How to change a QTreeView model on an action triggered signal in PyQt5?
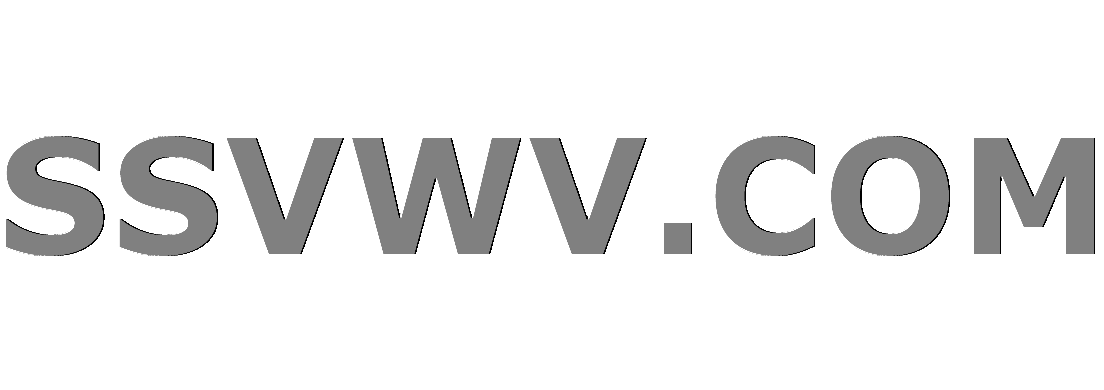
Multi tool use
I am trying to build a PyQt5 application that would display a tree view and populate it dynamically on a button press. But it crashes (Process finished with exit code 134 (interrupted by signal 6: SIGABRT)) whenever I try to set or populate the model from within a function assigned to an action triggered signal (although it works just fine if I instantiate the model, load the data and assign the model to the TreeView in the window __init__
itself rather than in a function assigned to a signal). How do I achieve the desired behaviour? The whole model content (including the set of columns) is meant to completely change often during runtime. The UI is designed in Qt Designer and generated with pyuic5.
Here is my window code:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
# model = MyModel() # UPDATE: useless, this wasn't here in the last pre-question version of the code actually
self.ui.actionLoad.triggered.connect(MainWindow.load) # UPDATE: Here is a mistake - should be self.load, not MainWindow.load
# @staticmethod # UPDATE: this wasn't here in the last pre-question version of the code actually
def load(self):
model = MyModel()
self.ui.treeViewLeft.setModel(model)
self.model.load() # UPDATE: Here is a mistake - should be model.load(), not self.model.load()
Here is my model code:
class MyModel(QStandardItemModel):
def __init__(self, *args, **kwargs):
super(MyModel, self).__init__(*args, **kwargs)
def load(self):
self.clear()
self.setHorizontalHeaderLabels(["Name", "Attr1", "Attr2"])
self.appendRow([QStandardItem('item1'), QStandardItem('attr11'), QStandardItem('attr21')])
self.appendRow([QStandardItem('item2'), QStandardItem('attr12'), QStandardItem('attr22')])
self.appendRow([QStandardItem('item3'), QStandardItem('attr13'), QStandardItem('attr23')])
python pyqt5 qtreeview
add a comment |
I am trying to build a PyQt5 application that would display a tree view and populate it dynamically on a button press. But it crashes (Process finished with exit code 134 (interrupted by signal 6: SIGABRT)) whenever I try to set or populate the model from within a function assigned to an action triggered signal (although it works just fine if I instantiate the model, load the data and assign the model to the TreeView in the window __init__
itself rather than in a function assigned to a signal). How do I achieve the desired behaviour? The whole model content (including the set of columns) is meant to completely change often during runtime. The UI is designed in Qt Designer and generated with pyuic5.
Here is my window code:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
# model = MyModel() # UPDATE: useless, this wasn't here in the last pre-question version of the code actually
self.ui.actionLoad.triggered.connect(MainWindow.load) # UPDATE: Here is a mistake - should be self.load, not MainWindow.load
# @staticmethod # UPDATE: this wasn't here in the last pre-question version of the code actually
def load(self):
model = MyModel()
self.ui.treeViewLeft.setModel(model)
self.model.load() # UPDATE: Here is a mistake - should be model.load(), not self.model.load()
Here is my model code:
class MyModel(QStandardItemModel):
def __init__(self, *args, **kwargs):
super(MyModel, self).__init__(*args, **kwargs)
def load(self):
self.clear()
self.setHorizontalHeaderLabels(["Name", "Attr1", "Attr2"])
self.appendRow([QStandardItem('item1'), QStandardItem('attr11'), QStandardItem('attr21')])
self.appendRow([QStandardItem('item2'), QStandardItem('attr12'), QStandardItem('attr22')])
self.appendRow([QStandardItem('item3'), QStandardItem('attr13'), QStandardItem('attr23')])
python pyqt5 qtreeview
add a comment |
I am trying to build a PyQt5 application that would display a tree view and populate it dynamically on a button press. But it crashes (Process finished with exit code 134 (interrupted by signal 6: SIGABRT)) whenever I try to set or populate the model from within a function assigned to an action triggered signal (although it works just fine if I instantiate the model, load the data and assign the model to the TreeView in the window __init__
itself rather than in a function assigned to a signal). How do I achieve the desired behaviour? The whole model content (including the set of columns) is meant to completely change often during runtime. The UI is designed in Qt Designer and generated with pyuic5.
