Error: Malformed heap size when trying to execute HTTP-Request on local tomcat
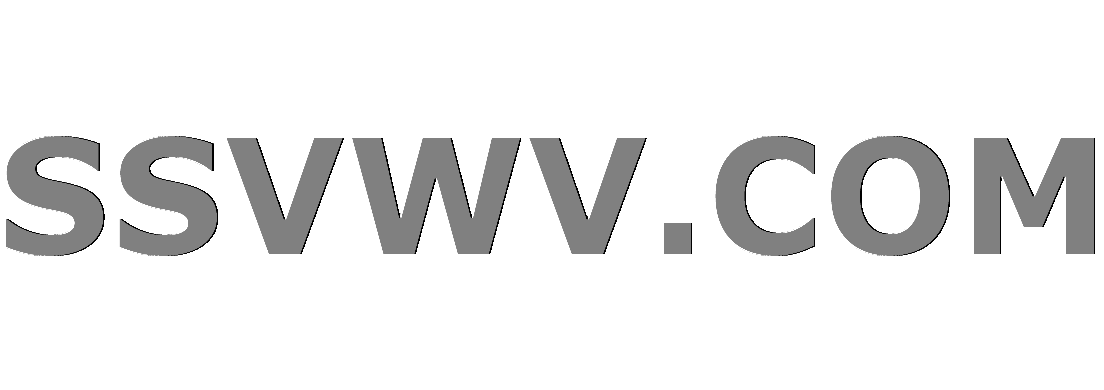
Multi tool use
I am trying to set up a Spring-Boot application that can be accessed via REST. For starting purposes, I wanted it to simply take a number and add the number 2
to it and return the result.
Now I built a WAR
-File and deployed it on my tomcat, so that I can try to access it from an Angular
project. First off, I'd like to test it with cURL
to ensure that it's working.
What did I do?
- Build
WAR
-file - Move
WAR
-file into myxampp/tomcat/webapps/
- Start my tomcat from
xampp
(running on ports 8080, 8005, 8009) - Open
cmd
and move into mycURL
-directory - Execute the following command:
curl.exe -H "number: 5" http://localhost:8009/number/
Error:
Throwing unexpected: Error: Malformed heap size 'number: 5'.
I got the following setup:
src/com/example/demo
|
---DemoApplication.java
|
---/controller
| |---NumberController.java
---/dto
| |---EntryDto.java
---/service
|---EntryService.java
NumberController.java
@RequestMapping("/number")
@RestController
public class NumberController {
@Autowired
EntryService entryService;
@RequestMapping(value="/number/{number}")
public EntryDto receiveNumber(int number) {
return entryService.createEntryDtoFromNumber(number);
}
}
EntryDto.java
public class EntryDto {
private int value;
public EntryDto(int value) {
this.value = value;
}
public void increaseValue(int increaseValue) {
this.value = this.value + increaseValue;
}
public int getValue() {
return this.value;
}
public void setValue(int value) {
this.value = value;
}
}
EntryService.java
@Service
public class EntryService {
public EntryDto createEntryDtoFromNumber(int number) {
entryDto = new EntryDto(number);
entryDto.increaseValue(2);
return this.entryDto;
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.restdocs</groupId>
<artifactId>spring-restdocs-mockmvc</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
java rest tomcat
add a comment |
I am trying to set up a Spring-Boot application that can be accessed via REST. For starting purposes, I wanted it to simply take a number and add the number 2
to it and return the result.
Now I built a WAR
-File and deployed it on my tomcat, so that I can try to access it from an Angular
project. First off, I'd like to test it with cURL
to ensure that it's working.
What did I do?
- Build
WAR
-file - Move
WAR
-file into myxampp/tomcat/webapps/
- Start my tomcat from
xampp
(running on ports 8080, 8005, 8009) - Open
cmd
and move into mycURL
-directory - Execute the following command:
curl.exe -H "number: 5" http://localhost:8009/number/
Error:
Throwing unexpected: Error: Malformed heap size 'number: 5'.
