Listener declared in a Kotlin Object class
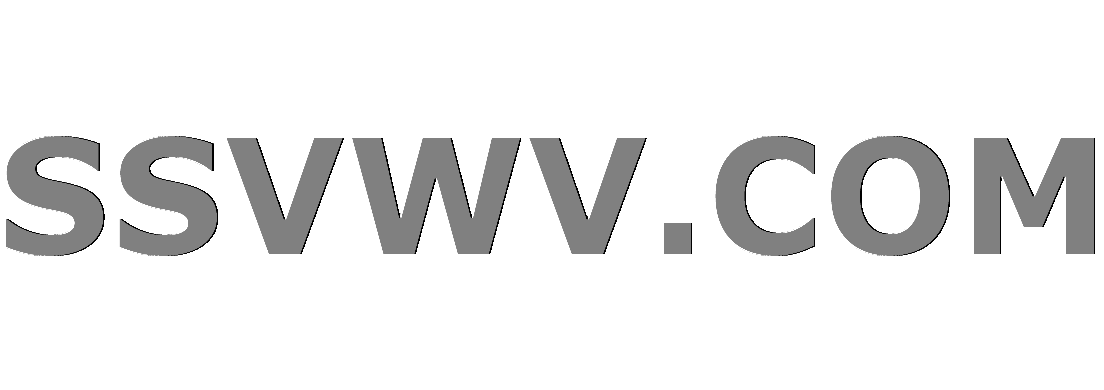
Multi tool use
I have an object
class in Kotlin which is a manager - let's call it KManager
- that manager is inside an independent module (moduleA). There is another module (moduleB) where I'm using KManager
.
Rule: moduleB knows about moduleA, but moduleA shouldn't know anything about moduleB.
I created like a bridge (interface) to communicate moduleA with moduleB, because of there is some shared logic that needs to run in both sides. The interface is declared in the KManager
as:
//moduleA
object KManager {
var bridgeShared: BridgeShared? = null
....
}
Interface:
interface BridgeShared {
fun foo()
}
Now, I have a class in the moduleB where implements BridgeShared
. When the app starts I'm initializing bridgeShared
like this:
//moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
....
}
I'm getting KManager.bridgeShared
in the moduleB and executing the interface's functions in another classes, something like:
KManager.bridgeShared.foo()
The question here is, is it harmful to have a listener declared inside an object
Kotlin class and grab it whenever I need it?
NOTE: No real names used here, just to describe the scenario.
UPDATE:
Extra Steps:
moduleA receives like an external event, it is processed and then the result is received by the "Observer" who subscribed to the BridgeShared
listener, that "Observer" is the moduleB but moduleA doesn't care about it (the rule).
moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
fun callAnotherFunction1() {
KManager.anotherFunction1()
}
}
moduleA
interface BridgeShared {
fun eventProcessed()
fun fetchedData()
}
object KManager {
var bridgeShared: BridgeShared? = null
fun anotherFunction1() {
//not-related to BridgeShared, called from outside
}
fun anotherFunction2() {
//not-related to BridgeShared, called from outside
}
}
class EventProcessor {
fun eventReceived() {
//stuff
KManager.bridgeShared?.eventProcessed()
}
}
class DataFetcher {
fun dataReceived() {
//stuff
KManager.bridgeShared?.fetchedData()
}
}
On the other hand, I'm taking advantage of KManager.bridgeShared
in the moduleB to call the same functions inside the same moduleB because I don't have access to the instance of BridgeSharedImpl
everywhere, just where it was instantiated.
Now, the moduleB uses the KManager
singleton to execute other functions not related to the BridgeShared.

|
show 6 more comments
I have an object
class in Kotlin which is a manager - let's call it KManager
- that manager is inside an independent module (moduleA). There is another module (moduleB) where I'm using KManager
.
Rule: moduleB knows about moduleA, but moduleA shouldn't know anything about moduleB.
I created like a bridge (interface) to communicate moduleA with moduleB, because of there is some shared logic that needs to run in both sides. The interface is declared in the KManager
as:
//moduleA
object KManager {
var bridgeShared: BridgeShared? = null
....
