JPA - Count by Status of Admission Form (Status Type Enum)
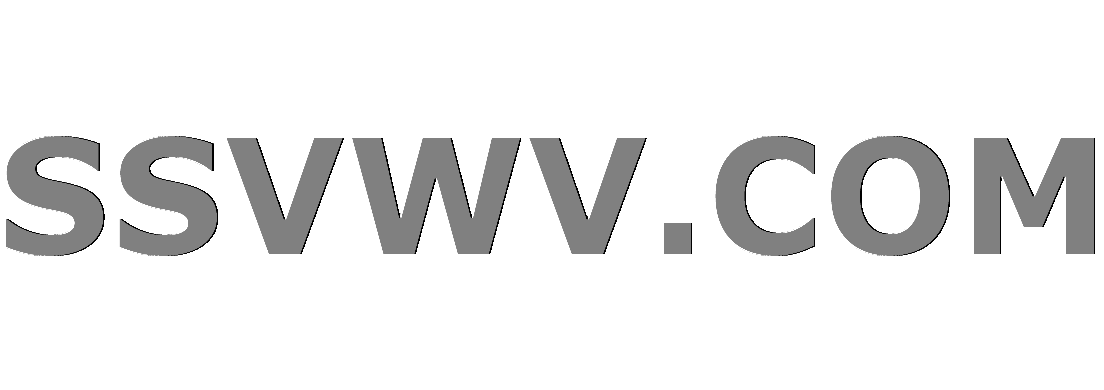
Multi tool use
I have declared an Enum
type StatusEnum as like:
public enum StatusEnum {
PENDING, ACCEPTED, REJECTED, IN_PROGRESS, COMPLETED
}
I am trying to get count of Admission form from their status, so i tried with query (in Repository):
@Query("SELECT COUNT(a.status), a.status FROM AdmissionForms a GROUP BY a.status ORDER BY a.status ASC")
List<Object> admissionFormCountByStatus();
then i parsed it to List of DTO that I have specified (in service class),
List<Object> objects = admissionFormRepository.admissionFormCountByStatus();
for (Object singleObject : objects ) {
admissionFormStatusCountList.add(new AdmissionFormStatusCountResponseDTO(
((Number)singleObject[0]).intValue(), (StatusEnum)singleObject[1]));
}
my DTO (AdmissionFormStatusCountResponseDTO)like:
public class AdmissionFormStatusCountResponseDTO {
private int count;
private StatusEnum status;
public AdmissionFormStatusCountResponseDTO(int count, StatusEnum status) {
this.count = count;
this.status = status;
}
}
resource class:
@GetMapping("/workorders/countByStatus")
@Timed
public List<AdmissionFormStatusCountResponseDTO> admissionFormCountByStatus() {
return admissionFormService.workorderCountByStatus();
}
results that I'm having is like:
[
{
"count": 6,
"status": "ACCEPTED"
},
{
"count": 2,
"status": "COMPLETED"
},
{
"count": 3,
"status": "IN_PROGRESS"
}
]
It's responding with count only if that status value is present but I also require count = 0 in result for status from Enum not assigned to any admission form yet.
java spring-boot jpa enums spring-data-jpa
add a comment |
I have declared an Enum
type StatusEnum as like:
public enum StatusEnum {
PENDING, ACCEPTED, REJECTED, IN_PROGRESS, COMPLETED
}
I am trying to get count of Admission form from their status, so i tried with query (in Repository):
@Query("SELECT COUNT(a.status), a.status FROM AdmissionForms a GROUP BY a.status ORDER BY a.status ASC")
List<Object> admissionFormCountByStatus();
then i parsed it to List of DTO that I have specified (in service class),
List<Object> objects = admissionFormRepository.admissionFormCountByStatus();
for (Object singleObject : objects ) {
admissionFormStatusCountList.add(new AdmissionFormStatusCountResponseDTO(
((Number)singleObject[0]).intValue(), (StatusEnum)singleObject[1]));
}
my DTO (AdmissionFormStatusCountResponseDTO)like:
public class AdmissionFormStatusCountResponseDTO {
private int count;
private StatusEnum status;
public AdmissionFormStatusCountResponseDTO(int count, StatusEnum status) {
this.count = count;
this.status = status;
}
}
resource class:
@GetMapping("/workorders/countByStatus")
@Timed
public List<AdmissionFormStatusCountResponseDTO> admissionFormCountByStatus() {
return admissionFormService.workorderCountByStatus();
}
results that I'm having is like:
[
{
"count": 6,
"status": "ACCEPTED"
},
{
"count": 2,
"status": "COMPLETED"
},
{
"count": 3,
"status": "IN_PROGRESS"
}
]
It's responding with count only if that status value is present but I also require count = 0 in result for status from Enum not assigned to any admission form yet.