Here is my window code:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
# model = MyModel() # UPDATE: useless, this wasn't here in the last pre-question version of the code actually
self.ui.actionLoad.triggered.connect(MainWindow.load) # UPDATE: Here is a mistake - should be self.load, not MainWindow.load
# @staticmethod # UPDATE: this wasn't here in the last pre-question version of the code actually
def load(self):
model = MyModel()
self.ui.treeViewLeft.setModel(model)
self.model.load() # UPDATE: Here is a mistake - should be model.load(), not self.model.load()
Here is my model code:
class MyModel(QStandardItemModel):
def __init__(self, *args, **kwargs):
super(MyModel, self).__init__(*args, **kwargs)
def load(self):
self.clear()
self.setHorizontalHeaderLabels(["Name", "Attr1", "Attr2"])
self.appendRow([QStandardItem('item1'), QStandardItem('attr11'), QStandardItem('attr21')])
self.appendRow([QStandardItem('item2'), QStandardItem('attr12'), QStandardItem('attr22')])
self.appendRow([QStandardItem('item3'), QStandardItem('attr13'), QStandardItem('attr23')])
python pyqt5 qtreeview
I am trying to build a PyQt5 application that would display a tree view and populate it dynamically on a button press. But it crashes (Process finished with exit code 134 (interrupted by signal 6: SIGABRT)) whenever I try to set or populate the model from within a function assigned to an action triggered signal (although it works just fine if I instantiate the model, load the data and assign the model to the TreeView in the window __init__
itself rather than in a function assigned to a signal). How do I achieve the desired behaviour? The whole model content (including the set of columns) is meant to completely change often during runtime. The UI is designed in Qt Designer and generated with pyuic5.
Here is my window code:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
# model = MyModel() # UPDATE: useless, this wasn't here in the last pre-question version of the code actually
self.ui.actionLoad.triggered.connect(MainWindow.load) # UPDATE: Here is a mistake - should be self.load, not MainWindow.load
# @staticmethod # UPDATE: this wasn't here in the last pre-question version of the code actually
def load(self):
model = MyModel()
self.ui.treeViewLeft.setModel(model)
self.model.load() # UPDATE: Here is a mistake - should be model.load(), not self.model.load()
Here is my model code:
class MyModel(QStandardItemModel):
def __init__(self, *args, **kwargs):
super(MyModel, self).__init__(*args, **kwargs)
def load(self):
self.clear()
self.setHorizontalHeaderLabels(["Name", "Attr1", "Attr2"])
self.appendRow([QStandardItem('item1'), QStandardItem('attr11'), QStandardItem('attr21')])
self.appendRow([QStandardItem('item2'), QStandardItem('attr12'), QStandardItem('attr22')])
self.appendRow([QStandardItem('item3'), QStandardItem('attr13'), QStandardItem('attr23')])
python pyqt5 qtreeview
python pyqt5 qtreeview
edited Nov 21 '18 at 6:15
Ivan
asked Nov 21 '18 at 5:13
IvanIvan
27.3k74209338
27.3k74209338
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I recommend you execute your code in the CMD or terminal in these cases since many IDEs have limitations in these cases. By running it you get this error message:
Traceback (most recent call last):
File "main.py", line 29, in load
self.ui.treeViewLeft.setModel(model)
AttributeError: 'bool' object has no attribute 'ui'
Aborted (core dumped)
A static method indicates that this method does not belong to an object of the class but to the class itself, and if you want to modify an object it should not be a static method so remove that decorator. On the other hand you must connect the signal to the slot by using self.
The solution is the next:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
model = MyModel()
self.ui.actionLoad.triggered.connect(self.load)
def load(self):
model = MyModel(self)
self.ui.treeViewLeft.setModel(model)
model.load()
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and callingself.model.load()
instead ofmodel.load()
was another mistake I haven't noticed. Alsomodel = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.
– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405627%2fhow-to-change-a-qtreeview-model-on-an-action-triggered-signal-in-pyqt5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I recommend you execute your code in the CMD or terminal in these cases since many IDEs have limitations in these cases. By running it you get this error message:
Traceback (most recent call last):
File "main.py", line 29, in load
self.ui.treeViewLeft.setModel(model)
AttributeError: 'bool' object has no attribute 'ui'
Aborted (core dumped)
A static method indicates that this method does not belong to an object of the class but to the class itself, and if you want to modify an object it should not be a static method so remove that decorator. On the other hand you must connect the signal to the slot by using self.
