Python show corresponding profile upon hovering/clicking over a grid
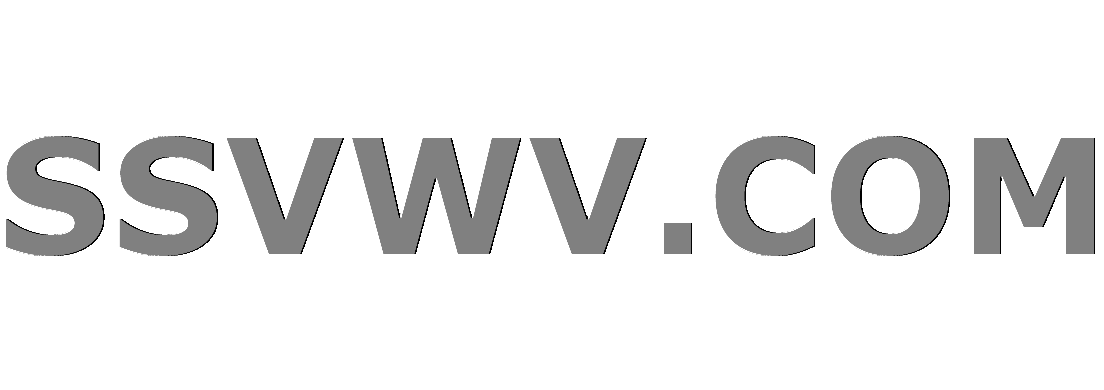
Multi tool use
I have a 2D array value_1
which depends on lon
(longitude) and lat
(latitude).
Now, I can use pcolormesh
to plot the value on one figure.
But, I have another 3D array value_2
which depends on lon
, lat
and pressure
(levels of pressure).
If I want to show the profile (depends on value_2
and pressure
) and coordinate like this: (-120,20)
when the mouse hover or click on one grid(lon,lat), how could I make it?
Here's the example of plotting pseudocolor plot
and profile plot
:
import numpy as np
import matplotlib.pyplot as plt
# coordination
lon = np.arange(-120,-110,1)
lat = np.arange(20,30,1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9,9)
pressure = np.arange(1110,500,-100)
lon,lat = np.meshgrid(lon,lat)
plt.pcolormesh(lon,lat,value_1)
plt.colorbar()
plt.show()
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9,9,pressure.shape[0])
python matplotlib
add a comment |
I have a 2D array value_1
which depends on lon
(longitude) and lat
(latitude).
Now, I can use pcolormesh
to plot the value on one figure.
But, I have another 3D array value_2
which depends on lon
, lat
and pressure
(levels of pressure).
If I want to show the profile (depends on value_2
and pressure
) and coordinate like this: (-120,20)
when the mouse hover or click on one grid(lon,lat), how could I make it?
Here's the example of plotting pseudocolor plot
and profile plot
:
import numpy as np
import matplotlib.pyplot as plt
# coordination
lon = np.arange(-120,-110,1)
lat = np.arange(20,30,1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9,9)
pressure = np.arange(1110,500,-100)
lon,lat = np.meshgrid(lon,lat)
plt.pcolormesh(lon,lat,value_1)
plt.colorbar()
plt.show()
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9,9,pressure.shape[0])
python matplotlib
add a comment |
I have a 2D array value_1
which depends on lon
(longitude) and lat
(latitude).
Now, I can use pcolormesh
to plot the value on one figure.
But, I have another 3D array value_2
which depends on lon
, lat
and pressure
(levels of pressure).
If I want to show the profile (depends on value_2
and pressure
) and coordinate like this: (-120,20)
when the mouse hover or click on one grid(lon,lat), how could I make it?
Here's the example of plotting pseudocolor plot
and profile plot
:
import numpy as np
import matplotlib.pyplot as plt
# coordination
lon = np.arange(-120,-110,1)
lat = np.arange(20,30,1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9,9)
pressure = np.arange(1110,500,-100)
lon,lat = np.meshgrid(lon,lat)
plt.pcolormesh(lon,lat,value_1)
plt.colorbar()
plt.show()
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9,9,pressure.shape[0])
python matplotlib
I have a 2D array value_1
which depends on lon
(longitude) and lat
(latitude).
Now, I can use pcolormesh
to plot the value on one figure.
But, I have another 3D array value_2
which depends on lon
, lat
and pressure
(levels of pressure).
If I want to show the profile (depends on value_2
and pressure
) and coordinate like this: (-120,20)
when the mouse hover or click on one grid(lon,lat), how could I make it?