java spring-boot jpa enums spring-data-jpa
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37
add a comment |
I have declared an Enum
type StatusEnum as like:
public enum StatusEnum {
PENDING, ACCEPTED, REJECTED, IN_PROGRESS, COMPLETED
}
I am trying to get count of Admission form from their status, so i tried with query (in Repository):
@Query("SELECT COUNT(a.status), a.status FROM AdmissionForms a GROUP BY a.status ORDER BY a.status ASC")
List<Object> admissionFormCountByStatus();
then i parsed it to List of DTO that I have specified (in service class),
List<Object> objects = admissionFormRepository.admissionFormCountByStatus();
for (Object singleObject : objects ) {
admissionFormStatusCountList.add(new AdmissionFormStatusCountResponseDTO(
((Number)singleObject[0]).intValue(), (StatusEnum)singleObject[1]));
}
my DTO (AdmissionFormStatusCountResponseDTO)like:
public class AdmissionFormStatusCountResponseDTO {
private int count;
private StatusEnum status;
public AdmissionFormStatusCountResponseDTO(int count, StatusEnum status) {
this.count = count;
this.status = status;
}
}
resource class:
@GetMapping("/workorders/countByStatus")
@Timed
public List<AdmissionFormStatusCountResponseDTO> admissionFormCountByStatus() {
return admissionFormService.workorderCountByStatus();
}
results that I'm having is like:
[
{
"count": 6,
"status": "ACCEPTED"
},
{
"count": 2,
"status": "COMPLETED"
},
{
"count": 3,
"status": "IN_PROGRESS"
}
]
It's responding with count only if that status value is present but I also require count = 0 in result for status from Enum not assigned to any admission form yet.
java spring-boot jpa enums spring-data-jpa
I have declared an Enum
type StatusEnum as like:
public enum StatusEnum {
PENDING, ACCEPTED, REJECTED, IN_PROGRESS, COMPLETED
}
I am trying to get count of Admission form from their status, so i tried with query (in Repository):
@Query("SELECT COUNT(a.status), a.status FROM AdmissionForms a GROUP BY a.status ORDER BY a.status ASC")
List<Object> admissionFormCountByStatus();
then i parsed it to List of DTO that I have specified (in service class),
List<Object> objects = admissionFormRepository.admissionFormCountByStatus();
for (Object singleObject : objects ) {
admissionFormStatusCountList.add(new AdmissionFormStatusCountResponseDTO(
((Number)singleObject[0]).intValue(), (StatusEnum)singleObject[1]));
}
my DTO (AdmissionFormStatusCountResponseDTO)like:
public class AdmissionFormStatusCountResponseDTO {
private int count;
private StatusEnum status;
public AdmissionFormStatusCountResponseDTO(int count, StatusEnum status) {
this.count = count;
this.status = status;
}
}
resource class:
@GetMapping("/workorders/countByStatus")
@Timed
public List<AdmissionFormStatusCountResponseDTO> admissionFormCountByStatus() {
return admissionFormService.workorderCountByStatus();
}
results that I'm having is like:
[
{
"count": 6,
"status": "ACCEPTED"
},
{
"count": 2,
"status": "COMPLETED"
},
{
"count": 3,
"status": "IN_PROGRESS"
}
]
It's responding with count only if that status value is present but I also require count = 0 in result for status from Enum not assigned to any admission form yet.
java spring-boot jpa enums spring-data-jpa
java spring-boot jpa enums spring-data-jpa
edited Nov 23 '18 at 12:36
Robert Niestroj
7,99074581
7,99074581
asked Nov 23 '18 at 12:23


Purvik RanaPurvik Rana
9817
9817
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37
add a comment |
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37
add a comment |
2 Answers
2
active
oldest
votes
After preparing your admissionFormStatusCountList
look manually which enums are missing in there and add them with value 0. You are not gonna get it out of the DB.
add a comment |
I did the changes as below that i required.
