How to do audio expansion/normalization (emphasise difference between high and low)
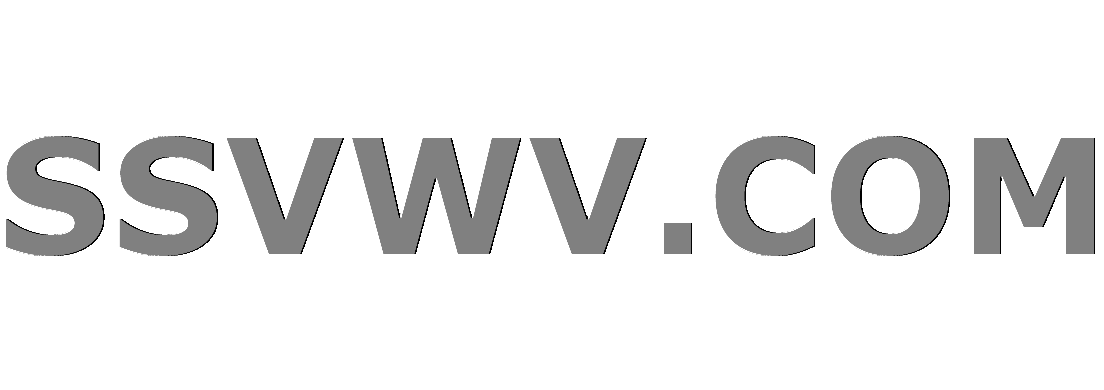
Multi tool use
I'm trying to find way to emphasise the difference between high and low points in the audio. I can't seem to find documentation on how to do this - perhaps this can be done with ffmpeg. Would really appreciate some pointers from someone who knows more about signal processing.
audio ffmpeg
add a comment |
I'm trying to find way to emphasise the difference between high and low points in the audio. I can't seem to find documentation on how to do this - perhaps this can be done with ffmpeg. Would really appreciate some pointers from someone who knows more about signal processing.
audio ffmpeg
1
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11
add a comment |
I'm trying to find way to emphasise the difference between high and low points in the audio. I can't seem to find documentation on how to do this - perhaps this can be done with ffmpeg. Would really appreciate some pointers from someone who knows more about signal processing.
audio ffmpeg
I'm trying to find way to emphasise the difference between high and low points in the audio. I can't seem to find documentation on how to do this - perhaps this can be done with ffmpeg. Would really appreciate some pointers from someone who knows more about signal processing.
audio ffmpeg
audio ffmpeg
asked Nov 12 '18 at 9:53


foobar
5282720
5282720
1
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11
add a comment |
1
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11
1
1
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11
add a comment |
1 Answer
1
active
oldest
votes
Fundamentally, an expander is the opposite of a compressor *; you may have more luck finding documentation about how to implement those. They also have a lot in common with a noise gate.
The expander
The basic approach is to implement an envelope follower, and use the value of the envelope to scale the audio source. An envelope follower tries to track the amplitude of the audio signal.
A basic pythonic pseudocode framework looks a bit like this:
envelope_follower e # some envelope follower, we'll replace this
for each sample x:
amplitude = e.get(x) # use the envelope follower to estimate the amplitude of e
x = expand(x, amplitude) # apply some expansion operation
At it's most basic, the expand
operation looks like this (assuming your samples are between -1.0 and 1.0):
def expand(x, amplitude):
return x * amplitude
There are more sophisticated approaches, for example clamping and scaling amplitude so it never drops below 0.5, or applying some non-linear function to amplitude before multiplying.
# just an example
def expand(x, amplitude):
return x * clamp(1.2 * amplitude - 0.2 * (amplitude * amplitude), 0.3, 1.0)
Envelope followers
The quality of the compressor/expander depends almost entirely on how you implement the envelope follower.
It's not an exact science, as a very accurate envelope follower might cause some nasty audible effects in certain situations - there are trade-offs to be made.
As with all of these things, there are a lot of approaches! Here's a couple:
Filtered rectifier
One of the simplest approaches - particularly if you already have a library of signal processing blocks - is the lowpass-filtered rectifier.
