Enum serialization in routes
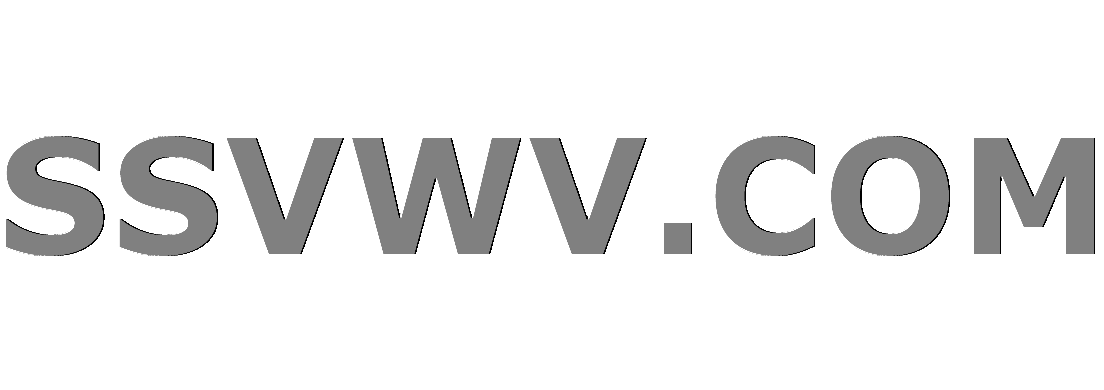
Multi tool use
We have a web API that uses snake case serialization for all the properties including enums, to enable it we used this in the startup:
services
.AddMvcCore()
.AddJsonOptions(opt =>
{
opt.SerializerSettings.DateTimeZoneHandling = Newtonsoft.Json.DateTimeZoneHandling.Local;
opt.SerializerSettings.Formatting = Newtonsoft.Json.Formatting.None;
opt.SerializerSettings.ContractResolver = new DefaultContractResolver
{
NamingStrategy = new SnakeCaseNamingStrategy { ProcessDictionaryKeys = true }
};
opt.SerializerSettings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore;
opt.SerializerSettings.Converters.Add(new StringEnumConverter());
})
.AddApiExplorer()
.AddJsonFormatters(j => j.ContractResolver = new DefaultContractResolver { NamingStrategy = new SnakeCaseNamingStrategy() { ProcessDictionaryKeys = true } })
That works great on properties, but we are having problems with routing and enums, for example we have this enum (we also tried with JsonProperty but fails in the same way):
[DataContract(Name = "document_type")]
public enum DocumentType
{
[EnumMember(Value = "passport")]
Passport,
[EnumMember(Value = "proof_of_address")]
ProofOfAddress,
}
and we are trying to search documents by type, so we have this route:
/clients/{clientId:guid/documents/{documentType}
and this in the controller:
[HttpGet]
[Route("/clients/{clientId:guid}/documents/{documentType}")]
public async Task<IActionResult> FindClientDocuments([FromRoute] Guid clientId, [FromRoute] DocumentType documentType)
with this route everything works great:
/clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/ProofOfAddress
but does not work with this:
clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/proof_of_address
In the latter case the enum is always the default value or if we add an action filter the error is "The value 'proof_of_address' is not valid."
Is there a way to make this escenario works besides trying to convert the value myself with a filter?
Thanks
c# asp.net-core json.net asp.net-core-2.1
add a comment |
We have a web API that uses snake case serialization for all the properties including enums, to enable it we used this in the startup:
services
.AddMvcCore()
.AddJsonOptions(opt =>
{
opt.SerializerSettings.DateTimeZoneHandling = Newtonsoft.Json.DateTimeZoneHandling.Local;
opt.SerializerSettings.Formatting = Newtonsoft.Json.Formatting.None;
opt.SerializerSettings.ContractResolver = new DefaultContractResolver
{
NamingStrategy = new SnakeCaseNamingStrategy { ProcessDictionaryKeys = true }
};
opt.SerializerSettings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore;
opt.SerializerSettings.Converters.Add(new StringEnumConverter());
})
.AddApiExplorer()
.AddJsonFormatters(j => j.ContractResolver = new DefaultContractResolver { NamingStrategy = new SnakeCaseNamingStrategy() { ProcessDictionaryKeys = true } })
That works great on properties, but we are having problems with routing and enums, for example we have this enum (we also tried with JsonProperty but fails in the same way):
[DataContract(Name = "document_type")]
public enum DocumentType
{
[EnumMember(Value = "passport")]
Passport,
[EnumMember(Value = "proof_of_address")]
ProofOfAddress,
}
and we are trying to search documents by type, so we have this route:
/clients/{clientId:guid/documents/{documentType}
and this in the controller:
[HttpGet]
[Route("/clients/{clientId:guid}/documents/{documentType}")]
public async Task<IActionResult> FindClientDocuments([FromRoute] Guid clientId, [FromRoute] DocumentType documentType)
with this route everything works great:
/clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/ProofOfAddress
but does not work with this:
clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/proof_of_address
In the latter case the enum is always the default value or if we add an action filter the error is "The value 'proof_of_address' is not valid."