}
Interface:
interface BridgeShared {
fun foo()
}
Now, I have a class in the moduleB where implements BridgeShared
. When the app starts I'm initializing bridgeShared
like this:
//moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
....
}
I'm getting KManager.bridgeShared
in the moduleB and executing the interface's functions in another classes, something like:
KManager.bridgeShared.foo()
The question here is, is it harmful to have a listener declared inside an object
Kotlin class and grab it whenever I need it?
NOTE: No real names used here, just to describe the scenario.
UPDATE:
Extra Steps:
moduleA receives like an external event, it is processed and then the result is received by the "Observer" who subscribed to the BridgeShared
listener, that "Observer" is the moduleB but moduleA doesn't care about it (the rule).
moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
fun callAnotherFunction1() {
KManager.anotherFunction1()
}
}
moduleA
interface BridgeShared {
fun eventProcessed()
fun fetchedData()
}
object KManager {
var bridgeShared: BridgeShared? = null
fun anotherFunction1() {
//not-related to BridgeShared, called from outside
}
fun anotherFunction2() {
//not-related to BridgeShared, called from outside
}
}
class EventProcessor {
fun eventReceived() {
//stuff
KManager.bridgeShared?.eventProcessed()
}
}
class DataFetcher {
fun dataReceived() {
//stuff
KManager.bridgeShared?.fetchedData()
}
}
On the other hand, I'm taking advantage of KManager.bridgeShared
in the moduleB to call the same functions inside the same moduleB because I don't have access to the instance of BridgeSharedImpl
everywhere, just where it was instantiated.
Now, the moduleB uses the KManager
singleton to execute other functions not related to the BridgeShared.

It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located inmoduleB
?
– Andrey Ilyunin
Nov 20 '18 at 23:48
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
If you could provide some abstract steps (fake, of course) that are being performed in both sides (...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is thatmoduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations ofBridgeShared
could just be passed as a constructor parameter to an object inmoduleA
instead of contacting between modules via the singleton.
– Andrey Ilyunin
Nov 21 '18 at 3:14
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need theBridgeShared
in another places in the samemoduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passingListener
to a constructor ofEventProcessor
andDataFetcher
classes. If you need a one instance, you can just create anAbstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.
– Andrey Ilyunin
Nov 22 '18 at 8:17
|
show 6 more comments
I have an object
class in Kotlin which is a manager - let's call it KManager
- that manager is inside an independent module (moduleA). There is another module (moduleB) where I'm using KManager
.
Rule: moduleB knows about moduleA, but moduleA shouldn't know anything about moduleB.
I created like a bridge (interface) to communicate moduleA with moduleB, because of there is some shared logic that needs to run in both sides. The interface is declared in the KManager
as:
//moduleA
object KManager {
var bridgeShared: BridgeShared? = null
....
}
Interface:
interface BridgeShared {
fun foo()
}
Now, I have a class in the moduleB where implements BridgeShared
. When the app starts I'm initializing bridgeShared
like this:
//moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
....
}
I'm getting KManager.bridgeShared
in the moduleB and executing the interface's functions in another classes, something like:
KManager.bridgeShared.foo()
The question here is, is it harmful to have a listener declared inside an object
Kotlin class and grab it whenever I need it?
NOTE: No real names used here, just to describe the scenario.
UPDATE:
Extra Steps:
moduleA receives like an external event, it is processed and then the result is received by the "Observer" who subscribed to the BridgeShared
listener, that "Observer" is the moduleB but moduleA doesn't care about it (the rule).
moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
fun callAnotherFunction1() {
KManager.anotherFunction1()
}
}
moduleA
interface BridgeShared {
fun eventProcessed()
fun fetchedData()
}
object KManager {
var bridgeShared: BridgeShared? = null
fun anotherFunction1() {
//not-related to BridgeShared, called from outside
}
fun anotherFunction2() {
//not-related to BridgeShared, called from outside
}
}
class EventProcessor {
fun eventReceived() {
//stuff
KManager.bridgeShared?.eventProcessed()
}
}
class DataFetcher {
fun dataReceived() {
//stuff
KManager.bridgeShared?.fetchedData()
}
}
On the other hand, I'm taking advantage of KManager.bridgeShared
in the moduleB to call the same functions inside the same moduleB because I don't have access to the instance of BridgeSharedImpl
everywhere, just where it was instantiated.