It works like this:
class envelope_follower:
lowpassfilter filter;
def get(x):
return filter.process( abs(x) )
The controls you get here are basically around your filter design, and lowpass cutoff. Using a simple leaky-accumulator filter will get you a long way.
Attack-Release follower
People usually want more control over their expander, and it's sometimes difficult to think about the actual effects of the filtered rectifier - tweaking one parameter might change a lot of its behaviour.
It's often very desirable for real-world signals for compressors/expanders to respond very quickly (for example, to a piano or drum impact), and be slow to release (so the tail of the piano note isn't suddenly cut off)
An Attack-Release follower gives more precise control by specifying a number of parameters:
- Two thresholds
- A threshold, above which sounds should be made louder
- A threshold, below which sounds should be made quieter (not necessarily the same threshold, but can be!)
- Two time periods:
- How long it takes to reach full loudness when the first threshold is crossed (This is the Attack parameter)
- How long it takes to reach quietness (Release)
One basic approach to implementing one of these is:
class envelope_follower:
# Parameters required. These are just made up
attack_threshold = 0.6
release_threshold = 0.3
attack_time = 10 # in samples
release_time = 1000 # in samples
amp = 0.0
def get(x):
# we still work with the absolute value.
# You might use another measure of amplitude here like RMS
# or even the filtered rectifier above
a = abs(x)
if x > attack_threshold:
amp += (1.0 / attack_time)
else if x < release_threshold:
amp -= (1.0 / release_time)
amp = clamp(amp, 0.0, 1.0)
return amp
One common extension to this type of follower is to add a Hold parameter, that specifies a minimum length of time that the expander should be wide open. This avoids the envelope creating an audible triangle or sawtooth wave on lower-frequency signals.
An even more sophisticated approach is to do a full Attack-Decay-Sustain-Release, which lets you control the transients and is commonly used as a drum treatment.
Getting wild
From here, you can:
Create a smoother
expand
functionFairly trivially adjust the above into a Compander - a combination device that quietens low sounds, but also quietens overly-loud sounds;
Split a signal into multiple frequency bands, and compress/expand each one separately. This is commonly done do get real in-your-face maximum amplitude during music mastering;
Adjust Attack/Hold/Release based on the spectral content of the sound you're expanding. Very short attack/release times are fine for high-frequency signals, but sound awful for low-frequency signals;
Add a mild saturating distortion for sounds over the threshold; this can make things perceptually louder, even if the signal still has same the maximum amplitude. You ideally want a saturator that doesn't affect signals under the threshold at all.
Good luck!
* not to be confused with MP3-style compression. A compressor squishes the dynamic range.
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53259601%2fhow-to-do-audio-expansion-normalization-emphasise-difference-between-high-and-l%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Fundamentally, an expander is the opposite of a compressor *; you may have more luck finding documentation about how to implement those. They also have a lot in common with a noise gate.
The expander
The basic approach is to implement an envelope follower, and use the value of the envelope to scale the audio source. An envelope follower tries to track the amplitude of the audio signal.
A basic pythonic pseudocode framework looks a bit like this:
envelope_follower e # some envelope follower, we'll replace this
for each sample x:
amplitude = e.get(x) # use the envelope follower to estimate the amplitude of e
x = expand(x, amplitude) # apply some expansion operation
At it's most basic, the expand
operation looks like this (assuming your samples are between -1.0 and 1.0):
def expand(x, amplitude):
return x * amplitude
There are more sophisticated approaches, for example clamping and scaling amplitude so it never drops below 0.5, or applying some non-linear function to amplitude before multiplying.
# just an example
def expand(x, amplitude):
return x * clamp(1.2 * amplitude - 0.2 * (amplitude * amplitude), 0.3, 1.0)
Envelope followers
The quality of the compressor/expander depends almost entirely on how you implement the envelope follower.
It's not an exact science, as a very accurate envelope follower might cause some nasty audible effects in certain situations - there are trade-offs to be made.