Is there a way to make this escenario works besides trying to convert the value myself with a filter?
Thanks
c# asp.net-core json.net asp.net-core-2.1
add a comment |
We have a web API that uses snake case serialization for all the properties including enums, to enable it we used this in the startup:
services
.AddMvcCore()
.AddJsonOptions(opt =>
{
opt.SerializerSettings.DateTimeZoneHandling = Newtonsoft.Json.DateTimeZoneHandling.Local;
opt.SerializerSettings.Formatting = Newtonsoft.Json.Formatting.None;
opt.SerializerSettings.ContractResolver = new DefaultContractResolver
{
NamingStrategy = new SnakeCaseNamingStrategy { ProcessDictionaryKeys = true }
};
opt.SerializerSettings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore;
opt.SerializerSettings.Converters.Add(new StringEnumConverter());
})
.AddApiExplorer()
.AddJsonFormatters(j => j.ContractResolver = new DefaultContractResolver { NamingStrategy = new SnakeCaseNamingStrategy() { ProcessDictionaryKeys = true } })
That works great on properties, but we are having problems with routing and enums, for example we have this enum (we also tried with JsonProperty but fails in the same way):
[DataContract(Name = "document_type")]
public enum DocumentType
{
[EnumMember(Value = "passport")]
Passport,
[EnumMember(Value = "proof_of_address")]
ProofOfAddress,
}
and we are trying to search documents by type, so we have this route:
/clients/{clientId:guid/documents/{documentType}
and this in the controller:
[HttpGet]
[Route("/clients/{clientId:guid}/documents/{documentType}")]
public async Task<IActionResult> FindClientDocuments([FromRoute] Guid clientId, [FromRoute] DocumentType documentType)
with this route everything works great:
/clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/ProofOfAddress
but does not work with this:
clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/proof_of_address
In the latter case the enum is always the default value or if we add an action filter the error is "The value 'proof_of_address' is not valid."
Is there a way to make this escenario works besides trying to convert the value myself with a filter?
Thanks
c# asp.net-core json.net asp.net-core-2.1
We have a web API that uses snake case serialization for all the properties including enums, to enable it we used this in the startup:
services
.AddMvcCore()
.AddJsonOptions(opt =>
{
opt.SerializerSettings.DateTimeZoneHandling = Newtonsoft.Json.DateTimeZoneHandling.Local;
opt.SerializerSettings.Formatting = Newtonsoft.Json.Formatting.None;
opt.SerializerSettings.ContractResolver = new DefaultContractResolver
{
NamingStrategy = new SnakeCaseNamingStrategy { ProcessDictionaryKeys = true }
};
opt.SerializerSettings.NullValueHandling = Newtonsoft.Json.NullValueHandling.Ignore;
opt.SerializerSettings.Converters.Add(new StringEnumConverter());
})
.AddApiExplorer()
.AddJsonFormatters(j => j.ContractResolver = new DefaultContractResolver { NamingStrategy = new SnakeCaseNamingStrategy() { ProcessDictionaryKeys = true } })
That works great on properties, but we are having problems with routing and enums, for example we have this enum (we also tried with JsonProperty but fails in the same way):
[DataContract(Name = "document_type")]
public enum DocumentType
{
[EnumMember(Value = "passport")]
Passport,
[EnumMember(Value = "proof_of_address")]
ProofOfAddress,
}
and we are trying to search documents by type, so we have this route:
/clients/{clientId:guid/documents/{documentType}
and this in the controller:
[HttpGet]
[Route("/clients/{clientId:guid}/documents/{documentType}")]
public async Task<IActionResult> FindClientDocuments([FromRoute] Guid clientId, [FromRoute] DocumentType documentType)
with this route everything works great:
/clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/ProofOfAddress
but does not work with this:
clients/60a00cd4-59e2-4f52-871a-4029370f6dd8/documents/proof_of_address
In the latter case the enum is always the default value or if we add an action filter the error is "The value 'proof_of_address' is not valid."
