutParams.MATCH_PARENT
);
scrollView.setLayoutParams(scroll_params);
scrollView.addView(create_relativeLayout());
return scrollView;
}
public RelativeLayout create_relativeLayout(){
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
return relativeLayout;
}
public void create_Player_Table_Layout(){
for(player_loop = 1; player_loop <= player_count; player_loop++){
relativeLayout.addView(player_table(getResources().getString(R.string.dummy_player_name) + player_loop, player_loop));
}
}
public TableLayout player_table(String playername, int playernumber){
tableLayout = new TableLayout(getContext());
tableLayout.setId(playernumber * 1000);
if (playernumber > 1) {
//TABLE PLACEMENT
RelativeLayout.LayoutParams tbl_params_New = new RelativeLayout.LayoutParams(RelativeLayout.LayoutParams.WRAP_CONTENT, RelativeLayout.LayoutParams.WRAP_CONTENT);
tbl_params_New.addRule(RelativeLayout.RIGHT_OF, (playernumber - 1) * 1000);
tableLayout.setLayoutParams(tbl_params_New);
}
//Add Playername
TableRow row_playername = new TableRow(getContext());
TextView view_name = new TextView(getContext());
TableRow.LayoutParams view_name_params = new TableRow.LayoutParams();
view_name_params.setMargins(20,20,20,20);
view_name.setLayoutParams(view_name_params);
view_name.setGravity(Gravity.CENTER);
view_name.setText(playername);
view_name.setTextSize(20);
view_name.setId(playernumber * 100);
row_playername.addView(view_name);
tableLayout.addView(row_playername);
//Add Lifepoints
TableRow row_lifepoints = new TableRow(getContext());
TextView view_lifepoints = new TextView(getContext());
TableRow.LayoutParams view_lifepoints_params = new TableRow.LayoutParams();
view_lifepoints_params.setMargins(20, 0, 20, 20);
view_lifepoints.setText("40");
view_lifepoints.setTextSize(40);
view_lifepoints.setGravity(Gravity.CENTER);
view_lifepoints.setId(playernumber * 100 + 10);
view_lifepoints.setLayoutParams(view_lifepoints_params);
row_lifepoints.addView(view_lifepoints);
tableLayout.addView(row_lifepoints);
Log.d("Test", "Player count:" + player_count);
for(int opponent_loop = 1; opponent_loop <= player_count; opponent_loop++){
tableLayout.addView(commander_damage_from_player(player_loop, opponent_loop));
}
return tableLayout;
}
private TableRow commander_damage_from_player(int player_loop, int opponent_loop){
TableRow row = new TableRow(getContext());
TextView textView = new TextView(getContext());
TableRow.LayoutParams layoutParams = new TableRow.LayoutParams();
layoutParams.setMargins(20, 0, 40, 20);
textView.setLayoutParams(layoutParams);
textView.setText("0 | " + getResources().getString(R.string.dummy_player_name)+ " " + opponent_loop);
textView.setTextSize(20);
textView.setId(player_loop + 100 + opponent_loop);
row.addView(textView);
return row;
}
private void setPlayer_count(int player_count){
this.player_count = player_count;
}
}
And for documentation the calling class:
public class Home extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
PlayerView playerView = new PlayerView(this, 8);
setContentView(playerView.create_scrollView());
}
}



I'm creating a ScrollView, nesting in a RelativeLayout (which contains tables). Everything is properly added to the layout. The problem is: vertically scrolling works fine, but horizontal scrolling does NOT work at all. I already tested various combinations of MATCH_PARENT
, WRAP_CONTENT
and FILL_PARENT
. No try even worked close. So my question is: What am I missing here?
