onKeyDown() event not triggered when in DialogFragment
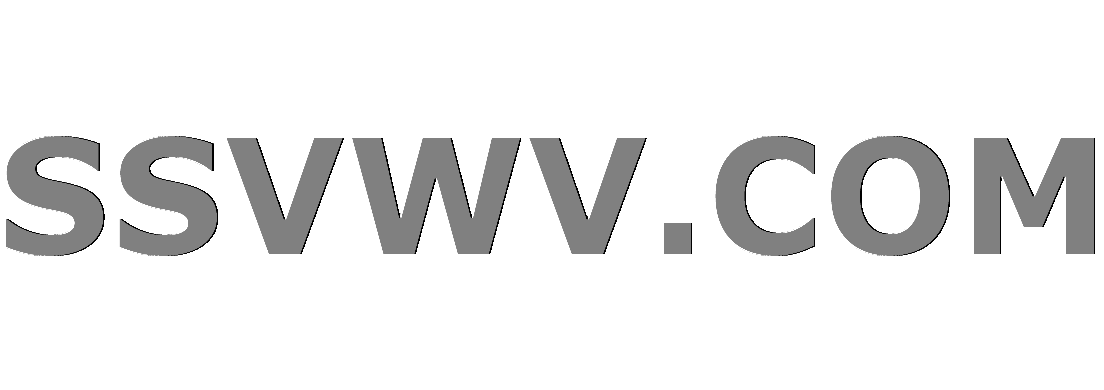
Multi tool use
I capture Key Events (from an external keyboard) within my App. I use onKeyDown()
method from Activity
. In my app I switch between different Fragments. If I am in a normal Fragment
then Activity's onKeyDown()
is triggered when pressing buttons. But when I use a DialogFragment
as a Dialog then pressing the button does not trigger Activity's
onKeyDown()` any more.
Here some sample code:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun onClick(view: View) {
// a) Key Event works if adding it via a fragment transaction by my own
// val fragment = MyDialogFragment.newInstance()
// val fragmentTransaction = supportFragmentManager.beginTransaction()
// fragmentTransaction.add(R.id.fr_container, fragment, fragment.javaClass.name)
// fragmentTransaction.commit()
// b) Key Event doesn't work if showing as a dialog
val fragment = MyDialogFragment.newInstance()
fragment.show(supportFragmentManager, fragment.javaClass.name)
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
Log.i(javaClass.name, "onKeyDown() keyCode: $keyCode")
return true
}
}
And my two fragments:
class MyNormalFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_normal, container, false)
}
}
class MyDialogFragment : DialogFragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
As soon as I call a) show()
to open the MyDialogFragment
then the key events are not captured any more. But if I open MyDialogFragment
b) via custom Fragments transaction then the key events are still captured, but my Fragment isn't shown as a Dialog any more.
What do I have to do to let the event also trigger when my dialog is displayed?



add a comment |
I capture Key Events (from an external keyboard) within my App. I use onKeyDown()
method from Activity
. In my app I switch between different Fragments. If I am in a normal Fragment
then Activity's onKeyDown()
is triggered when pressing buttons. But when I use a DialogFragment
as a Dialog then pressing the button does not trigger Activity's
onKeyDown()` any more.
Here some sample code:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun onClick(view: View) {
// a) Key Event works if adding it via a fragment transaction by my own
// val fragment = MyDialogFragment.newInstance()
// val fragmentTransaction = supportFragmentManager.beginTransaction()
// fragmentTransaction.add(R.id.fr_container, fragment, fragment.javaClass.name)
// fragmentTransaction.commit()
// b) Key Event doesn't work if showing as a dialog
val fragment = MyDialogFragment.newInstance()
fragment.show(supportFragmentManager, fragment.javaClass.name)
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
Log.i(javaClass.name, "onKeyDown() keyCode: $keyCode")
return true
}
}
And my two fragments:
class MyNormalFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_normal, container, false)
}
}
class MyDialogFragment : DialogFragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
As soon as I call a) show()
to open the MyDialogFragment
then the key events are not captured any more. But if I open MyDialogFragment
b) via custom Fragments transaction then the key events are still captured, but my Fragment isn't shown as a Dialog any more.
What do I have to do to let the event also trigger when my dialog is displayed?



add a comment |
I capture Key Events (from an external keyboard) within my App. I use onKeyDown()
method from Activity
. In my app I switch between different Fragments. If I am in a normal Fragment
then Activity's onKeyDown()
is triggered when pressing buttons. But when I use a DialogFragment
as a Dialog then pressing the button does not trigger Activity's
onKeyDown()` any more.
Here some sample code:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun onClick(view: View) {
// a) Key Event works if adding it via a fragment transaction by my own
// val fragment = MyDialogFragment.newInstance()
// val fragmentTransaction = supportFragmentManager.beginTransaction()
// fragmentTransaction.add(R.id.fr_container, fragment, fragment.javaClass.name)
// fragmentTransaction.commit()
// b) Key Event doesn't work if showing as a dialog
val fragment = MyDialogFragment.newInstance()
fragment.show(supportFragmentManager, fragment.javaClass.name)
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
Log.i(javaClass.name, "onKeyDown() keyCode: $keyCode")
return true
}
}
And my two fragments:
class MyNormalFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_normal, container, false)
}
}
class MyDialogFragment : DialogFragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
As soon as I call a) show()
to open the MyDialogFragment
then the key events are not captured any more. But if I open MyDialogFragment
b) via custom Fragments transaction then the key events are still captured, but my Fragment isn't shown as a Dialog any more.
What do I have to do to let the event also trigger when my dialog is displayed?



