python - how to disable cache in Flask? Getting 500 server error during second POST request sent to server...
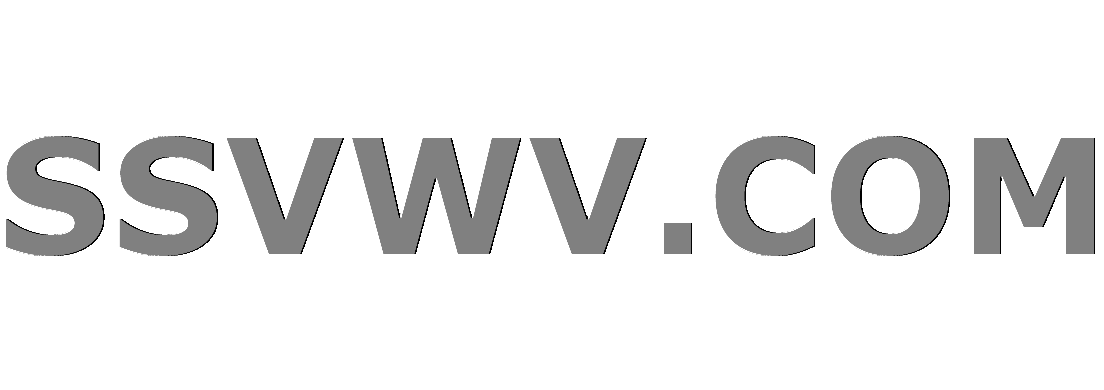
Multi tool use
I 'think' I have some caching issue.
my webservice is receiving data through POST request and saving it to disk.
Following is my code:
@app.route('/ws_part' , methods=['POST'])
def ws_part():
request_data = request.get_json()
#placeholder for workstation number and part number
received_data ={'ws_no':request_data['workstation'],
'part_no':request_data['part']}
#Checking if workstation number is already available
global updated
updated = 'no'
for i in repository:
if i['ws'] == received_data['ws']:
i['part'] = received_data['part']
updated = 'yes'
if(updated!='yes'):
new_input = received_data
repository.append(new_input)
return jsonify({'repository': repository})
Issue I am facing:
1.The very first request goes through successfully and gives 200 OK response and workstation number and part coming with the request get saved in 'repository' placeholder.
2.But the very next request throws 500 server error
Error:
File "API_ws_par.py", line 23, in ws_part
if i['ws'] == received_data['ws']:
KeyError: 'ws'
3.Funny thing happening is:
If at this point I restart my web service and trigger the POST request again, the data coming with this new request is getting overwritten and I am losing my previously saved data.
4.Also, the consequent second POST request throws the same 500 server error
Please, guide if this is something to do with cache?
If yes, then please let me know how to do it?
Thanks in advance.
python web-services caching flask flask-restful
add a comment |
I 'think' I have some caching issue.
my webservice is receiving data through POST request and saving it to disk.
Following is my code:
@app.route('/ws_part' , methods=['POST'])
def ws_part():
request_data = request.get_json()
#placeholder for workstation number and part number
received_data ={'ws_no':request_data['workstation'],
'part_no':request_data['part']}
#Checking if workstation number is already available
global updated
updated = 'no'
for i in repository:
if i['ws'] == received_data['ws']:
i['part'] = received_data['part']
updated = 'yes'
if(updated!='yes'):
new_input = received_data
repository.append(new_input)
return jsonify({'repository': repository})
Issue I am facing:
1.The very first request goes through successfully and gives 200 OK response and workstation number and part coming with the request get saved in 'repository' placeholder.
2.But the very next request throws 500 server error
Error:
File "API_ws_par.py", line 23, in ws_part
if i['ws'] == received_data['ws']:
KeyError: 'ws'
3.Funny thing happening is:
If at this point I restart my web service and trigger the POST request again, the data coming with this new request is getting overwritten and I am losing my previously saved data.
4.Also, the consequent second POST request throws the same 500 server error
Please, guide if this is something to do with cache?
If yes, then please let me know how to do it?
Thanks in advance.
python web-services caching flask flask-restful
add a comment |
I 'think' I have some caching issue.
my webservice is receiving data through POST request and saving it to disk.
Following is my code:
@app.route('/ws_part' , methods=['POST'])
def ws_part():
request_data = request.get_json()
#placeholder for workstation number and part number
received_data ={'ws_no':request_data['workstation'],
'part_no':request_data['part']}
#Checking if workstation number is already available
global updated
updated = 'no'
for i in repository:
if i['ws'] == received_data['ws']:
i['part'] = received_data['part']
updated = 'yes'
if(updated!='yes'):
new_input = received_data
repository.append(new_input)
return jsonify({'repository': repository})
Issue I am facing:
1.The very first request goes through successfully and gives 200 OK response and workstation number and part coming with the request get saved in 'repository' placeholder.