Now, the moduleB uses the KManager
singleton to execute other functions not related to the BridgeShared.

I have an object
class in Kotlin which is a manager - let's call it KManager
- that manager is inside an independent module (moduleA). There is another module (moduleB) where I'm using KManager
.
Rule: moduleB knows about moduleA, but moduleA shouldn't know anything about moduleB.
I created like a bridge (interface) to communicate moduleA with moduleB, because of there is some shared logic that needs to run in both sides. The interface is declared in the KManager
as:
//moduleA
object KManager {
var bridgeShared: BridgeShared? = null
....
}
Interface:
interface BridgeShared {
fun foo()
}
Now, I have a class in the moduleB where implements BridgeShared
. When the app starts I'm initializing bridgeShared
like this:
//moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
....
}
I'm getting KManager.bridgeShared
in the moduleB and executing the interface's functions in another classes, something like:
KManager.bridgeShared.foo()
The question here is, is it harmful to have a listener declared inside an object
Kotlin class and grab it whenever I need it?
NOTE: No real names used here, just to describe the scenario.
UPDATE:
Extra Steps:
moduleA receives like an external event, it is processed and then the result is received by the "Observer" who subscribed to the BridgeShared
listener, that "Observer" is the moduleB but moduleA doesn't care about it (the rule).
moduleB
class BridgeSharedImpl : BridgeShared {
KManager.bridgeShared = this
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
fun callAnotherFunction1() {
KManager.anotherFunction1()
}
}
moduleA
interface BridgeShared {
fun eventProcessed()
fun fetchedData()
}
object KManager {
var bridgeShared: BridgeShared? = null
fun anotherFunction1() {
//not-related to BridgeShared, called from outside
}
fun anotherFunction2() {
//not-related to BridgeShared, called from outside
}
}
class EventProcessor {
fun eventReceived() {
//stuff
KManager.bridgeShared?.eventProcessed()
}
}
class DataFetcher {
fun dataReceived() {
//stuff
KManager.bridgeShared?.fetchedData()
}
}
On the other hand, I'm taking advantage of KManager.bridgeShared
in the moduleB to call the same functions inside the same moduleB because I don't have access to the instance of BridgeSharedImpl
everywhere, just where it was instantiated.
Now, the moduleB uses the KManager
singleton to execute other functions not related to the BridgeShared.


edited Nov 21 '18 at 17:07
Wilson Castiblanco
asked Nov 20 '18 at 23:24
Wilson CastiblancoWilson Castiblanco
4017
4017
It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located inmoduleB
?
– Andrey Ilyunin
Nov 20 '18 at 23:48
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
If you could provide some abstract steps (fake, of course) that are being performed in both sides (...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is thatmoduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations ofBridgeShared
could just be passed as a constructor parameter to an object inmoduleA
instead of contacting between modules via the singleton.
– Andrey Ilyunin
Nov 21 '18 at 3:14
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need theBridgeShared
in another places in the samemoduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passingListener
to a constructor ofEventProcessor
andDataFetcher
classes. If you need a one instance, you can just create anAbstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.
– Andrey Ilyunin
Nov 22 '18 at 8:17
|
show 6 more comments
It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located inmoduleB
?
– Andrey Ilyunin
Nov 20 '18 at 23:48
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
If you could provide some abstract steps (fake, of course) that are being performed in both sides (...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is thatmoduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations ofBridgeShared
could just be passed as a constructor parameter to an object inmoduleA
instead of contacting between modules via the singleton.
– Andrey Ilyunin
Nov 21 '18 at 3:14
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need theBridgeShared
in another places in the samemoduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passingListener
to a constructor ofEventProcessor
andDataFetcher
classes. If you need a one instance, you can just create anAbstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.
