Why does my Hailstone Sequence function using recursion only output two values?
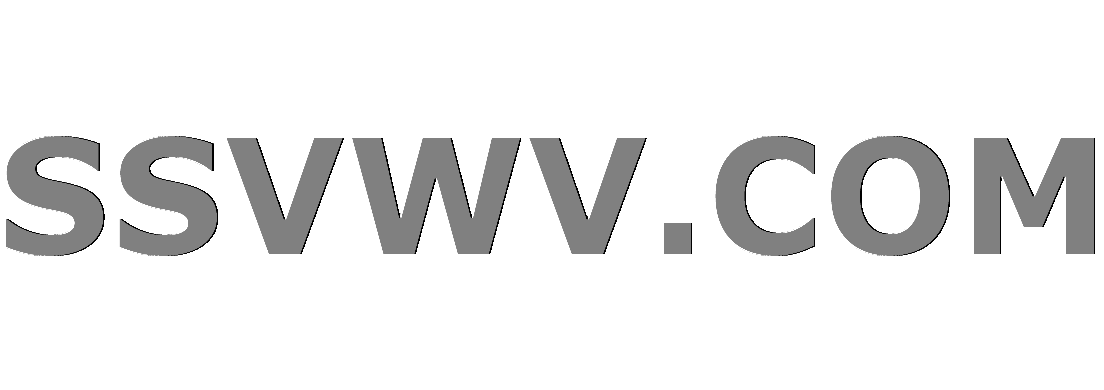
Multi tool use
up vote
2
down vote
favorite
I have the following code:
fn hailSeq(number: i32) -> Vec<i32> {
let mut vec = Vec::new();
vec.push(number);
if number == 1 {
vec.push(1);
return vec;
}
if number % 2 == 0 {
let num = number / 2;
vec.push(num);
hailSeq(num);
} else {
let num = 3 * number + 1;
vec.push(num);
hailSeq(num);
}
return vec;
}
It calculates the Hailstone sequence and stops at 1. The output should look like this for hailSeq(11)
:
[11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1]
However, my output looks like this:
[11, 34]
I am not really sure why this is occurring. Perhaps there is a limit on recursion in Rust that I don't know about, but I'm sure there's probably just an error in my code.
recursion rust
add a comment |
up vote
2
down vote
favorite
I have the following code:
fn hailSeq(number: i32) -> Vec<i32> {
let mut vec = Vec::new();
vec.push(number);
if number == 1 {
vec.push(1);
return vec;
}
if number % 2 == 0 {
let num = number / 2;
vec.push(num);
hailSeq(num);
} else {
let num = 3 * number + 1;
vec.push(num);
hailSeq(num);
}
return vec;
}
It calculates the Hailstone sequence and stops at 1. The output should look like this for hailSeq(11)
:
[11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1]
However, my output looks like this:
[11, 34]
I am not really sure why this is occurring. Perhaps there is a limit on recursion in Rust that I don't know about, but I'm sure there's probably just an error in my code.
recursion rust
1
Please userustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicitreturn
for the last statement, that is very unrusty. Just writevec
(withouth return and;
)
– hellow
Nov 9 at 12:51
1
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
1
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have the following code:
fn hailSeq(number: i32) -> Vec<i32> {
let mut vec = Vec::new();
vec.push(number);
if number == 1 {
vec.push(1);
return vec;
}
if number % 2 == 0 {
let num = number / 2;
vec.push(num);
hailSeq(num);
} else {
let num = 3 * number + 1;
vec.push(num);
hailSeq(num);
}
return vec;
}
It calculates the Hailstone sequence and stops at 1. The output should look like this for hailSeq(11)
:
[11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1]
However, my output looks like this:
[11, 34]
I am not really sure why this is occurring. Perhaps there is a limit on recursion in Rust that I don't know about, but I'm sure there's probably just an error in my code.
