“Video select screen” via radio buttons
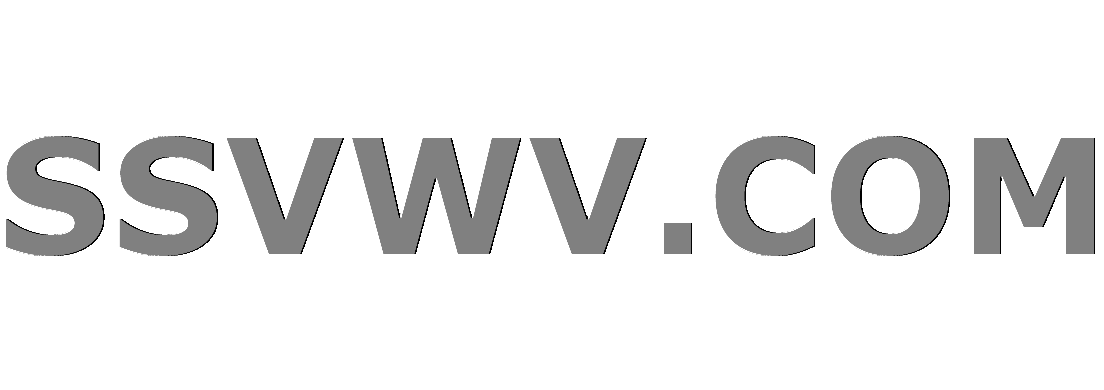
Multi tool use
i have the following problem. I try to make some kind of "video select screen" where you have the option to choose what kind of video you want to see AND choose what music genre you want to listen to while the video plays (there is one video-file for every possible choice). I tried to write a function that changes the src of the video but i just cant make it work and i dont know why.
This is my code so far:
HTML:
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Javascript:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
if (music.value == "com" && selection.value == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (music.value == "com" && selection.value == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (music.value == "mix" && selection.value == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (music.value == "mix" && selection.value == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (music.value == "vid" && selection.value == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (music.value == "vid" && selection.value == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
This is what i came up with. I hope my attempt to takle the problem is even pointing in the right direction. (im new to javascript and html and im teaching myself how to code. please dont be too harsh)
javascript html
add a comment |
i have the following problem. I try to make some kind of "video select screen" where you have the option to choose what kind of video you want to see AND choose what music genre you want to listen to while the video plays (there is one video-file for every possible choice). I tried to write a function that changes the src of the video but i just cant make it work and i dont know why.
This is my code so far:
HTML:
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Javascript:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
if (music.value == "com" && selection.value == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (music.value == "com" && selection.value == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (music.value == "mix" && selection.value == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (music.value == "mix" && selection.value == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (music.value == "vid" && selection.value == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (music.value == "vid" && selection.value == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
This is what i came up with. I hope my attempt to takle the problem is even pointing in the right direction. (im new to javascript and html and im teaching myself how to code. please dont be too harsh)
javascript html
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals:theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)
– David Thomas
Nov 10 at 18:10
add a comment |
i have the following problem. I try to make some kind of "video select screen" where you have the option to choose what kind of video you want to see AND choose what music genre you want to listen to while the video plays (there is one video-file for every possible choice). I tried to write a function that changes the src of the video but i just cant make it work and i dont know why.
This is my code so far:
HTML:
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Javascript:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
if (music.value == "com" && selection.value == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (music.value == "com" && selection.value == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (music.value == "mix" && selection.value == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (music.value == "mix" && selection.value == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (music.value == "vid" && selection.value == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (music.value == "vid" && selection.value == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
This is what i came up with. I hope my attempt to takle the problem is even pointing in the right direction. (im new to javascript and html and im teaching myself how to code. please dont be too harsh)
javascript html
i have the following problem. I try to make some kind of "video select screen" where you have the option to choose what kind of video you want to see AND choose what music genre you want to listen to while the video plays (there is one video-file for every possible choice). I tried to write a function that changes the src of the video but i just cant make it work and i dont know why.