I got the following setup:
src/com/example/demo
|
---DemoApplication.java
|
---/controller
| |---NumberController.java
---/dto
| |---EntryDto.java
---/service
|---EntryService.java
NumberController.java
@RequestMapping("/number")
@RestController
public class NumberController {
@Autowired
EntryService entryService;
@RequestMapping(value="/number/{number}")
public EntryDto receiveNumber(int number) {
return entryService.createEntryDtoFromNumber(number);
}
}
EntryDto.java
public class EntryDto {
private int value;
public EntryDto(int value) {
this.value = value;
}
public void increaseValue(int increaseValue) {
this.value = this.value + increaseValue;
}
public int getValue() {
return this.value;
}
public void setValue(int value) {
this.value = value;
}
}
EntryService.java
@Service
public class EntryService {
public EntryDto createEntryDtoFromNumber(int number) {
entryDto = new EntryDto(number);
entryDto.increaseValue(2);
return this.entryDto;
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.restdocs</groupId>
<artifactId>spring-restdocs-mockmvc</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
java rest tomcat
add a comment |
I am trying to set up a Spring-Boot application that can be accessed via REST. For starting purposes, I wanted it to simply take a number and add the number 2
to it and return the result.
Now I built a WAR
-File and deployed it on my tomcat, so that I can try to access it from an Angular
project. First off, I'd like to test it with cURL
to ensure that it's working.
What did I do?
- Build
WAR
-file - Move
WAR
-file into myxampp/tomcat/webapps/
- Start my tomcat from
xampp
(running on ports 8080, 8005, 8009) - Open
cmd
and move into mycURL
-directory - Execute the following command:
curl.exe -H "number: 5" http://localhost:8009/number/
Error:
Throwing unexpected: Error: Malformed heap size 'number: 5'.
I got the following setup:
src/com/example/demo
|
---DemoApplication.java
|
---/controller
| |---NumberController.java
---/dto
| |---EntryDto.java
---/service
|---EntryService.java
NumberController.java
@RequestMapping("/number")
@RestController
public class NumberController {
@Autowired
EntryService entryService;
@RequestMapping(value="/number/{number}")
public EntryDto receiveNumber(int number) {
return entryService.createEntryDtoFromNumber(number);
}
}
EntryDto.java
public class EntryDto {
private int value;
public EntryDto(int value) {
this.value = value;
}
public void increaseValue(int increaseValue) {
this.value = this.value + increaseValue;
}
public int getValue() {
return this.value;
}
public void setValue(int value) {
this.value = value;
}
}
EntryService.java
@Service
public class EntryService {
public EntryDto createEntryDtoFromNumber(int number) {
entryDto = new EntryDto(number);
entryDto.increaseValue(2);
return this.entryDto;
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.restdocs</groupId>
<artifactId>spring-restdocs-mockmvc</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
java rest tomcat
I am trying to set up a Spring-Boot application that can be accessed via REST. For starting purposes, I wanted it to simply take a number and add the number 2
to it and return the result.
Now I built a WAR
-File and deployed it on my tomcat, so that I can try to access it from an Angular
project. First off, I'd like to test it with cURL
to ensure that it's working.
What did I do?
- Build
WAR
-file - Move
WAR
-file into myxampp/tomcat/webapps/
- Start my tomcat from
xampp
(running on ports 8080, 8005, 8009) - Open
cmd
and move into mycURL
-directory - Execute the following command:
curl.exe -H "number: 5" http://localhost:8009/number/
Error:
Throwing unexpected: Error: Malformed heap size 'number: 5'.
I got the following setup:
src/com/example/demo
|
---DemoApplication.java
|
---/controller
| |---NumberController.java
---/dto
| |---EntryDto.java
---/service
|---EntryService.java
NumberController.java
@RequestMapping("/number")
@RestController
public class NumberController {
@Autowired
EntryService entryService;
@RequestMapping(value="/number/{number}")
public EntryDto receiveNumber(int number) {
return entryService.createEntryDtoFromNumber(number);
}
}
EntryDto.java
public class EntryDto {
private int value;
public EntryDto(int value) {
this.value = value;
}
public void increaseValue(int increaseValue) {
this.value = this.value + increaseValue;
}
public int getValue() {
return this.value;
}
public void setValue(int value) {
this.value = value;
}
}
EntryService.java
@Service
public class EntryService {
public EntryDto createEntryDtoFromNumber(int number) {
entryDto = new EntryDto(number);
entryDto.increaseValue(2);
return this.entryDto;
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-rest</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.restdocs</groupId>
<artifactId>spring-restdocs-mockmvc</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
java rest tomcat
java rest tomcat
asked Nov 10 at 17:02
Rüdiger
256219
256219
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241295%2ferror-malformed-heap-size-when-trying-to-execute-http-request-on-local-tomcat%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241295%2ferror-malformed-heap-size-when-trying-to-execute-http-request-on-local-tomcat%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GD39mYe4ahR,F5ejF,ZmAAaDqJyrA5Vh1apw 1QXNoPTg,K6SpgTHRZo2 l8xTdZa8MBlPEYpiuAarfqN