2.But the very next request throws 500 server error
Error:
File "API_ws_par.py", line 23, in ws_part
if i['ws'] == received_data['ws']:
KeyError: 'ws'
3.Funny thing happening is:
If at this point I restart my web service and trigger the POST request again, the data coming with this new request is getting overwritten and I am losing my previously saved data.
4.Also, the consequent second POST request throws the same 500 server error
Please, guide if this is something to do with cache?
If yes, then please let me know how to do it?
Thanks in advance.
python web-services caching flask flask-restful
I 'think' I have some caching issue.
my webservice is receiving data through POST request and saving it to disk.
Following is my code:
@app.route('/ws_part' , methods=['POST'])
def ws_part():
request_data = request.get_json()
#placeholder for workstation number and part number
received_data ={'ws_no':request_data['workstation'],
'part_no':request_data['part']}
#Checking if workstation number is already available
global updated
updated = 'no'
for i in repository:
if i['ws'] == received_data['ws']:
i['part'] = received_data['part']
updated = 'yes'
if(updated!='yes'):
new_input = received_data
repository.append(new_input)
return jsonify({'repository': repository})
Issue I am facing:
1.The very first request goes through successfully and gives 200 OK response and workstation number and part coming with the request get saved in 'repository' placeholder.
2.But the very next request throws 500 server error
Error:
File "API_ws_par.py", line 23, in ws_part
if i['ws'] == received_data['ws']:
KeyError: 'ws'
3.Funny thing happening is:
If at this point I restart my web service and trigger the POST request again, the data coming with this new request is getting overwritten and I am losing my previously saved data.
4.Also, the consequent second POST request throws the same 500 server error
Please, guide if this is something to do with cache?
If yes, then please let me know how to do it?
Thanks in advance.
python web-services caching flask flask-restful
python web-services caching flask flask-restful
asked Nov 21 '18 at 5:12
SummaSumma
206
206
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
this is not a caching problem, it's a problem in the logic of the function,
on the first call, you probably don't get to the line of code generating the exception,
The problem is on the second call, the key name is not 'ws' it's 'ws_no',
if you change these lines:
for i in repository:
if i['ws'] == received_data['ws_no']:
i['part'] = received_data['part_no']
updated = 'yes'
to use 'ws_no', and 'part_no' you won't have the key error.
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405614%2fpython-how-to-disable-cache-in-flask-getting-500-server-error-during-second-p%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
this is not a caching problem, it's a problem in the logic of the function,
on the first call, you probably don't get to the line of code generating the exception,
The problem is on the second call, the key name is not 'ws' it's 'ws_no',
if you change these lines:
for i in repository:
if i['ws'] == received_data['ws_no']:
i['part'] = received_data['part_no']
updated = 'yes'
to use 'ws_no', and 'part_no' you won't have the key error.
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
add a comment |
this is not a caching problem, it's a problem in the logic of the function,
on the first call, you probably don't get to the line of code generating the exception,
The problem is on the second call, the key name is not 'ws' it's 'ws_no',
if you change these lines:
for i in repository:
if i['ws'] == received_data['ws_no']:
i['part'] = received_data['part_no']
updated = 'yes'
to use 'ws_no', and 'part_no' you won't have the key error.
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
add a comment |
this is not a caching problem, it's a problem in the logic of the function,
on the first call, you probably don't get to the line of code generating the exception,
The problem is on the second call, the key name is not 'ws' it's 'ws_no',
if you change these lines:
for i in repository:
if i['ws'] == received_data['ws_no']:
i['part'] = received_data['part_no']
updated = 'yes'
to use 'ws_no', and 'part_no' you won't have the key error.
this is not a caching problem, it's a problem in the logic of the function,
on the first call, you probably don't get to the line of code generating the exception,
The problem is on the second call, the key name is not 'ws' it's 'ws_no',
if you change these lines:
for i in repository:
if i['ws'] == received_data['ws_no']:
i['part'] = received_data['part_no']
updated = 'yes'
to use 'ws_no', and 'part_no' you won't have the key error.
answered Nov 21 '18 at 5:33
rnkl904rnkl904
16
16
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
add a comment |
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
Thanks @rnkl904 for pointing this out. I have changed my code accordingly: for i in repository: if i['ws_no'] == received_data['ws_no']: i['part_no'] = received_data['part_no'] updated = 'yes' Then I restarted my service and triggered the requests once again.But during the second POST, again getting the same error: File "API_ws_par.py", line 24, in ws_part if i['ws_no'] == received_data['ws_no']: KeyError: 'ws' --Where my code doesn't have 'ws' variable anymore.is that cache issue this time? Thanks in advance
– Summa
Nov 21 '18 at 5:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53405614%2fpython-how-to-disable-cache-in-flask-getting-500-server-error-during-second-p%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R720w1TzzSUedA