recursion rust
I have the following code:
fn hailSeq(number: i32) -> Vec<i32> {
let mut vec = Vec::new();
vec.push(number);
if number == 1 {
vec.push(1);
return vec;
}
if number % 2 == 0 {
let num = number / 2;
vec.push(num);
hailSeq(num);
} else {
let num = 3 * number + 1;
vec.push(num);
hailSeq(num);
}
return vec;
}
It calculates the Hailstone sequence and stops at 1. The output should look like this for hailSeq(11)
:
[11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, 1]
However, my output looks like this:
[11, 34]
I am not really sure why this is occurring. Perhaps there is a limit on recursion in Rust that I don't know about, but I'm sure there's probably just an error in my code.
recursion rust
recursion rust
edited Nov 9 at 14:58
Shepmaster
145k11279413
145k11279413
asked Nov 9 at 12:47
SeePlusPlus
375
375
1
Please userustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicitreturn
for the last statement, that is very unrusty. Just writevec
(withouth return and;
)
– hellow
Nov 9 at 12:51
1
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
1
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39
add a comment |
1
Please userustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicitreturn
for the last statement, that is very unrusty. Just writevec
(withouth return and;
)
– hellow
Nov 9 at 12:51
1
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
1
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39
1
1
Please use
rustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicit return
for the last statement, that is very unrusty. Just write vec
(withouth return and ;
)– hellow
Nov 9 at 12:51
Please use
rustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicit return
for the last statement, that is very unrusty. Just write vec
(withouth return and ;
)– hellow
Nov 9 at 12:51
1
1
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
1
1
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39
add a comment |
1 Answer
1
active
oldest
votes
up vote
3
down vote
accepted
Your problem is not Rust-specific, but a more general problem.
On every call of hailSeq
you create a new Vec
every time, so that only the first vec
(from the first call) would be used and returned, hence the [11, 34]
(11
from the third line, 34
from the tenth line).
To fix this you have two options, I will provide one here.
The first one would be to extend the current vec
with the returned vec
, e.g. myvec.extend_from_slice(&returned_vec)
.
The second solution involves creating a vec
on startup and passing the same instance to every call of the function.
fn hail_seq(number: i32) -> Vec<i32> {
fn inner(number: i32, vec: &mut Vec<i32>) {
vec.push(number);
if number == 1 {
return;
}
if number % 2 == 0 {
let num = number / 2;
inner(num, vec);
} else {
let num = 3 * number + 1;
inner(num, vec);
}
}
let mut v = vec!;
inner(number, &mut v);
v
}
fn main() {
println!("{:?}", hail_seq(11));
}
(playground)
As a side-note: If you know that a number can't be negative, use a u32
instead because you will find errors at compile time instead of runtime.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53225992%2fwhy-does-my-hailstone-sequence-function-using-recursion-only-output-two-values%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
Your problem is not Rust-specific, but a more general problem.
On every call of hailSeq
you create a new Vec
every time, so that only the first vec
(from the first call) would be used and returned, hence the [11, 34]
(11
from the third line, 34
from the tenth line).
To fix this you have two options, I will provide one here.
The first one would be to extend the current vec
with the returned vec
, e.g. myvec.extend_from_slice(&returned_vec)
.
The second solution involves creating a vec
on startup and passing the same instance to every call of the function.
fn hail_seq(number: i32) -> Vec<i32> {
fn inner(number: i32, vec: &mut Vec<i32>) {
vec.push(number);
if number == 1 {
return;
}
if number % 2 == 0 {
let num = number / 2;
inner(num, vec);
} else {
let num = 3 * number + 1;
inner(num, vec);
}
}
let mut v = vec!;
inner(number, &mut v);
v
}
fn main() {
println!("{:?}", hail_seq(11));
}
(playground)
As a side-note: If you know that a number can't be negative, use a u32
instead because you will find errors at compile time instead of runtime.
add a comment |
up vote
3
down vote
accepted
Your problem is not Rust-specific, but a more general problem.
On every call of hailSeq
you create a new Vec
every time, so that only the first vec
(from the first call) would be used and returned, hence the [11, 34]
(11
from the third line, 34
from the tenth line).
