Which is really immutable in redux data or state or both?
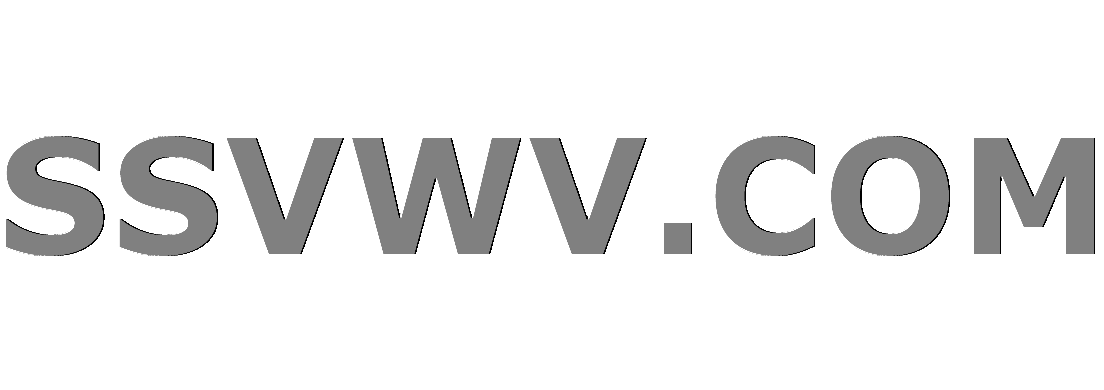
Multi tool use
I always read that state in redux is and should be immutable, while logging store.getState() will return the state which may represent a modified data after dispatching a certain action.
This part is confusing to me, how we can consider state is immutable in this case, do state here refer to the original data or the initial data that passed as the first argument to the reducer??
const reducer = (state = , action) => {
}
Is the immutable state here the initial state which is an empty array or the data that dispatched after fetching???
I need some clarification about this confusing part???
reactjs redux
add a comment |
I always read that state in redux is and should be immutable, while logging store.getState() will return the state which may represent a modified data after dispatching a certain action.
This part is confusing to me, how we can consider state is immutable in this case, do state here refer to the original data or the initial data that passed as the first argument to the reducer??
const reducer = (state = , action) => {
}
Is the immutable state here the initial state which is an empty array or the data that dispatched after fetching???
I need some clarification about this confusing part???
reactjs redux
add a comment |
I always read that state in redux is and should be immutable, while logging store.getState() will return the state which may represent a modified data after dispatching a certain action.
This part is confusing to me, how we can consider state is immutable in this case, do state here refer to the original data or the initial data that passed as the first argument to the reducer??
const reducer = (state = , action) => {
}
Is the immutable state here the initial state which is an empty array or the data that dispatched after fetching???
I need some clarification about this confusing part???
reactjs redux
I always read that state in redux is and should be immutable, while logging store.getState() will return the state which may represent a modified data after dispatching a certain action.
This part is confusing to me, how we can consider state is immutable in this case, do state here refer to the original data or the initial data that passed as the first argument to the reducer??
const reducer = (state = , action) => {
}
Is the immutable state here the initial state which is an empty array or the data that dispatched after fetching???
I need some clarification about this confusing part???
reactjs redux
reactjs redux
edited Nov 10 at 18:17
asked Nov 10 at 18:11
Saher Elgendy
496
496
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The redux state itself is not immutable but it is composed from immutable data structures. Saying that state is immutable is not correct.
The whole point of a data structure being immutable is that you never mutate it. When speaking about arrays, mutating means adding, removing or setting values (e.g. push
, shift
, splice
etc). Therefore, to change a value, you always have to create a new array (instead of mutating the existing array).
For example:
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
return [...state, addedValue];
}
not
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
state.push(addedValue);
return state;
}
In this sense both the previous state and the new state (the returned value) are both immutable data structures.