Here is the code:
public class PlayerView extends View {
private RelativeLayout relativeLayout;
private TableLayout tableLayout;
private int player_loop;
private int player_count;
public PlayerView(Context context, int player_count){
super(context);
setPlayer_count(player_count);
}
public ScrollView create_scrollView(){
ScrollView scrollView = new ScrollView(getContext());
ScrollView.LayoutParams scroll_params = new ScrollView.LayoutParams(
ScrollView.LayoutParams.MATCH_PARENT,
ScrollView.LayoutParams.MATCH_PARENT
);
scrollView.setLayoutParams(scroll_params);
scrollView.addView(create_relativeLayout());
return scrollView;
}
public RelativeLayout create_relativeLayout(){
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
return relativeLayout;
}
public void create_Player_Table_Layout(){
for(player_loop = 1; player_loop <= player_count; player_loop++){
relativeLayout.addView(player_table(getResources().getString(R.string.dummy_player_name) + player_loop, player_loop));
}
}
public TableLayout player_table(String playername, int playernumber){
tableLayout = new TableLayout(getContext());
tableLayout.setId(playernumber * 1000);
if (playernumber > 1) {
//TABLE PLACEMENT
RelativeLayout.LayoutParams tbl_params_New = new RelativeLayout.LayoutParams(RelativeLayout.LayoutParams.WRAP_CONTENT, RelativeLayout.LayoutParams.WRAP_CONTENT);
tbl_params_New.addRule(RelativeLayout.RIGHT_OF, (playernumber - 1) * 1000);
tableLayout.setLayoutParams(tbl_params_New);
}
//Add Playername
TableRow row_playername = new TableRow(getContext());
TextView view_name = new TextView(getContext());
TableRow.LayoutParams view_name_params = new TableRow.LayoutParams();
view_name_params.setMargins(20,20,20,20);
view_name.setLayoutParams(view_name_params);
view_name.setGravity(Gravity.CENTER);
view_name.setText(playername);
view_name.setTextSize(20);
view_name.setId(playernumber * 100);
row_playername.addView(view_name);
tableLayout.addView(row_playername);
//Add Lifepoints
TableRow row_lifepoints = new TableRow(getContext());
TextView view_lifepoints = new TextView(getContext());
TableRow.LayoutParams view_lifepoints_params = new TableRow.LayoutParams();
view_lifepoints_params.setMargins(20, 0, 20, 20);
view_lifepoints.setText("40");
view_lifepoints.setTextSize(40);
view_lifepoints.setGravity(Gravity.CENTER);
view_lifepoints.setId(playernumber * 100 + 10);
view_lifepoints.setLayoutParams(view_lifepoints_params);
row_lifepoints.addView(view_lifepoints);
tableLayout.addView(row_lifepoints);
Log.d("Test", "Player count:" + player_count);
for(int opponent_loop = 1; opponent_loop <= player_count; opponent_loop++){
tableLayout.addView(commander_damage_from_player(player_loop, opponent_loop));
}
return tableLayout;
}
private TableRow commander_damage_from_player(int player_loop, int opponent_loop){
TableRow row = new TableRow(getContext());
TextView textView = new TextView(getContext());
TableRow.LayoutParams layoutParams = new TableRow.LayoutParams();
layoutParams.setMargins(20, 0, 40, 20);
textView.setLayoutParams(layoutParams);
textView.setText("0 | " + getResources().getString(R.string.dummy_player_name)+ " " + opponent_loop);
textView.setTextSize(20);
textView.setId(player_loop + 100 + opponent_loop);
row.addView(textView);
return row;
}
private void setPlayer_count(int player_count){
this.player_count = player_count;
}
}
And for documentation the calling class:
public class Home extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
PlayerView playerView = new PlayerView(this, 8);
setContentView(playerView.create_scrollView());
}
}






asked Nov 24 '18 at 10:09
prextorprextor
12519
12519
"What am I missing here?" – Nothing.ScrollView
only scrolls vertically.
– Mike M.
Nov 24 '18 at 10:11
It sounds like aRecyclerView
may be better suited to your needs since you can scroll in both directions.
– Paul
Nov 24 '18 at 10:12
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58
add a comment |
"What am I missing here?" – Nothing.ScrollView
only scrolls vertically.
– Mike M.