Is there a way to make this escenario works besides trying to convert the value myself with a filter?
Thanks
c# asp.net-core json.net asp.net-core-2.1
c# asp.net-core json.net asp.net-core-2.1
edited Nov 13 '18 at 14:19
Kirk Larkin
19.9k33756
19.9k33756
asked Nov 13 '18 at 0:29


Juan ZamudioJuan Zamudio
131629
131629
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think this question can be broken into 2 parts,
- Custom string to Enum conversion using the
EnumMemberAttribute
- Telling MVC to use this custom conversion to bind model inputs.
I'm going to answer the 2nd part, and hopefully the first part should be relatively easy to implement/research. I included a link to a question directly about the first part.
Telling MVC to use custom enum conversion to bind model inputs.
Getting values from the client is always done via model binding, whereas json serialization mainly deals with formatting response data as json for output. Thus your solution should look into telling the model binder of the enum deserialization you want to use. (The default implementation of enum<=>string conversions does not look into attributes).
I tried the following custom model binder for my enum and it worked well. FooType
is my enum:
public enum FooType
{
[Description("test")]
TestFoo,
[Description("another")]
AnotherFooType
}
I skipped the [EnumMember]
attribute and opted for the [Description]
attribute only because I wanted to use Humanizer's Enum utility, but you can implement your own way of getting enum values from the EnumMember
attribute and just replace my call to DeHumanizeTo<FooType>()
, an example can be found on this question.
public class FooTypeBinder : IModelBinder
{
public Task BindModelAsync(ModelBindingContext bindingContext) => Task.Run(() => this.BindModel(bindingContext));
private void BindModel(ModelBindingContext bindingContext)
{
var valueProviderResult = bindingContext.ValueProvider.GetValue(bindingContext.ModelName);
if (valueProviderResult.Length == 0)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, "No value was provided for enum");
return;
}
var stringValue = valueProviderResult.FirstValue;
try
{
bindingContext.Model = this.FromString(stringValue);
}
catch (NoMatchFoundException ex)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, ex.Message);
}
}
private FooType FromString(string input)
{
//Here you should implement your custom way of checking the [EnumMember] attribute, and convert input into your enum.
if (Enum.TryParse(typeof(FooType), input, true, out object value))
{
return (FooType)value;
}
else return input.DehumanizeTo<FooType>();
}
}
In my controller, I then tell MVC to use this binder for binding my parameters as follows:
[HttpGet("{fooType}")]
public IActionResult GetFooItems([ModelBinder(BinderType = typeof(FooTypeBinder))] FooType fooType)
{
// Do your thing, your enum fooType is bound correctly
return this.Ok(fooType);
}
I hope this helps.
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272070%2fenum-serialization-in-routes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think this question can be broken into 2 parts,
- Custom string to Enum conversion using the
EnumMemberAttribute
- Telling MVC to use this custom conversion to bind model inputs.
I'm going to answer the 2nd part, and hopefully the first part should be relatively easy to implement/research. I included a link to a question directly about the first part.
Telling MVC to use custom enum conversion to bind model inputs.
Getting values from the client is always done via model binding, whereas json serialization mainly deals with formatting response data as json for output. Thus your solution should look into telling the model binder of the enum deserialization you want to use. (The default implementation of enum<=>string conversions does not look into attributes).
I tried the following custom model binder for my enum and it worked well. FooType
is my enum:
public enum FooType
{
[Description("test")]
TestFoo,
[Description("another")]
AnotherFooType
}
I skipped the [EnumMember]
attribute and opted for the [Description]
attribute only because I wanted to use Humanizer's Enum utility, but you can implement your own way of getting enum values from the EnumMember
attribute and just replace my call to DeHumanizeTo<FooType>()
, an example can be found on this question.