To explain the concept of timewalking mentioned in comments, first we suppose there is some redux state in some variable:
let state = /* redux state */
Whenever an action is triggered, all reducers run recursively to create a new state. The whole concept in Redux is expressed by something like this:
let oldState = state;
state = reducers(oldState, action);
The concept of timewalking means that we do something like this:
const stateHistory = ;
stateHistory.push(state)
state = reducers(oldState, action);
This way our stateHistory
variable contains the complete previous state. To timewalk, we can simply:
state = stateHistory[index];
and rerender.
However, this wouldn't work without immutable data structures. Immutable data structures ensure that all states in history are independent and mutating one won't affect the rest.
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better thannull
orundefined
because your app won't crash when you try to read itslength
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can benull
). The reason to use an empty array is specific to a given use case.
– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (seePureComponent
).
– Sulthan
Nov 10 at 22:19
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
|
show 5 more comments
I always read that state in redux is and should be immutable, while
logging store.getState() will return the state which may represent a
modified data after dispatching a certain action.
This is true.
This part is confusing to me, how we can consider state is immutable
in this case, do state here refer to the original data or the initial
data that passed as the first argument to the reducer??
State as an argument of the reducer refers to the actual state. If it's the first time calling the reducer, then state will be initialized with the default value, in this case . This feature is called Default parameters.
Is the immutable state here the initial state which is an empty array
or the data that dispatched after fetching???
Redux requires to write reducers as pure functions so that the state modifications are handled exclusively by Redux.
For more information please refer to Reducer's documentation.
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241963%2fwhich-is-really-immutable-in-redux-data-or-state-or-both%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The redux state itself is not immutable but it is composed from immutable data structures. Saying that state is immutable is not correct.
The whole point of a data structure being immutable is that you never mutate it. When speaking about arrays, mutating means adding, removing or setting values (e.g. push
, shift
, splice
etc). Therefore, to change a value, you always have to create a new array (instead of mutating the existing array).
For example:
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
return [...state, addedValue];
}
not
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
state.push(addedValue);
return state;
}
In this sense both the previous state and the new state (the returned value) are both immutable data structures.
To explain the concept of timewalking mentioned in comments, first we suppose there is some redux state in some variable:
let state = /* redux state */
Whenever an action is triggered, all reducers run recursively to create a new state. The whole concept in Redux is expressed by something like this:
let oldState = state;
state = reducers(oldState, action);
The concept of timewalking means that we do something like this:
const stateHistory = ;
stateHistory.push(state)
state = reducers(oldState, action);
This way our stateHistory
variable contains the complete previous state. To timewalk, we can simply:
state = stateHistory[index];
and rerender.
However, this wouldn't work without immutable data structures. Immutable data structures ensure that all states in history are independent and mutating one won't affect the rest.
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better thannull
orundefined
because your app won't crash when you try to read itslength
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can benull
). The reason to use an empty array is specific to a given use case.
– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (seePureComponent
).
– Sulthan
Nov 10 at 22:19
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
|
show 5 more comments
The redux state itself is not immutable but it is composed from immutable data structures. Saying that state is immutable is not correct.
The whole point of a data structure being immutable is that you never mutate it. When speaking about arrays, mutating means adding, removing or setting values (e.g. push
, shift
, splice
etc). Therefore, to change a value, you always have to create a new array (instead of mutating the existing array).
For example:
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
return [...state, addedValue];
}
not
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
state.push(addedValue);
return state;
}
In this sense both the previous state and the new state (the returned value) are both immutable data structures.
To explain the concept of timewalking mentioned in comments, first we suppose there is some redux state in some variable:
let state = /* redux state */
Whenever an action is triggered, all reducers run recursively to create a new state. The whole concept in Redux is expressed by something like this:
let oldState = state;
state = reducers(oldState, action);
The concept of timewalking means that we do something like this:
const stateHistory = ;
stateHistory.push(state)
state = reducers(oldState, action);
This way our stateHistory
variable contains the complete previous state. To timewalk, we can simply:
state = stateHistory[index];
and rerender.
However, this wouldn't work without immutable data structures. Immutable data structures ensure that all states in history are independent and mutating one won't affect the rest.
