C++ using operator overload
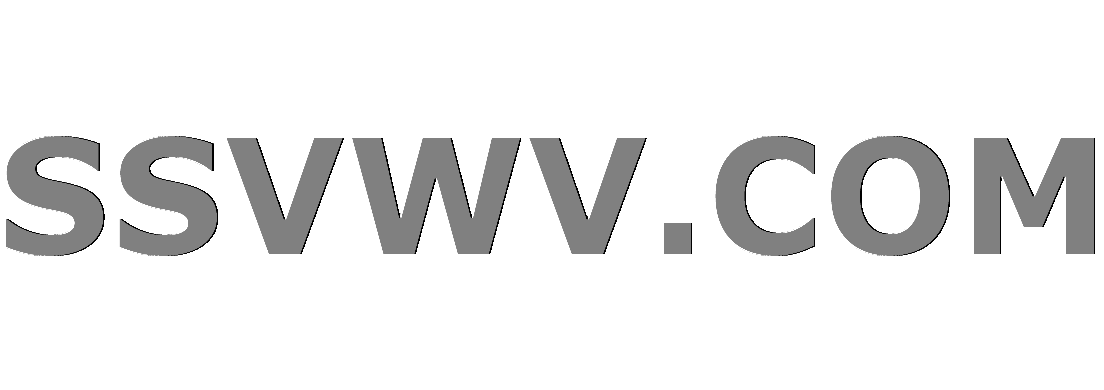
Multi tool use
up vote
-2
down vote
favorite
#include <iostream>
using namespace std;
class matrix
{
private:
int row;
int col;
int **data;
public:
matrix(int r=0,int c=0)
{
row=r;
col=c;
data=new int*[row];
for(int i=0; i<row; i++)
{
data[i]=new int [col];
}
}
friend void createMatrix (int row, int col, int num, matrix& mat);
friend istream &operator>>(istream&in,matrix &mat);
friend ostream &operator<<(ostream&out,matrix &mat);
matrix operator+ (matrix mat)
{
matrix z(row,col);
if((row==mat.row)&&(col==mat.col))
{
for(int i=0 ; i<row ; i++)
{
for(int j=0 ; j<col; j++)
{
z.data[i][j]=data[i][j]+mat.data[i][j];
}
}
}
else
{
cout<<"Matrix that aren't the same size can't be added"<<endl;
}
return z;
}
};
ostream &operator<<(ostream&out,matrix &mat)
{
for(int i=0; i<mat.row; i++)
{
for(int j=0; j<mat.col; j++)
{
out<<mat.data[i][j]<<" ";
}
out<<endl;
}
return out;
}
istream &operator >>(istream &in,matrix& mat)
{
cout<<"Enter the size of your matrix"<<endl;
in>>mat.row;
in>>mat.col;
mat.data=new int*[mat.row];
cout<<"Enter the elements of your matrix"<<endl;
for(int x=0; x<mat.row; x++)
{
mat.data[x]=new int[mat.col];
}
for(int i=0; i<mat.row; i++)
{
for(int j=0 ; j<mat.col; j++)
{
in>>mat.data[i][j];
}
}
return in;
}
int main()
{
matrix x,y;
cin>>x>>y;
cout<<x+y<<endl;
return 0;
}
void createMatrix (int row, int col, int num, matrix& mat)
{
mat.row = row;
mat.col = col;
mat.data = new int* [row];
for (int i = 0; i < row; i++)
mat.data[i] = new int [col];
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
mat.data[i][j] = num[i * col + j];
}
When I try to run, I get the error:
|83|error: no match for 'operator<<' (operand types are 'std::ostream {aka std::basic_ostream<char>}' and 'matrix')|
but when I change the main to:
matrix x,y,z;
cin>>x>>y;
z=x+y;
cout<<z<<endl;
return 0;
I get no problems although I can't use that because I have to do about 14 different operator overloading I'm not sure if i'ts a code error or a compiler error. Any idea what I can do to solve it?