– Andrey Ilyunin
Nov 22 '18 at 8:17
It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located in
moduleB
?– Andrey Ilyunin
Nov 20 '18 at 23:48
It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located in
moduleB
?– Andrey Ilyunin
Nov 20 '18 at 23:48
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
If you could provide some abstract steps (fake, of course) that are being performed in both sides (
...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is that moduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations of BridgeShared
could just be passed as a constructor parameter to an object in moduleA
instead of contacting between modules via the singleton.– Andrey Ilyunin
Nov 21 '18 at 3:14
If you could provide some abstract steps (fake, of course) that are being performed in both sides (
...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is that moduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations of BridgeShared
could just be passed as a constructor parameter to an object in moduleA
instead of contacting between modules via the singleton.– Andrey Ilyunin
Nov 21 '18 at 3:14
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need the
BridgeShared
in another places in the same moduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need the
BridgeShared
in another places in the same moduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passing
Listener
to a constructor of EventProcessor
and DataFetcher
classes. If you need a one instance, you can just create an Abstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.– Andrey Ilyunin
Nov 22 '18 at 8:17
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passing
Listener
to a constructor of EventProcessor
and DataFetcher
classes. If you need a one instance, you can just create an Abstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.– Andrey Ilyunin
Nov 22 '18 at 8:17
|
show 6 more comments
1 Answer
1
active
oldest
votes
Based on the recommendations, I applied the Factory solution to avoid saving the BridgeShared
inside a Singleton.
moduleA
class BridgeFactory(private val bridgeShared: BridgeShared) {
companion object {
lateinit var bridgeFactory: BridgeFactory
fun initialize(bridgeShared: BridgeShared) {
bridgeFactory = BridgeFactory(emmaShared)
}
}
fun eventProcessor(): EventProcessor {
return EventProcessor(bridgeShared)
}
fun dataFetcher(): DataFetcher {
return DataFetcher(bridgeShared)
}
}
class EventProcessor(private val bridgeShared: BridgeShared) {
fun eventReceived() {
//stuff
bridgeShared.eventProcessed()
}
}
class DataFetcher(private val bridgeShared: BridgeShared) {
fun dataReceived() {
//stuff
bridgeShared.fetchedData()
}
}
moduleB
class BridgeSharedImpl : BridgeShared {
init {
BridgeFactory.initialize(this)
}
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403128%2flistener-declared-in-a-kotlin-object-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Based on the recommendations, I applied the Factory solution to avoid saving the BridgeShared
inside a Singleton.
moduleA
class BridgeFactory(private val bridgeShared: BridgeShared) {
companion object {
lateinit var bridgeFactory: BridgeFactory
fun initialize(bridgeShared: BridgeShared) {
bridgeFactory = BridgeFactory(emmaShared)
}
}
fun eventProcessor(): EventProcessor {
return EventProcessor(bridgeShared)
}
fun dataFetcher(): DataFetcher {
return DataFetcher(bridgeShared)
}
}
class EventProcessor(private val bridgeShared: BridgeShared) {
fun eventReceived() {
//stuff
bridgeShared.eventProcessed()
}
}
class DataFetcher(private val bridgeShared: BridgeShared) {
fun dataReceived() {
//stuff
bridgeShared.fetchedData()
}
}
moduleB
class BridgeSharedImpl : BridgeShared {
init {
BridgeFactory.initialize(this)
}
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
}
add a comment |
Based on the recommendations, I applied the Factory solution to avoid saving the BridgeShared
inside a Singleton.
moduleA
class BridgeFactory(private val bridgeShared: BridgeShared) {
companion object {
lateinit var bridgeFactory: BridgeFactory
fun initialize(bridgeShared: BridgeShared) {
bridgeFactory = BridgeFactory(emmaShared)
}
}
fun eventProcessor(): EventProcessor {
return EventProcessor(bridgeShared)
}
fun dataFetcher(): DataFetcher {
return DataFetcher(bridgeShared)
}
}
class EventProcessor(private val bridgeShared: BridgeShared) {
fun eventReceived() {
//stuff
bridgeShared.eventProcessed()
}
}
class DataFetcher(private val bridgeShared: BridgeShared) {
fun dataReceived() {
//stuff
bridgeShared.fetchedData()
}
}
moduleB
class BridgeSharedImpl : BridgeShared {
init {
BridgeFactory.initialize(this)
}
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
}
add a comment |
Based on the recommendations, I applied the Factory solution to avoid saving the BridgeShared
inside a Singleton.