I capture Key Events (from an external keyboard) within my App. I use onKeyDown()
method from Activity
. In my app I switch between different Fragments. If I am in a normal Fragment
then Activity's onKeyDown()
is triggered when pressing buttons. But when I use a DialogFragment
as a Dialog then pressing the button does not trigger Activity's
onKeyDown()` any more.
Here some sample code:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun onClick(view: View) {
// a) Key Event works if adding it via a fragment transaction by my own
// val fragment = MyDialogFragment.newInstance()
// val fragmentTransaction = supportFragmentManager.beginTransaction()
// fragmentTransaction.add(R.id.fr_container, fragment, fragment.javaClass.name)
// fragmentTransaction.commit()
// b) Key Event doesn't work if showing as a dialog
val fragment = MyDialogFragment.newInstance()
fragment.show(supportFragmentManager, fragment.javaClass.name)
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
Log.i(javaClass.name, "onKeyDown() keyCode: $keyCode")
return true
}
}
And my two fragments:
class MyNormalFragment : Fragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_normal, container, false)
}
}
class MyDialogFragment : DialogFragment() {
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
As soon as I call a) show()
to open the MyDialogFragment
then the key events are not captured any more. But if I open MyDialogFragment
b) via custom Fragments transaction then the key events are still captured, but my Fragment isn't shown as a Dialog any more.
What do I have to do to let the event also trigger when my dialog is displayed?






edited Nov 23 '18 at 12:49
Nikos Hidalgo
1,2532418
1,2532418
asked Nov 23 '18 at 12:37
unlimited101unlimited101
1,33131330
1,33131330
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
A Dialog is shown in/as a separate Window, so your Activity doesn't have the focus for keypresses any more. However, the Dialog has it's own onKeyDown method, so you can make use of that.
add a comment |
Ridcully's answer is right. I just wanted to post what I changed inside MyDialogFragment
to keep on capturing key events:
class MyDialogFragment : DialogFragment() {
private val keyEventListener = DialogInterface.OnKeyListener { dialog, keyCode, event ->
Log.i(javaClass.name, "onKey() keyCode: $keyCode")
true
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
dialog.setOnKeyListener(keyEventListener)
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
override fun onDestroyView() {
dialog.setOnKeyListener(null)
super.onDestroyView()
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446852%2fonkeydown-event-not-triggered-when-in-dialogfragment%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
A Dialog is shown in/as a separate Window, so your Activity doesn't have the focus for keypresses any more. However, the Dialog has it's own onKeyDown method, so you can make use of that.
add a comment |
A Dialog is shown in/as a separate Window, so your Activity doesn't have the focus for keypresses any more. However, the Dialog has it's own onKeyDown method, so you can make use of that.
add a comment |
A Dialog is shown in/as a separate Window, so your Activity doesn't have the focus for keypresses any more. However, the Dialog has it's own onKeyDown method, so you can make use of that.
A Dialog is shown in/as a separate Window, so your Activity doesn't have the focus for keypresses any more. However, the Dialog has it's own onKeyDown method, so you can make use of that.
answered Nov 23 '18 at 12:59
RidcullyRidcully
18.8k75471
18.8k75471
add a comment |
add a comment |
Ridcully's answer is right. I just wanted to post what I changed inside MyDialogFragment
to keep on capturing key events:
class MyDialogFragment : DialogFragment() {
private val keyEventListener = DialogInterface.OnKeyListener { dialog, keyCode, event ->
Log.i(javaClass.name, "onKey() keyCode: $keyCode")
true
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
dialog.setOnKeyListener(keyEventListener)
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
override fun onDestroyView() {
dialog.setOnKeyListener(null)
super.onDestroyView()
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
add a comment |
Ridcully's answer is right. I just wanted to post what I changed inside MyDialogFragment
to keep on capturing key events:
class MyDialogFragment : DialogFragment() {
private val keyEventListener = DialogInterface.OnKeyListener { dialog, keyCode, event ->
Log.i(javaClass.name, "onKey() keyCode: $keyCode")
true
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
dialog.setOnKeyListener(keyEventListener)
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
override fun onDestroyView() {
dialog.setOnKeyListener(null)
super.onDestroyView()
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
add a comment |
Ridcully's answer is right. I just wanted to post what I changed inside MyDialogFragment
to keep on capturing key events:
class MyDialogFragment : DialogFragment() {
private val keyEventListener = DialogInterface.OnKeyListener { dialog, keyCode, event ->
Log.i(javaClass.name, "onKey() keyCode: $keyCode")
true
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
dialog.setOnKeyListener(keyEventListener)
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
override fun onDestroyView() {
dialog.setOnKeyListener(null)
super.onDestroyView()
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
Ridcully's answer is right. I just wanted to post what I changed inside MyDialogFragment
to keep on capturing key events:
class MyDialogFragment : DialogFragment() {
private val keyEventListener = DialogInterface.OnKeyListener { dialog, keyCode, event ->
Log.i(javaClass.name, "onKey() keyCode: $keyCode")
true
}
override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? {
dialog.setOnKeyListener(keyEventListener)
return inflater.inflate(R.layout.fragment_my_dialog, container, false)
}
override fun onDestroyView() {
dialog.setOnKeyListener(null)
super.onDestroyView()
}
companion object {
fun newInstance() = MyDialogFragment()
}
}
answered Nov 23 '18 at 15:14
unlimited101unlimited101
1,33131330
1,33131330
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446852%2fonkeydown-event-not-triggered-when-in-dialogfragment%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yRG8A4h,OUfiEjEuRGnPEwHyd6wQX7rJw,9qUOnmEMamPv7m5KIRAt