As with all of these things, there are a lot of approaches! Here's a couple:
Filtered rectifier
One of the simplest approaches - particularly if you already have a library of signal processing blocks - is the lowpass-filtered rectifier.
It works like this:
class envelope_follower:
lowpassfilter filter;
def get(x):
return filter.process( abs(x) )
The controls you get here are basically around your filter design, and lowpass cutoff. Using a simple leaky-accumulator filter will get you a long way.
Attack-Release follower
People usually want more control over their expander, and it's sometimes difficult to think about the actual effects of the filtered rectifier - tweaking one parameter might change a lot of its behaviour.
It's often very desirable for real-world signals for compressors/expanders to respond very quickly (for example, to a piano or drum impact), and be slow to release (so the tail of the piano note isn't suddenly cut off)
An Attack-Release follower gives more precise control by specifying a number of parameters:
- Two thresholds
- A threshold, above which sounds should be made louder
- A threshold, below which sounds should be made quieter (not necessarily the same threshold, but can be!)
- Two time periods:
- How long it takes to reach full loudness when the first threshold is crossed (This is the Attack parameter)
- How long it takes to reach quietness (Release)
One basic approach to implementing one of these is:
class envelope_follower:
# Parameters required. These are just made up
attack_threshold = 0.6
release_threshold = 0.3
attack_time = 10 # in samples
release_time = 1000 # in samples
amp = 0.0
def get(x):
# we still work with the absolute value.
# You might use another measure of amplitude here like RMS
# or even the filtered rectifier above
a = abs(x)
if x > attack_threshold:
amp += (1.0 / attack_time)
else if x < release_threshold:
amp -= (1.0 / release_time)
amp = clamp(amp, 0.0, 1.0)
return amp
One common extension to this type of follower is to add a Hold parameter, that specifies a minimum length of time that the expander should be wide open. This avoids the envelope creating an audible triangle or sawtooth wave on lower-frequency signals.
An even more sophisticated approach is to do a full Attack-Decay-Sustain-Release, which lets you control the transients and is commonly used as a drum treatment.
Getting wild
From here, you can:
Create a smoother
expand
functionFairly trivially adjust the above into a Compander - a combination device that quietens low sounds, but also quietens overly-loud sounds;
Split a signal into multiple frequency bands, and compress/expand each one separately. This is commonly done do get real in-your-face maximum amplitude during music mastering;
Adjust Attack/Hold/Release based on the spectral content of the sound you're expanding. Very short attack/release times are fine for high-frequency signals, but sound awful for low-frequency signals;
Add a mild saturating distortion for sounds over the threshold; this can make things perceptually louder, even if the signal still has same the maximum amplitude. You ideally want a saturator that doesn't affect signals under the threshold at all.
Good luck!
* not to be confused with MP3-style compression. A compressor squishes the dynamic range.
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
add a comment |
Fundamentally, an expander is the opposite of a compressor *; you may have more luck finding documentation about how to implement those. They also have a lot in common with a noise gate.
The expander
The basic approach is to implement an envelope follower, and use the value of the envelope to scale the audio source. An envelope follower tries to track the amplitude of the audio signal.
A basic pythonic pseudocode framework looks a bit like this:
envelope_follower e # some envelope follower, we'll replace this
for each sample x:
amplitude = e.get(x) # use the envelope follower to estimate the amplitude of e
x = expand(x, amplitude) # apply some expansion operation
At it's most basic, the expand
operation looks like this (assuming your samples are between -1.0 and 1.0):
def expand(x, amplitude):
return x * amplitude
There are more sophisticated approaches, for example clamping and scaling amplitude so it never drops below 0.5, or applying some non-linear function to amplitude before multiplying.
# just an example
def expand(x, amplitude):
return x * clamp(1.2 * amplitude - 0.2 * (amplitude * amplitude), 0.3, 1.0)
Envelope followers
The quality of the compressor/expander depends almost entirely on how you implement the envelope follower.