moduleA
class BridgeFactory(private val bridgeShared: BridgeShared) {
companion object {
lateinit var bridgeFactory: BridgeFactory
fun initialize(bridgeShared: BridgeShared) {
bridgeFactory = BridgeFactory(emmaShared)
}
}
fun eventProcessor(): EventProcessor {
return EventProcessor(bridgeShared)
}
fun dataFetcher(): DataFetcher {
return DataFetcher(bridgeShared)
}
}
class EventProcessor(private val bridgeShared: BridgeShared) {
fun eventReceived() {
//stuff
bridgeShared.eventProcessed()
}
}
class DataFetcher(private val bridgeShared: BridgeShared) {
fun dataReceived() {
//stuff
bridgeShared.fetchedData()
}
}
moduleB
class BridgeSharedImpl : BridgeShared {
init {
BridgeFactory.initialize(this)
}
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
}
Based on the recommendations, I applied the Factory solution to avoid saving the BridgeShared
inside a Singleton.
moduleA
class BridgeFactory(private val bridgeShared: BridgeShared) {
companion object {
lateinit var bridgeFactory: BridgeFactory
fun initialize(bridgeShared: BridgeShared) {
bridgeFactory = BridgeFactory(emmaShared)
}
}
fun eventProcessor(): EventProcessor {
return EventProcessor(bridgeShared)
}
fun dataFetcher(): DataFetcher {
return DataFetcher(bridgeShared)
}
}
class EventProcessor(private val bridgeShared: BridgeShared) {
fun eventReceived() {
//stuff
bridgeShared.eventProcessed()
}
}
class DataFetcher(private val bridgeShared: BridgeShared) {
fun dataReceived() {
//stuff
bridgeShared.fetchedData()
}
}
moduleB
class BridgeSharedImpl : BridgeShared {
init {
BridgeFactory.initialize(this)
}
override fun eventProcessed() {
//stuff
}
override fun fetchData() {
//stuff
}
}
answered Nov 23 '18 at 21:15
Wilson CastiblancoWilson Castiblanco
4017
4017
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403128%2flistener-declared-in-a-kotlin-object-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
l w8Dzb,6lJse6KATfv3m0c1IVUqNkhyHmq
It is OOD question and it will, no doubt, spawn a lot of controversial answers. Here is a dangerous line between objective and subjective... Also, details are too opaque to offer a single win-win solution. Surely, this architecutre looks dreadfully. I mean, singleton mutable object to serve as a mediator? Haven't you consider insantiating an object that knows how to perform business process and relies on abstractions to delegate or ask for some functionality, whereas concrete implementors of these interfaces will be located in
moduleB
?– Andrey Ilyunin
Nov 20 '18 at 23:48
@AndreyIlyunin yeah .. I don't feel good with this. is the object that you propose like BridgeSharedImpl, what kind of details can I give you to clarify the implementation?
– Wilson Castiblanco
Nov 21 '18 at 0:33
If you could provide some abstract steps (fake, of course) that are being performed in both sides (
...because of there is some shared logic that needs to run in both sides
), it would be cool. What I see now is thatmoduleA
uses a singleton object so obtain an object that should be used to execute a part of the buisness-logic flow. Then why the singleton is needed? If I understood correctly, then specific implementations ofBridgeShared
could just be passed as a constructor parameter to an object inmoduleA
instead of contacting between modules via the singleton.– Andrey Ilyunin
Nov 21 '18 at 3:14
@AndreyIlyunin just added an update. (... then specific implementations of BridgeShared could just be passed as a constructor parameter to an object in moduleA... ) .. got it, but I need the
BridgeShared
in another places in the samemoduleA
– Wilson Castiblanco
Nov 21 '18 at 17:11
It's extremely complicated architecture... First thing I would recommend is to eliminate "singleton access". The most lightweight thing that can be done is, sure, passing
Listener
to a constructor ofEventProcessor
andDataFetcher
classes. If you need a one instance, you can just create anAbstract Factory
that will take singleton instance and will pass it to the constructors of mentioned classes. Thus, unwanted coupling and dependency will be removed from these classes.– Andrey Ilyunin
Nov 22 '18 at 8:17