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better thannull
orundefined
because your app won't crash when you try to read itslength
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can benull
). The reason to use an empty array is specific to a given use case.
– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (seePureComponent
).
– Sulthan
Nov 10 at 22:19
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
|
show 5 more comments
The redux state itself is not immutable but it is composed from immutable data structures. Saying that state is immutable is not correct.
The whole point of a data structure being immutable is that you never mutate it. When speaking about arrays, mutating means adding, removing or setting values (e.g. push
, shift
, splice
etc). Therefore, to change a value, you always have to create a new array (instead of mutating the existing array).
For example:
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
return [...state, addedValue];
}
not
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
state.push(addedValue);
return state;
}
In this sense both the previous state and the new state (the returned value) are both immutable data structures.
To explain the concept of timewalking mentioned in comments, first we suppose there is some redux state in some variable:
let state = /* redux state */
Whenever an action is triggered, all reducers run recursively to create a new state. The whole concept in Redux is expressed by something like this:
let oldState = state;
state = reducers(oldState, action);
The concept of timewalking means that we do something like this:
const stateHistory = ;
stateHistory.push(state)
state = reducers(oldState, action);
This way our stateHistory
variable contains the complete previous state. To timewalk, we can simply:
state = stateHistory[index];
and rerender.
However, this wouldn't work without immutable data structures. Immutable data structures ensure that all states in history are independent and mutating one won't affect the rest.
The redux state itself is not immutable but it is composed from immutable data structures. Saying that state is immutable is not correct.
The whole point of a data structure being immutable is that you never mutate it. When speaking about arrays, mutating means adding, removing or setting values (e.g. push
, shift
, splice
etc). Therefore, to change a value, you always have to create a new array (instead of mutating the existing array).
For example:
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
return [...state, addedValue];
}
not
const reducer = (state = , action) => {
const addedValue = action.payload.addedValue;
state.push(addedValue);
return state;
}
In this sense both the previous state and the new state (the returned value) are both immutable data structures.
To explain the concept of timewalking mentioned in comments, first we suppose there is some redux state in some variable:
let state = /* redux state */
Whenever an action is triggered, all reducers run recursively to create a new state. The whole concept in Redux is expressed by something like this:
let oldState = state;
state = reducers(oldState, action);
The concept of timewalking means that we do something like this:
const stateHistory = ;
stateHistory.push(state)
state = reducers(oldState, action);
This way our stateHistory
variable contains the complete previous state. To timewalk, we can simply:
state = stateHistory[index];
and rerender.
However, this wouldn't work without immutable data structures. Immutable data structures ensure that all states in history are independent and mutating one won't affect the rest.
edited Nov 11 at 8:50
answered Nov 10 at 21:56


Sulthan
93.6k16151196
93.6k16151196
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better thannull
orundefined
because your app won't crash when you try to read itslength
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can benull
). The reason to use an empty array is specific to a given use case.
– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (seePureComponent
).
– Sulthan
Nov 10 at 22:19
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
|
show 5 more comments
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better thannull
orundefined
because your app won't crash when you try to read itslength
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can benull
). The reason to use an empty array is specific to a given use case.
– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (seePureComponent
).
– Sulthan
Nov 10 at 22:19
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
Thanks , i understand all of that, but if the initial state is an empty array so our non-mutated original state would be an empty array, what is the point then to store an empty array??
– Saher Elgendy
Nov 10 at 22:02
@SaherElgendy Empty array is better than
null
or undefined
because your app won't crash when you try to read its length
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can be null
). The reason to use an empty array is specific to a given use case.– Sulthan
Nov 10 at 22:04
@SaherElgendy Empty array is better than
null
or undefined
because your app won't crash when you try to read its length
or index it. That's the concept of prefering non-optional variables over optional variables (variables that can be null
). The reason to use an empty array is specific to a given use case.– Sulthan
Nov 10 at 22:04
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
I think I don't understand you question then. An empty array is a valid value describing the situation when there are no items?