It's not an exact science, as a very accurate envelope follower might cause some nasty audible effects in certain situations - there are trade-offs to be made.
As with all of these things, there are a lot of approaches! Here's a couple:
Filtered rectifier
One of the simplest approaches - particularly if you already have a library of signal processing blocks - is the lowpass-filtered rectifier.
It works like this:
class envelope_follower:
lowpassfilter filter;
def get(x):
return filter.process( abs(x) )
The controls you get here are basically around your filter design, and lowpass cutoff. Using a simple leaky-accumulator filter will get you a long way.
Attack-Release follower
People usually want more control over their expander, and it's sometimes difficult to think about the actual effects of the filtered rectifier - tweaking one parameter might change a lot of its behaviour.
It's often very desirable for real-world signals for compressors/expanders to respond very quickly (for example, to a piano or drum impact), and be slow to release (so the tail of the piano note isn't suddenly cut off)
An Attack-Release follower gives more precise control by specifying a number of parameters:
- Two thresholds
- A threshold, above which sounds should be made louder
- A threshold, below which sounds should be made quieter (not necessarily the same threshold, but can be!)
- Two time periods:
- How long it takes to reach full loudness when the first threshold is crossed (This is the Attack parameter)
- How long it takes to reach quietness (Release)
One basic approach to implementing one of these is:
class envelope_follower:
# Parameters required. These are just made up
attack_threshold = 0.6
release_threshold = 0.3
attack_time = 10 # in samples
release_time = 1000 # in samples
amp = 0.0
def get(x):
# we still work with the absolute value.
# You might use another measure of amplitude here like RMS
# or even the filtered rectifier above
a = abs(x)
if x > attack_threshold:
amp += (1.0 / attack_time)
else if x < release_threshold:
amp -= (1.0 / release_time)
amp = clamp(amp, 0.0, 1.0)
return amp
One common extension to this type of follower is to add a Hold parameter, that specifies a minimum length of time that the expander should be wide open. This avoids the envelope creating an audible triangle or sawtooth wave on lower-frequency signals.
An even more sophisticated approach is to do a full Attack-Decay-Sustain-Release, which lets you control the transients and is commonly used as a drum treatment.
Getting wild
From here, you can:
Create a smoother
expand
functionFairly trivially adjust the above into a Compander - a combination device that quietens low sounds, but also quietens overly-loud sounds;
Split a signal into multiple frequency bands, and compress/expand each one separately. This is commonly done do get real in-your-face maximum amplitude during music mastering;
Adjust Attack/Hold/Release based on the spectral content of the sound you're expanding. Very short attack/release times are fine for high-frequency signals, but sound awful for low-frequency signals;
Add a mild saturating distortion for sounds over the threshold; this can make things perceptually louder, even if the signal still has same the maximum amplitude. You ideally want a saturator that doesn't affect signals under the threshold at all.
Good luck!
* not to be confused with MP3-style compression. A compressor squishes the dynamic range.
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
add a comment |
Fundamentally, an expander is the opposite of a compressor *; you may have more luck finding documentation about how to implement those. They also have a lot in common with a noise gate.
The expander
The basic approach is to implement an envelope follower, and use the value of the envelope to scale the audio source. An envelope follower tries to track the amplitude of the audio signal.
A basic pythonic pseudocode framework looks a bit like this:
envelope_follower e # some envelope follower, we'll replace this
for each sample x:
amplitude = e.get(x) # use the envelope follower to estimate the amplitude of e
x = expand(x, amplitude) # apply some expansion operation
At it's most basic, the expand
operation looks like this (assuming your samples are between -1.0 and 1.0):
def expand(x, amplitude):
return x * amplitude
There are more sophisticated approaches, for example clamping and scaling amplitude so it never drops below 0.5, or applying some non-linear function to amplitude before multiplying.
# just an example
def expand(x, amplitude):
return x * clamp(1.2 * amplitude - 0.2 * (amplitude * amplitude), 0.3, 1.0)
Envelope followers
The quality of the compressor/expander depends almost entirely on how you implement the envelope follower.