To fix this you have two options, I will provide one here.
The first one would be to extend the current vec
with the returned vec
, e.g. myvec.extend_from_slice(&returned_vec)
.
The second solution involves creating a vec
on startup and passing the same instance to every call of the function.
fn hail_seq(number: i32) -> Vec<i32> {
fn inner(number: i32, vec: &mut Vec<i32>) {
vec.push(number);
if number == 1 {
return;
}
if number % 2 == 0 {
let num = number / 2;
inner(num, vec);
} else {
let num = 3 * number + 1;
inner(num, vec);
}
}
let mut v = vec!;
inner(number, &mut v);
v
}
fn main() {
println!("{:?}", hail_seq(11));
}
(playground)
As a side-note: If you know that a number can't be negative, use a u32
instead because you will find errors at compile time instead of runtime.
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
Your problem is not Rust-specific, but a more general problem.
On every call of hailSeq
you create a new Vec
every time, so that only the first vec
(from the first call) would be used and returned, hence the [11, 34]
(11
from the third line, 34
from the tenth line).
To fix this you have two options, I will provide one here.
The first one would be to extend the current vec
with the returned vec
, e.g. myvec.extend_from_slice(&returned_vec)
.
The second solution involves creating a vec
on startup and passing the same instance to every call of the function.
fn hail_seq(number: i32) -> Vec<i32> {
fn inner(number: i32, vec: &mut Vec<i32>) {
vec.push(number);
if number == 1 {
return;
}
if number % 2 == 0 {
let num = number / 2;
inner(num, vec);
} else {
let num = 3 * number + 1;
inner(num, vec);
}
}
let mut v = vec!;
inner(number, &mut v);
v
}
fn main() {
println!("{:?}", hail_seq(11));
}
(playground)
As a side-note: If you know that a number can't be negative, use a u32
instead because you will find errors at compile time instead of runtime.
Your problem is not Rust-specific, but a more general problem.
On every call of hailSeq
you create a new Vec
every time, so that only the first vec
(from the first call) would be used and returned, hence the [11, 34]
(11
from the third line, 34
from the tenth line).
To fix this you have two options, I will provide one here.
The first one would be to extend the current vec
with the returned vec
, e.g. myvec.extend_from_slice(&returned_vec)
.
The second solution involves creating a vec
on startup and passing the same instance to every call of the function.
fn hail_seq(number: i32) -> Vec<i32> {
fn inner(number: i32, vec: &mut Vec<i32>) {
vec.push(number);
if number == 1 {
return;
}
if number % 2 == 0 {
let num = number / 2;
inner(num, vec);
} else {
let num = 3 * number + 1;
inner(num, vec);
}
}
let mut v = vec!;
inner(number, &mut v);
v
}
fn main() {
println!("{:?}", hail_seq(11));
}
(playground)
As a side-note: If you know that a number can't be negative, use a u32
instead because you will find errors at compile time instead of runtime.
edited Nov 9 at 15:00
Shepmaster
145k11279413
145k11279413
answered Nov 9 at 12:59
hellow
4,44432042
4,44432042
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53225992%2fwhy-does-my-hailstone-sequence-function-using-recursion-only-output-two-values%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s5QIWcy486rHZRWVd6a F
1
Please use
rustfmt
to format your code properly next time. Second, in rust we do not use camelCase, but snake_case, e.g. your function name should be hail_seq. Third, don't use an explicitreturn
for the last statement, that is very unrusty. Just writevec
(withouth return and;
)– hellow
Nov 9 at 12:51
1
You may explain or link how to generate a hail sequence.
– hellow
Nov 9 at 12:52
1
The more idiomatic in Rust is to use an iterator.
– Boiethios
Nov 9 at 13:10
The fun is there is an implementation for Rust on the link that I added rosettacode.org/wiki/Hailstone_sequence#Rust ;)
– Stargateur
Nov 9 at 13:10
See also stackoverflow.com/a/52905147/279627
– Sven Marnach
Nov 9 at 13:39