c++ operator-overloading
add a comment |
up vote
-2
down vote
favorite
#include <iostream>
using namespace std;
class matrix
{
private:
int row;
int col;
int **data;
public:
matrix(int r=0,int c=0)
{
row=r;
col=c;
data=new int*[row];
for(int i=0; i<row; i++)
{
data[i]=new int [col];
}
}
friend void createMatrix (int row, int col, int num, matrix& mat);
friend istream &operator>>(istream&in,matrix &mat);
friend ostream &operator<<(ostream&out,matrix &mat);
matrix operator+ (matrix mat)
{
matrix z(row,col);
if((row==mat.row)&&(col==mat.col))
{
for(int i=0 ; i<row ; i++)
{
for(int j=0 ; j<col; j++)
{
z.data[i][j]=data[i][j]+mat.data[i][j];
}
}
}
else
{
cout<<"Matrix that aren't the same size can't be added"<<endl;
}
return z;
}
};
ostream &operator<<(ostream&out,matrix &mat)
{
for(int i=0; i<mat.row; i++)
{
for(int j=0; j<mat.col; j++)
{
out<<mat.data[i][j]<<" ";
}
out<<endl;
}
return out;
}
istream &operator >>(istream &in,matrix& mat)
{
cout<<"Enter the size of your matrix"<<endl;
in>>mat.row;
in>>mat.col;
mat.data=new int*[mat.row];
cout<<"Enter the elements of your matrix"<<endl;
for(int x=0; x<mat.row; x++)
{
mat.data[x]=new int[mat.col];
}
for(int i=0; i<mat.row; i++)
{
for(int j=0 ; j<mat.col; j++)
{
in>>mat.data[i][j];
}
}
return in;
}
int main()
{
matrix x,y;
cin>>x>>y;
cout<<x+y<<endl;
return 0;
}
void createMatrix (int row, int col, int num, matrix& mat)
{
mat.row = row;
mat.col = col;
mat.data = new int* [row];
for (int i = 0; i < row; i++)
mat.data[i] = new int [col];
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
mat.data[i][j] = num[i * col + j];
}
When I try to run, I get the error:
|83|error: no match for 'operator<<' (operand types are 'std::ostream {aka std::basic_ostream<char>}' and 'matrix')|
but when I change the main to:
matrix x,y,z;
cin>>x>>y;
z=x+y;
cout<<z<<endl;
return 0;
I get no problems although I can't use that because I have to do about 14 different operator overloading I'm not sure if i'ts a code error or a compiler error. Any idea what I can do to solve it?
c++ operator-overloading
2
Please format the code better
– Peter
Nov 9 at 13:15
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
#include <iostream>
using namespace std;
class matrix
{
private:
int row;
int col;
int **data;
public:
matrix(int r=0,int c=0)
{
row=r;
col=c;
data=new int*[row];
for(int i=0; i<row; i++)
{
data[i]=new int [col];
}
}
friend void createMatrix (int row, int col, int num, matrix& mat);
friend istream &operator>>(istream&in,matrix &mat);
friend ostream &operator<<(ostream&out,matrix &mat);
matrix operator+ (matrix mat)
{
matrix z(row,col);
if((row==mat.row)&&(col==mat.col))
{
for(int i=0 ; i<row ; i++)
{
for(int j=0 ; j<col; j++)
{
z.data[i][j]=data[i][j]+mat.data[i][j];
}
}
}
else
{
cout<<"Matrix that aren't the same size can't be added"<<endl;
}
return z;
}
};
ostream &operator<<(ostream&out,matrix &mat)
{
for(int i=0; i<mat.row; i++)
{
for(int j=0; j<mat.col; j++)
{
out<<mat.data[i][j]<<" ";
}
out<<endl;
}
return out;
}
istream &operator >>(istream &in,matrix& mat)
{
cout<<"Enter the size of your matrix"<<endl;
in>>mat.row;
in>>mat.col;
mat.data=new int*[mat.row];
cout<<"Enter the elements of your matrix"<<endl;
for(int x=0; x<mat.row; x++)
{
mat.data[x]=new int[mat.col];
}
for(int i=0; i<mat.row; i++)
{
for(int j=0 ; j<mat.col; j++)
{
in>>mat.data[i][j];
}
}
return in;
}
int main()
{
matrix x,y;
cin>>x>>y;
cout<<x+y<<endl;
return 0;
}
void createMatrix (int row, int col, int num, matrix& mat)
{
mat.row = row;
mat.col = col;
mat.data = new int* [row];
for (int i = 0; i < row; i++)
mat.data[i] = new int [col];
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
mat.data[i][j] = num[i * col + j];
}
When I try to run, I get the error:
|83|error: no match for 'operator<<' (operand types are 'std::ostream {aka std::basic_ostream<char>}' and 'matrix')|
but when I change the main to:
matrix x,y,z;
cin>>x>>y;
z=x+y;
cout<<z<<endl;
return 0;
I get no problems although I can't use that because I have to do about 14 different operator overloading I'm not sure if i'ts a code error or a compiler error. Any idea what I can do to solve it?