public class FooTypeBinder : IModelBinder
{
public Task BindModelAsync(ModelBindingContext bindingContext) => Task.Run(() => this.BindModel(bindingContext));
private void BindModel(ModelBindingContext bindingContext)
{
var valueProviderResult = bindingContext.ValueProvider.GetValue(bindingContext.ModelName);
if (valueProviderResult.Length == 0)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, "No value was provided for enum");
return;
}
var stringValue = valueProviderResult.FirstValue;
try
{
bindingContext.Model = this.FromString(stringValue);
}
catch (NoMatchFoundException ex)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, ex.Message);
}
}
private FooType FromString(string input)
{
//Here you should implement your custom way of checking the [EnumMember] attribute, and convert input into your enum.
if (Enum.TryParse(typeof(FooType), input, true, out object value))
{
return (FooType)value;
}
else return input.DehumanizeTo<FooType>();
}
}
In my controller, I then tell MVC to use this binder for binding my parameters as follows:
[HttpGet("{fooType}")]
public IActionResult GetFooItems([ModelBinder(BinderType = typeof(FooTypeBinder))] FooType fooType)
{
// Do your thing, your enum fooType is bound correctly
return this.Ok(fooType);
}
I hope this helps.
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
add a comment |
I think this question can be broken into 2 parts,
- Custom string to Enum conversion using the
EnumMemberAttribute
- Telling MVC to use this custom conversion to bind model inputs.
I'm going to answer the 2nd part, and hopefully the first part should be relatively easy to implement/research. I included a link to a question directly about the first part.
Telling MVC to use custom enum conversion to bind model inputs.
Getting values from the client is always done via model binding, whereas json serialization mainly deals with formatting response data as json for output. Thus your solution should look into telling the model binder of the enum deserialization you want to use. (The default implementation of enum<=>string conversions does not look into attributes).
I tried the following custom model binder for my enum and it worked well. FooType
is my enum:
public enum FooType
{
[Description("test")]
TestFoo,
[Description("another")]
AnotherFooType
}
I skipped the [EnumMember]
attribute and opted for the [Description]
attribute only because I wanted to use Humanizer's Enum utility, but you can implement your own way of getting enum values from the EnumMember
attribute and just replace my call to DeHumanizeTo<FooType>()
, an example can be found on this question.
public class FooTypeBinder : IModelBinder
{
public Task BindModelAsync(ModelBindingContext bindingContext) => Task.Run(() => this.BindModel(bindingContext));
private void BindModel(ModelBindingContext bindingContext)
{
var valueProviderResult = bindingContext.ValueProvider.GetValue(bindingContext.ModelName);
if (valueProviderResult.Length == 0)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, "No value was provided for enum");
return;
}
var stringValue = valueProviderResult.FirstValue;
try
{
bindingContext.Model = this.FromString(stringValue);
}
catch (NoMatchFoundException ex)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, ex.Message);
}
}
private FooType FromString(string input)
{
//Here you should implement your custom way of checking the [EnumMember] attribute, and convert input into your enum.
if (Enum.TryParse(typeof(FooType), input, true, out object value))
{
return (FooType)value;
}
else return input.DehumanizeTo<FooType>();
}
}
In my controller, I then tell MVC to use this binder for binding my parameters as follows:
[HttpGet("{fooType}")]
public IActionResult GetFooItems([ModelBinder(BinderType = typeof(FooTypeBinder))] FooType fooType)
{
// Do your thing, your enum fooType is bound correctly
return this.Ok(fooType);
}
I hope this helps.
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
add a comment |
I think this question can be broken into 2 parts,
- Custom string to Enum conversion using the
EnumMemberAttribute
- Telling MVC to use this custom conversion to bind model inputs.
I'm going to answer the 2nd part, and hopefully the first part should be relatively easy to implement/research. I included a link to a question directly about the first part.
Telling MVC to use custom enum conversion to bind model inputs.
Getting values from the client is always done via model binding, whereas json serialization mainly deals with formatting response data as json for output. Thus your solution should look into telling the model binder of the enum deserialization you want to use. (The default implementation of enum<=>string conversions does not look into attributes).