I defined the admissionFormStatusCountResponseDTO
like:
public class AdmissionFormStatusCountResponseDTO {
private Long accepted;
private Long completed;
private Long inProgress;
private Long pending;
private Long rejected;
public AdmissionFormStatusCountResponseDTO() {
}
public AdmissionFormStatusCountResponseDTO(Long accepted, Long completed, Long inProgress, Long pending, Long rejected) {
this.accepted = accepted;
this.completed = completed;
this.inProgress = inProgress;
this.pending = pending;
this.rejected = rejected;
}
//getters and setters
}
then update repository query using this DTO as:
@Query("select new com.purvik.app1.service.dto.AdmissionFormStatusCountResponseDTO(" +
"SUM(CASE WHEN a.status='ACCEPTED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='COMPLETED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='IN_PROGRESS' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='PENDING' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='REJECTED' then 1 else 0 END)" +
") from AdmissionForm a")
AdmissionFormStatusCountResponseDTO admissionFormCountByStatusNew();
Service class:
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus(){
log.debug("Request to get count of AdmissionForm by it's Status ");
return admissionFormRepository.admissionFormCountByStatusNew();
}
Resource endpoint:
@GetMapping("/admissionForms/countByStatus")
@Timed
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus() {
log.debug("get count of status from stored admissionForm");
return admissionFormService.admissionFormCountByStatus();
}
response that now I've:
{
"accepted": 5,
"completed": 0,
"inProgress": 4,
"pending": 1,
"rejected": 1
}
I do not require to have all the statics defined in Enum
. It's a constructor based JPQL query that bring expected results. Hope this will help others.
@Robert Niestroj is right if you want to have all the statics in response defined in your Enum
. Just editing my code that help me out.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446676%2fjpa-count-by-status-of-admission-form-status-type-enum%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
After preparing your admissionFormStatusCountList
look manually which enums are missing in there and add them with value 0. You are not gonna get it out of the DB.
add a comment |
After preparing your admissionFormStatusCountList
look manually which enums are missing in there and add them with value 0. You are not gonna get it out of the DB.
add a comment |
After preparing your admissionFormStatusCountList
look manually which enums are missing in there and add them with value 0. You are not gonna get it out of the DB.
After preparing your admissionFormStatusCountList
look manually which enums are missing in there and add them with value 0. You are not gonna get it out of the DB.
answered Nov 23 '18 at 12:39
Robert NiestrojRobert Niestroj
7,99074581
7,99074581
add a comment |
add a comment |
I did the changes as below that i required.
I defined the admissionFormStatusCountResponseDTO
like:
public class AdmissionFormStatusCountResponseDTO {
private Long accepted;
private Long completed;
private Long inProgress;
private Long pending;
private Long rejected;
public AdmissionFormStatusCountResponseDTO() {
}
public AdmissionFormStatusCountResponseDTO(Long accepted, Long completed, Long inProgress, Long pending, Long rejected) {
this.accepted = accepted;
this.completed = completed;
this.inProgress = inProgress;
this.pending = pending;
this.rejected = rejected;
}
//getters and setters
}
then update repository query using this DTO as:
@Query("select new com.purvik.app1.service.dto.AdmissionFormStatusCountResponseDTO(" +
"SUM(CASE WHEN a.status='ACCEPTED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='COMPLETED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='IN_PROGRESS' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='PENDING' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='REJECTED' then 1 else 0 END)" +
") from AdmissionForm a")
AdmissionFormStatusCountResponseDTO admissionFormCountByStatusNew();
Service class:
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus(){
log.debug("Request to get count of AdmissionForm by it's Status ");
return admissionFormRepository.admissionFormCountByStatusNew();
}
Resource endpoint:
@GetMapping("/admissionForms/countByStatus")
@Timed
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus() {
log.debug("get count of status from stored admissionForm");
return admissionFormService.admissionFormCountByStatus();
}
response that now I've:
{
"accepted": 5,
"completed": 0,
"inProgress": 4,
"pending": 1,
"rejected": 1
}
I do not require to have all the statics defined in Enum
. It's a constructor based JPQL query that bring expected results. Hope this will help others.
@Robert Niestroj is right if you want to have all the statics in response defined in your Enum
. Just editing my code that help me out.
add a comment |
I did the changes as below that i required.
I defined the admissionFormStatusCountResponseDTO
like:
public class AdmissionFormStatusCountResponseDTO {
private Long accepted;
private Long completed;
private Long inProgress;
private Long pending;
private Long rejected;
public AdmissionFormStatusCountResponseDTO() {
}
public AdmissionFormStatusCountResponseDTO(Long accepted, Long completed, Long inProgress, Long pending, Long rejected) {
this.accepted = accepted;
this.completed = completed;
this.inProgress = inProgress;
this.pending = pending;
this.rejected = rejected;
}
//getters and setters
}
then update repository query using this DTO as:
@Query("select new com.purvik.app1.service.dto.AdmissionFormStatusCountResponseDTO(" +
"SUM(CASE WHEN a.status='ACCEPTED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='COMPLETED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='IN_PROGRESS' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='PENDING' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='REJECTED' then 1 else 0 END)" +
") from AdmissionForm a")
AdmissionFormStatusCountResponseDTO admissionFormCountByStatusNew();
Service class:
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus(){
log.debug("Request to get count of AdmissionForm by it's Status ");
return admissionFormRepository.admissionFormCountByStatusNew();
}
Resource endpoint:
@GetMapping("/admissionForms/countByStatus")
@Timed
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus() {
log.debug("get count of status from stored admissionForm");
return admissionFormService.admissionFormCountByStatus();
}
response that now I've:
{
"accepted": 5,
"completed": 0,
"inProgress": 4,
"pending": 1,
"rejected": 1
}
I do not require to have all the statics defined in Enum
. It's a constructor based JPQL query that bring expected results. Hope this will help others.