The solution is the next:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
model = MyModel()
self.ui.actionLoad.triggered.connect(self.load)
def load(self):
model = MyModel(self)
self.ui.treeViewLeft.setModel(model)
model.load()
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and callingself.model.load()
instead ofmodel.load()
was another mistake I haven't noticed. Alsomodel = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.
– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
|
show 2 more comments
I recommend you execute your code in the CMD or terminal in these cases since many IDEs have limitations in these cases. By running it you get this error message:
Traceback (most recent call last):
File "main.py", line 29, in load
self.ui.treeViewLeft.setModel(model)
AttributeError: 'bool' object has no attribute 'ui'
Aborted (core dumped)
A static method indicates that this method does not belong to an object of the class but to the class itself, and if you want to modify an object it should not be a static method so remove that decorator. On the other hand you must connect the signal to the slot by using self.
The solution is the next:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
model = MyModel()
self.ui.actionLoad.triggered.connect(self.load)
def load(self):
model = MyModel(self)
self.ui.treeViewLeft.setModel(model)
model.load()
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and callingself.model.load()
instead ofmodel.load()
was another mistake I haven't noticed. Alsomodel = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.
– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
|
show 2 more comments
I recommend you execute your code in the CMD or terminal in these cases since many IDEs have limitations in these cases. By running it you get this error message:
Traceback (most recent call last):
File "main.py", line 29, in load
self.ui.treeViewLeft.setModel(model)
AttributeError: 'bool' object has no attribute 'ui'
Aborted (core dumped)
A static method indicates that this method does not belong to an object of the class but to the class itself, and if you want to modify an object it should not be a static method so remove that decorator. On the other hand you must connect the signal to the slot by using self.
The solution is the next:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
model = MyModel()
self.ui.actionLoad.triggered.connect(self.load)
def load(self):
model = MyModel(self)
self.ui.treeViewLeft.setModel(model)
model.load()
I recommend you execute your code in the CMD or terminal in these cases since many IDEs have limitations in these cases. By running it you get this error message:
Traceback (most recent call last):
File "main.py", line 29, in load
self.ui.treeViewLeft.setModel(model)
AttributeError: 'bool' object has no attribute 'ui'
Aborted (core dumped)
A static method indicates that this method does not belong to an object of the class but to the class itself, and if you want to modify an object it should not be a static method so remove that decorator. On the other hand you must connect the signal to the slot by using self.
The solution is the next:
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
model = MyModel()
self.ui.actionLoad.triggered.connect(self.load)
def load(self):
model = MyModel(self)
self.ui.treeViewLeft.setModel(model)
model.load()
answered Nov 21 '18 at 5:36


eyllanesceyllanesc
80.8k103259
80.8k103259
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and callingself.model.load()
instead ofmodel.load()
was another mistake I haven't noticed. Alsomodel = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.
– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
|
show 2 more comments
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and callingself.model.load()
instead ofmodel.load()
was another mistake I haven't noticed. Alsomodel = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.
– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
Thanks. The suggestion to use a terninal was unexpectedly useful - indeed much more useful error messages are shown then. I have never seen PyCharm hiding these before while I was only coding purely console apps without GUI so I wasn't prepared.
– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and calling
self.model.load()
instead of model.load()
was another mistake I haven't noticed. Also model = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.– Ivan
Nov 21 '18 at 6:09
I have actually removed @staticmethod before asking and only copy-pasted it here by a mistake yet I have actually forgotten to replace the reference to the class with self and calling
self.model.load()
instead of model.load()
was another mistake I haven't noticed. Also model = MyModel()
in the init does nothing here and I have just forgotten to remove it, you can remove it in the answer.– Ivan
Nov 21 '18 at 6:09
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
A funny thing I still can't understand is hod did it happen to be a bool…
– Ivan
Nov 21 '18 at 6:10
1
1
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan triggered is a signal that transports a Boolean that by default is false, unless you enable the QAction to be checked, but by default it is not and that value is False. So since it is a static method the object is not transported only the data and it is passed as a parameter so the self will take the Boolean value that transports the signal (remember that the self is a nickname by custom representing the instance but the static method does not use the instance so sel does not have meaning and will be the first parameter of the function)
– eyllanesc
Nov 21 '18 at 6:15
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
@Ivan If my answer helps you, do not forget to mark it as correct.
– eyllanesc
Nov 21 '18 at 6:16
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405627%2fhow-to-change-a-qtreeview-model-on-an-action-triggered-signal-in-pyqt5%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XpO,M,vPoNom5nG CnA8n HmZ