Here's the example of plotting pseudocolor plot
and profile plot
:
import numpy as np
import matplotlib.pyplot as plt
# coordination
lon = np.arange(-120,-110,1)
lat = np.arange(20,30,1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9,9)
pressure = np.arange(1110,500,-100)
lon,lat = np.meshgrid(lon,lat)
plt.pcolormesh(lon,lat,value_1)
plt.colorbar()
plt.show()
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9,9,pressure.shape[0])
python matplotlib
python matplotlib
asked Nov 23 '18 at 12:24
Xin ZhangXin Zhang
59311
59311
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I'm sure there a more efficient way to get the right indices when hovering over the pcolormesh, but this does the trick:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gs
from math import floor
# coordination
lon = np.arange(-120, -110, 1)
lat = np.arange(20, 30, 1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9, 9)
pressure = np.arange(1110, 500, -100)
mlon, mlat = np.meshgrid(lon, lat)
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9, 9, pressure.shape[0])
# global variables to keep track of which values
# are currently plotted in ax2
current_lat, curret_lon = None, None
fig, (ax1, ax2) = plt.subplots(2,1)
m = ax1.pcolormesh(mlon, mlat, value_1)
fig.colorbar(m, ax=ax1)
fig.tight_layout()
def on_move(event):
global current_lat, current_lon
if event.inaxes is ax1:
event_lat = floor(event.ydata)
event_lon = floor(event.xdata)
# find the indices corresponding to lat,lon
id_lat = np.searchsorted(lat, event_lat)
id_lon = np.searchsorted(lon, event_lon)
# only plot if we have different values than the previous plot
if id_lat != current_lat or id_lon != current_lon:
current_lat = id_lat
current_lon = id_lon
ax2.cla()
ax2.plot(value_2[id_lat, id_lon, :], pressure)
ax2.set_title("lat: {:.0f}, lon: {:.0f}".format(event_lat, event_lon))
fig.canvas.draw_idle()
cid = fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
Thank you! It works! But, 1. if the length oflon
andlat
are both3000
. It will be slower. 2. When zooming in, the profile won't change anymore.
– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you usefloor
which results in integer, there's no change when mouse moves in the integer . Thank you!
– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446684%2fpython-show-corresponding-profile-upon-hovering-clicking-over-a-grid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm sure there a more efficient way to get the right indices when hovering over the pcolormesh, but this does the trick:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gs
from math import floor
# coordination
lon = np.arange(-120, -110, 1)
lat = np.arange(20, 30, 1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9, 9)
pressure = np.arange(1110, 500, -100)
mlon, mlat = np.meshgrid(lon, lat)
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9, 9, pressure.shape[0])
# global variables to keep track of which values
# are currently plotted in ax2
current_lat, curret_lon = None, None
fig, (ax1, ax2) = plt.subplots(2,1)
m = ax1.pcolormesh(mlon, mlat, value_1)
fig.colorbar(m, ax=ax1)
fig.tight_layout()
def on_move(event):
global current_lat, current_lon
if event.inaxes is ax1:
event_lat = floor(event.ydata)
event_lon = floor(event.xdata)
# find the indices corresponding to lat,lon
id_lat = np.searchsorted(lat, event_lat)
id_lon = np.searchsorted(lon, event_lon)
# only plot if we have different values than the previous plot
if id_lat != current_lat or id_lon != current_lon:
current_lat = id_lat
current_lon = id_lon
ax2.cla()
ax2.plot(value_2[id_lat, id_lon, :], pressure)
ax2.set_title("lat: {:.0f}, lon: {:.0f}".format(event_lat, event_lon))
fig.canvas.draw_idle()
cid = fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
Thank you! It works! But, 1. if the length oflon
andlat
are both3000
. It will be slower. 2. When zooming in, the profile won't change anymore.
– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you usefloor
which results in integer, there's no change when mouse moves in the integer . Thank you!
– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
|
show 1 more comment
I'm sure there a more efficient way to get the right indices when hovering over the pcolormesh, but this does the trick:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gs
from math import floor
# coordination
lon = np.arange(-120, -110, 1)
lat = np.arange(20, 30, 1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9, 9)
pressure = np.arange(1110, 500, -100)
mlon, mlat = np.meshgrid(lon, lat)
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9, 9, pressure.shape[0])
# global variables to keep track of which values
# are currently plotted in ax2
current_lat, curret_lon = None, None
fig, (ax1, ax2) = plt.subplots(2,1)
m = ax1.pcolormesh(mlon, mlat, value_1)
fig.colorbar(m, ax=ax1)
fig.tight_layout()
def on_move(event):
global current_lat, current_lon
if event.inaxes is ax1:
event_lat = floor(event.ydata)
event_lon = floor(event.xdata)
# find the indices corresponding to lat,lon
id_lat = np.searchsorted(lat, event_lat)
id_lon = np.searchsorted(lon, event_lon)
# only plot if we have different values than the previous plot
if id_lat != current_lat or id_lon != current_lon:
current_lat = id_lat
current_lon = id_lon
ax2.cla()
ax2.plot(value_2[id_lat, id_lon, :], pressure)
ax2.set_title("lat: {:.0f}, lon: {:.0f}".format(event_lat, event_lon))
fig.canvas.draw_idle()
cid = fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
Thank you! It works! But, 1. if the length oflon
andlat
are both3000
. It will be slower. 2. When zooming in, the profile won't change anymore.
– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you usefloor
which results in integer, there's no change when mouse moves in the integer . Thank you!
– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
|
show 1 more comment
I'm sure there a more efficient way to get the right indices when hovering over the pcolormesh, but this does the trick:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gs
from math import floor
# coordination
lon = np.arange(-120, -110, 1)
lat = np.arange(20, 30, 1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9, 9)
pressure = np.arange(1110, 500, -100)
mlon, mlat = np.meshgrid(lon, lat)
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9, 9, pressure.shape[0])
# global variables to keep track of which values
# are currently plotted in ax2
current_lat, curret_lon = None, None
fig, (ax1, ax2) = plt.subplots(2,1)
m = ax1.pcolormesh(mlon, mlat, value_1)
fig.colorbar(m, ax=ax1)
fig.tight_layout()
def on_move(event):
global current_lat, current_lon
if event.inaxes is ax1:
event_lat = floor(event.ydata)
event_lon = floor(event.xdata)
# find the indices corresponding to lat,lon
id_lat = np.searchsorted(lat, event_lat)
id_lon = np.searchsorted(lon, event_lon)
# only plot if we have different values than the previous plot
if id_lat != current_lat or id_lon != current_lon:
current_lat = id_lat
current_lon = id_lon
ax2.cla()
ax2.plot(value_2[id_lat, id_lon, :], pressure)
ax2.set_title("lat: {:.0f}, lon: {:.0f}".format(event_lat, event_lon))
fig.canvas.draw_idle()
cid = fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
I'm sure there a more efficient way to get the right indices when hovering over the pcolormesh, but this does the trick:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gs
from math import floor
# coordination
lon = np.arange(-120, -110, 1)
lat = np.arange(20, 30, 1)
# shape of value_1: (lon,lat)
# pseudocolor plot
value_1 = np.random.rand(9, 9)
pressure = np.arange(1110, 500, -100)
mlon, mlat = np.meshgrid(lon, lat)
# shape of value_2: (lon,lat,pressure)
# profile plot
# Used to plot profile when mouse hovers on one grid
value_2 = np.random.rand(9, 9, pressure.shape[0])
# global variables to keep track of which values
# are currently plotted in ax2
current_lat, curret_lon = None, None
fig, (ax1, ax2) = plt.subplots(2,1)
m = ax1.pcolormesh(mlon, mlat, value_1)
fig.colorbar(m, ax=ax1)
fig.tight_layout()
def on_move(event):
global current_lat, current_lon
if event.inaxes is ax1:
event_lat = floor(event.ydata)
event_lon = floor(event.xdata)
# find the indices corresponding to lat,lon
id_lat = np.searchsorted(lat, event_lat)
id_lon = np.searchsorted(lon, event_lon)
# only plot if we have different values than the previous plot
if id_lat != current_lat or id_lon != current_lon:
current_lat = id_lat
current_lon = id_lon
ax2.cla()
ax2.plot(value_2[id_lat, id_lon, :], pressure)
ax2.set_title("lat: {:.0f}, lon: {:.0f}".format(event_lat, event_lon))
fig.canvas.draw_idle()
cid = fig.canvas.mpl_connect('motion_notify_event', on_move)
plt.show()
edited Nov 23 '18 at 14:10
answered Nov 23 '18 at 13:43
Diziet AsahiDiziet Asahi
9,14331731
9,14331731
Thank you! It works! But, 1. if the length oflon
andlat
are both3000
. It will be slower. 2. When zooming in, the profile won't change anymore.
– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you usefloor
which results in integer, there's no change when mouse moves in the integer . Thank you!
– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
|
show 1 more comment
Thank you! It works! But, 1. if the length oflon
andlat
are both3000
. It will be slower. 2. When zooming in, the profile won't change anymore.
– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you usefloor
which results in integer, there's no change when mouse moves in the integer . Thank you!
– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
Thank you! It works! But, 1. if the length of
lon
and lat
are both 3000
. It will be slower. 2. When zooming in, the profile won't change anymore.– Xin Zhang
Nov 23 '18 at 14:32
Thank you! It works! But, 1. if the length of
lon
and lat
are both 3000
. It will be slower. 2. When zooming in, the profile won't change anymore.– Xin Zhang
Nov 23 '18 at 14:32
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
To make it somewhat faster, you could replace the "motion_notify_event" by a "button_release_event", that way the calculations are only performed when you click, instead of everytime the mouse moves. About the zoom, I don't know what you mean. The code still works fine if I zoom in on the top axes on my computer
– Diziet Asahi
Nov 23 '18 at 14:55
Oh, I see .... Because you use
floor
which results in integer, there's no change when mouse moves in the integer . Thank you!– Xin Zhang
Nov 23 '18 at 15:06
Oh, I see .... Because you use
floor
which results in integer, there's no change when mouse moves in the integer . Thank you!– Xin Zhang
Nov 23 '18 at 15:06
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
If lon and lat are irregular decimals, it seems hard to make it work.
– Xin Zhang
Nov 23 '18 at 15:26
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
@Diziet Asahi this is a perfect example of how to handle events. Saving for later.
– Guimoute
Nov 23 '18 at 16:09
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446684%2fpython-show-corresponding-profile-upon-hovering-clicking-over-a-grid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dD33JwMgo02 sjs moKPt7B6kE0bZyardEIv6PfPpsj 8F,H4OhudbnMyZhFgFd21kzk FvTPGK26VCMK,tAjy6jh,IG,vW 9tykFeFxQ