@Robert Niestroj is right if you want to have all the statics in response defined in your Enum
. Just editing my code that help me out.
add a comment |
I did the changes as below that i required.
I defined the admissionFormStatusCountResponseDTO
like:
public class AdmissionFormStatusCountResponseDTO {
private Long accepted;
private Long completed;
private Long inProgress;
private Long pending;
private Long rejected;
public AdmissionFormStatusCountResponseDTO() {
}
public AdmissionFormStatusCountResponseDTO(Long accepted, Long completed, Long inProgress, Long pending, Long rejected) {
this.accepted = accepted;
this.completed = completed;
this.inProgress = inProgress;
this.pending = pending;
this.rejected = rejected;
}
//getters and setters
}
then update repository query using this DTO as:
@Query("select new com.purvik.app1.service.dto.AdmissionFormStatusCountResponseDTO(" +
"SUM(CASE WHEN a.status='ACCEPTED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='COMPLETED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='IN_PROGRESS' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='PENDING' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='REJECTED' then 1 else 0 END)" +
") from AdmissionForm a")
AdmissionFormStatusCountResponseDTO admissionFormCountByStatusNew();
Service class:
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus(){
log.debug("Request to get count of AdmissionForm by it's Status ");
return admissionFormRepository.admissionFormCountByStatusNew();
}
Resource endpoint:
@GetMapping("/admissionForms/countByStatus")
@Timed
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus() {
log.debug("get count of status from stored admissionForm");
return admissionFormService.admissionFormCountByStatus();
}
response that now I've:
{
"accepted": 5,
"completed": 0,
"inProgress": 4,
"pending": 1,
"rejected": 1
}
I do not require to have all the statics defined in Enum
. It's a constructor based JPQL query that bring expected results. Hope this will help others.
@Robert Niestroj is right if you want to have all the statics in response defined in your Enum
. Just editing my code that help me out.
I did the changes as below that i required.
I defined the admissionFormStatusCountResponseDTO
like:
public class AdmissionFormStatusCountResponseDTO {
private Long accepted;
private Long completed;
private Long inProgress;
private Long pending;
private Long rejected;
public AdmissionFormStatusCountResponseDTO() {
}
public AdmissionFormStatusCountResponseDTO(Long accepted, Long completed, Long inProgress, Long pending, Long rejected) {
this.accepted = accepted;
this.completed = completed;
this.inProgress = inProgress;
this.pending = pending;
this.rejected = rejected;
}
//getters and setters
}
then update repository query using this DTO as:
@Query("select new com.purvik.app1.service.dto.AdmissionFormStatusCountResponseDTO(" +
"SUM(CASE WHEN a.status='ACCEPTED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='COMPLETED' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='IN_PROGRESS' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='PENDING' then 1 else 0 END)," +
"SUM(CASE WHEN a.status='REJECTED' then 1 else 0 END)" +
") from AdmissionForm a")
AdmissionFormStatusCountResponseDTO admissionFormCountByStatusNew();
Service class:
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus(){
log.debug("Request to get count of AdmissionForm by it's Status ");
return admissionFormRepository.admissionFormCountByStatusNew();
}
Resource endpoint:
@GetMapping("/admissionForms/countByStatus")
@Timed
public AdmissionFormStatusCountResponseDTO admissionFormCountByStatus() {
log.debug("get count of status from stored admissionForm");
return admissionFormService.admissionFormCountByStatus();
}
response that now I've:
{
"accepted": 5,
"completed": 0,
"inProgress": 4,
"pending": 1,
"rejected": 1
}
I do not require to have all the statics defined in Enum
. It's a constructor based JPQL query that bring expected results. Hope this will help others.
@Robert Niestroj is right if you want to have all the statics in response defined in your Enum
. Just editing my code that help me out.
answered Nov 26 '18 at 8:06


Purvik RanaPurvik Rana
9817
9817
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446676%2fjpa-count-by-status-of-admission-form-status-type-enum%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
E,PFhqjoI5,YxnP B6X,dxCUs,EFOR,c0 vbJsxyq76uSoA D6RmJ,Ix,8GcviO1m qTg eRQ38h,8F5A1KPNQ qy8fTJ2zK,Y BW
You would need a table with all possible status values to be able to get that out of the DB.
– K.Nicholas
Nov 23 '18 at 15:37