It's not an exact science, as a very accurate envelope follower might cause some nasty audible effects in certain situations - there are trade-offs to be made.
As with all of these things, there are a lot of approaches! Here's a couple:
Filtered rectifier
One of the simplest approaches - particularly if you already have a library of signal processing blocks - is the lowpass-filtered rectifier.
It works like this:
class envelope_follower:
lowpassfilter filter;
def get(x):
return filter.process( abs(x) )
The controls you get here are basically around your filter design, and lowpass cutoff. Using a simple leaky-accumulator filter will get you a long way.
Attack-Release follower
People usually want more control over their expander, and it's sometimes difficult to think about the actual effects of the filtered rectifier - tweaking one parameter might change a lot of its behaviour.
It's often very desirable for real-world signals for compressors/expanders to respond very quickly (for example, to a piano or drum impact), and be slow to release (so the tail of the piano note isn't suddenly cut off)
An Attack-Release follower gives more precise control by specifying a number of parameters:
- Two thresholds
- A threshold, above which sounds should be made louder
- A threshold, below which sounds should be made quieter (not necessarily the same threshold, but can be!)
- Two time periods:
- How long it takes to reach full loudness when the first threshold is crossed (This is the Attack parameter)
- How long it takes to reach quietness (Release)
One basic approach to implementing one of these is:
class envelope_follower:
# Parameters required. These are just made up
attack_threshold = 0.6
release_threshold = 0.3
attack_time = 10 # in samples
release_time = 1000 # in samples
amp = 0.0
def get(x):
# we still work with the absolute value.
# You might use another measure of amplitude here like RMS
# or even the filtered rectifier above
a = abs(x)
if x > attack_threshold:
amp += (1.0 / attack_time)
else if x < release_threshold:
amp -= (1.0 / release_time)
amp = clamp(amp, 0.0, 1.0)
return amp
One common extension to this type of follower is to add a Hold parameter, that specifies a minimum length of time that the expander should be wide open. This avoids the envelope creating an audible triangle or sawtooth wave on lower-frequency signals.
An even more sophisticated approach is to do a full Attack-Decay-Sustain-Release, which lets you control the transients and is commonly used as a drum treatment.
Getting wild
From here, you can:
Create a smoother
expand
functionFairly trivially adjust the above into a Compander - a combination device that quietens low sounds, but also quietens overly-loud sounds;
Split a signal into multiple frequency bands, and compress/expand each one separately. This is commonly done do get real in-your-face maximum amplitude during music mastering;
Adjust Attack/Hold/Release based on the spectral content of the sound you're expanding. Very short attack/release times are fine for high-frequency signals, but sound awful for low-frequency signals;
Add a mild saturating distortion for sounds over the threshold; this can make things perceptually louder, even if the signal still has same the maximum amplitude. You ideally want a saturator that doesn't affect signals under the threshold at all.
Good luck!
* not to be confused with MP3-style compression. A compressor squishes the dynamic range.
Fundamentally, an expander is the opposite of a compressor *; you may have more luck finding documentation about how to implement those. They also have a lot in common with a noise gate.
The expander
The basic approach is to implement an envelope follower, and use the value of the envelope to scale the audio source. An envelope follower tries to track the amplitude of the audio signal.
A basic pythonic pseudocode framework looks a bit like this:
envelope_follower e # some envelope follower, we'll replace this
for each sample x:
amplitude = e.get(x) # use the envelope follower to estimate the amplitude of e
x = expand(x, amplitude) # apply some expansion operation
At it's most basic, the expand
operation looks like this (assuming your samples are between -1.0 and 1.0):
def expand(x, amplitude):
return x * amplitude
There are more sophisticated approaches, for example clamping and scaling amplitude so it never drops below 0.5, or applying some non-linear function to amplitude before multiplying.
# just an example
def expand(x, amplitude):
return x * clamp(1.2 * amplitude - 0.2 * (amplitude * amplitude), 0.3, 1.0)
Envelope followers
The quality of the compressor/expander depends almost entirely on how you implement the envelope follower.