c++ operator-overloading
#include <iostream>
using namespace std;
class matrix
{
private:
int row;
int col;
int **data;
public:
matrix(int r=0,int c=0)
{
row=r;
col=c;
data=new int*[row];
for(int i=0; i<row; i++)
{
data[i]=new int [col];
}
}
friend void createMatrix (int row, int col, int num, matrix& mat);
friend istream &operator>>(istream&in,matrix &mat);
friend ostream &operator<<(ostream&out,matrix &mat);
matrix operator+ (matrix mat)
{
matrix z(row,col);
if((row==mat.row)&&(col==mat.col))
{
for(int i=0 ; i<row ; i++)
{
for(int j=0 ; j<col; j++)
{
z.data[i][j]=data[i][j]+mat.data[i][j];
}
}
}
else
{
cout<<"Matrix that aren't the same size can't be added"<<endl;
}
return z;
}
};
ostream &operator<<(ostream&out,matrix &mat)
{
for(int i=0; i<mat.row; i++)
{
for(int j=0; j<mat.col; j++)
{
out<<mat.data[i][j]<<" ";
}
out<<endl;
}
return out;
}
istream &operator >>(istream &in,matrix& mat)
{
cout<<"Enter the size of your matrix"<<endl;
in>>mat.row;
in>>mat.col;
mat.data=new int*[mat.row];
cout<<"Enter the elements of your matrix"<<endl;
for(int x=0; x<mat.row; x++)
{
mat.data[x]=new int[mat.col];
}
for(int i=0; i<mat.row; i++)
{
for(int j=0 ; j<mat.col; j++)
{
in>>mat.data[i][j];
}
}
return in;
}
int main()
{
matrix x,y;
cin>>x>>y;
cout<<x+y<<endl;
return 0;
}
void createMatrix (int row, int col, int num, matrix& mat)
{
mat.row = row;
mat.col = col;
mat.data = new int* [row];
for (int i = 0; i < row; i++)
mat.data[i] = new int [col];
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
mat.data[i][j] = num[i * col + j];
}
When I try to run, I get the error:
|83|error: no match for 'operator<<' (operand types are 'std::ostream {aka std::basic_ostream<char>}' and 'matrix')|
but when I change the main to:
matrix x,y,z;
cin>>x>>y;
z=x+y;
cout<<z<<endl;
return 0;
I get no problems although I can't use that because I have to do about 14 different operator overloading I'm not sure if i'ts a code error or a compiler error. Any idea what I can do to solve it?
c++ operator-overloading
c++ operator-overloading
edited Nov 9 at 13:16
Matthieu Brucher
10.1k21935
10.1k21935
asked Nov 9 at 13:12


Mohammad Sameh
1
1
2
Please format the code better
– Peter
Nov 9 at 13:15
add a comment |
2
Please format the code better
– Peter
Nov 9 at 13:15
2
2
Please format the code better
– Peter
Nov 9 at 13:15
Please format the code better
– Peter
Nov 9 at 13:15
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
You have defined your operator<<
to take a matrix reference
ostream &operator<<(ostream&out,matrix &mat)
while the output of the expression
x+y
is a temporary object that cannot be passed as a reference. You must either pass it as a const reference const matrix& mat
or by value matrix mat
. In this case, you should use a const reference, to avoid copying the whole matrix.