I tried the following custom model binder for my enum and it worked well. FooType
is my enum:
public enum FooType
{
[Description("test")]
TestFoo,
[Description("another")]
AnotherFooType
}
I skipped the [EnumMember]
attribute and opted for the [Description]
attribute only because I wanted to use Humanizer's Enum utility, but you can implement your own way of getting enum values from the EnumMember
attribute and just replace my call to DeHumanizeTo<FooType>()
, an example can be found on this question.
public class FooTypeBinder : IModelBinder
{
public Task BindModelAsync(ModelBindingContext bindingContext) => Task.Run(() => this.BindModel(bindingContext));
private void BindModel(ModelBindingContext bindingContext)
{
var valueProviderResult = bindingContext.ValueProvider.GetValue(bindingContext.ModelName);
if (valueProviderResult.Length == 0)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, "No value was provided for enum");
return;
}
var stringValue = valueProviderResult.FirstValue;
try
{
bindingContext.Model = this.FromString(stringValue);
}
catch (NoMatchFoundException ex)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, ex.Message);
}
}
private FooType FromString(string input)
{
//Here you should implement your custom way of checking the [EnumMember] attribute, and convert input into your enum.
if (Enum.TryParse(typeof(FooType), input, true, out object value))
{
return (FooType)value;
}
else return input.DehumanizeTo<FooType>();
}
}
In my controller, I then tell MVC to use this binder for binding my parameters as follows:
[HttpGet("{fooType}")]
public IActionResult GetFooItems([ModelBinder(BinderType = typeof(FooTypeBinder))] FooType fooType)
{
// Do your thing, your enum fooType is bound correctly
return this.Ok(fooType);
}
I hope this helps.
I think this question can be broken into 2 parts,
- Custom string to Enum conversion using the
EnumMemberAttribute
- Telling MVC to use this custom conversion to bind model inputs.
I'm going to answer the 2nd part, and hopefully the first part should be relatively easy to implement/research. I included a link to a question directly about the first part.
Telling MVC to use custom enum conversion to bind model inputs.
Getting values from the client is always done via model binding, whereas json serialization mainly deals with formatting response data as json for output. Thus your solution should look into telling the model binder of the enum deserialization you want to use. (The default implementation of enum<=>string conversions does not look into attributes).
I tried the following custom model binder for my enum and it worked well. FooType
is my enum:
public enum FooType
{
[Description("test")]
TestFoo,
[Description("another")]
AnotherFooType
}
I skipped the [EnumMember]
attribute and opted for the [Description]
attribute only because I wanted to use Humanizer's Enum utility, but you can implement your own way of getting enum values from the EnumMember
attribute and just replace my call to DeHumanizeTo<FooType>()
, an example can be found on this question.
public class FooTypeBinder : IModelBinder
{
public Task BindModelAsync(ModelBindingContext bindingContext) => Task.Run(() => this.BindModel(bindingContext));
private void BindModel(ModelBindingContext bindingContext)
{
var valueProviderResult = bindingContext.ValueProvider.GetValue(bindingContext.ModelName);
if (valueProviderResult.Length == 0)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, "No value was provided for enum");
return;
}
var stringValue = valueProviderResult.FirstValue;
try
{
bindingContext.Model = this.FromString(stringValue);
}
catch (NoMatchFoundException ex)
{
bindingContext.ModelState.AddModelError(bindingContext.ModelName, ex.Message);
}
}
private FooType FromString(string input)
{
//Here you should implement your custom way of checking the [EnumMember] attribute, and convert input into your enum.
if (Enum.TryParse(typeof(FooType), input, true, out object value))
{
return (FooType)value;
}
else return input.DehumanizeTo<FooType>();
}
}
In my controller, I then tell MVC to use this binder for binding my parameters as follows:
[HttpGet("{fooType}")]
public IActionResult GetFooItems([ModelBinder(BinderType = typeof(FooTypeBinder))] FooType fooType)
{
// Do your thing, your enum fooType is bound correctly
return this.Ok(fooType);
}
I hope this helps.
answered Nov 14 '18 at 10:24


gerryc.incgerryc.inc
55225
55225
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
add a comment |
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
your code works, but the enum in the controller was still the default, i had to change this bindingContext.Model = this.FromString(stringValue); to this bindingContext.Result = ModelBindingResult.Success(enumeration); thanks a lot
– Juan Zamudio
Nov 14 '18 at 17:20
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
@JuanZamudio Happy to help.
– gerryc.inc
Nov 15 '18 at 7:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272070%2fenum-serialization-in-routes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UPktwKbBLQB 3PrB X958,0,uBme0bU6qZbDmRJ7XiJ2mSsgdgnc83F,vNxqqOvTiPgRK,yOcjxlI RymmyVzO7DFnFxTEXwvS28sx d