Nov 24 '18 at 10:11
It sounds like aRecyclerView
may be better suited to your needs since you can scroll in both directions.
– Paul
Nov 24 '18 at 10:12
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58
"What am I missing here?" – Nothing.
ScrollView
only scrolls vertically.– Mike M.
Nov 24 '18 at 10:11
"What am I missing here?" – Nothing.
ScrollView
only scrolls vertically.– Mike M.
Nov 24 '18 at 10:11
It sounds like a
RecyclerView
may be better suited to your needs since you can scroll in both directions.– Paul
Nov 24 '18 at 10:12
It sounds like a
RecyclerView
may be better suited to your needs since you can scroll in both directions.– Paul
Nov 24 '18 at 10:12
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58
add a comment |
1 Answer
1
active
oldest
votes
The only way you can do this is by creating a horizontalscrollview to be the child of the scrollview. If you make the minor modification to your create_relativeLayout() to create a horizontalscroll view then it will work correctly. I have seen this happen in other examples.
public HorizontalScrollView create_relativeLayout(){
HorizontalScrollView horizontalScrollView = new HorizontalScrollView(getContext());
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
horizontalScrollView.addView(relativeLayout);
return horizontalScrollView;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53457106%2fscroll-horizontally-in-programmatically-created-scrollview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The only way you can do this is by creating a horizontalscrollview to be the child of the scrollview. If you make the minor modification to your create_relativeLayout() to create a horizontalscroll view then it will work correctly. I have seen this happen in other examples.
public HorizontalScrollView create_relativeLayout(){
HorizontalScrollView horizontalScrollView = new HorizontalScrollView(getContext());
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
horizontalScrollView.addView(relativeLayout);
return horizontalScrollView;
}
add a comment |
The only way you can do this is by creating a horizontalscrollview to be the child of the scrollview. If you make the minor modification to your create_relativeLayout() to create a horizontalscroll view then it will work correctly. I have seen this happen in other examples.
public HorizontalScrollView create_relativeLayout(){
HorizontalScrollView horizontalScrollView = new HorizontalScrollView(getContext());
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
horizontalScrollView.addView(relativeLayout);
return horizontalScrollView;
}
add a comment |
The only way you can do this is by creating a horizontalscrollview to be the child of the scrollview. If you make the minor modification to your create_relativeLayout() to create a horizontalscroll view then it will work correctly. I have seen this happen in other examples.
public HorizontalScrollView create_relativeLayout(){
HorizontalScrollView horizontalScrollView = new HorizontalScrollView(getContext());
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
horizontalScrollView.addView(relativeLayout);
return horizontalScrollView;
}
The only way you can do this is by creating a horizontalscrollview to be the child of the scrollview. If you make the minor modification to your create_relativeLayout() to create a horizontalscroll view then it will work correctly. I have seen this happen in other examples.
public HorizontalScrollView create_relativeLayout(){
HorizontalScrollView horizontalScrollView = new HorizontalScrollView(getContext());
relativeLayout = new RelativeLayout(getContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.MATCH_PARENT,
RelativeLayout.LayoutParams.MATCH_PARENT
);
relativeLayout.setLayoutParams(relativeParams);
create_Player_Table_Layout();
horizontalScrollView.addView(relativeLayout);
return horizontalScrollView;
}
answered Nov 24 '18 at 10:23
RajuRaju
245
245
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53457106%2fscroll-horizontally-in-programmatically-created-scrollview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RncNy 0 YoUH4oqY8ad5DihE,E22a,GHKgLz4tQ,b8
"What am I missing here?" – Nothing.
ScrollView
only scrolls vertically.– Mike M.
Nov 24 '18 at 10:11
It sounds like a
RecyclerView
may be better suited to your needs since you can scroll in both directions.– Paul
Nov 24 '18 at 10:12
@Paul: Thanks for the hint! I'll consider it further. For a quick solution I'll go with the nesting an additional horizontal scrollview.
– prextor
Nov 24 '18 at 11:58