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
add a comment |
up vote
1
down vote
Use a const matrix:
ostream &operator<<(ostream&out,const matrix &mat)
This creates a temporary matrix
cout<<x+y<<endl;
It cannot be passed by ref only. And as it is for output, it should be const ref anyway.
1
As well as modifying the signature infriend ostream &operator<<(ostream&out, const matrix &mat);
to addconst
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226374%2fc-using-operator-overload%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
You have defined your operator<<
to take a matrix reference
ostream &operator<<(ostream&out,matrix &mat)
while the output of the expression
x+y
is a temporary object that cannot be passed as a reference. You must either pass it as a const reference const matrix& mat
or by value matrix mat
. In this case, you should use a const reference, to avoid copying the whole matrix.
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
add a comment |
up vote
2
down vote
You have defined your operator<<
to take a matrix reference
ostream &operator<<(ostream&out,matrix &mat)
while the output of the expression
x+y
is a temporary object that cannot be passed as a reference. You must either pass it as a const reference const matrix& mat
or by value matrix mat
. In this case, you should use a const reference, to avoid copying the whole matrix.
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
add a comment |
up vote
2
down vote
up vote
2
down vote
You have defined your operator<<
to take a matrix reference
ostream &operator<<(ostream&out,matrix &mat)
while the output of the expression
x+y
is a temporary object that cannot be passed as a reference. You must either pass it as a const reference const matrix& mat
or by value matrix mat
. In this case, you should use a const reference, to avoid copying the whole matrix.
You have defined your operator<<
to take a matrix reference
ostream &operator<<(ostream&out,matrix &mat)
while the output of the expression
x+y
is a temporary object that cannot be passed as a reference. You must either pass it as a const reference const matrix& mat
or by value matrix mat
. In this case, you should use a const reference, to avoid copying the whole matrix.
answered Nov 9 at 13:35


sveinbr
965
965
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
add a comment |
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
worked like a charm,thanks.
– Mohammad Sameh
Nov 9 at 14:24
add a comment |
up vote
1
down vote
Use a const matrix:
ostream &operator<<(ostream&out,const matrix &mat)
This creates a temporary matrix
cout<<x+y<<endl;
It cannot be passed by ref only. And as it is for output, it should be const ref anyway.
1
As well as modifying the signature infriend ostream &operator<<(ostream&out, const matrix &mat);
to addconst
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
add a comment |
up vote
1
down vote
Use a const matrix:
ostream &operator<<(ostream&out,const matrix &mat)
This creates a temporary matrix
cout<<x+y<<endl;
It cannot be passed by ref only. And as it is for output, it should be const ref anyway.
1
As well as modifying the signature infriend ostream &operator<<(ostream&out, const matrix &mat);
to addconst
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
add a comment |
up vote
1
down vote
up vote
1
down vote
Use a const matrix:
ostream &operator<<(ostream&out,const matrix &mat)
This creates a temporary matrix
cout<<x+y<<endl;
It cannot be passed by ref only. And as it is for output, it should be const ref anyway.
Use a const matrix:
ostream &operator<<(ostream&out,const matrix &mat)
This creates a temporary matrix
cout<<x+y<<endl;
It cannot be passed by ref only. And as it is for output, it should be const ref anyway.
answered Nov 9 at 13:17
Matthieu Brucher
10.1k21935
10.1k21935
1
As well as modifying the signature infriend ostream &operator<<(ostream&out, const matrix &mat);
to addconst
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
add a comment |
1
As well as modifying the signature infriend ostream &operator<<(ostream&out, const matrix &mat);
to addconst
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
1
1
As well as modifying the signature in
friend ostream &operator<<(ostream&out, const matrix &mat);
to add const
– Everyone
Nov 9 at 13:21
As well as modifying the signature in
friend ostream &operator<<(ostream&out, const matrix &mat);
to add const
– Everyone
Nov 9 at 13:21
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
Of course, both declarations have to be changed indeed.
– Matthieu Brucher
Nov 9 at 13:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53226374%2fc-using-operator-overload%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eL6O hL1Q6g,v9 pA7kNC,viuuiqMEYCXbPaZQjgnl16dFhU
2
Please format the code better
– Peter
Nov 9 at 13:15