This is my code so far:
HTML:
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Javascript:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
if (music.value == "com" && selection.value == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (music.value == "com" && selection.value == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (music.value == "mix" && selection.value == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (music.value == "mix" && selection.value == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (music.value == "vid" && selection.value == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (music.value == "vid" && selection.value == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
This is what i came up with. I hope my attempt to takle the problem is even pointing in the right direction. (im new to javascript and html and im teaching myself how to code. please dont be too harsh)
javascript html
javascript html
asked Nov 10 at 18:05


Joshua
82
82
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals:theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)
– David Thomas
Nov 10 at 18:10
add a comment |
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals:theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)
– David Thomas
Nov 10 at 18:10
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:
theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals: theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)– David Thomas
Nov 10 at 18:10
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:
theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals: theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)– David Thomas
Nov 10 at 18:10
add a comment |
1 Answer
1
active
oldest
votes
That's because getElementsByName
returns a NodeList, which means that you have to iterate through the collection to access the value of the selected radio button. Therefore, you can convert the NodeList into an array using Array.prototype.slice.call(<NodeList>)
, perform filtering on it to return radio buttons that are checked, and then use it to access its value:
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
Further explanation on the code block above:
Array.prototype.slice.call(music)
converts themusic
NodeList into an array of elements- We then use
.filter()
to go through the returned array. In the callback, we ensure that we only select/filter for radio buttons that are checked, i.e..checked
property returns a truthy value. - Chain
[0]
after the.filter()
call to get the first checked radio button - Chain
.value
to get the value of the first checked radio button
Then in your if/else blocks, instead of checking for music.value
or selection.value
, you can refer to musicValue
and selectionValue
instead:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.
– Terry
Nov 11 at 9:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241916%2fvideo-select-screen-via-radio-buttons%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
That's because getElementsByName
returns a NodeList, which means that you have to iterate through the collection to access the value of the selected radio button. Therefore, you can convert the NodeList into an array using Array.prototype.slice.call(<NodeList>)
, perform filtering on it to return radio buttons that are checked, and then use it to access its value:
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
Further explanation on the code block above:
Array.prototype.slice.call(music)
converts themusic
NodeList into an array of elements- We then use
.filter()
to go through the returned array. In the callback, we ensure that we only select/filter for radio buttons that are checked, i.e..checked
property returns a truthy value. - Chain
[0]
after the.filter()
call to get the first checked radio button - Chain
.value
to get the value of the first checked radio button
Then in your if/else blocks, instead of checking for music.value
or selection.value
, you can refer to musicValue
and selectionValue
instead:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.
– Terry
Nov 11 at 9:10
add a comment |
That's because getElementsByName
returns a NodeList, which means that you have to iterate through the collection to access the value of the selected radio button. Therefore, you can convert the NodeList into an array using Array.prototype.slice.call(<NodeList>)
, perform filtering on it to return radio buttons that are checked, and then use it to access its value:
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
Further explanation on the code block above:
Array.prototype.slice.call(music)
converts themusic
NodeList into an array of elements- We then use
.filter()
to go through the returned array. In the callback, we ensure that we only select/filter for radio buttons that are checked, i.e..checked
property returns a truthy value. - Chain
[0]
after the.filter()
call to get the first checked radio button - Chain
.value
to get the value of the first checked radio button
Then in your if/else blocks, instead of checking for music.value
or selection.value
, you can refer to musicValue
and selectionValue
instead:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.