– Sulthan
Nov 10 at 22:06
1
1
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use
===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (see PureComponent
).– Sulthan
Nov 10 at 22:19
@SaherElgendy Using only immutable structures in state (which is a bit awkward in pure JS) has some very positive implications. For example, when checking whether a part of the state has changed, you can simply use
===
(object equality). And that makes rerenders very fast because only the actually changed parts will have to rerendered (see PureComponent
).– Sulthan
Nov 10 at 22:19
1
1
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
With immutable state you can hold state history in memory. With mutable states changing the state would destroy saved history. If you dont see it, try to implement it.
– Sulthan
Nov 10 at 23:13
|
show 5 more comments
I always read that state in redux is and should be immutable, while
logging store.getState() will return the state which may represent a
modified data after dispatching a certain action.
This is true.
This part is confusing to me, how we can consider state is immutable
in this case, do state here refer to the original data or the initial
data that passed as the first argument to the reducer??
State as an argument of the reducer refers to the actual state. If it's the first time calling the reducer, then state will be initialized with the default value, in this case . This feature is called Default parameters.
Is the immutable state here the initial state which is an empty array
or the data that dispatched after fetching???
Redux requires to write reducers as pure functions so that the state modifications are handled exclusively by Redux.
For more information please refer to Reducer's documentation.
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
add a comment |
I always read that state in redux is and should be immutable, while
logging store.getState() will return the state which may represent a
modified data after dispatching a certain action.
This is true.
This part is confusing to me, how we can consider state is immutable
in this case, do state here refer to the original data or the initial
data that passed as the first argument to the reducer??
State as an argument of the reducer refers to the actual state. If it's the first time calling the reducer, then state will be initialized with the default value, in this case . This feature is called Default parameters.
Is the immutable state here the initial state which is an empty array
or the data that dispatched after fetching???
Redux requires to write reducers as pure functions so that the state modifications are handled exclusively by Redux.
For more information please refer to Reducer's documentation.
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
add a comment |
I always read that state in redux is and should be immutable, while
logging store.getState() will return the state which may represent a
modified data after dispatching a certain action.
This is true.
This part is confusing to me, how we can consider state is immutable
in this case, do state here refer to the original data or the initial
data that passed as the first argument to the reducer??
State as an argument of the reducer refers to the actual state. If it's the first time calling the reducer, then state will be initialized with the default value, in this case . This feature is called Default parameters.
Is the immutable state here the initial state which is an empty array
or the data that dispatched after fetching???
Redux requires to write reducers as pure functions so that the state modifications are handled exclusively by Redux.
For more information please refer to Reducer's documentation.
I always read that state in redux is and should be immutable, while
logging store.getState() will return the state which may represent a
modified data after dispatching a certain action.
This is true.
This part is confusing to me, how we can consider state is immutable
in this case, do state here refer to the original data or the initial
data that passed as the first argument to the reducer??
State as an argument of the reducer refers to the actual state. If it's the first time calling the reducer, then state will be initialized with the default value, in this case . This feature is called Default parameters.
Is the immutable state here the initial state which is an empty array
or the data that dispatched after fetching???
Redux requires to write reducers as pure functions so that the state modifications are handled exclusively by Redux.
For more information please refer to Reducer's documentation.
answered Nov 10 at 18:53


Joaquin Timerman
1245
1245
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
add a comment |
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
Thank you but this does not answer anything, i know that state would be what is returned from the reducer after a certain action, otherwise the default one will be returned, so our state data seems changing so from where come this fact " state in redux is immutable"
– Saher Elgendy
Nov 10 at 19:53
1
1
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
State should not be mutated by your code, it's modification is handled purely by Redux. Therefore it is said that state is immutable.
– Joaquin Timerman
Nov 10 at 20:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241963%2fwhich-is-really-immutable-in-redux-data-or-state-or-both%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Jh pn m9 kR9lltZ JJNr47HOEos WbN9LJv2OCQ