It's not an exact science, as a very accurate envelope follower might cause some nasty audible effects in certain situations - there are trade-offs to be made.
As with all of these things, there are a lot of approaches! Here's a couple:
Filtered rectifier
One of the simplest approaches - particularly if you already have a library of signal processing blocks - is the lowpass-filtered rectifier.
It works like this:
class envelope_follower:
lowpassfilter filter;
def get(x):
return filter.process( abs(x) )
The controls you get here are basically around your filter design, and lowpass cutoff. Using a simple leaky-accumulator filter will get you a long way.
Attack-Release follower
People usually want more control over their expander, and it's sometimes difficult to think about the actual effects of the filtered rectifier - tweaking one parameter might change a lot of its behaviour.
It's often very desirable for real-world signals for compressors/expanders to respond very quickly (for example, to a piano or drum impact), and be slow to release (so the tail of the piano note isn't suddenly cut off)
An Attack-Release follower gives more precise control by specifying a number of parameters:
- Two thresholds
- A threshold, above which sounds should be made louder
- A threshold, below which sounds should be made quieter (not necessarily the same threshold, but can be!)
- Two time periods:
- How long it takes to reach full loudness when the first threshold is crossed (This is the Attack parameter)
- How long it takes to reach quietness (Release)
One basic approach to implementing one of these is:
class envelope_follower:
# Parameters required. These are just made up
attack_threshold = 0.6
release_threshold = 0.3
attack_time = 10 # in samples
release_time = 1000 # in samples
amp = 0.0
def get(x):
# we still work with the absolute value.
# You might use another measure of amplitude here like RMS
# or even the filtered rectifier above
a = abs(x)
if x > attack_threshold:
amp += (1.0 / attack_time)
else if x < release_threshold:
amp -= (1.0 / release_time)
amp = clamp(amp, 0.0, 1.0)
return amp
One common extension to this type of follower is to add a Hold parameter, that specifies a minimum length of time that the expander should be wide open. This avoids the envelope creating an audible triangle or sawtooth wave on lower-frequency signals.
An even more sophisticated approach is to do a full Attack-Decay-Sustain-Release, which lets you control the transients and is commonly used as a drum treatment.
Getting wild
From here, you can:
Create a smoother
expand
functionFairly trivially adjust the above into a Compander - a combination device that quietens low sounds, but also quietens overly-loud sounds;
Split a signal into multiple frequency bands, and compress/expand each one separately. This is commonly done do get real in-your-face maximum amplitude during music mastering;
Adjust Attack/Hold/Release based on the spectral content of the sound you're expanding. Very short attack/release times are fine for high-frequency signals, but sound awful for low-frequency signals;
Add a mild saturating distortion for sounds over the threshold; this can make things perceptually louder, even if the signal still has same the maximum amplitude. You ideally want a saturator that doesn't affect signals under the threshold at all.
Good luck!
* not to be confused with MP3-style compression. A compressor squishes the dynamic range.
edited Nov 28 '18 at 13:01
answered Nov 15 '18 at 1:23
kibibu
4,20412836
4,20412836
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
add a comment |
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
Wow, this is super helpful, thank you. Great overview to get me started!
– foobar
Nov 21 '18 at 12:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53259601%2fhow-to-do-audio-expansion-normalization-emphasise-difference-between-high-and-l%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kQno lWjuKfA8X1Ho,MSix4KB8S,0 RaKT,FuA MuzP,ns,4ERAWSjg,01b BbPjeoVjbm 94j,47ccRH3weFEQf 0bXzCVRw5WUH,sbdLH
1
Are you looking for advice as to how to code up the expander algorithm, or a library to do it?
– kibibu
Nov 12 '18 at 10:35
If a library with such a capability exists I would love to know about it (I would have to do it for many files, so not by hand). Otherwise, I would like some pointers on how to implement such an algorithm.
– foobar
Nov 12 '18 at 18:07
A paper or article that talks about it would also work
– foobar
Nov 12 '18 at 18:11