– Terry
Nov 11 at 9:10
add a comment |
That's because getElementsByName
returns a NodeList, which means that you have to iterate through the collection to access the value of the selected radio button. Therefore, you can convert the NodeList into an array using Array.prototype.slice.call(<NodeList>)
, perform filtering on it to return radio buttons that are checked, and then use it to access its value:
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
Further explanation on the code block above:
Array.prototype.slice.call(music)
converts themusic
NodeList into an array of elements- We then use
.filter()
to go through the returned array. In the callback, we ensure that we only select/filter for radio buttons that are checked, i.e..checked
property returns a truthy value. - Chain
[0]
after the.filter()
call to get the first checked radio button - Chain
.value
to get the value of the first checked radio button
Then in your if/else blocks, instead of checking for music.value
or selection.value
, you can refer to musicValue
and selectionValue
instead:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
That's because getElementsByName
returns a NodeList, which means that you have to iterate through the collection to access the value of the selected radio button. Therefore, you can convert the NodeList into an array using Array.prototype.slice.call(<NodeList>)
, perform filtering on it to return radio buttons that are checked, and then use it to access its value:
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
Further explanation on the code block above:
Array.prototype.slice.call(music)
converts themusic
NodeList into an array of elements- We then use
.filter()
to go through the returned array. In the callback, we ensure that we only select/filter for radio buttons that are checked, i.e..checked
property returns a truthy value. - Chain
[0]
after the.filter()
call to get the first checked radio button - Chain
.value
to get the value of the first checked radio button
Then in your if/else blocks, instead of checking for music.value
or selection.value
, you can refer to musicValue
and selectionValue
instead:
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
function playselected() {
var music = document.getElementsByName("musicselection");
var selection = document.getElementsByName("selection");
var theVid = document.getElementById("theVideo");
var musicValue = Array.prototype.slice.call(music).filter(function(musicRadioButton) {
return musicRadioButton.checked;
})[0].value;
var selectionValue = Array.prototype.slice.call(selection).filter(function(selectionRadioButton) {
return selectionRadiobutton.checked;
})[0].value;
if (musicValue == "com" && selectionValue == "pop") {
theVid.src = "videos/com_pop.mp4";
}
if (musicValue == "com" && selectionValue == "rock") {
theVid.src = "videos/com_rock.mp4";
}
if (musicValue == "mix" && selectionValue == "pop") {
theVid.src = "videos/mix_pop.mp4";
}
if (musicValue == "mix" && selectionValue == "rock") {
theVid.src = "videos/mix_rock.mp4";
}
if (musicValue == "vid" && selectionValue == "pop") {
theVid.src = "videos/vid_pop.mp4";
}
if (musicValue == "vid" && selectionValue == "rock") {
theVid.src = "videos/vid_rock.mp4";
}
}
<form>
<h3>Customize your own Showreel</h3>
<fieldset>
<input type="radio" id="commercials" name="selection" value="com">
<label for="commercials">Commercials</label>
<input type="radio" id="mixed" name="selection" value="mix" checked="checked">
<label for="mixed">Mixed</label>
<input type="radio" id="videoclip" name="selection" value="vid">
<label for="videoclip">Videoclips</label>
</fieldset>
<h4>Select Music</h4>
<fieldset>
<input type="radio" id="pop" name="musicselection" value="pop" checked="checked">
<label for="pop">Pop</label>
<input type="radio" id="rock" name="musicselection" value="rock">
<label for="rock">Rock</label>
</fieldset>
<video id="theVideo" controls></video>
<button type="button" id="theVideo" onclick="playselected();">play</button>
</form>
answered Nov 10 at 18:18


Terry
27.3k44167
27.3k44167
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.
– Terry
Nov 11 at 9:10
add a comment |
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.
– Terry
Nov 11 at 9:10
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
Thank you for the detailed answer. I tried to implement your answer into my code, but when i hit the "play" button its still not loading the chosen video. I really dont know why, can you point me in the right direction?
– Joshua
Nov 11 at 2:52
@Joshua Setting the video source does not automatically play the video. You need to invoke
.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.– Terry
Nov 11 at 9:10
@Joshua Setting the video source does not automatically play the video. You need to invoke
.play()
on the video element manually. Note that this runs into the territory of video auto play, which browsers have recently placed a lot of restrictions on.– Terry
Nov 11 at 9:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241916%2fvideo-select-screen-via-radio-buttons%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sXiKA10unB UXFIokq9 mQugXNcYJ,4yXOLzB3lZ6yjc,p,oCQOP,k,Qpf8Ct3z2alf,6MR4Mq9k7moKC4S7bSyPu8pF2OutQlf075kJK4O
Assuming those URLs are correct, that looks like you could - with some sanity-checking - just do:
theVid.src = 'videos/' + music.value + '_' + selection.value + '.mp4';
(or, using template literals:theVid.src = `videos/${music.value}_${selection.value}.mp4;`
)– David Thomas
Nov 10 at 18:10